Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Reflection / Cache / InternalCache.cs / 1305376 / InternalCache.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: InternalCache ** ** ** Purpose: A high-performance internal caching class. All key ** lookups are done on the basis of the CacheObjType enum. The ** cache is limited to one object of any particular type per ** instance. ** ** ============================================================*/ using System; using System.Threading; using System.Diagnostics; using System.Runtime.CompilerServices; namespace System.Reflection.Cache { [Serializable] internal enum CacheAction { AllocateCache = 1, AddItem = 2, ClearCache = 3, LookupItemHit = 4, LookupItemMiss = 5, GrowCache = 6, SetItemReplace = 7, ReplaceFailed = 8 } [Serializable] internal class InternalCache { private const int MinCacheSize = 2; //We'll start the cache as null and only grow it as we need it. private InternalCacheItem[] m_cache=null; private int m_numItems = 0; // private bool m_copying = false; //Knowing the name of the cache is very useful for debugging, but we don't //want to take the working-set hit in the debug build. We'll only include //the field in that build. #if _LOGGING private String m_cacheName; #endif internal InternalCache(String cacheName) { #if _LOGGING m_cacheName = cacheName; #endif //We won't allocate any items until the first time that they're requested. } internal Object this[CacheObjType cacheType] { [System.Security.SecurityCritical] // auto-generated get { //Let's snapshot a reference to the array up front so that //we don't have to worry about any writers. It's important //to grab the cache first and then numItems. In the event that //the cache gets cleared, m_numItems will be set to 0 before //we actually release the cache. Getting it second will cause //us to walk only 0 elements, but not to fault. InternalCacheItem[] cache = m_cache; int numItems = m_numItems; int position = FindObjectPosition(cache, numItems, cacheType, false); if (position>=0) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemHit, cacheType, cache[position].Value); return cache[position].Value; } //Couldn't find it -- oh, well. if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemMiss, cacheType); return null; } [System.Security.SecurityCritical] // auto-generated set { int position; if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AddItem, cacheType, value); lock(this) { position = FindObjectPosition(m_cache, m_numItems, cacheType, true); if (position>0) { m_cache[position].Value = value; m_cache[position].Key = cacheType; if (position==m_numItems) { m_numItems++; } return; } if (m_cache==null) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AllocateCache, cacheType); // m_copying = true; m_cache = new InternalCacheItem[MinCacheSize]; m_cache[0].Value = value; m_cache[0].Key = cacheType; m_numItems = 1; // m_copying = false; } else { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.GrowCache, cacheType); // m_copying = true; InternalCacheItem[] tempCache = new InternalCacheItem[m_numItems * 2]; for (int i=0; icache.Length) { itemCount = cache.Length; } for (int i=0; i =0) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemHit, cacheType, cache[position].Value); return cache[position].Value; } //Couldn't find it -- oh, well. if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemMiss, cacheType); return null; } [System.Security.SecurityCritical] // auto-generated set { int position; if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AddItem, cacheType, value); lock(this) { position = FindObjectPosition(m_cache, m_numItems, cacheType, true); if (position>0) { m_cache[position].Value = value; m_cache[position].Key = cacheType; if (position==m_numItems) { m_numItems++; } return; } if (m_cache==null) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AllocateCache, cacheType); // m_copying = true; m_cache = new InternalCacheItem[MinCacheSize]; m_cache[0].Value = value; m_cache[0].Key = cacheType; m_numItems = 1; // m_copying = false; } else { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.GrowCache, cacheType); // m_copying = true; InternalCacheItem[] tempCache = new InternalCacheItem[m_numItems * 2]; for (int i=0; i cache.Length) { itemCount = cache.Length; } for (int i=0; i
Link Menu
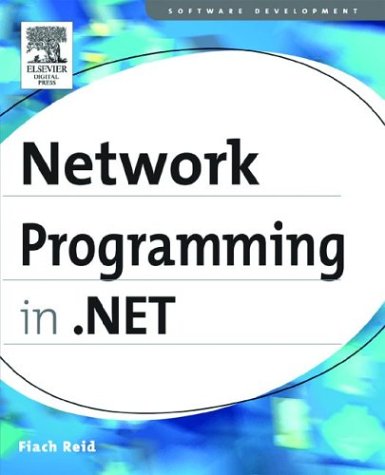
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DateTimeFormatInfo.cs
- MarkupWriter.cs
- HtmlListAdapter.cs
- CompilerInfo.cs
- DropShadowEffect.cs
- CacheDict.cs
- TextTabProperties.cs
- PieceNameHelper.cs
- ConfigErrorGlyph.cs
- ProcessMonitor.cs
- AmbientValueAttribute.cs
- SafeNativeMethodsOther.cs
- ExpressionBuilderCollection.cs
- SqlUtil.cs
- TextEditorThreadLocalStore.cs
- Rights.cs
- MimeMapping.cs
- ConnectionStringSettings.cs
- TaiwanCalendar.cs
- TreeView.cs
- Ticks.cs
- PageThemeParser.cs
- PngBitmapDecoder.cs
- LinkUtilities.cs
- ServiceMetadataBehavior.cs
- WebPartZoneDesigner.cs
- TranslateTransform.cs
- LineSegment.cs
- MemoryRecordBuffer.cs
- IdentityNotMappedException.cs
- RawStylusInputCustomDataList.cs
- TextureBrush.cs
- Stream.cs
- _NestedMultipleAsyncResult.cs
- XamlSerializer.cs
- SystemIPGlobalProperties.cs
- Sql8ExpressionRewriter.cs
- Dispatcher.cs
- DefaultParameterValueAttribute.cs
- RadioButtonRenderer.cs
- ListDictionaryInternal.cs
- HtmlInputFile.cs
- SafeFindHandle.cs
- EntitySqlQueryCacheEntry.cs
- FixedTextBuilder.cs
- ListSortDescriptionCollection.cs
- BitmapVisualManager.cs
- PersonalizableTypeEntry.cs
- Blend.cs
- MessageHeaderDescriptionCollection.cs
- FieldMetadata.cs
- SubqueryRules.cs
- IPAddress.cs
- ExpressionBinding.cs
- PeerMaintainer.cs
- ControlBuilderAttribute.cs
- DynamicFilter.cs
- AssociationType.cs
- XamlSerializerUtil.cs
- DllNotFoundException.cs
- RoutedCommand.cs
- SiteMap.cs
- StorageEntityTypeMapping.cs
- TypeSystem.cs
- TabletCollection.cs
- StringConverter.cs
- ListViewPagedDataSource.cs
- LineInfo.cs
- DataRelation.cs
- WindowsScroll.cs
- PlaceHolder.cs
- GeneralTransform3DTo2DTo3D.cs
- MergeLocalizationDirectives.cs
- XmlSchemaObjectTable.cs
- RowUpdatedEventArgs.cs
- ImageCodecInfo.cs
- FlatButtonAppearance.cs
- ColumnResult.cs
- Hyperlink.cs
- PageParser.cs
- Configuration.cs
- InstanceCompleteException.cs
- PropertyMetadata.cs
- AppDomain.cs
- BindingGroup.cs
- MetafileHeader.cs
- Action.cs
- SimpleFileLog.cs
- DesignerActionVerbItem.cs
- WebPartMinimizeVerb.cs
- PropertyDescriptorGridEntry.cs
- TextFindEngine.cs
- CompilerTypeWithParams.cs
- DataErrorValidationRule.cs
- SqlErrorCollection.cs
- DataGridViewDesigner.cs
- RoleServiceManager.cs
- EdmToObjectNamespaceMap.cs
- TaskHelper.cs
- NamespaceTable.cs