Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / TreeChangeInfo.cs / 1 / TreeChangeInfo.cs
//---------------------------------------------------------------------------- // // File: TreeChangeInfo.cs // // Description: // This data-structure is used // 1. As the data that is passed around by the DescendentsWalker // during an AncestorChanged tree-walk. // // Copyright (C) by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Media; using System.Windows.Controls; using System.Windows.Documents; using MS.Internal; using MS.Utility; namespace System.Windows { ////// This is the data that is passed through the DescendentsWalker /// during an AncestorChange tree-walk. /// internal struct TreeChangeInfo { #region Properties public TreeChangeInfo(DependencyObject root, DependencyObject parent, bool isAddOperation) { _rootOfChange = root; _isAddOperation = isAddOperation; _topmostCollapsedParentNode = null; _rootInheritableValues = null; _inheritablePropertiesStack = null; _valueIndexer = 0; // Create the InheritableProperties cache for the parent // and push it to start the stack ... we don't need to // pop this when we're done because we'll be throing the // stack away at that point. InheritablePropertiesStack.Push(CreateParentInheritableProperties(root, parent, isAddOperation)); } // // This method // 1. Is called from AncestorChange InvalidateTree. // 2. It is used to create the InheritableProperties on the given node. // 3. It also accumulates oldValues for the inheritable properties that are about to be invalidated // internal FrugalObjectListCreateParentInheritableProperties( DependencyObject d, DependencyObject parent, bool isAddOperation) { Debug.Assert(d != null, "Must have non-null current node"); if (parent == null) { return new FrugalObjectList (0); } DependencyObjectType treeObjDOT = d.DependencyObjectType; // See if we have a cached value. EffectiveValueEntry[] parentEffectiveValues = null; uint parentEffectiveValuesCount = 0; uint inheritablePropertiesCount = 0; // If inheritable properties aren't cached on you then use the effective // values cache on the parent to discover those inherited properties that // may need to be invalidated on the children nodes. if (!parent.IsSelfInheritanceParent) { DependencyObject inheritanceParent = parent.InheritanceParent; if (inheritanceParent != null) { parentEffectiveValues = inheritanceParent.EffectiveValues; parentEffectiveValuesCount = inheritanceParent.EffectiveValuesCount; inheritablePropertiesCount = inheritanceParent.InheritableEffectiveValuesCount; } } else { parentEffectiveValues = parent.EffectiveValues; parentEffectiveValuesCount = parent.EffectiveValuesCount; inheritablePropertiesCount = parent.InheritableEffectiveValuesCount; } FrugalObjectList inheritableProperties = new FrugalObjectList ((int) inheritablePropertiesCount); if (inheritablePropertiesCount == 0) { return inheritableProperties; } _rootInheritableValues = new InheritablePropertyChangeInfo[(int) inheritablePropertiesCount]; int inheritableIndex = 0; FrameworkObject foParent = new FrameworkObject(parent); for (uint i=0; i /// This is a stack that is used during an AncestorChange operation. /// I holds the InheritableProperties cache on the parent nodes in /// the tree in the order of traversal. The top of the stack holds /// the cache for the immediate parent node. /// internal Stack > InheritablePropertiesStack { get { if (_inheritablePropertiesStack == null) { _inheritablePropertiesStack = new Stack >(1); } return _inheritablePropertiesStack; } } /// /// When we enter a Visibility=Collapsed subtree, we remember that node /// using this property. As we process the children of this collapsed /// node, we see this property as non-null and know we're collapsed. /// As we exit the subtree, this reference is nulled out which means /// we don't know whether we're in a collapsed tree and the optimizations /// based on Visibility=Collapsed are not valid and should not be used. /// internal object TopmostCollapsedParentNode { get { return _topmostCollapsedParentNode; } set { _topmostCollapsedParentNode = value; } } // Indicates if this is a add child tree operation internal bool IsAddOperation { get { return _isAddOperation; } } // This is the element at the root of the sub-tree that had a parent change. internal DependencyObject Root { get { return _rootOfChange; } } #endregion Properties #region Data private Stack> _inheritablePropertiesStack; private object _topmostCollapsedParentNode; private bool _isAddOperation; private DependencyObject _rootOfChange; private InheritablePropertyChangeInfo[] _rootInheritableValues; private int _valueIndexer; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TreeChangeInfo.cs // // Description: // This data-structure is used // 1. As the data that is passed around by the DescendentsWalker // during an AncestorChanged tree-walk. // // Copyright (C) by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Media; using System.Windows.Controls; using System.Windows.Documents; using MS.Internal; using MS.Utility; namespace System.Windows { /// /// This is the data that is passed through the DescendentsWalker /// during an AncestorChange tree-walk. /// internal struct TreeChangeInfo { #region Properties public TreeChangeInfo(DependencyObject root, DependencyObject parent, bool isAddOperation) { _rootOfChange = root; _isAddOperation = isAddOperation; _topmostCollapsedParentNode = null; _rootInheritableValues = null; _inheritablePropertiesStack = null; _valueIndexer = 0; // Create the InheritableProperties cache for the parent // and push it to start the stack ... we don't need to // pop this when we're done because we'll be throing the // stack away at that point. InheritablePropertiesStack.Push(CreateParentInheritableProperties(root, parent, isAddOperation)); } // // This method // 1. Is called from AncestorChange InvalidateTree. // 2. It is used to create the InheritableProperties on the given node. // 3. It also accumulates oldValues for the inheritable properties that are about to be invalidated // internal FrugalObjectListCreateParentInheritableProperties( DependencyObject d, DependencyObject parent, bool isAddOperation) { Debug.Assert(d != null, "Must have non-null current node"); if (parent == null) { return new FrugalObjectList (0); } DependencyObjectType treeObjDOT = d.DependencyObjectType; // See if we have a cached value. EffectiveValueEntry[] parentEffectiveValues = null; uint parentEffectiveValuesCount = 0; uint inheritablePropertiesCount = 0; // If inheritable properties aren't cached on you then use the effective // values cache on the parent to discover those inherited properties that // may need to be invalidated on the children nodes. if (!parent.IsSelfInheritanceParent) { DependencyObject inheritanceParent = parent.InheritanceParent; if (inheritanceParent != null) { parentEffectiveValues = inheritanceParent.EffectiveValues; parentEffectiveValuesCount = inheritanceParent.EffectiveValuesCount; inheritablePropertiesCount = inheritanceParent.InheritableEffectiveValuesCount; } } else { parentEffectiveValues = parent.EffectiveValues; parentEffectiveValuesCount = parent.EffectiveValuesCount; inheritablePropertiesCount = parent.InheritableEffectiveValuesCount; } FrugalObjectList inheritableProperties = new FrugalObjectList ((int) inheritablePropertiesCount); if (inheritablePropertiesCount == 0) { return inheritableProperties; } _rootInheritableValues = new InheritablePropertyChangeInfo[(int) inheritablePropertiesCount]; int inheritableIndex = 0; FrameworkObject foParent = new FrameworkObject(parent); for (uint i=0; i /// This is a stack that is used during an AncestorChange operation. /// I holds the InheritableProperties cache on the parent nodes in /// the tree in the order of traversal. The top of the stack holds /// the cache for the immediate parent node. /// internal Stack > InheritablePropertiesStack { get { if (_inheritablePropertiesStack == null) { _inheritablePropertiesStack = new Stack >(1); } return _inheritablePropertiesStack; } } /// /// When we enter a Visibility=Collapsed subtree, we remember that node /// using this property. As we process the children of this collapsed /// node, we see this property as non-null and know we're collapsed. /// As we exit the subtree, this reference is nulled out which means /// we don't know whether we're in a collapsed tree and the optimizations /// based on Visibility=Collapsed are not valid and should not be used. /// internal object TopmostCollapsedParentNode { get { return _topmostCollapsedParentNode; } set { _topmostCollapsedParentNode = value; } } // Indicates if this is a add child tree operation internal bool IsAddOperation { get { return _isAddOperation; } } // This is the element at the root of the sub-tree that had a parent change. internal DependencyObject Root { get { return _rootOfChange; } } #endregion Properties #region Data private Stack> _inheritablePropertiesStack; private object _topmostCollapsedParentNode; private bool _isAddOperation; private DependencyObject _rootOfChange; private InheritablePropertyChangeInfo[] _rootInheritableValues; private int _valueIndexer; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
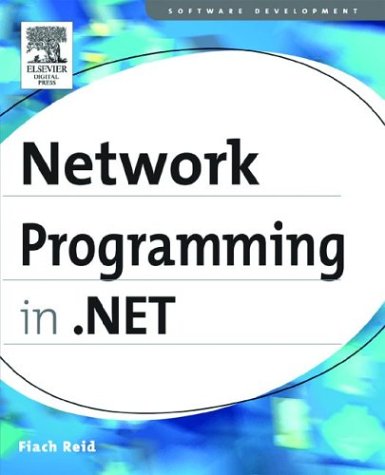
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DnsEndpointIdentity.cs
- DataListDesigner.cs
- AnnotationHighlightLayer.cs
- ExceptionRoutedEventArgs.cs
- EntityDataSourceWrapperCollection.cs
- WebPartZone.cs
- AVElementHelper.cs
- WindowsScrollBar.cs
- ParsedAttributeCollection.cs
- PresentationTraceSources.cs
- XPathItem.cs
- DocumentOutline.cs
- BinHexDecoder.cs
- StringUtil.cs
- VerificationException.cs
- Root.cs
- DesignTimeTemplateParser.cs
- WebRequest.cs
- GC.cs
- ObjectNotFoundException.cs
- FlagsAttribute.cs
- CommonXSendMessage.cs
- PersonalizableAttribute.cs
- ReaderOutput.cs
- webbrowsersite.cs
- SqlRecordBuffer.cs
- GridErrorDlg.cs
- DisableDpiAwarenessAttribute.cs
- SQLCharsStorage.cs
- LongSumAggregationOperator.cs
- ContextStaticAttribute.cs
- HyperlinkAutomationPeer.cs
- XmlSchemaParticle.cs
- OdbcFactory.cs
- SHA1.cs
- connectionpool.cs
- ImportCatalogPart.cs
- EventLogEntryCollection.cs
- Barrier.cs
- MenuEventArgs.cs
- EntitySqlQueryCacheKey.cs
- StorageEntitySetMapping.cs
- Types.cs
- DataGridViewCheckBoxCell.cs
- ServicesExceptionNotHandledEventArgs.cs
- OperatingSystem.cs
- DbConvert.cs
- _TransmitFileOverlappedAsyncResult.cs
- Int32RectValueSerializer.cs
- OptimizedTemplateContent.cs
- Vector3DValueSerializer.cs
- TraceUtils.cs
- ManagedWndProcTracker.cs
- XMLUtil.cs
- StackSpiller.cs
- ExtensibleClassFactory.cs
- MULTI_QI.cs
- ZipFileInfo.cs
- IndexedEnumerable.cs
- PropertyItem.cs
- RuntimeEnvironment.cs
- Point3D.cs
- ProcessManager.cs
- Preprocessor.cs
- Button.cs
- _TransmitFileOverlappedAsyncResult.cs
- MimeMultiPart.cs
- PenThreadWorker.cs
- ToolStripRenderer.cs
- Array.cs
- ObjectConverter.cs
- X509CertificateCollection.cs
- ScaleTransform.cs
- XmlName.cs
- StrokeNode.cs
- XsdDuration.cs
- ServiceBehaviorElementCollection.cs
- DesignTimeParseData.cs
- oledbmetadatacolumnnames.cs
- Pkcs7Signer.cs
- ServicesUtilities.cs
- _Win32.cs
- PrintControllerWithStatusDialog.cs
- SecurityKeyIdentifier.cs
- ValueUtilsSmi.cs
- ObjectListGeneralPage.cs
- HttpModuleCollection.cs
- FileLoadException.cs
- DoubleLinkList.cs
- ChangeConflicts.cs
- TypeForwardedToAttribute.cs
- DataIdProcessor.cs
- Wildcard.cs
- XmlWhitespace.cs
- FileStream.cs
- ByteFacetDescriptionElement.cs
- DayRenderEvent.cs
- OleDbPermission.cs
- CompiledQuery.cs
- ZipIOBlockManager.cs