Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / ImageInfo.cs / 1 / ImageInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Threading; using System; using System.Diagnostics; using System.Drawing.Imaging; ////// Animates one or more images that have time-based frames. /// This file contains the nested ImageInfo class - See ImageAnimator.cs for the definition of the outer class. /// public sealed partial class ImageAnimator { ////// ImageAnimator nested helper class used to store extra image state info. /// private class ImageInfo { const int PropertyTagFrameDelay = 0x5100; Image image; int frame; int frameCount; bool frameDirty; bool animated; EventHandler onFrameChangedHandler; int[] frameDelay; int frameTimer; ////// public ImageInfo(Image image) { this.image = image; animated = ImageAnimator.CanAnimate(image); if (animated) { frameCount = image.GetFrameCount(FrameDimension.Time); PropertyItem frameDelayItem = image.GetPropertyItem(PropertyTagFrameDelay); // If the image does not have a frame delay, we just return 0. // if (frameDelayItem != null) { // Convert the frame delay from byte[] to int // byte[] values = frameDelayItem.Value; Debug.Assert(values.Length == 4 * FrameCount, "PropertyItem has invalid value byte array"); frameDelay = new int[FrameCount]; for (int i=0; i < FrameCount; ++i) { frameDelay[i] = values[i * 4] + 256 * values[i * 4 + 1] + 256 * 256 * values[i * 4 + 2] + 256 * 256 * 256 * values[i * 4 + 3]; } } } else { frameCount = 1; } if (frameDelay == null) { frameDelay = new int[FrameCount]; } } ////// Whether the image supports animation. /// public bool Animated { get { return animated; } } ////// The current frame. /// public int Frame { get { return frame; } set { if (frame != value) { if (value < 0 || value >= FrameCount) { throw new ArgumentException(SR.GetString(SR.InvalidFrame), "value"); } if (Animated) { frame = value; frameDirty = true; OnFrameChanged(EventArgs.Empty); } } } } ////// The current frame has not been updated. /// public bool FrameDirty { get { return frameDirty; } } ////// public EventHandler FrameChangedHandler { get { return onFrameChangedHandler; } set { onFrameChangedHandler = value; } } ////// The number of frames in the image. /// public int FrameCount { get { return frameCount; } } ////// The delay associated with the frame at the specified index. /// public int FrameDelay(int frame) { return frameDelay[frame]; } ////// internal int FrameTimer { get { return frameTimer; } set { frameTimer = value; } } ////// The image this object wraps. /// internal Image Image { get { return image; } } ////// Selects the current frame as the active frame in the image. /// internal void UpdateFrame() { if (frameDirty) { image.SelectActiveFrame(FrameDimension.Time, Frame); frameDirty = false; } } ////// Raises the FrameChanged event. /// protected void OnFrameChanged(EventArgs e) { if( this.onFrameChangedHandler != null ){ this.onFrameChangedHandler(image, e); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
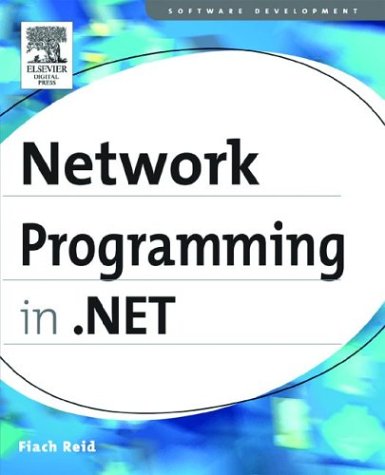
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CfgParser.cs
- ChangeBlockUndoRecord.cs
- FileUtil.cs
- DisplayNameAttribute.cs
- NativeMethods.cs
- BaseTemplateParser.cs
- KnownTypeDataContractResolver.cs
- OleTxTransactionInfo.cs
- CustomErrorCollection.cs
- XPathQueryGenerator.cs
- LoginName.cs
- VarRefManager.cs
- OleStrCAMarshaler.cs
- StrokeNodeOperations.cs
- OrderedParallelQuery.cs
- RuntimeIdentifierPropertyAttribute.cs
- MatcherBuilder.cs
- externdll.cs
- UnknownBitmapEncoder.cs
- Vector3DConverter.cs
- BindableTemplateBuilder.cs
- OutputCacheProfileCollection.cs
- SizeKeyFrameCollection.cs
- HostSecurityManager.cs
- CellRelation.cs
- WrappedKeySecurityTokenParameters.cs
- DynamicResourceExtensionConverter.cs
- HostedBindingBehavior.cs
- BidOverLoads.cs
- GenericUriParser.cs
- mediaclock.cs
- NameService.cs
- SchemaSetCompiler.cs
- ClusterSafeNativeMethods.cs
- XmlSchemaSimpleTypeRestriction.cs
- PersonalizationStateQuery.cs
- TimelineClockCollection.cs
- WebPartRestoreVerb.cs
- CommandHelpers.cs
- XmlMtomWriter.cs
- IgnoreFlushAndCloseStream.cs
- ClassDataContract.cs
- DataReceivedEventArgs.cs
- JavaScriptSerializer.cs
- DataGridCaption.cs
- RealizationDrawingContextWalker.cs
- RequestTimeoutManager.cs
- TextElementCollection.cs
- OleDbInfoMessageEvent.cs
- IntegerValidatorAttribute.cs
- CorrelationToken.cs
- HtmlTableCellCollection.cs
- DataListItemEventArgs.cs
- CombinedGeometry.cs
- EditCommandColumn.cs
- CodeCastExpression.cs
- ComponentCommands.cs
- DisplayInformation.cs
- GenerateHelper.cs
- TextWriterTraceListener.cs
- CapacityStreamGeometryContext.cs
- FilterableAttribute.cs
- LayoutTableCell.cs
- PropertyChange.cs
- ChildChangedEventArgs.cs
- Assign.cs
- BezierSegment.cs
- EncryptedData.cs
- HtmlTableRow.cs
- CapiSymmetricAlgorithm.cs
- LinearKeyFrames.cs
- QueryAsyncResult.cs
- ListBox.cs
- TextMarkerSource.cs
- NetNamedPipeBindingElement.cs
- XmlSerializerSection.cs
- documentsequencetextcontainer.cs
- Membership.cs
- XXXOnTypeBuilderInstantiation.cs
- EdmComplexPropertyAttribute.cs
- Metafile.cs
- AlphaSortedEnumConverter.cs
- SqlSupersetValidator.cs
- _HeaderInfoTable.cs
- InvalidFilterCriteriaException.cs
- Bezier.cs
- RootBrowserWindow.cs
- RegexNode.cs
- FieldToken.cs
- ForwardPositionQuery.cs
- AncillaryOps.cs
- HideDisabledControlAdapter.cs
- MdiWindowListItemConverter.cs
- SafeEventLogReadHandle.cs
- SkewTransform.cs
- ConfigurationCollectionAttribute.cs
- WeakReference.cs
- TypeListConverter.cs
- RelationshipDetailsRow.cs
- ErrorHandler.cs