Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / TableItemStyle.cs / 1 / TableItemStyle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.Web; using System.Web.Configuration; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class TableItemStyle : Style { ///Specifies the style of the table item. ////// internal const int PROP_HORZALIGN = 0x00010000; ///Specifies the horizontal alignment property. This field is /// constant. ////// internal const int PROP_VERTALIGN = 0x00020000; ///Specifies the vertical alignment property. This field is /// constant. ////// internal const int PROP_WRAP = 0x00040000; ///Specifies the /// wrap property. This field is constant. ////// public TableItemStyle() : base() { } ///Creates a new instance of the ///class. /// public TableItemStyle(StateBag bag) : base(bag) { } private bool EnableLegacyRendering { get { return (RuntimeConfig.GetAppConfig().XhtmlConformance.Mode == XhtmlConformanceMode.Legacy); } } ////// Creates a new instance of the ///class with the /// specified state bag. /// /// [ WebCategory("Layout"), DefaultValue(HorizontalAlign.NotSet), WebSysDescription(SR.TableItem_HorizontalAlign), NotifyParentProperty(true) ] public virtual HorizontalAlign HorizontalAlign { get { if (IsSet(PROP_HORZALIGN)) { return(HorizontalAlign)(ViewState["HorizontalAlign"]); } return HorizontalAlign.NotSet; } set { if (value < HorizontalAlign.NotSet || value > HorizontalAlign.Justify) { throw new ArgumentOutOfRangeException("value"); } ViewState["HorizontalAlign"] = value; SetBit(PROP_HORZALIGN); } } ///Gets or sets the horizontal alignment of the table item. ////// [ WebCategory("Layout"), DefaultValue(VerticalAlign.NotSet), WebSysDescription(SR.TableItem_VerticalAlign), NotifyParentProperty(true) ] public virtual VerticalAlign VerticalAlign { get { if (IsSet(PROP_VERTALIGN)) { return(VerticalAlign)(ViewState["VerticalAlign"]); } return VerticalAlign.NotSet; } set { if (value < VerticalAlign.NotSet || value > VerticalAlign.Bottom) { throw new ArgumentOutOfRangeException("value"); } ViewState["VerticalAlign"] = value; SetBit(PROP_VERTALIGN); } } ///Gets or sets the vertical alignment of the table item. ////// [ WebCategory("Layout"), DefaultValue(true), WebSysDescription(SR.TableItemStyle_Wrap), NotifyParentProperty(true) ] public virtual bool Wrap { get { if (IsSet(PROP_WRAP)) { return(bool)(ViewState["Wrap"]); } return true; } set { ViewState["Wrap"] = value; SetBit(PROP_WRAP); } } ///Gets or sets a value indicating whether the cell content wraps within the cell. ////// public override void AddAttributesToRender(HtmlTextWriter writer, WebControl owner) { base.AddAttributesToRender(writer, owner); if (!Wrap) { if (IsControlEnableLegacyRendering(owner)) { writer.AddAttribute(HtmlTextWriterAttribute.Nowrap, "nowrap"); } else { writer.AddStyleAttribute(HtmlTextWriterStyle.WhiteSpace, "nowrap"); } } HorizontalAlign hAlign = HorizontalAlign; if (hAlign != HorizontalAlign.NotSet) { TypeConverter hac = TypeDescriptor.GetConverter(typeof(HorizontalAlign)); writer.AddAttribute(HtmlTextWriterAttribute.Align, hac.ConvertToString(hAlign).ToLower(CultureInfo.InvariantCulture)); } VerticalAlign vAlign = VerticalAlign; if (vAlign != VerticalAlign.NotSet) { TypeConverter hac = TypeDescriptor.GetConverter(typeof(VerticalAlign)); writer.AddAttribute(HtmlTextWriterAttribute.Valign, hac.ConvertToString(vAlign).ToLower(CultureInfo.InvariantCulture)); } } ///Adds information about horizontal alignment, vertical alignment, and wrap to the list of attributes to render. ////// public override void CopyFrom(Style s) { if (s != null && !s.IsEmpty) { base.CopyFrom(s); if (s is TableItemStyle) { TableItemStyle ts = (TableItemStyle)s; if (s.RegisteredCssClass.Length != 0) { if (ts.IsSet(PROP_WRAP)) { ViewState.Remove("Wrap"); ClearBit(PROP_WRAP); } } else { if (ts.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_HORZALIGN)) this.HorizontalAlign = ts.HorizontalAlign; if (ts.IsSet(PROP_VERTALIGN)) this.VerticalAlign = ts.VerticalAlign; } } } private bool IsControlEnableLegacyRendering(Control control) { if (control != null) { return control.EnableLegacyRendering; } else { return EnableLegacyRendering; } } ///Copies non-blank elements from the specified style, overwriting existing /// style elements if necessary. ////// public override void MergeWith(Style s) { if (s != null && !s.IsEmpty) { if (IsEmpty) { // merge into an empty style is equivalent to a copy, which // is more efficient CopyFrom(s); return; } base.MergeWith(s); if (s is TableItemStyle) { TableItemStyle ts = (TableItemStyle)s; // Since we're already copying the registered CSS class in base.MergeWith, we don't // need to any attributes that would be included in that class. if (s.RegisteredCssClass.Length == 0) { if (ts.IsSet(PROP_WRAP) && !this.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_HORZALIGN) && !this.IsSet(PROP_HORZALIGN)) this.HorizontalAlign = ts.HorizontalAlign; if (ts.IsSet(PROP_VERTALIGN) && !this.IsSet(PROP_VERTALIGN)) this.VerticalAlign = ts.VerticalAlign; } } } ///Copies non-blank elements from the specified style, but will not overwrite /// any existing style elements. ////// public override void Reset() { if (IsSet(PROP_HORZALIGN)) ViewState.Remove("HorizontalAlign"); if (IsSet(PROP_VERTALIGN)) ViewState.Remove("VerticalAlign"); if (IsSet(PROP_WRAP)) ViewState.Remove("Wrap"); base.Reset(); } ////// Clears out any defined style elements from the state bag. /// ////// Only serialize if the Wrap property has changed. This means that we serialize "false" /// if they were set to false in the designer. /// private void ResetWrap() { ViewState.Remove("Wrap"); ClearBit(PROP_WRAP); } ////// Only serialize if the Wrap property has changed. This means that we serialize "false" /// if they were set to false in the designer. /// private bool ShouldSerializeWrap() { return IsSet(PROP_WRAP); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.Web; using System.Web.Configuration; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class TableItemStyle : Style { ///Specifies the style of the table item. ////// internal const int PROP_HORZALIGN = 0x00010000; ///Specifies the horizontal alignment property. This field is /// constant. ////// internal const int PROP_VERTALIGN = 0x00020000; ///Specifies the vertical alignment property. This field is /// constant. ////// internal const int PROP_WRAP = 0x00040000; ///Specifies the /// wrap property. This field is constant. ////// public TableItemStyle() : base() { } ///Creates a new instance of the ///class. /// public TableItemStyle(StateBag bag) : base(bag) { } private bool EnableLegacyRendering { get { return (RuntimeConfig.GetAppConfig().XhtmlConformance.Mode == XhtmlConformanceMode.Legacy); } } ////// Creates a new instance of the ///class with the /// specified state bag. /// /// [ WebCategory("Layout"), DefaultValue(HorizontalAlign.NotSet), WebSysDescription(SR.TableItem_HorizontalAlign), NotifyParentProperty(true) ] public virtual HorizontalAlign HorizontalAlign { get { if (IsSet(PROP_HORZALIGN)) { return(HorizontalAlign)(ViewState["HorizontalAlign"]); } return HorizontalAlign.NotSet; } set { if (value < HorizontalAlign.NotSet || value > HorizontalAlign.Justify) { throw new ArgumentOutOfRangeException("value"); } ViewState["HorizontalAlign"] = value; SetBit(PROP_HORZALIGN); } } ///Gets or sets the horizontal alignment of the table item. ////// [ WebCategory("Layout"), DefaultValue(VerticalAlign.NotSet), WebSysDescription(SR.TableItem_VerticalAlign), NotifyParentProperty(true) ] public virtual VerticalAlign VerticalAlign { get { if (IsSet(PROP_VERTALIGN)) { return(VerticalAlign)(ViewState["VerticalAlign"]); } return VerticalAlign.NotSet; } set { if (value < VerticalAlign.NotSet || value > VerticalAlign.Bottom) { throw new ArgumentOutOfRangeException("value"); } ViewState["VerticalAlign"] = value; SetBit(PROP_VERTALIGN); } } ///Gets or sets the vertical alignment of the table item. ////// [ WebCategory("Layout"), DefaultValue(true), WebSysDescription(SR.TableItemStyle_Wrap), NotifyParentProperty(true) ] public virtual bool Wrap { get { if (IsSet(PROP_WRAP)) { return(bool)(ViewState["Wrap"]); } return true; } set { ViewState["Wrap"] = value; SetBit(PROP_WRAP); } } ///Gets or sets a value indicating whether the cell content wraps within the cell. ////// public override void AddAttributesToRender(HtmlTextWriter writer, WebControl owner) { base.AddAttributesToRender(writer, owner); if (!Wrap) { if (IsControlEnableLegacyRendering(owner)) { writer.AddAttribute(HtmlTextWriterAttribute.Nowrap, "nowrap"); } else { writer.AddStyleAttribute(HtmlTextWriterStyle.WhiteSpace, "nowrap"); } } HorizontalAlign hAlign = HorizontalAlign; if (hAlign != HorizontalAlign.NotSet) { TypeConverter hac = TypeDescriptor.GetConverter(typeof(HorizontalAlign)); writer.AddAttribute(HtmlTextWriterAttribute.Align, hac.ConvertToString(hAlign).ToLower(CultureInfo.InvariantCulture)); } VerticalAlign vAlign = VerticalAlign; if (vAlign != VerticalAlign.NotSet) { TypeConverter hac = TypeDescriptor.GetConverter(typeof(VerticalAlign)); writer.AddAttribute(HtmlTextWriterAttribute.Valign, hac.ConvertToString(vAlign).ToLower(CultureInfo.InvariantCulture)); } } ///Adds information about horizontal alignment, vertical alignment, and wrap to the list of attributes to render. ////// public override void CopyFrom(Style s) { if (s != null && !s.IsEmpty) { base.CopyFrom(s); if (s is TableItemStyle) { TableItemStyle ts = (TableItemStyle)s; if (s.RegisteredCssClass.Length != 0) { if (ts.IsSet(PROP_WRAP)) { ViewState.Remove("Wrap"); ClearBit(PROP_WRAP); } } else { if (ts.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_HORZALIGN)) this.HorizontalAlign = ts.HorizontalAlign; if (ts.IsSet(PROP_VERTALIGN)) this.VerticalAlign = ts.VerticalAlign; } } } private bool IsControlEnableLegacyRendering(Control control) { if (control != null) { return control.EnableLegacyRendering; } else { return EnableLegacyRendering; } } ///Copies non-blank elements from the specified style, overwriting existing /// style elements if necessary. ////// public override void MergeWith(Style s) { if (s != null && !s.IsEmpty) { if (IsEmpty) { // merge into an empty style is equivalent to a copy, which // is more efficient CopyFrom(s); return; } base.MergeWith(s); if (s is TableItemStyle) { TableItemStyle ts = (TableItemStyle)s; // Since we're already copying the registered CSS class in base.MergeWith, we don't // need to any attributes that would be included in that class. if (s.RegisteredCssClass.Length == 0) { if (ts.IsSet(PROP_WRAP) && !this.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_HORZALIGN) && !this.IsSet(PROP_HORZALIGN)) this.HorizontalAlign = ts.HorizontalAlign; if (ts.IsSet(PROP_VERTALIGN) && !this.IsSet(PROP_VERTALIGN)) this.VerticalAlign = ts.VerticalAlign; } } } ///Copies non-blank elements from the specified style, but will not overwrite /// any existing style elements. ////// public override void Reset() { if (IsSet(PROP_HORZALIGN)) ViewState.Remove("HorizontalAlign"); if (IsSet(PROP_VERTALIGN)) ViewState.Remove("VerticalAlign"); if (IsSet(PROP_WRAP)) ViewState.Remove("Wrap"); base.Reset(); } ////// Clears out any defined style elements from the state bag. /// ////// Only serialize if the Wrap property has changed. This means that we serialize "false" /// if they were set to false in the designer. /// private void ResetWrap() { ViewState.Remove("Wrap"); ClearBit(PROP_WRAP); } ////// Only serialize if the Wrap property has changed. This means that we serialize "false" /// if they were set to false in the designer. /// private bool ShouldSerializeWrap() { return IsSet(PROP_WRAP); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
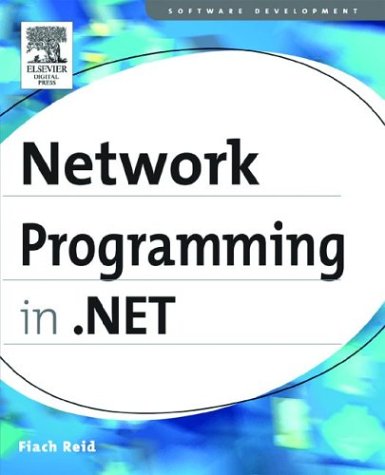
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeView.cs
- ButtonField.cs
- ObjectQueryProvider.cs
- ObjRef.cs
- Terminate.cs
- ConstraintStruct.cs
- SqlConnectionStringBuilder.cs
- TransferRequestHandler.cs
- shaperfactoryquerycacheentry.cs
- SqlFacetAttribute.cs
- x509utils.cs
- GenericUriParser.cs
- TwoPhaseCommitProxy.cs
- CodeVariableReferenceExpression.cs
- BookmarkNameHelper.cs
- Matrix.cs
- SequentialWorkflowRootDesigner.cs
- WorkflowEventArgs.cs
- BeginEvent.cs
- CursorConverter.cs
- BaseTransportHeaders.cs
- CharAnimationUsingKeyFrames.cs
- KeyPressEvent.cs
- ActivityInstance.cs
- WriteFileContext.cs
- Int32AnimationBase.cs
- HybridWebProxyFinder.cs
- ObjectPersistData.cs
- UniqueIdentifierService.cs
- ScrollChrome.cs
- JsonObjectDataContract.cs
- RemoteTokenFactory.cs
- ReferenceEqualityComparer.cs
- Animatable.cs
- ObjectContext.cs
- ProfilePropertyNameValidator.cs
- ExpandableObjectConverter.cs
- XamlTypeMapper.cs
- ClientBuildManagerTypeDescriptionProviderBridge.cs
- SoundPlayerAction.cs
- Rule.cs
- Descriptor.cs
- HitTestResult.cs
- ExpandedProjectionNode.cs
- SqlConnectionPoolGroupProviderInfo.cs
- DelegatingConfigHost.cs
- XsltConvert.cs
- AutoGeneratedField.cs
- XmlName.cs
- WebPartUserCapability.cs
- Simplifier.cs
- ResourceIDHelper.cs
- ListControlDesigner.cs
- DiscoveryInnerClientAdhocCD1.cs
- SemanticBasicElement.cs
- ProtectedConfigurationProviderCollection.cs
- SessionSymmetricTransportSecurityProtocolFactory.cs
- ContainerControl.cs
- ServicePoint.cs
- ApplicationInfo.cs
- HtmlInputFile.cs
- WindowsToolbar.cs
- AppDomainProtocolHandler.cs
- ClientScriptManagerWrapper.cs
- RootBrowserWindowAutomationPeer.cs
- DllNotFoundException.cs
- AppDomainProtocolHandler.cs
- XmlTextReaderImpl.cs
- ListViewUpdatedEventArgs.cs
- KeyPullup.cs
- SimpleRecyclingCache.cs
- SequentialUshortCollection.cs
- PngBitmapEncoder.cs
- CodePrimitiveExpression.cs
- ExpandCollapsePattern.cs
- QilStrConcatenator.cs
- _NegotiateClient.cs
- AppDomain.cs
- StringToken.cs
- JsonCollectionDataContract.cs
- ColorMap.cs
- DbConnectionHelper.cs
- ElementUtil.cs
- ReflectionTypeLoadException.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- MsmqSecureHashAlgorithm.cs
- WebExceptionStatus.cs
- ThreadAbortException.cs
- RelatedView.cs
- WindowsFormsSynchronizationContext.cs
- WebService.cs
- APCustomTypeDescriptor.cs
- SizeAnimation.cs
- MediaSystem.cs
- SrgsRule.cs
- TextChange.cs
- FixedSOMContainer.cs
- SqlAliasesReferenced.cs
- CheckableControlBaseAdapter.cs
- AssociatedControlConverter.cs