Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / NullableDecimalAverageAggregationOperator.cs / 1305376 / NullableDecimalAverageAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableDecimalAverageAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for Nullable decimals. /// internal sealed class NullableDecimalAverageAggregationOperator : InlinedAggregationOperator, decimal?> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal NullableDecimalAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override decimal? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // If the sequence was empty, return null right away. if (!enumerator.MoveNext()) { return null; } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableDecimalAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableDecimalAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableDecimalAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. decimal sum = 0.0m; long count = 0; QueryOperatorEnumerator source = m_source; decimal? current = default(decimal?); TKey currentKey = default(TKey); int i = 0; while (source.MoveNext(ref current, ref currentKey)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (current.HasValue) { sum += current.GetValueOrDefault(); count++; } } currentElement = new Pair (sum, count); return count > 0; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // NullableDecimalAverageAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for Nullable decimals. /// internal sealed class NullableDecimalAverageAggregationOperator : InlinedAggregationOperator, decimal?> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal NullableDecimalAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override decimal? InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // If the sequence was empty, return null right away. if (!enumerator.MoveNext()) { return null; } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new NullableDecimalAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class NullableDecimalAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal NullableDecimalAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. decimal sum = 0.0m; long count = 0; QueryOperatorEnumerator source = m_source; decimal? current = default(decimal?); TKey currentKey = default(TKey); int i = 0; while (source.MoveNext(ref current, ref currentKey)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (current.HasValue) { sum += current.GetValueOrDefault(); count++; } } currentElement = new Pair (sum, count); return count > 0; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
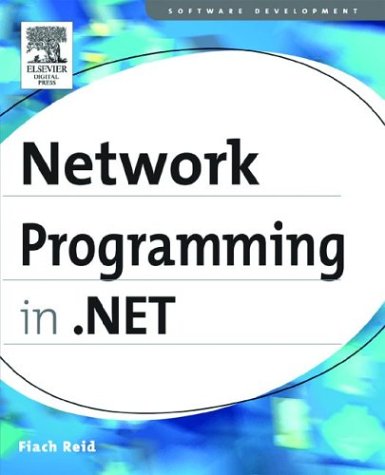
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReliableInputConnection.cs
- Model3D.cs
- NavigationHelper.cs
- HistoryEventArgs.cs
- DataServiceQueryOfT.cs
- HWStack.cs
- HostProtectionException.cs
- ResourceReferenceKeyNotFoundException.cs
- XmlCustomFormatter.cs
- ContractComponent.cs
- UserControlParser.cs
- EncodingFallbackAwareXmlTextWriter.cs
- SafeProcessHandle.cs
- TextPatternIdentifiers.cs
- URLAttribute.cs
- SqlUserDefinedTypeAttribute.cs
- XsltSettings.cs
- TypeGeneratedEventArgs.cs
- Graphics.cs
- StorageEntityTypeMapping.cs
- IdentityNotMappedException.cs
- EndpointDiscoveryMetadata11.cs
- AccessText.cs
- COM2PictureConverter.cs
- SelectionBorderGlyph.cs
- DynamicActivityProperty.cs
- PerfService.cs
- PolicyStatement.cs
- BevelBitmapEffect.cs
- BinaryOperationBinder.cs
- AppSettings.cs
- Single.cs
- UserNamePasswordServiceCredential.cs
- RemotingConfigParser.cs
- XmlSchemaAppInfo.cs
- PasswordTextNavigator.cs
- UInt32Converter.cs
- ObjectHelper.cs
- DataGridSortCommandEventArgs.cs
- FileDataSourceCache.cs
- Matrix3DConverter.cs
- SoapSchemaExporter.cs
- XamlGridLengthSerializer.cs
- XmlStringTable.cs
- FunctionDefinition.cs
- AlgoModule.cs
- PropertyIDSet.cs
- WeakRefEnumerator.cs
- RoutedPropertyChangedEventArgs.cs
- DiffuseMaterial.cs
- EntityDescriptor.cs
- HttpServerUtilityWrapper.cs
- ObjectMaterializedEventArgs.cs
- ServiceModelStringsVersion1.cs
- UpdateManifestForBrowserApplication.cs
- ImageKeyConverter.cs
- XsltArgumentList.cs
- UIElementParagraph.cs
- XmlDictionaryString.cs
- SortDescription.cs
- RelationshipWrapper.cs
- WindowsTokenRoleProvider.cs
- StretchValidation.cs
- CheckBoxBaseAdapter.cs
- BitmapImage.cs
- SapiInterop.cs
- StreamSecurityUpgradeProvider.cs
- ActivationServices.cs
- StringUtil.cs
- ThemeDirectoryCompiler.cs
- DataRecordObjectView.cs
- XmlAtomicValue.cs
- RedirectionProxy.cs
- DataGridViewCellValueEventArgs.cs
- ComplexObject.cs
- Environment.cs
- WmlValidationSummaryAdapter.cs
- FontCollection.cs
- SecurityException.cs
- ZipIOLocalFileHeader.cs
- OleStrCAMarshaler.cs
- WindowsIPAddress.cs
- WindowsRebar.cs
- NullRuntimeConfig.cs
- PersonalizationStateQuery.cs
- TraceContext.cs
- GZipDecoder.cs
- DoubleLinkListEnumerator.cs
- TextElementEnumerator.cs
- InvokeHandlers.cs
- SqlParameterizer.cs
- PluggableProtocol.cs
- StylusPointProperties.cs
- XamlSerializer.cs
- DSACryptoServiceProvider.cs
- CallContext.cs
- TimelineGroup.cs
- ChannelManagerService.cs
- ImpersonateTokenRef.cs
- ParenthesizePropertyNameAttribute.cs