Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / Transform3D.cs / 1305600 / Transform3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D transform implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/04/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using MS.Internal.Media3D; using MS.Internal.PresentationCore; using System; using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; namespace System.Windows.Media.Media3D { ////// 3D transformation. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // cannot be read & localized as string public abstract partial class Transform3D : GeneralTransform3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Prevent 3rd parties from extending this abstract base class. internal Transform3D() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Transforms the given point. /// /// Point to transform. ///Transformed point. public new Point3D Transform(Point3D point) { // this function is included due to forward compatability reasons return base.Transform(point); } ////// Transforms the given vector. /// /// Vector to transform. ///Transformed vector. public Vector3D Transform(Vector3D vector) { return Value.Transform(vector); } ////// Transforms the given point. /// /// Point to transform. ///Transformed point. public Point4D Transform(Point4D point) { return Value.Transform(point); } ////// Transforms the given list of points. /// /// List of points. public void Transform(Point3D[] points) { Value.Transform(points); } ////// Transforms the given list of vectors. /// /// List of vectors. public void Transform(Vector3D[] vectors) { Value.Transform(vectors); } ////// Transforms the given list of points. /// /// List of points. public void Transform(Point4D[] points) { Value.Transform(points); } ////// Transform a point /// /// Input point /// Output point ///True if the point was transformed successfuly, false otherwise public override bool TryTransform(Point3D inPoint, out Point3D result) { result = Value.Transform(inPoint); return true; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Bounding box ///The transformed bounding box public override Rect3D TransformBounds(Rect3D rect) { return M3DUtil.ComputeTransformedAxisAlignedBoundingBox(ref rect, this); } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform3D Inverse { get { ReadPreamble(); Matrix3D matrix = Value; if (!matrix.HasInverse) { return null; } matrix.Invert(); return new MatrixTransform3D(matrix); } } ////// Returns a best effort affine transform /// internal override Transform3D AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { return this; } } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Identity transformation. /// public static Transform3D Identity { get { // Make sure identity matrix is initialized. if (s_identity == null) { MatrixTransform3D identity = new MatrixTransform3D(); identity.Freeze(); s_identity = identity; } return s_identity; } } ////// Determines whether the matrix is affine. /// public abstract bool IsAffine {get;} ////// Return the current transformation value. /// public abstract Matrix3D Value { get; } #endregion Public Properties internal abstract void Append(ref Matrix3D matrix); //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private static Transform3D s_identity; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
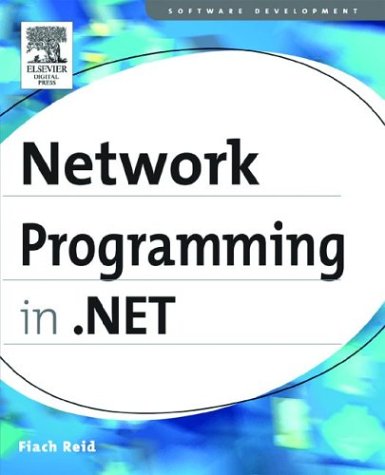
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLInt16.cs
- SettingsPropertyValueCollection.cs
- StackBuilderSink.cs
- DataObjectPastingEventArgs.cs
- SQLMoneyStorage.cs
- CategoryGridEntry.cs
- SamlAdvice.cs
- DoubleAnimation.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- RenderCapability.cs
- GridViewItemAutomationPeer.cs
- lengthconverter.cs
- PieceNameHelper.cs
- StrongTypingException.cs
- MergeFailedEvent.cs
- ListCommandEventArgs.cs
- ComboBox.cs
- WindowPattern.cs
- TimeIntervalCollection.cs
- EventKeyword.cs
- UpdateProgress.cs
- SiteMapPath.cs
- DataGridViewElement.cs
- ModulesEntry.cs
- WindowsListBox.cs
- FontDifferentiator.cs
- DataSourceHelper.cs
- WsiProfilesElementCollection.cs
- SQLByteStorage.cs
- TextEditorCharacters.cs
- FileDataSource.cs
- PixelShader.cs
- COMException.cs
- AttributeProviderAttribute.cs
- SafeRightsManagementPubHandle.cs
- AuthenticationModuleElementCollection.cs
- IdentityManager.cs
- ModifierKeysConverter.cs
- MeasureItemEvent.cs
- DependencySource.cs
- XComponentModel.cs
- TypeConverterHelper.cs
- PartitionResolver.cs
- ProtocolElementCollection.cs
- AdRotator.cs
- RenderCapability.cs
- GlyphRunDrawing.cs
- FormParameter.cs
- ActiveXSite.cs
- VideoDrawing.cs
- IsolationInterop.cs
- SynchronizationLockException.cs
- NetworkAddressChange.cs
- Psha1DerivedKeyGenerator.cs
- WebServiceEnumData.cs
- DataBindingCollectionEditor.cs
- XmlHierarchicalEnumerable.cs
- LineInfo.cs
- SelectionWordBreaker.cs
- DrawTreeNodeEventArgs.cs
- ControlDesigner.cs
- TextContainer.cs
- AttributeData.cs
- FormViewModeEventArgs.cs
- Rotation3DKeyFrameCollection.cs
- Page.cs
- CompositeFontFamily.cs
- CodeExpressionCollection.cs
- smtppermission.cs
- _NegoState.cs
- BigInt.cs
- ECDsaCng.cs
- ColumnCollectionEditor.cs
- ToolStripDropDownButton.cs
- ToolBar.cs
- ClosableStream.cs
- ToggleProviderWrapper.cs
- Gdiplus.cs
- ClrPerspective.cs
- TreeIterators.cs
- ControlPropertyNameConverter.cs
- CodeArrayIndexerExpression.cs
- TextElementEnumerator.cs
- MarkupExtensionSerializer.cs
- TransactionManagerProxy.cs
- SHA1Managed.cs
- WebDisplayNameAttribute.cs
- BinaryWriter.cs
- WindowsSpinner.cs
- PolygonHotSpot.cs
- WebRequest.cs
- RtfToXamlLexer.cs
- ExpressionCopier.cs
- DataGridViewCellEventArgs.cs
- StreamWriter.cs
- RegexWriter.cs
- DeferredTextReference.cs
- SQLByteStorage.cs
- ToolStripPanelRow.cs
- OrderedHashRepartitionStream.cs