Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / PtsHost / ListParagraph.cs / 1 / ListParagraph.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ListParagraph.cs // // Description: ListParagraph represents collection of list items. // // History: // 06/06/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // avoid generating warnings about unknown // message numbers and unknown pragmas for PRESharp contol using System; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using MS.Internal.Text; namespace MS.Internal.PtsHost { ////// ListParagraph represents collection of list items. /// internal sealed class ListParagraph : ContainerParagraph { ////// Constructor. /// /// /// Element associated with paragraph. /// /// /// Content's structural cache /// internal ListParagraph(DependencyObject element, StructuralCache structuralCache) : base(element, structuralCache) { } ////// CreateParaclient /// /// /// OUT: opaque to PTS paragraph client /// internal override void CreateParaclient( out IntPtr paraClientHandle) { #pragma warning disable 6518 // Disable PRESharp warning 6518. ListParaClient is an UnmamangedHandle, that adds itself // to HandleMapper that holds a reference to it. PTS manages lifetime of this object, and // calls DestroyParaclient to get rid of it. DestroyParaclient will call Dispose() on the object // and remove it from HandleMapper. ListParaClient paraClient = new ListParaClient(this); paraClientHandle = paraClient.Handle; #pragma warning restore 6518 } ////// Determine paragraph type at the current TextPointer and /// create it. Only ListItem elements are considered. Any other /// content is skipped. /// /// /// TextPointer at which paragraph is to be created /// /// /// True if empty paragraph is acceptable /// ////// BaseParagraph that was created /// protected override BaseParagraph GetParagraph(ITextPointer textPointer, bool fEmptyOk) { Invariant.Assert(textPointer is TextPointer); BaseParagraph paragraph = null; while (paragraph == null) { TextPointerContext runType = textPointer.GetPointerContext(LogicalDirection.Forward); if (runType == TextPointerContext.ElementStart) { TextElement element = ((TextPointer)textPointer).GetAdjacentElementFromOuterPosition(LogicalDirection.Forward); if (element is ListItem) { // paragraph = new ListItemParagraph(element, StructuralCache); break; } else if (element is List) { // paragraph = new ListParagraph(element, StructuralCache); break; } // Skip all elements, which are not valid list item children if (((TextPointer)textPointer).IsFrozen) { // Need to clone TextPointer before moving it. textPointer = textPointer.CreatePointer(); } textPointer.MoveToPosition(element.ElementEnd); } else if (runType == TextPointerContext.ElementEnd) { // End of list, if the same as Owner of associated element // Skip content otherwise if (Element == ((TextPointer)textPointer).Parent) { break; } if (((TextPointer)textPointer).IsFrozen) { // Need to clone TextPointer before moving it. textPointer = textPointer.CreatePointer(); } textPointer.MoveToNextContextPosition(LogicalDirection.Forward); } else { // Skip content if (((TextPointer)textPointer).IsFrozen) { // Need to clone TextPointer before moving it. textPointer = textPointer.CreatePointer(); } textPointer.MoveToNextContextPosition(LogicalDirection.Forward); } } if (paragraph != null) { StructuralCache.CurrentFormatContext.DependentMax = (TextPointer)textPointer; } return paragraph; } } } #pragma warning enable 1634, 1691 // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ListParagraph.cs // // Description: ListParagraph represents collection of list items. // // History: // 06/06/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // avoid generating warnings about unknown // message numbers and unknown pragmas for PRESharp contol using System; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using MS.Internal.Text; namespace MS.Internal.PtsHost { ////// ListParagraph represents collection of list items. /// internal sealed class ListParagraph : ContainerParagraph { ////// Constructor. /// /// /// Element associated with paragraph. /// /// /// Content's structural cache /// internal ListParagraph(DependencyObject element, StructuralCache structuralCache) : base(element, structuralCache) { } ////// CreateParaclient /// /// /// OUT: opaque to PTS paragraph client /// internal override void CreateParaclient( out IntPtr paraClientHandle) { #pragma warning disable 6518 // Disable PRESharp warning 6518. ListParaClient is an UnmamangedHandle, that adds itself // to HandleMapper that holds a reference to it. PTS manages lifetime of this object, and // calls DestroyParaclient to get rid of it. DestroyParaclient will call Dispose() on the object // and remove it from HandleMapper. ListParaClient paraClient = new ListParaClient(this); paraClientHandle = paraClient.Handle; #pragma warning restore 6518 } ////// Determine paragraph type at the current TextPointer and /// create it. Only ListItem elements are considered. Any other /// content is skipped. /// /// /// TextPointer at which paragraph is to be created /// /// /// True if empty paragraph is acceptable /// ////// BaseParagraph that was created /// protected override BaseParagraph GetParagraph(ITextPointer textPointer, bool fEmptyOk) { Invariant.Assert(textPointer is TextPointer); BaseParagraph paragraph = null; while (paragraph == null) { TextPointerContext runType = textPointer.GetPointerContext(LogicalDirection.Forward); if (runType == TextPointerContext.ElementStart) { TextElement element = ((TextPointer)textPointer).GetAdjacentElementFromOuterPosition(LogicalDirection.Forward); if (element is ListItem) { // paragraph = new ListItemParagraph(element, StructuralCache); break; } else if (element is List) { // paragraph = new ListParagraph(element, StructuralCache); break; } // Skip all elements, which are not valid list item children if (((TextPointer)textPointer).IsFrozen) { // Need to clone TextPointer before moving it. textPointer = textPointer.CreatePointer(); } textPointer.MoveToPosition(element.ElementEnd); } else if (runType == TextPointerContext.ElementEnd) { // End of list, if the same as Owner of associated element // Skip content otherwise if (Element == ((TextPointer)textPointer).Parent) { break; } if (((TextPointer)textPointer).IsFrozen) { // Need to clone TextPointer before moving it. textPointer = textPointer.CreatePointer(); } textPointer.MoveToNextContextPosition(LogicalDirection.Forward); } else { // Skip content if (((TextPointer)textPointer).IsFrozen) { // Need to clone TextPointer before moving it. textPointer = textPointer.CreatePointer(); } textPointer.MoveToNextContextPosition(LogicalDirection.Forward); } } if (paragraph != null) { StructuralCache.CurrentFormatContext.DependentMax = (TextPointer)textPointer; } return paragraph; } } } #pragma warning enable 1634, 1691 // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
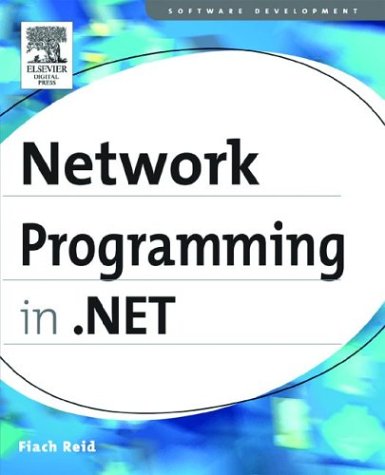
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlTableCell.cs
- WindowsRichEditRange.cs
- BinaryMethodMessage.cs
- ProcessThreadCollection.cs
- Mappings.cs
- LinearKeyFrames.cs
- DragStartedEventArgs.cs
- BinaryNode.cs
- EncodingTable.cs
- SchemaImporterExtensionElementCollection.cs
- SourceFileInfo.cs
- XamlReaderHelper.cs
- DataColumnChangeEvent.cs
- ContentPresenter.cs
- TextRangeEditLists.cs
- Label.cs
- GestureRecognitionResult.cs
- FlowDocumentPage.cs
- NamespaceInfo.cs
- LineSegment.cs
- KeyGesture.cs
- WriteableOnDemandPackagePart.cs
- DateTimeSerializationSection.cs
- BaseHashHelper.cs
- InProcStateClientManager.cs
- KeyValueInternalCollection.cs
- documentsequencetextpointer.cs
- MiniParameterInfo.cs
- TableLayoutStyle.cs
- ArgIterator.cs
- TemplateBindingExpression.cs
- TreeViewItemAutomationPeer.cs
- ThreadExceptionDialog.cs
- PersistChildrenAttribute.cs
- _NativeSSPI.cs
- NamespaceCollection.cs
- QuaternionAnimation.cs
- DBDataPermission.cs
- WorkflowRuntimeServiceElementCollection.cs
- VisualBrush.cs
- BitmapEffectDrawing.cs
- DataGridViewButtonCell.cs
- TrailingSpaceComparer.cs
- SchemaMapping.cs
- ToolStripSeparator.cs
- HandlerMappingMemo.cs
- ThreadAttributes.cs
- JoinTreeSlot.cs
- DirectoryGroupQuery.cs
- XmlHelper.cs
- AssemblyAttributesGoHere.cs
- ElementNotEnabledException.cs
- WebBrowserHelper.cs
- Empty.cs
- FrameworkRichTextComposition.cs
- BinaryCommonClasses.cs
- InfoCardTrace.cs
- SystemIPInterfaceStatistics.cs
- TemplateControlBuildProvider.cs
- ResourceAssociationSet.cs
- TransformerConfigurationWizardBase.cs
- RadioButtonPopupAdapter.cs
- SchemaNames.cs
- GridSplitter.cs
- RelationshipSet.cs
- xsdvalidator.cs
- LocationUpdates.cs
- XsltConvert.cs
- ImageIndexConverter.cs
- ObfuscateAssemblyAttribute.cs
- ListViewHitTestInfo.cs
- HostingPreferredMapPath.cs
- TextEffectResolver.cs
- DelegateHelpers.cs
- PersonalizableAttribute.cs
- ADMembershipProvider.cs
- PersonalizationAdministration.cs
- AbandonedMutexException.cs
- CreateUserWizardStep.cs
- DataGridItemEventArgs.cs
- WindowsHyperlink.cs
- BaseDataListComponentEditor.cs
- X509ScopedServiceCertificateElementCollection.cs
- XmlStreamNodeWriter.cs
- TextFragmentEngine.cs
- ConsumerConnectionPointCollection.cs
- ValidationRuleCollection.cs
- ObjectRef.cs
- SqlClientPermission.cs
- EditorZoneBase.cs
- LinkDescriptor.cs
- TemplateLookupAction.cs
- LeaseManager.cs
- DesigntimeLicenseContext.cs
- ServiceOperationViewControl.cs
- DesignerDataView.cs
- ReachFixedDocumentSerializer.cs
- LambdaCompiler.Logical.cs
- SqlUDTStorage.cs
- ConfigXmlElement.cs