Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Shared / MS / Internal / XmlHelper.cs / 1 / XmlHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements some helper functions for Xml nodes. // //--------------------------------------------------------------------------- using System; using System.Xml; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Internal { [FriendAccessAllowed] static class XmlHelper { ////// Return true if the given item is an XML node. /// internal static bool IsXmlNode(object item) { if (item != null) { Type type = item.GetType(); return type.FullName.StartsWith("System.Xml", StringComparison.Ordinal) && IsXmlNodeHelper(item); } else return false; } // separate function to avoid JIT-ing System.Xml until we have a good reason private static bool IsXmlNodeHelper(object item) { return item is System.Xml.XmlNode; } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query) { return SelectStringValue(node, query, null); } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query, XmlNamespaceManager namespaceManager) { string strValue; XmlNode result; result = node.SelectSingleNode(query, namespaceManager); if (result != null) { strValue = XmlHelper.ExtractString(result); } else { strValue = String.Empty; } return strValue; } ////// Get a string from an XmlNode (of any kind: element, attribute, etc.) /// internal static string ExtractString(XmlNode node) { string value = ""; if (node.NodeType == XmlNodeType.Element) { for (int i = 0; i < node.ChildNodes.Count; i++) { if (node.ChildNodes[i].NodeType == XmlNodeType.Text) { value += node.ChildNodes[i].Value; } } } else { value = node.Value; } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements some helper functions for Xml nodes. // //--------------------------------------------------------------------------- using System; using System.Xml; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Internal { [FriendAccessAllowed] static class XmlHelper { ////// Return true if the given item is an XML node. /// internal static bool IsXmlNode(object item) { if (item != null) { Type type = item.GetType(); return type.FullName.StartsWith("System.Xml", StringComparison.Ordinal) && IsXmlNodeHelper(item); } else return false; } // separate function to avoid JIT-ing System.Xml until we have a good reason private static bool IsXmlNodeHelper(object item) { return item is System.Xml.XmlNode; } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query) { return SelectStringValue(node, query, null); } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query, XmlNamespaceManager namespaceManager) { string strValue; XmlNode result; result = node.SelectSingleNode(query, namespaceManager); if (result != null) { strValue = XmlHelper.ExtractString(result); } else { strValue = String.Empty; } return strValue; } ////// Get a string from an XmlNode (of any kind: element, attribute, etc.) /// internal static string ExtractString(XmlNode node) { string value = ""; if (node.NodeType == XmlNodeType.Element) { for (int i = 0; i < node.ChildNodes.Count; i++) { if (node.ChildNodes[i].NodeType == XmlNodeType.Text) { value += node.ChildNodes[i].Value; } } } else { value = node.Value; } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
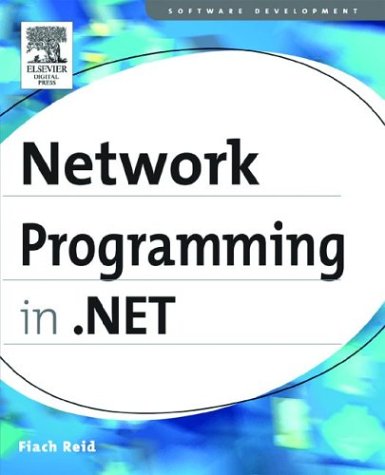
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSignificantWhitespace.cs
- MenuStrip.cs
- MembershipSection.cs
- XmlSchemaCompilationSettings.cs
- Table.cs
- IntranetCredentialPolicy.cs
- TreeChangeInfo.cs
- TypeDescriptionProviderAttribute.cs
- ClientSideProviderDescription.cs
- OleDbEnumerator.cs
- SiteMapDataSourceView.cs
- WizardPanel.cs
- TextElementAutomationPeer.cs
- RestClientProxyHandler.cs
- PageContent.cs
- WindowExtensionMethods.cs
- DisplayInformation.cs
- RemoteWebConfigurationHostStream.cs
- ToolStripSystemRenderer.cs
- ContainerParagraph.cs
- SetterBaseCollection.cs
- CultureTableRecord.cs
- MenuItem.cs
- DbProviderFactory.cs
- ImmutableAssemblyCacheEntry.cs
- Stackframe.cs
- StrokeFIndices.cs
- VisualTreeHelper.cs
- Peer.cs
- StrongNameIdentityPermission.cs
- XmlHelper.cs
- SqlMethods.cs
- SecurityToken.cs
- WorkflowNamespace.cs
- CodeEntryPointMethod.cs
- SocketStream.cs
- AnonymousIdentificationSection.cs
- IpcChannelHelper.cs
- TCPListener.cs
- CodeAttributeArgumentCollection.cs
- DbReferenceCollection.cs
- ACE.cs
- SqlBuffer.cs
- CustomBindingCollectionElement.cs
- _LoggingObject.cs
- ObjectCloneHelper.cs
- UserControlParser.cs
- XmlSchemaValidator.cs
- ToolStripSystemRenderer.cs
- ThumbAutomationPeer.cs
- SubclassTypeValidator.cs
- XmlSchemaInferenceException.cs
- TransformProviderWrapper.cs
- ComAdminInterfaces.cs
- ObjectListField.cs
- JoinElimination.cs
- UrlPath.cs
- DataGridBoolColumn.cs
- InternalSafeNativeMethods.cs
- __Error.cs
- TypeForwardedToAttribute.cs
- ComplexBindingPropertiesAttribute.cs
- BinHexEncoder.cs
- HttpServerVarsCollection.cs
- SignatureConfirmations.cs
- ParentQuery.cs
- EntityDesignerUtils.cs
- RegionData.cs
- OutputCacheModule.cs
- Floater.cs
- XmlC14NWriter.cs
- TextDecoration.cs
- SessionSwitchEventArgs.cs
- DerivedKeySecurityToken.cs
- AdapterUtil.cs
- ToolStripHighContrastRenderer.cs
- DataGridViewButtonCell.cs
- CharAnimationBase.cs
- DesignTimeParseData.cs
- GZipStream.cs
- IdentityHolder.cs
- ScrollEventArgs.cs
- HtmlMobileTextWriter.cs
- FlatButtonAppearance.cs
- EmptyControlCollection.cs
- TraceListener.cs
- RuntimeArgument.cs
- SimpleType.cs
- IntranetCredentialPolicy.cs
- NumericUpDownAccelerationCollection.cs
- XmlnsCompatibleWithAttribute.cs
- ParserOptions.cs
- OdbcConnectionPoolProviderInfo.cs
- OracleDataReader.cs
- DateTimeOffsetAdapter.cs
- RemoteCryptoDecryptRequest.cs
- UndirectedGraph.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- FormsIdentity.cs
- XamlFrame.cs