Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Automation / Peers / TextElementAutomationPeer.cs / 1 / TextElementAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TextElementAutomationPeer.cs // // Description: AutomationPeer associated with TextElements. // //--------------------------------------------------------------------------- using System.Collections; using System.Collections.Generic; // Listusing System.Security; // SecurityCritical using System.Windows.Documents; // ITextContainer using System.Windows.Media; // Geometry using System.Windows.Interop; // HwndSource using MS.Internal.Automation; // TextAdaptor using MS.Internal; using MS.Internal.Documents; // TextContainerHelper namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with TextElements. /// public class TextElementAutomationPeer : ContentTextAutomationPeer { ////// Constructor. /// /// Owner of the AutomationPeer. public TextElementAutomationPeer(TextElement owner) : base(owner) { } ////// ////// /// Since DocumentAutomationPeer gives access to its content through /// TextPattern, this method always returns null. /// protected override ListGetChildrenCore() { TextElement textElement = (TextElement)Owner; return TextContainerHelper.GetAutomationPeersFromRange(textElement.ContentStart, textElement.ContentEnd, null); } /// /// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Rect GetBoundingRectangleCore() { TextElement textElement = (TextElement)Owner; ITextView textView = textElement.TextContainer.TextView; if (textView == null || !textView.IsValid) { return Rect.Empty; } Geometry geometry = textView.GetTightBoundingGeometryFromTextPositions(textElement.ContentStart, textElement.ContentEnd); if (geometry != null) { PresentationSource presentationSource = PresentationSource.CriticalFromVisual(textView.RenderScope); if (presentationSource == null) { return Rect.Empty; } HwndSource hwndSource = presentationSource as HwndSource; // If the source isn't an HwnSource, there's not much we can do, return empty rect if (hwndSource == null) { return Rect.Empty; } Rect rectElement = geometry.Bounds; Rect rectRoot = PointUtil.ElementToRoot(rectElement, textView.RenderScope, presentationSource); Rect rectClient = PointUtil.RootToClient(rectRoot, presentationSource); Rect rectScreen = PointUtil.ClientToScreen(rectClient, hwndSource); return rectScreen; } else { return Rect.Empty; } } ////// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Point GetClickablePointCore() { Point pt = new Point(); TextElement textElement = (TextElement)Owner; ITextView textView = textElement.TextContainer.TextView; if (textView == null || !textView.IsValid || (!textView.Contains(textElement.ContentStart) && !textView.Contains(textElement.ContentEnd))) { return pt; } PresentationSource presentationSource = PresentationSource.CriticalFromVisual(textView.RenderScope); if (presentationSource == null) { return pt; } HwndSource hwndSource = presentationSource as HwndSource; // If the source isn't an HwnSource, there's not much we can do, return empty rect if (hwndSource == null) { return pt; } TextPointer endPosition = textElement.ContentStart.GetNextInsertionPosition(LogicalDirection.Forward); if (endPosition.CompareTo(textElement.ContentEnd) > 0) endPosition = textElement.ContentEnd; Rect rectElement = CalculateVisibleRect(textView, textElement, textElement.ContentStart, endPosition); Rect rectRoot = PointUtil.ElementToRoot(rectElement, textView.RenderScope, presentationSource); Rect rectClient = PointUtil.RootToClient(rectRoot, presentationSource); Rect rectScreen = PointUtil.ClientToScreen(rectClient, hwndSource); pt = new Point(rectScreen.Left + rectScreen.Width * 0.5, rectScreen.Top + rectScreen.Height * 0.5); return pt; } ////// ////// protected override bool IsOffscreenCore() { TextElement textElement = (TextElement)Owner; ITextView textView = textElement.TextContainer.TextView; if (textView == null || !textView.IsValid || (!textView.Contains(textElement.ContentStart) && !textView.Contains(textElement.ContentEnd))) { return true; } if (CalculateVisibleRect(textView, textElement, textElement.ContentStart, textElement.ContentEnd) == Rect.Empty) { return true; } return false; } /// /// Compute visible rectangle. /// private Rect CalculateVisibleRect(ITextView textView, TextElement textElement, TextPointer startPointer, TextPointer endPointer) { Geometry geometry = textView.GetTightBoundingGeometryFromTextPositions(startPointer, endPointer); Rect visibleRect = (geometry != null) ? geometry.Bounds : Rect.Empty; Visual visual = textView.RenderScope; while (visual != null && visibleRect != Rect.Empty) { if (VisualTreeHelper.GetClip(visual) != null) { GeneralTransform transform = textView.RenderScope.TransformToAncestor(visual).Inverse; // Safer version of transform to descendent (doing the inverse ourself), // we want the rect inside of our space. (Which is always rectangular and much nicer to work with) if (transform != null) { Rect rectBounds = VisualTreeHelper.GetClip(visual).Bounds; rectBounds = transform.TransformBounds(rectBounds); visibleRect.Intersect(rectBounds); } else { // No visibility if non-invertable transform exists. return Rect.Empty; } } visual = VisualTreeHelper.GetParent(visual) as Visual; } return visibleRect; } ////// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { // Force children connection to automation tree. GetChildren(); TextElement textElement = (TextElement)Owner; return TextContainerHelper.GetAutomationPeersFromRange(start, end, textElement.ContentStart); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TextElementAutomationPeer.cs // // Description: AutomationPeer associated with TextElements. // //--------------------------------------------------------------------------- using System.Collections; using System.Collections.Generic; // List using System.Security; // SecurityCritical using System.Windows.Documents; // ITextContainer using System.Windows.Media; // Geometry using System.Windows.Interop; // HwndSource using MS.Internal.Automation; // TextAdaptor using MS.Internal; using MS.Internal.Documents; // TextContainerHelper namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with TextElements. /// public class TextElementAutomationPeer : ContentTextAutomationPeer { ////// Constructor. /// /// Owner of the AutomationPeer. public TextElementAutomationPeer(TextElement owner) : base(owner) { } ////// ////// /// Since DocumentAutomationPeer gives access to its content through /// TextPattern, this method always returns null. /// protected override ListGetChildrenCore() { TextElement textElement = (TextElement)Owner; return TextContainerHelper.GetAutomationPeersFromRange(textElement.ContentStart, textElement.ContentEnd, null); } /// /// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Rect GetBoundingRectangleCore() { TextElement textElement = (TextElement)Owner; ITextView textView = textElement.TextContainer.TextView; if (textView == null || !textView.IsValid) { return Rect.Empty; } Geometry geometry = textView.GetTightBoundingGeometryFromTextPositions(textElement.ContentStart, textElement.ContentEnd); if (geometry != null) { PresentationSource presentationSource = PresentationSource.CriticalFromVisual(textView.RenderScope); if (presentationSource == null) { return Rect.Empty; } HwndSource hwndSource = presentationSource as HwndSource; // If the source isn't an HwnSource, there's not much we can do, return empty rect if (hwndSource == null) { return Rect.Empty; } Rect rectElement = geometry.Bounds; Rect rectRoot = PointUtil.ElementToRoot(rectElement, textView.RenderScope, presentationSource); Rect rectClient = PointUtil.RootToClient(rectRoot, presentationSource); Rect rectScreen = PointUtil.ClientToScreen(rectClient, hwndSource); return rectScreen; } else { return Rect.Empty; } } ////// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Point GetClickablePointCore() { Point pt = new Point(); TextElement textElement = (TextElement)Owner; ITextView textView = textElement.TextContainer.TextView; if (textView == null || !textView.IsValid || (!textView.Contains(textElement.ContentStart) && !textView.Contains(textElement.ContentEnd))) { return pt; } PresentationSource presentationSource = PresentationSource.CriticalFromVisual(textView.RenderScope); if (presentationSource == null) { return pt; } HwndSource hwndSource = presentationSource as HwndSource; // If the source isn't an HwnSource, there's not much we can do, return empty rect if (hwndSource == null) { return pt; } TextPointer endPosition = textElement.ContentStart.GetNextInsertionPosition(LogicalDirection.Forward); if (endPosition.CompareTo(textElement.ContentEnd) > 0) endPosition = textElement.ContentEnd; Rect rectElement = CalculateVisibleRect(textView, textElement, textElement.ContentStart, endPosition); Rect rectRoot = PointUtil.ElementToRoot(rectElement, textView.RenderScope, presentationSource); Rect rectClient = PointUtil.RootToClient(rectRoot, presentationSource); Rect rectScreen = PointUtil.ClientToScreen(rectClient, hwndSource); pt = new Point(rectScreen.Left + rectScreen.Width * 0.5, rectScreen.Top + rectScreen.Height * 0.5); return pt; } ////// ////// protected override bool IsOffscreenCore() { TextElement textElement = (TextElement)Owner; ITextView textView = textElement.TextContainer.TextView; if (textView == null || !textView.IsValid || (!textView.Contains(textElement.ContentStart) && !textView.Contains(textElement.ContentEnd))) { return true; } if (CalculateVisibleRect(textView, textElement, textElement.ContentStart, textElement.ContentEnd) == Rect.Empty) { return true; } return false; } /// /// Compute visible rectangle. /// private Rect CalculateVisibleRect(ITextView textView, TextElement textElement, TextPointer startPointer, TextPointer endPointer) { Geometry geometry = textView.GetTightBoundingGeometryFromTextPositions(startPointer, endPointer); Rect visibleRect = (geometry != null) ? geometry.Bounds : Rect.Empty; Visual visual = textView.RenderScope; while (visual != null && visibleRect != Rect.Empty) { if (VisualTreeHelper.GetClip(visual) != null) { GeneralTransform transform = textView.RenderScope.TransformToAncestor(visual).Inverse; // Safer version of transform to descendent (doing the inverse ourself), // we want the rect inside of our space. (Which is always rectangular and much nicer to work with) if (transform != null) { Rect rectBounds = VisualTreeHelper.GetClip(visual).Bounds; rectBounds = transform.TransformBounds(rectBounds); visibleRect.Intersect(rectBounds); } else { // No visibility if non-invertable transform exists. return Rect.Empty; } } visual = VisualTreeHelper.GetParent(visual) as Visual; } return visibleRect; } ////// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { // Force children connection to automation tree. GetChildren(); TextElement textElement = (TextElement)Owner; return TextContainerHelper.GetAutomationPeersFromRange(start, end, textElement.ContentStart); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
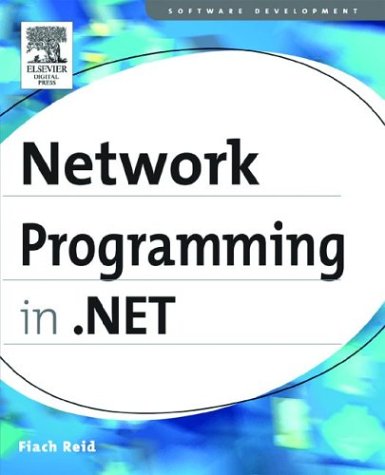
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CssTextWriter.cs
- BitmapEffect.cs
- ReadOnlyCollection.cs
- SQLInt32Storage.cs
- ListViewUpdatedEventArgs.cs
- MonthCalendar.cs
- FixedHighlight.cs
- UnsafeCollabNativeMethods.cs
- ObjectViewEntityCollectionData.cs
- Compiler.cs
- CodeVariableDeclarationStatement.cs
- ObjectConverter.cs
- BooleanProjectedSlot.cs
- PartialCachingAttribute.cs
- Matrix3D.cs
- ColorTypeConverter.cs
- DataDocumentXPathNavigator.cs
- HttpWrapper.cs
- HttpModuleAction.cs
- CaseInsensitiveComparer.cs
- TearOffProxy.cs
- ScrollPatternIdentifiers.cs
- TextEditorParagraphs.cs
- KeyboardDevice.cs
- TypeSystem.cs
- XmlAnyAttributeAttribute.cs
- ImageListImage.cs
- ByteStack.cs
- ClientApiGenerator.cs
- GenericRootAutomationPeer.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- SchemaElementDecl.cs
- DynamicValidatorEventArgs.cs
- OledbConnectionStringbuilder.cs
- DrawListViewSubItemEventArgs.cs
- TableFieldsEditor.cs
- MachineKeyValidationConverter.cs
- ClassDataContract.cs
- RuleSettingsCollection.cs
- CodeAssignStatement.cs
- LocatorBase.cs
- Oid.cs
- StrongBox.cs
- DomainUpDown.cs
- HttpsTransportBindingElement.cs
- XDRSchema.cs
- Compress.cs
- GuidelineCollection.cs
- ToolStripDropDownItemDesigner.cs
- wgx_sdk_version.cs
- DbBuffer.cs
- CoTaskMemHandle.cs
- FocusManager.cs
- AuthenticationModuleElementCollection.cs
- InvalidOperationException.cs
- RequiredAttributeAttribute.cs
- RelationshipDetailsRow.cs
- TreePrinter.cs
- Interlocked.cs
- SourceChangedEventArgs.cs
- VisualTarget.cs
- MimeFormatExtensions.cs
- adornercollection.cs
- XmlDigitalSignatureProcessor.cs
- AsyncStreamReader.cs
- PropertyValue.cs
- FunctionQuery.cs
- Predicate.cs
- safex509handles.cs
- SelectedCellsChangedEventArgs.cs
- ManipulationInertiaStartingEventArgs.cs
- TypeListConverter.cs
- ResourceManagerWrapper.cs
- PropertyPathWorker.cs
- TracingConnectionInitiator.cs
- ServiceDescriptions.cs
- PerformanceCounterLib.cs
- NativeObjectSecurity.cs
- WebZone.cs
- VirtualDirectoryMapping.cs
- ChangeInterceptorAttribute.cs
- TypeBuilder.cs
- ReferencedType.cs
- WebHttpEndpointElement.cs
- UnsafeNativeMethods.cs
- DBConnectionString.cs
- EntityKeyElement.cs
- OdbcConnectionStringbuilder.cs
- MethodRental.cs
- WeakReference.cs
- SerializationTrace.cs
- HijriCalendar.cs
- RIPEMD160.cs
- ApplicationException.cs
- NativeMethods.cs
- XamlReader.cs
- WebServiceBindingAttribute.cs
- FrameworkElementAutomationPeer.cs
- PageThemeParser.cs
- GZipStream.cs