Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Remoting / Channels / CORE / SocketStream.cs / 1305376 / SocketStream.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //========================================================================== // File: SocketStream.cs // // Summary: Stream used for reading from a socket by remoting channels. // //========================================================================= using System; using System.IO; using System.Runtime.Remoting; using System.Net; using System.Net.Sockets; namespace System.Runtime.Remoting.Channels { // Basically the same as NetworkStream, but adds support for timeouts. internal sealed class SocketStream : Stream { private Socket _socket; private int _timeout = 0; // throw timout exception if a read takes longer than this many milliseconds // as per http://support.microsoft.com/default.aspx?scid=kb%3ben-us%3b201213 // we shouldn't write more than 64K synchronously to a socket const int maxSocketWrite = 64 * 1024; // 64k const int maxSocketRead = 4 * 1024 * 1024; // 4 MB public SocketStream(Socket socket) { if (socket == null) throw new ArgumentNullException("socket"); _socket = socket; } // SocketStream // Stream implementation public override bool CanRead { get { return true; } } public override bool CanSeek { get { return false; } } public override bool CanWrite { get { return true; } } public override long Length { get { throw new NotSupportedException(); } } public override long Position { get { throw new NotSupportedException(); } set { throw new NotSupportedException(); } } // Position public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(); } public override int Read(byte[] buffer, int offset, int size) { if (_timeout <= 0) { return _socket.Receive(buffer, offset, Math.Min(size, maxSocketRead), SocketFlags.None); } else { IAsyncResult ar = _socket.BeginReceive(buffer, offset, Math.Min(size, maxSocketRead), SocketFlags.None, null, null); if (_timeout>0 && !ar.IsCompleted) { ar.AsyncWaitHandle.WaitOne(_timeout, false); if (!ar.IsCompleted) throw new RemotingTimeoutException(); } return _socket.EndReceive(ar); } } // Read public override void Write(byte[] buffer, int offset, int count) { int bytesToWrite = count; while (bytesToWrite > 0) { count = Math.Min(bytesToWrite, maxSocketWrite); _socket.Send(buffer, offset, count, SocketFlags.None); bytesToWrite -= count; offset += count; } } // Write protected override void Dispose(bool disposing) { try { if (disposing) _socket.Close(); } finally { base.Dispose(disposing); } } public override void Flush() {} public override IAsyncResult BeginRead( byte[] buffer, int offset, int size, AsyncCallback callback, Object state) { IAsyncResult asyncResult = _socket.BeginReceive( buffer, offset, Math.Min(size, maxSocketRead), SocketFlags.None, callback, state); return asyncResult; } // BeginRead public override int EndRead(IAsyncResult asyncResult) { return _socket.EndReceive(asyncResult); } // EndRead public override IAsyncResult BeginWrite( byte[] buffer, int offset, int size, AsyncCallback callback, Object state) { IAsyncResult asyncResult = _socket.BeginSend( buffer, offset, size, SocketFlags.None, callback, state); return asyncResult; } // BeginWrite public override void EndWrite(IAsyncResult asyncResult) { _socket.EndSend(asyncResult); } // EndWrite public override void SetLength(long value) { throw new NotSupportedException(); } } // class SocketStream } // namespace System.Runtime.Remoting.Channels // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
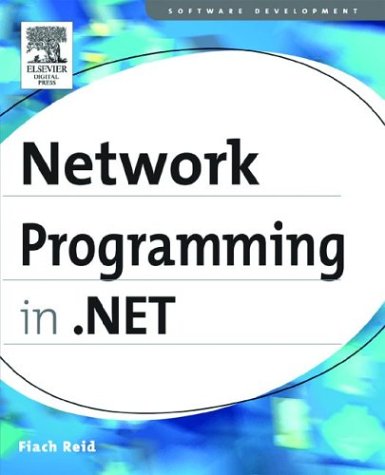
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmiEventStream.cs
- HtmlControl.cs
- XhtmlBasicLinkAdapter.cs
- ManagementObject.cs
- FieldNameLookup.cs
- ButtonBase.cs
- Executor.cs
- EncryptedPackageFilter.cs
- HWStack.cs
- ReadOnlyCollectionBase.cs
- Expression.cs
- CopyNamespacesAction.cs
- WebColorConverter.cs
- InfoCardCryptoHelper.cs
- xsdvalidator.cs
- XamlSerializer.cs
- TrustManager.cs
- ResourceReader.cs
- TypeDescriptionProvider.cs
- QilTypeChecker.cs
- XmlBinaryReader.cs
- StringUtil.cs
- ServiceDescriptionReflector.cs
- CodeTypeDelegate.cs
- XmlUnspecifiedAttribute.cs
- MemoryStream.cs
- MatrixIndependentAnimationStorage.cs
- XpsPartBase.cs
- XmlMemberMapping.cs
- ImmComposition.cs
- TreeIterator.cs
- EventRecordWrittenEventArgs.cs
- TimestampInformation.cs
- ComponentCommands.cs
- NotFiniteNumberException.cs
- RowToParametersTransformer.cs
- ExtentKey.cs
- ClientRequest.cs
- ContourSegment.cs
- ConfigXmlWhitespace.cs
- CachedBitmap.cs
- HandlerBase.cs
- DataServiceResponse.cs
- ControlParameter.cs
- SessionStateItemCollection.cs
- ObjectStateEntry.cs
- LinearGradientBrush.cs
- UndirectedGraph.cs
- ListViewCommandEventArgs.cs
- LinkedResource.cs
- SmtpTransport.cs
- ProcessManager.cs
- ToolStrip.cs
- XamlFilter.cs
- BaseProcessor.cs
- StringExpressionSet.cs
- SpeechEvent.cs
- CompatibleIComparer.cs
- FacetEnabledSchemaElement.cs
- Gdiplus.cs
- DesignerAttribute.cs
- CompilerInfo.cs
- EditingMode.cs
- TemplateBindingExpressionConverter.cs
- DetailsViewModeEventArgs.cs
- FirstMatchCodeGroup.cs
- DispatcherEventArgs.cs
- QuaternionAnimation.cs
- XamlSerializer.cs
- IfAction.cs
- DataGridViewCellStyleEditor.cs
- DataGridViewTextBoxCell.cs
- DiagnosticTrace.cs
- TextAction.cs
- PathFigureCollection.cs
- XmlAttributeCollection.cs
- JsonReaderDelegator.cs
- Thumb.cs
- SqlUDTStorage.cs
- LayoutManager.cs
- MatrixStack.cs
- _DisconnectOverlappedAsyncResult.cs
- Config.cs
- NumericUpDown.cs
- HtmlUtf8RawTextWriter.cs
- HighlightComponent.cs
- DeviceContext.cs
- CurrencyManager.cs
- ImpersonateTokenRef.cs
- ColorIndependentAnimationStorage.cs
- StyleTypedPropertyAttribute.cs
- PageRanges.cs
- SpStreamWrapper.cs
- DataTableClearEvent.cs
- TreeSet.cs
- OleDbWrapper.cs
- Int64AnimationBase.cs
- DbConnectionPoolGroupProviderInfo.cs
- _SafeNetHandles.cs
- Scanner.cs