Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / IO / Packaging / CompoundFile / VersionedStream.cs / 1 / VersionedStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class provides file versioning support for streams provided by // IDataTransform implementations. // // History: // 02/21/2006: BruceMac: Initial implementation. // //----------------------------------------------------------------------------- using System; using System.IO; // for Stream using System.Windows; // ExceptionStringTable using System.Globalization; // for CultureInfo namespace MS.Internal.IO.Packaging.CompoundFile { ////// Maintains a FormatVersion for this stream and any number of sibling streams that semantically /// share the same version information (which is only persisted in one of the streams). /// internal class VersionedStream : Stream { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Stream Methods ////// Return the bytes requested from the container /// public override int Read(byte[] buffer, int offset, int count) { CheckDisposed(); // ReadAttempt accepts an optional boolean. If this is true, that means // we are expecting a legal FormatVersion to exist and that it is readable by our // code version. We do not want to force this check if we are empty. _versionOwner.ReadAttempt(_stream.Length > 0); return _stream.Read(buffer, offset, count); } ////// Write /// public override void Write(byte[] buffer, int offset, int count) { CheckDisposed(); _versionOwner.WriteAttempt(); _stream.Write(buffer, offset, count); } ////// ReadByte /// public override int ReadByte() { CheckDisposed(); // ReadAttempt accepts an optional boolean. If this is true, that means // we are expecting a legal FormatVersion to exist and that it is readable by our // code version. We do not want to force this check if we are empty. _versionOwner.ReadAttempt(_stream.Length > 0); return _stream.ReadByte(); } ////// WriteByte /// public override void WriteByte(byte b) { CheckDisposed(); _versionOwner.WriteAttempt(); _stream.WriteByte(b); } ////// Seek /// /// offset /// origin ///zero public override long Seek(long offset, SeekOrigin origin) { CheckDisposed(); return _stream.Seek(offset, origin); } ////// SetLength /// public override void SetLength(long newLength) { CheckDisposed(); if (newLength < 0) throw new ArgumentOutOfRangeException("newLength"); _versionOwner.WriteAttempt(); _stream.SetLength(newLength); } ////// Flush /// public override void Flush() { CheckDisposed(); _stream.Flush(); } #endregion Stream Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Stream Properties ////// Current logical position within the stream /// public override long Position { get { CheckDisposed(); return _stream.Position; } set { Seek(value, SeekOrigin.Begin); } } ////// Length /// public override long Length { get { CheckDisposed(); return _stream.Length; } } ////// Is stream readable? /// ///returns false when called on disposed stream public override bool CanRead { get { return (_stream != null) && _stream.CanRead && _versionOwner.IsReadable; } } ////// Is stream seekable - should be handled by our owner /// ///returns false when called on disposed stream public override bool CanSeek { get { return (_stream != null) && _stream.CanSeek && _versionOwner.IsReadable; } } ////// Is stream writeable? /// ///returns false when called on disposed stream public override bool CanWrite { get { return (_stream != null) && _stream.CanWrite && _versionOwner.IsUpdatable; } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Constructor to use for any stream that shares versioning information with another stream /// but is not the one that houses the FormatVersion data itself. /// /// /// internal VersionedStream(Stream baseStream, VersionedStreamOwner versionOwner) { if (baseStream == null) throw new ArgumentNullException("baseStream"); if (versionOwner == null) throw new ArgumentNullException("versionOwner"); _stream = baseStream; _versionOwner = versionOwner; } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// Constructor for use by our subclass VersionedStreamOwner. /// /// protected VersionedStream(Stream baseStream) { if (baseStream == null) throw new ArgumentNullException("baseStream"); _stream = baseStream; // we are actually a VersionedStreamOwner _versionOwner = (VersionedStreamOwner)this; } ////// Sometimes our subclass needs to read/write directly to the stream /// ///Don't use CheckDisposed() here as we need to return null if we are disposed protected Stream BaseStream { get { return _stream; } } ////// Dispose(bool) /// /// protected override void Dispose(bool disposing) { try { if (disposing && (_stream != null)) { _stream.Close(); } } finally { _stream = null; base.Dispose(disposing); } } ////// Call this before accepting any public API call (except some Stream calls that /// are allowed to respond even when Closed /// protected void CheckDisposed() { if (_stream == null) throw new ObjectDisposedException(null, SR.Get(SRID.StreamObjectDisposed)); } //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- private VersionedStreamOwner _versionOwner; private Stream _stream; // null indicates Disposed state } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class provides file versioning support for streams provided by // IDataTransform implementations. // // History: // 02/21/2006: BruceMac: Initial implementation. // //----------------------------------------------------------------------------- using System; using System.IO; // for Stream using System.Windows; // ExceptionStringTable using System.Globalization; // for CultureInfo namespace MS.Internal.IO.Packaging.CompoundFile { ////// Maintains a FormatVersion for this stream and any number of sibling streams that semantically /// share the same version information (which is only persisted in one of the streams). /// internal class VersionedStream : Stream { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Stream Methods ////// Return the bytes requested from the container /// public override int Read(byte[] buffer, int offset, int count) { CheckDisposed(); // ReadAttempt accepts an optional boolean. If this is true, that means // we are expecting a legal FormatVersion to exist and that it is readable by our // code version. We do not want to force this check if we are empty. _versionOwner.ReadAttempt(_stream.Length > 0); return _stream.Read(buffer, offset, count); } ////// Write /// public override void Write(byte[] buffer, int offset, int count) { CheckDisposed(); _versionOwner.WriteAttempt(); _stream.Write(buffer, offset, count); } ////// ReadByte /// public override int ReadByte() { CheckDisposed(); // ReadAttempt accepts an optional boolean. If this is true, that means // we are expecting a legal FormatVersion to exist and that it is readable by our // code version. We do not want to force this check if we are empty. _versionOwner.ReadAttempt(_stream.Length > 0); return _stream.ReadByte(); } ////// WriteByte /// public override void WriteByte(byte b) { CheckDisposed(); _versionOwner.WriteAttempt(); _stream.WriteByte(b); } ////// Seek /// /// offset /// origin ///zero public override long Seek(long offset, SeekOrigin origin) { CheckDisposed(); return _stream.Seek(offset, origin); } ////// SetLength /// public override void SetLength(long newLength) { CheckDisposed(); if (newLength < 0) throw new ArgumentOutOfRangeException("newLength"); _versionOwner.WriteAttempt(); _stream.SetLength(newLength); } ////// Flush /// public override void Flush() { CheckDisposed(); _stream.Flush(); } #endregion Stream Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Stream Properties ////// Current logical position within the stream /// public override long Position { get { CheckDisposed(); return _stream.Position; } set { Seek(value, SeekOrigin.Begin); } } ////// Length /// public override long Length { get { CheckDisposed(); return _stream.Length; } } ////// Is stream readable? /// ///returns false when called on disposed stream public override bool CanRead { get { return (_stream != null) && _stream.CanRead && _versionOwner.IsReadable; } } ////// Is stream seekable - should be handled by our owner /// ///returns false when called on disposed stream public override bool CanSeek { get { return (_stream != null) && _stream.CanSeek && _versionOwner.IsReadable; } } ////// Is stream writeable? /// ///returns false when called on disposed stream public override bool CanWrite { get { return (_stream != null) && _stream.CanWrite && _versionOwner.IsUpdatable; } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Constructor to use for any stream that shares versioning information with another stream /// but is not the one that houses the FormatVersion data itself. /// /// /// internal VersionedStream(Stream baseStream, VersionedStreamOwner versionOwner) { if (baseStream == null) throw new ArgumentNullException("baseStream"); if (versionOwner == null) throw new ArgumentNullException("versionOwner"); _stream = baseStream; _versionOwner = versionOwner; } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// Constructor for use by our subclass VersionedStreamOwner. /// /// protected VersionedStream(Stream baseStream) { if (baseStream == null) throw new ArgumentNullException("baseStream"); _stream = baseStream; // we are actually a VersionedStreamOwner _versionOwner = (VersionedStreamOwner)this; } ////// Sometimes our subclass needs to read/write directly to the stream /// ///Don't use CheckDisposed() here as we need to return null if we are disposed protected Stream BaseStream { get { return _stream; } } ////// Dispose(bool) /// /// protected override void Dispose(bool disposing) { try { if (disposing && (_stream != null)) { _stream.Close(); } } finally { _stream = null; base.Dispose(disposing); } } ////// Call this before accepting any public API call (except some Stream calls that /// are allowed to respond even when Closed /// protected void CheckDisposed() { if (_stream == null) throw new ObjectDisposedException(null, SR.Get(SRID.StreamObjectDisposed)); } //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- private VersionedStreamOwner _versionOwner; private Stream _stream; // null indicates Disposed state } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
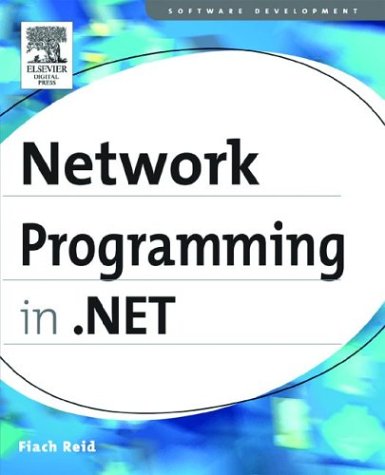
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Stylesheet.cs
- WebProxyScriptElement.cs
- HiddenFieldDesigner.cs
- EnvironmentPermission.cs
- CatalogPartCollection.cs
- BuildResultCache.cs
- EditorZone.cs
- ToolStripManager.cs
- ConnectionManagementSection.cs
- DisableDpiAwarenessAttribute.cs
- CodeDirectiveCollection.cs
- ManagedWndProcTracker.cs
- TextEncodedRawTextWriter.cs
- BoundColumn.cs
- TargetPerspective.cs
- CompiledELinqQueryState.cs
- XmlTextEncoder.cs
- CodeMethodInvokeExpression.cs
- EventLogTraceListener.cs
- DataGridViewColumnConverter.cs
- DataGridViewHeaderCell.cs
- SubclassTypeValidatorAttribute.cs
- XmlAggregates.cs
- WebZone.cs
- ResourceProviderFactory.cs
- ComponentSerializationService.cs
- DynamicRenderer.cs
- BitStack.cs
- DataServiceProviderMethods.cs
- DesignTimeType.cs
- KeyValueInternalCollection.cs
- UInt32Converter.cs
- UInt64Converter.cs
- StackOverflowException.cs
- WindowsClientElement.cs
- SafeRightsManagementPubHandle.cs
- CancellationTokenSource.cs
- HashHelper.cs
- ServiceHost.cs
- DiagnosticsConfigurationHandler.cs
- Membership.cs
- WebPartConnectVerb.cs
- AttachedAnnotation.cs
- smtppermission.cs
- LockedBorderGlyph.cs
- ResourceDescriptionAttribute.cs
- ToolboxComponentsCreatedEventArgs.cs
- XmlStreamStore.cs
- SingleResultAttribute.cs
- CompilationUtil.cs
- AbandonedMutexException.cs
- ColumnTypeConverter.cs
- DuplicateMessageDetector.cs
- PrintingPermission.cs
- LocatorBase.cs
- AppSettingsExpressionEditor.cs
- XmlAnyElementAttribute.cs
- SqlDataSourceDesigner.cs
- TypeToken.cs
- WebBrowserDesigner.cs
- Operators.cs
- ServiceAuthorizationBehavior.cs
- ActivityExecutionWorkItem.cs
- EntitySet.cs
- InvalidPrinterException.cs
- RawStylusInputReport.cs
- RegexRunner.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- DataGridViewRowEventArgs.cs
- Queue.cs
- TypeHelper.cs
- WrapPanel.cs
- DocumentPageViewAutomationPeer.cs
- GuidTagList.cs
- XmlSerializerAssemblyAttribute.cs
- DataStreams.cs
- TypedRowHandler.cs
- ReadOnlyDataSource.cs
- XmlUtilWriter.cs
- RouteValueExpressionBuilder.cs
- Span.cs
- smtppermission.cs
- TypeDefinition.cs
- MappingException.cs
- PathSegment.cs
- SingleObjectCollection.cs
- documentsequencetextview.cs
- CheckStoreFileValidityRequest.cs
- ListBindingConverter.cs
- MethodAccessException.cs
- MultiPageTextView.cs
- ReferenceEqualityComparer.cs
- InternalCache.cs
- DateTimeConstantAttribute.cs
- _UncName.cs
- COM2Enum.cs
- AddInDeploymentState.cs
- PointCollectionConverter.cs
- TypeElementCollection.cs
- dataprotectionpermission.cs