Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Configuration / System / Configuration / PropertyInformation.cs / 1 / PropertyInformation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration; using System.Collections.Specialized; using System.ComponentModel; using System.Collections; using System.Runtime.Serialization; namespace System.Configuration { // PropertyInformation // // Contains information about a property // public sealed class PropertyInformation { private ConfigurationElement ThisElement = null; private string PropertyName; private ConfigurationProperty _Prop = null; private const string LockAll = "*"; private ConfigurationProperty Prop { get { if (_Prop == null) { _Prop = ThisElement.Properties[PropertyName]; } return _Prop; } } internal PropertyInformation(ConfigurationElement thisElement, string propertyName) { PropertyName = propertyName; ThisElement = thisElement; } public string Name { get { return PropertyName; } } internal string ProvidedName { get { return Prop.ProvidedName; } } public object Value { get { return ThisElement[PropertyName]; } set { ThisElement[PropertyName] = value; } } // DefaultValue // // What is the default value for this property // public object DefaultValue { get { return Prop.DefaultValue; } } // ValueOrigin // // Where was the property retrieved from // public PropertyValueOrigin ValueOrigin { get { if (ThisElement.Values[PropertyName] == null) { return PropertyValueOrigin.Default; } if (ThisElement.Values.IsInherited(PropertyName)) { return PropertyValueOrigin.Inherited; } return PropertyValueOrigin.SetHere; } } // IsModified // // Was the property Modified // public bool IsModified { get { if (ThisElement.Values[PropertyName] == null) { return false; } if (ThisElement.Values.IsModified(PropertyName)) { return true; } return false; } } // IsKey // // Is this property a key? // public bool IsKey { get { return Prop.IsKey; } } // IsRequired // // Is this property required? // public bool IsRequired { get { return Prop.IsRequired; } } // IsLocked // // Is this property locked? // public bool IsLocked { get { return ((ThisElement.LockedAllExceptAttributesList != null && !ThisElement.LockedAllExceptAttributesList.DefinedInParent(PropertyName)) || (ThisElement.LockedAttributesList != null && (ThisElement.LockedAttributesList.DefinedInParent(PropertyName) || ThisElement.LockedAttributesList.DefinedInParent(LockAll))) || (((ThisElement.ItemLocked & ConfigurationValueFlags.Locked) != 0) && ((ThisElement.ItemLocked & ConfigurationValueFlags.Inherited) != 0))); } } // Source // // What is the source file where this data came from // public string Source { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return String.Empty; } return psi.FileName; } } // LineNumber // // What is the line number associated with the source // // Note: // 1 is the first line in the file. 0 is returned when there is no // source // public int LineNumber { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return 0; } return psi.LineNumber; } } // Type // // What is the type for the property // public Type Type { get { return Prop.Type; } } // Validator // public ConfigurationValidatorBase Validator { get { return Prop.Validator; } } // Converter // public TypeConverter Converter { get { return Prop.Converter; } } // Property description ( comments etc ) public string Description { get { return Prop.Description; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration; using System.Collections.Specialized; using System.ComponentModel; using System.Collections; using System.Runtime.Serialization; namespace System.Configuration { // PropertyInformation // // Contains information about a property // public sealed class PropertyInformation { private ConfigurationElement ThisElement = null; private string PropertyName; private ConfigurationProperty _Prop = null; private const string LockAll = "*"; private ConfigurationProperty Prop { get { if (_Prop == null) { _Prop = ThisElement.Properties[PropertyName]; } return _Prop; } } internal PropertyInformation(ConfigurationElement thisElement, string propertyName) { PropertyName = propertyName; ThisElement = thisElement; } public string Name { get { return PropertyName; } } internal string ProvidedName { get { return Prop.ProvidedName; } } public object Value { get { return ThisElement[PropertyName]; } set { ThisElement[PropertyName] = value; } } // DefaultValue // // What is the default value for this property // public object DefaultValue { get { return Prop.DefaultValue; } } // ValueOrigin // // Where was the property retrieved from // public PropertyValueOrigin ValueOrigin { get { if (ThisElement.Values[PropertyName] == null) { return PropertyValueOrigin.Default; } if (ThisElement.Values.IsInherited(PropertyName)) { return PropertyValueOrigin.Inherited; } return PropertyValueOrigin.SetHere; } } // IsModified // // Was the property Modified // public bool IsModified { get { if (ThisElement.Values[PropertyName] == null) { return false; } if (ThisElement.Values.IsModified(PropertyName)) { return true; } return false; } } // IsKey // // Is this property a key? // public bool IsKey { get { return Prop.IsKey; } } // IsRequired // // Is this property required? // public bool IsRequired { get { return Prop.IsRequired; } } // IsLocked // // Is this property locked? // public bool IsLocked { get { return ((ThisElement.LockedAllExceptAttributesList != null && !ThisElement.LockedAllExceptAttributesList.DefinedInParent(PropertyName)) || (ThisElement.LockedAttributesList != null && (ThisElement.LockedAttributesList.DefinedInParent(PropertyName) || ThisElement.LockedAttributesList.DefinedInParent(LockAll))) || (((ThisElement.ItemLocked & ConfigurationValueFlags.Locked) != 0) && ((ThisElement.ItemLocked & ConfigurationValueFlags.Inherited) != 0))); } } // Source // // What is the source file where this data came from // public string Source { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return String.Empty; } return psi.FileName; } } // LineNumber // // What is the line number associated with the source // // Note: // 1 is the first line in the file. 0 is returned when there is no // source // public int LineNumber { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return 0; } return psi.LineNumber; } } // Type // // What is the type for the property // public Type Type { get { return Prop.Type; } } // Validator // public ConfigurationValidatorBase Validator { get { return Prop.Validator; } } // Converter // public TypeConverter Converter { get { return Prop.Converter; } } // Property description ( comments etc ) public string Description { get { return Prop.Description; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
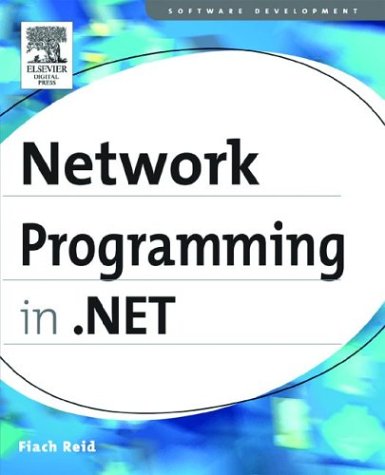
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IdentityModelDictionary.cs
- WmlListAdapter.cs
- TableLayoutPanel.cs
- XmlSchemaComplexContentRestriction.cs
- ListViewTableCell.cs
- CompiledAction.cs
- ContainsRowNumberChecker.cs
- ControlPropertyNameConverter.cs
- ClassicBorderDecorator.cs
- PeerUnsafeNativeMethods.cs
- Normalization.cs
- ConfigurationValue.cs
- safePerfProviderHandle.cs
- ComContractElement.cs
- FileDialogPermission.cs
- DbModificationCommandTree.cs
- FilteredAttributeCollection.cs
- TemplatedWizardStep.cs
- XmlSchemaSimpleType.cs
- DbMetaDataFactory.cs
- AmbientEnvironment.cs
- ToggleProviderWrapper.cs
- Section.cs
- DotExpr.cs
- URLBuilder.cs
- LocatorManager.cs
- WebEvents.cs
- Pair.cs
- SrgsRule.cs
- OperationCanceledException.cs
- DbConnectionHelper.cs
- ToolStripDropDownClosedEventArgs.cs
- PropertyDescriptorComparer.cs
- Int32CollectionConverter.cs
- PropertyReference.cs
- ColumnTypeConverter.cs
- SelectorAutomationPeer.cs
- EntityDataSourceStatementEditor.cs
- externdll.cs
- NativeObjectSecurity.cs
- WebEncodingValidatorAttribute.cs
- AnimationLayer.cs
- ControlBuilder.cs
- SqlDataSourceEnumerator.cs
- Rect3D.cs
- SymLanguageType.cs
- Interlocked.cs
- DnsPermission.cs
- MenuItem.cs
- SystemIPv6InterfaceProperties.cs
- ThicknessAnimationUsingKeyFrames.cs
- CompilationUtil.cs
- MbpInfo.cs
- wmiprovider.cs
- CatchBlock.cs
- OrderByExpression.cs
- TextReader.cs
- MessageDecoder.cs
- DynamicILGenerator.cs
- XmlDocument.cs
- InputLanguageCollection.cs
- ValueUnavailableException.cs
- HybridWebProxyFinder.cs
- IntranetCredentialPolicy.cs
- DataFormats.cs
- HtmlElementCollection.cs
- BuildProvidersCompiler.cs
- DataGridViewHitTestInfo.cs
- SqlCacheDependency.cs
- Typeface.cs
- PropertyInfoSet.cs
- XmlNamedNodeMap.cs
- BaseCodePageEncoding.cs
- FragmentNavigationEventArgs.cs
- SaveWorkflowCommand.cs
- RightNameExpirationInfoPair.cs
- sqlstateclientmanager.cs
- TdsRecordBufferSetter.cs
- JsonDeserializer.cs
- TextDecoration.cs
- JulianCalendar.cs
- ModelUIElement3D.cs
- TextFormatterHost.cs
- connectionpool.cs
- ConnectAlgorithms.cs
- URL.cs
- Point3D.cs
- ColorAnimationUsingKeyFrames.cs
- Nodes.cs
- EDesignUtil.cs
- MexBindingElement.cs
- Span.cs
- TextSelectionProcessor.cs
- Exceptions.cs
- __Error.cs
- InputBinding.cs
- DataMemberListEditor.cs
- QueryStringParameter.cs
- SortExpressionBuilder.cs
- ConsoleKeyInfo.cs