Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PageCanvasSize.cs / 1 / PageCanvasSize.cs
/*++ Copyright (C) 2003 Microsoft Corporation All rights reserved. Module Name: PageCanvasSize.cs Abstract: Definition and implementation of this public property type. Author: [....] ([....]) 8/22/2003 --*/ using System; using System.IO; using System.Xml; using System.Collections; using System.Diagnostics; using System.Printing; using MS.Internal.Printing.Configuration; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages namespace MS.Internal.Printing.Configuration { ////// Specifies the imageable area of the canvas. /// internal class CanvasImageableArea { #region Constructors internal CanvasImageableArea(ImageableSizeCapability ownerProperty) { this._ownerProperty = ownerProperty; _originWidth = _originHeight = _extentWidth = _extentHeight = PrintSchema.UnspecifiedIntValue; } #endregion Constructors #region Public Properties ////// Specifies the horizontal origin of the imageable area relative to the application media size, in 1/96 inch unit. /// public double OriginWidth { get { return UnitConverter.LengthValueFromMicronToDIP(_originWidth); } } ////// Specifies the vertical origin of the imageable area relative to the application media size, in 1/96 inch unit. /// public double OriginHeight { get { return UnitConverter.LengthValueFromMicronToDIP(_originHeight); } } ////// Specifies the horizontal distance between the origin and /// the bounding limit of the application media size, in 1/96 inch unit. /// public double ExtentWidth { get { return UnitConverter.LengthValueFromMicronToDIP(_extentWidth); } } ////// Specifies the vertical distance between the origin and /// the bounding limit of the application media size, in 1/96 inch unit. /// public double ExtentHeight { get { return UnitConverter.LengthValueFromMicronToDIP(_extentHeight); } } #endregion Public Properties #region Public Methods ////// Converts the imageable area to human-readable string. /// ///A string that represents this imageable area. public override string ToString() { return "[ImageableArea: Origin (" + OriginWidth + "," + OriginHeight + "), Extent (" + ExtentWidth + "x" + ExtentHeight + ")]"; } #endregion Public Methods #region Internal Fields internal ImageableSizeCapability _ownerProperty; // Integer values are always in micron unit internal int _originWidth; internal int _originHeight; internal int _extentWidth; internal int _extentHeight; #endregion Internal Fields } ////// Represents imageable size capability. /// internal class ImageableSizeCapability : PrintCapabilityRootProperty { #region Constructors internal ImageableSizeCapability() : base() { _imageableSizeWidth = _imageableSizeHeight = PrintSchema.UnspecifiedIntValue; } #endregion Constructors #region Public Properties ////// Specifies the horizontal dimension of application media size relative to the PageOrientation, in 1/96 inch unit. /// public double ImageableSizeWidth { get { return UnitConverter.LengthValueFromMicronToDIP(_imageableSizeWidth); } } ////// Specifies the vertical dimension of the application media size relative to the PageOrientation, in 1/96 inch unit. /// public double ImageableSizeHeight { get { return UnitConverter.LengthValueFromMicronToDIP(_imageableSizeHeight); } } ////// Specifies the imageable area of the canvas. /// public CanvasImageableArea ImageableArea { get { return _imageableArea; } } #endregion Public Properties #region Public Methods ////// Converts the imageable size capability object to human-readable string. /// ///A string that represents this imageable size capability object. public override string ToString() { return "ImageableSizeWidth=" + ImageableSizeWidth + ", ImageableSizeHeight=" + ImageableSizeHeight + " " + ((ImageableArea != null) ? ImageableArea.ToString() : "[ImageableArea: null]"); } #endregion Public Methods #region Internal Methods ///XML parser finds non-well-formness of XML internal override sealed bool BuildProperty(XmlPrintCapReader reader) { #if _DEBUG Trace.Assert(reader.CurrentElementNodeType == PrintSchemaNodeTypes.Property, "THIS SHOULD NOT HAPPEN: RootPropertyPropCallback gets non-Property node"); #endif int subPropDepth = reader.CurrentElementDepth + 1; // Loops over immediate property children of the root-level property while (reader.MoveToNextSchemaElement(subPropDepth, PrintSchemaNodeTypes.Property)) { if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ImageableSizeWidth) { try { this._imageableSizeWidth = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ImageableSizeHeight) { try { this._imageableSizeHeight = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ImageableArea) { this._imageableArea = new CanvasImageableArea(this); // When need to loop at a deeper depth, the code should cache the desired depth // value and use the cached value in the while-loop. Using CurrentElementDepth // in while-loop won't work correctly since the CurrentElementDepth value is changing. int iaPropDepth = reader.CurrentElementDepth + 1; // loop over one level down to read "ImageableArea" child-element properties while (reader.MoveToNextSchemaElement(iaPropDepth, PrintSchemaNodeTypes.Property)) { if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.OriginWidth) { try { this._imageableArea._originWidth = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.OriginHeight) { try { this._imageableArea._originHeight = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ExtentWidth) { try { this._imageableArea._extentWidth = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine ("-Error- " + e.Message); #endif } } else if (reader.CurrentElementNameAttrValue == PrintSchemaTags.Keywords.PageImageableSizeKeys.ExtentHeight) { try { this._imageableArea._extentHeight = reader.GetCurrentPropertyIntValueWithException(); } // We want to catch internal FormatException to skip recoverable XML content syntax error #pragma warning suppress 56502 #if _DEBUG catch (FormatException e) #else catch (FormatException) #endif { #if _DEBUG Trace.WriteLine ("-Error- " + e.Message); #endif } } else { #if _DEBUG Trace.WriteLine("-Warning- skip unknown ImageableArea sub-property '" + reader.CurrentElementNameAttrValue + "' at line " + reader._xmlReader.LineNumber + ", position " + reader._xmlReader.LinePosition); #endif } } } else { #if _DEBUG Trace.WriteLine("-Warning- skip unknown PageImageableSize sub-Property '" + reader.CurrentElementNameAttrValue + "' at line " + reader._xmlReader.LineNumber + ", position " + reader._xmlReader.LinePosition); #endif } } bool isValid = false; // We require ImageableSizeWidth/Height and ExtentWidth/Height values must be non-negative if ((this._imageableSizeWidth >= 0) && (this._imageableSizeHeight >= 0)) { isValid = true; // If ImageableArea is present, then its ExtentWidth/Height values must be non-negative. if (this.ImageableArea != null) { isValid = false; if ((this.ImageableArea._extentWidth >= 0) && (this.ImageableArea._extentHeight >= 0)) { isValid = true; } } } else { #if _DEBUG Trace.WriteLine("-Error- invalid PageImageableSize size values: " + this.ToString()); #endif } return isValid; } #endregion Internal Methods #region Internal Fields internal int _imageableSizeWidth; internal int _imageableSizeHeight; internal CanvasImageableArea _imageableArea; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
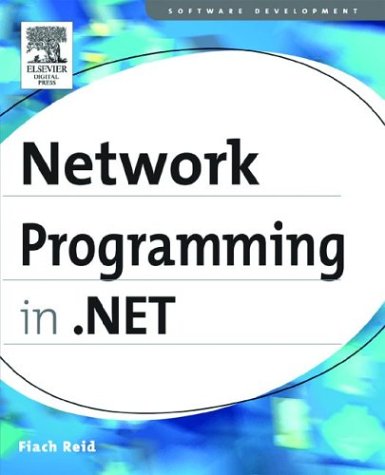
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlTriggerAttribute.cs
- ResourceIDHelper.cs
- LiteralSubsegment.cs
- Button.cs
- StopStoryboard.cs
- RenderCapability.cs
- DetailsViewUpdateEventArgs.cs
- XamlFilter.cs
- Substitution.cs
- SeverityFilter.cs
- UrlPath.cs
- WebBrowserHelper.cs
- XmlWellformedWriter.cs
- MultiViewDesigner.cs
- XmlChoiceIdentifierAttribute.cs
- controlskin.cs
- DataViewManager.cs
- ContentDefinition.cs
- DbConnectionPool.cs
- StringFunctions.cs
- BlobPersonalizationState.cs
- GAC.cs
- CompressStream.cs
- HttpHandlerAction.cs
- ZipPackage.cs
- AnnotationService.cs
- TreeNodeCollection.cs
- AvTraceFormat.cs
- CustomAttributeSerializer.cs
- DataTransferEventArgs.cs
- EventMappingSettingsCollection.cs
- TrackingProfile.cs
- CalendarDay.cs
- DesignSurfaceCollection.cs
- LinearKeyFrames.cs
- XamlSerializer.cs
- AttributeAction.cs
- Message.cs
- HtmlTableCellCollection.cs
- EntityType.cs
- DataControlFieldCell.cs
- RequestCacheValidator.cs
- SmtpNtlmAuthenticationModule.cs
- HtmlEncodedRawTextWriter.cs
- DocumentEventArgs.cs
- CroppedBitmap.cs
- DataGridViewTopLeftHeaderCell.cs
- ResourceWriter.cs
- LicFileLicenseProvider.cs
- _SpnDictionary.cs
- SpotLight.cs
- OneOfTypeConst.cs
- LocalizabilityAttribute.cs
- wgx_render.cs
- CreateUserErrorEventArgs.cs
- WebPartTransformerCollection.cs
- FlowDocumentReader.cs
- Int32RectValueSerializer.cs
- DataViewListener.cs
- SimpleApplicationHost.cs
- DataGridViewComponentPropertyGridSite.cs
- DoubleLink.cs
- ExtensionFile.cs
- InputLanguageProfileNotifySink.cs
- NullableIntMinMaxAggregationOperator.cs
- CodeEntryPointMethod.cs
- Metadata.cs
- RuntimeCompatibilityAttribute.cs
- LiteralLink.cs
- PathSegmentCollection.cs
- TokenCreationParameter.cs
- ElementAction.cs
- _BufferOffsetSize.cs
- StreamBodyWriter.cs
- BrowserCapabilitiesFactoryBase.cs
- DistinctQueryOperator.cs
- FilteredDataSetHelper.cs
- QilList.cs
- EntityDataSourceChangedEventArgs.cs
- KeyValuePairs.cs
- mediapermission.cs
- BitmapSourceSafeMILHandle.cs
- ActivityDelegate.cs
- VirtualizedItemPattern.cs
- XsdDataContractImporter.cs
- SpecularMaterial.cs
- DependencySource.cs
- DecoderFallback.cs
- OracleSqlParser.cs
- CreateUserErrorEventArgs.cs
- GcSettings.cs
- ApplicationInfo.cs
- WebPartMovingEventArgs.cs
- StreamUpdate.cs
- BindingContext.cs
- PageContent.cs
- Stroke.cs
- ValueQuery.cs
- _HeaderInfo.cs
- ArgumentOutOfRangeException.cs