Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / ContentPlaceHolder.cs / 1 / ContentPlaceHolder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using System.Web.UI; using System.Web.Util; internal class ContentPlaceHolderBuilder : ControlBuilder { private string _contentPlaceHolderID; private string _templateName; internal string Name { get { return _templateName; } } public override void Init(TemplateParser parser, ControlBuilder parentBuilder, Type type, string tagName, string ID, IDictionary attribs) { // Copy the ID so that it will be available when BuildObject is called _contentPlaceHolderID = ID; if (parser.FInDesigner) { // shortcut for designer base.Init(parser, parentBuilder, type, tagName, ID, attribs); return; } if (String.IsNullOrEmpty(ID)) { throw new HttpException(SR.GetString(SR.Control_Missing_Attribute, "ID", type.Name)); } _templateName = ID; MasterPageParser masterPageParser = parser as MasterPageParser; if (masterPageParser == null) { throw new HttpException(SR.GetString(SR.ContentPlaceHolder_only_in_master)); } base.Init(parser, parentBuilder, type, tagName, ID, attribs); if (masterPageParser.PlaceHolderList.Contains(Name)) throw new HttpException(SR.GetString(SR.ContentPlaceHolder_duplicate_contentPlaceHolderID, Name)); masterPageParser.PlaceHolderList.Add(Name); } public override object BuildObject() { MasterPage masterPage = TemplateControl as MasterPage; Debug.Assert(masterPage != null || InDesigner); // Instantiate the ContentPlaceHolder ContentPlaceHolder cph = (ContentPlaceHolder) base.BuildObject(); // If the page is providing content, instantiate it in the holder if (PageProvidesMatchingContent(masterPage)) { ITemplate tpl = ((System.Web.UI.ITemplate)(masterPage.ContentTemplates[_contentPlaceHolderID])); HttpContext context = HttpContext.Current; // Remember the old TemplateControl TemplateControl oldControl = context.TemplateControl; // Storing the template control into the context // since each thread needs to set it differently. context.TemplateControl = masterPage._ownerControl; try { // Instantiate the template using the correct TemplateControl tpl.InstantiateIn(cph); } finally { // Revert back to the old templateControl context.TemplateControl = oldControl; } } return cph; } internal override void BuildChildren(object parentObj) { MasterPage masterPage = TemplateControl as MasterPage; // If the page is providing content, don't call the base, which would // instantiate the default content (which we don't want) if (PageProvidesMatchingContent(masterPage)) return; base.BuildChildren(parentObj); } private bool PageProvidesMatchingContent(MasterPage masterPage) { if (masterPage != null && masterPage.ContentTemplates != null && masterPage.ContentTemplates.Contains(_contentPlaceHolderID)) { return true; } return false; } } // Factory used to efficiently create builder instances internal class ContentPlaceHolderBuilderFactory: IWebObjectFactory { object IWebObjectFactory.CreateInstance() { return new ContentPlaceHolderBuilder(); } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [ControlBuilderAttribute(typeof(ContentPlaceHolderBuilder))] [Designer("System.Web.UI.Design.WebControls.ContentPlaceHolderDesigner, " + AssemblyRef.SystemDesign)] [ToolboxItemFilter("System.Web.UI")] [ToolboxItemFilter("Microsoft.VisualStudio.Web.WebForms.MasterPageWebFormDesigner", ToolboxItemFilterType.Require)] [ToolboxData("<{0}:ContentPlaceHolder runat=\"server\">{0}:ContentPlaceHolder>")] public class ContentPlaceHolder : Control, INonBindingContainer { } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using System.Web.UI; using System.Web.Util; internal class ContentPlaceHolderBuilder : ControlBuilder { private string _contentPlaceHolderID; private string _templateName; internal string Name { get { return _templateName; } } public override void Init(TemplateParser parser, ControlBuilder parentBuilder, Type type, string tagName, string ID, IDictionary attribs) { // Copy the ID so that it will be available when BuildObject is called _contentPlaceHolderID = ID; if (parser.FInDesigner) { // shortcut for designer base.Init(parser, parentBuilder, type, tagName, ID, attribs); return; } if (String.IsNullOrEmpty(ID)) { throw new HttpException(SR.GetString(SR.Control_Missing_Attribute, "ID", type.Name)); } _templateName = ID; MasterPageParser masterPageParser = parser as MasterPageParser; if (masterPageParser == null) { throw new HttpException(SR.GetString(SR.ContentPlaceHolder_only_in_master)); } base.Init(parser, parentBuilder, type, tagName, ID, attribs); if (masterPageParser.PlaceHolderList.Contains(Name)) throw new HttpException(SR.GetString(SR.ContentPlaceHolder_duplicate_contentPlaceHolderID, Name)); masterPageParser.PlaceHolderList.Add(Name); } public override object BuildObject() { MasterPage masterPage = TemplateControl as MasterPage; Debug.Assert(masterPage != null || InDesigner); // Instantiate the ContentPlaceHolder ContentPlaceHolder cph = (ContentPlaceHolder) base.BuildObject(); // If the page is providing content, instantiate it in the holder if (PageProvidesMatchingContent(masterPage)) { ITemplate tpl = ((System.Web.UI.ITemplate)(masterPage.ContentTemplates[_contentPlaceHolderID])); HttpContext context = HttpContext.Current; // Remember the old TemplateControl TemplateControl oldControl = context.TemplateControl; // Storing the template control into the context // since each thread needs to set it differently. context.TemplateControl = masterPage._ownerControl; try { // Instantiate the template using the correct TemplateControl tpl.InstantiateIn(cph); } finally { // Revert back to the old templateControl context.TemplateControl = oldControl; } } return cph; } internal override void BuildChildren(object parentObj) { MasterPage masterPage = TemplateControl as MasterPage; // If the page is providing content, don't call the base, which would // instantiate the default content (which we don't want) if (PageProvidesMatchingContent(masterPage)) return; base.BuildChildren(parentObj); } private bool PageProvidesMatchingContent(MasterPage masterPage) { if (masterPage != null && masterPage.ContentTemplates != null && masterPage.ContentTemplates.Contains(_contentPlaceHolderID)) { return true; } return false; } } // Factory used to efficiently create builder instances internal class ContentPlaceHolderBuilderFactory: IWebObjectFactory { object IWebObjectFactory.CreateInstance() { return new ContentPlaceHolderBuilder(); } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [ControlBuilderAttribute(typeof(ContentPlaceHolderBuilder))] [Designer("System.Web.UI.Design.WebControls.ContentPlaceHolderDesigner, " + AssemblyRef.SystemDesign)] [ToolboxItemFilter("System.Web.UI")] [ToolboxItemFilter("Microsoft.VisualStudio.Web.WebForms.MasterPageWebFormDesigner", ToolboxItemFilterType.Require)] [ToolboxData("<{0}:ContentPlaceHolder runat=\"server\">{0}:ContentPlaceHolder>")] public class ContentPlaceHolder : Control, INonBindingContainer { } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
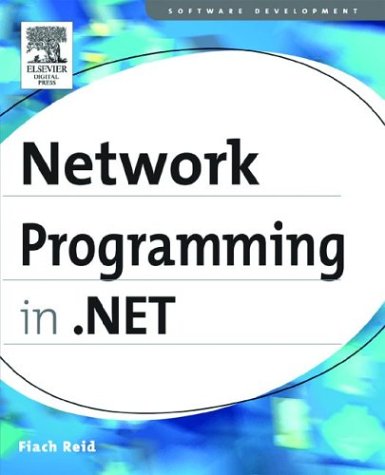
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LicenseManager.cs
- Operand.cs
- TypedTableBase.cs
- GiveFeedbackEvent.cs
- InputMethodStateChangeEventArgs.cs
- FirstMatchCodeGroup.cs
- NavigationService.cs
- MappingMetadataHelper.cs
- EdmToObjectNamespaceMap.cs
- FrameworkElementAutomationPeer.cs
- BindingElementCollection.cs
- CodeRegionDirective.cs
- ReceiveActivityDesignerTheme.cs
- NavigationPropertySingletonExpression.cs
- CallbackHandler.cs
- Validator.cs
- CompilerScopeManager.cs
- ColorContextHelper.cs
- ObjRef.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- CodePageEncoding.cs
- LineVisual.cs
- ButtonAutomationPeer.cs
- RegisteredHiddenField.cs
- MSAAWinEventWrap.cs
- BitHelper.cs
- WebPartsPersonalizationAuthorization.cs
- CopyAction.cs
- CaretElement.cs
- TypeHelper.cs
- HtmlGenericControl.cs
- SqlDataSourceParameterParser.cs
- ContentDisposition.cs
- StorageMappingItemCollection.cs
- CopyNodeSetAction.cs
- Regex.cs
- PrintDialog.cs
- WindowsSolidBrush.cs
- CompositeScriptReference.cs
- BrushConverter.cs
- SettingsPropertyCollection.cs
- XmlTextEncoder.cs
- StyleSheetRefUrlEditor.cs
- OleCmdHelper.cs
- IteratorFilter.cs
- TearOffProxy.cs
- FunctionImportElement.cs
- BaseValidator.cs
- _DigestClient.cs
- ListView.cs
- AttributeCollection.cs
- AssociationTypeEmitter.cs
- OleDbInfoMessageEvent.cs
- XmlExpressionDumper.cs
- ModulesEntry.cs
- HtmlListAdapter.cs
- DataTableReader.cs
- SqlBuilder.cs
- Pool.cs
- Attributes.cs
- ClientUrlResolverWrapper.cs
- TypeBuilder.cs
- Expander.cs
- TextParagraphProperties.cs
- ConfigXmlAttribute.cs
- RadioButtonBaseAdapter.cs
- DelegatedStream.cs
- BuildManager.cs
- HyperlinkAutomationPeer.cs
- TableLayoutSettings.cs
- ReliableReplySessionChannel.cs
- PageContent.cs
- DataControlButton.cs
- CodeLinePragma.cs
- Debug.cs
- SqlEnums.cs
- FrameworkTemplate.cs
- XmlAttributeHolder.cs
- AmbientLight.cs
- ObjectRef.cs
- InputProviderSite.cs
- DayRenderEvent.cs
- SerializableAttribute.cs
- SizeAnimationClockResource.cs
- PartialCachingControl.cs
- xsdvalidator.cs
- WindowsHyperlink.cs
- EntryPointNotFoundException.cs
- TimeoutStream.cs
- CollectionBuilder.cs
- PrivilegeNotHeldException.cs
- String.cs
- WebPartAddingEventArgs.cs
- ItemList.cs
- ImageMap.cs
- SafeNativeMethods.cs
- DataGridViewAdvancedBorderStyle.cs
- RuleInfoComparer.cs
- FileDialogCustomPlaces.cs
- LinqToSqlWrapper.cs