Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / SessionPageStatePersister.cs / 1 / SessionPageStatePersister.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.Collections.Specialized; using System.IO; using System.Text; using System.Web.SessionState; using System.Web.Configuration; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class SessionPageStatePersister : PageStatePersister { private const string _viewStateSessionKey = "__SESSIONVIEWSTATE"; private const string _viewStateQueueKey = "__VIEWSTATEQUEUE"; public SessionPageStatePersister(Page page) : base (page) { HttpSessionState session = null; try { session = page.Session; } catch { // ignore, throw if session is null. } if (session == null) { throw new ArgumentException(SR.GetString(SR.SessionPageStatePersister_SessionMustBeEnabled)); } } public override void Load() { NameValueCollection requestValueCollection = Page.RequestValueCollection; if (requestValueCollection == null) { return; } try { string combinedSerializedStateString = Page.RequestViewStateString; string persistedStateID = null; bool controlStateInSession = false; // SessionState will persist a Pair of, // where if requiresControlStateInSession is true, second will just be the sessionID, as // we will store both control state and view state in session. Otherwise, we store just the // view state in session and the pair will be if (!String.IsNullOrEmpty(combinedSerializedStateString)) { Pair combinedState = (Pair)Util.DeserializeWithAssert(StateFormatter, combinedSerializedStateString); // Check if we are storing control state in session as well if ((bool)combinedState.First) { // So the second is the persistedID persistedStateID = (string)combinedState.Second; controlStateInSession = true; } else { // Second is Pair pair = (Pair)combinedState.Second; persistedStateID = (string)pair.First; ControlState = pair.Second; } } if (persistedStateID != null) { object sessionData = Page.Session[_viewStateSessionKey + persistedStateID]; if (controlStateInSession) { Pair combinedState = sessionData as Pair; if (combinedState != null) { ViewState = combinedState.First; ControlState = combinedState.Second; } } else { ViewState = sessionData; } } } catch (Exception e) { // Setup the formatter for this exception, to make sure this message shows up // in an error page as opposed to the inner-most exception's message. HttpException newException = new HttpException(SR.GetString(SR.Invalid_ControlState), e); newException.SetFormatter(new UseLastUnhandledErrorFormatter(newException)); throw newException; } } /// /// To be supplied. /// public override void Save() { bool requiresControlStateInSession = false; object clientData = null; Triplet vsTrip = ViewState as Triplet; // no session view state to store. if ((ControlState != null) || ((vsTrip == null || vsTrip.Second != null || vsTrip.Third != null) && ViewState != null)) { HttpSessionState session = Page.Session; string sessionViewStateID = Convert.ToString(DateTime.Now.Ticks, 16); object state = null; requiresControlStateInSession = Page.Request.Browser.RequiresControlStateInSession; if (requiresControlStateInSession) { // ClientState will just be sessionID state = new Pair(ViewState, ControlState); clientData = sessionViewStateID; } else { // ClientState will be astate = ViewState; clientData = new Pair(sessionViewStateID, ControlState); } string sessionKey = _viewStateSessionKey + sessionViewStateID; session[sessionKey] = state; Queue queue = session[_viewStateQueueKey] as Queue; if (queue == null) { queue = new Queue(); session[_viewStateQueueKey] = queue; } queue.Enqueue(sessionKey); SessionPageStateSection cfg = RuntimeConfig.GetConfig(Page.Request.Context).SessionPageState; int queueCount = queue.Count; if (cfg != null && queueCount > cfg.HistorySize || cfg == null && queueCount > SessionPageStateSection.DefaultHistorySize) { string oldSessionKey = (string)queue.Dequeue(); session.Remove(oldSessionKey); } } if (clientData != null) { Page.ClientState = Util.SerializeWithAssert(StateFormatter, new Pair(requiresControlStateInSession, clientData)); } } } }
Link Menu
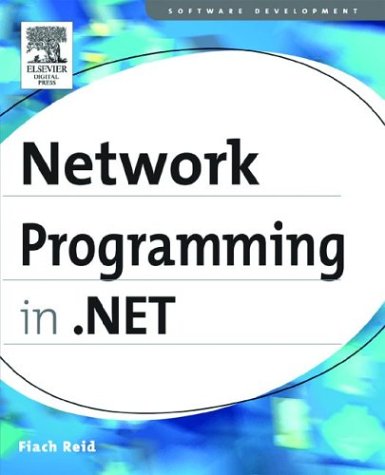
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IgnoreSection.cs
- AssertSection.cs
- PropertyOverridesDialog.cs
- ProcessManager.cs
- sqlcontext.cs
- ThreadInterruptedException.cs
- MenuItemCollection.cs
- ActionItem.cs
- WebPartTransformer.cs
- SchemaImporterExtensionElementCollection.cs
- CodeDelegateCreateExpression.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- FilteredReadOnlyMetadataCollection.cs
- ActivityCodeDomSerializationManager.cs
- MobileTemplatedControlDesigner.cs
- PowerModeChangedEventArgs.cs
- KeyInterop.cs
- HyperLinkStyle.cs
- XmlWellformedWriter.cs
- CodeExporter.cs
- PrincipalPermission.cs
- SchemaImporterExtensionElementCollection.cs
- CacheRequest.cs
- HtmlTernaryTree.cs
- DynamicActivityTypeDescriptor.cs
- KeyFrames.cs
- SoapClientMessage.cs
- TypedDataSourceCodeGenerator.cs
- EdmItemCollection.cs
- SafeFindHandle.cs
- InkCanvasFeedbackAdorner.cs
- Int32EqualityComparer.cs
- TransformPatternIdentifiers.cs
- CustomAttributeFormatException.cs
- NullReferenceException.cs
- NegatedCellConstant.cs
- ManagementClass.cs
- MarginCollapsingState.cs
- Propagator.cs
- PersonalizationState.cs
- PrimitiveXmlSerializers.cs
- TypefaceMetricsCache.cs
- SqlFactory.cs
- SqlCaseSimplifier.cs
- ClientUrlResolverWrapper.cs
- TreeNode.cs
- OdbcHandle.cs
- Set.cs
- DesignBinding.cs
- FlagsAttribute.cs
- Material.cs
- TriggerAction.cs
- HttpRuntimeSection.cs
- Timeline.cs
- StorageAssociationSetMapping.cs
- TimeSpanStorage.cs
- InvalidPrinterException.cs
- dtdvalidator.cs
- TextSimpleMarkerProperties.cs
- SecurityManager.cs
- DataGridSortCommandEventArgs.cs
- PerformanceCounterPermissionEntryCollection.cs
- TreeView.cs
- TemplateEditingFrame.cs
- SourceFilter.cs
- MemberAccessException.cs
- RadioButtonAutomationPeer.cs
- WebDescriptionAttribute.cs
- Activator.cs
- XmlDataImplementation.cs
- BrowserCapabilitiesFactoryBase.cs
- SchemaImporterExtension.cs
- XsdValidatingReader.cs
- PopupEventArgs.cs
- SocketStream.cs
- Pair.cs
- DataGridItemEventArgs.cs
- UInt64.cs
- ColorConvertedBitmapExtension.cs
- DoubleLink.cs
- DataGridViewRowCollection.cs
- TabPanel.cs
- UIElement.cs
- VariableDesigner.xaml.cs
- NotCondition.cs
- FontCacheUtil.cs
- NameSpaceExtractor.cs
- OdbcEnvironmentHandle.cs
- ImageSource.cs
- DataGridViewRowsAddedEventArgs.cs
- ArgIterator.cs
- Page.cs
- WebBrowsableAttribute.cs
- MarshalDirectiveException.cs
- SqlServer2KCompatibilityCheck.cs
- SBCSCodePageEncoding.cs
- QueryOutputWriterV1.cs
- RMEnrollmentPage3.cs
- ModuleBuilder.cs
- DataGridViewCellStyle.cs