Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / ChannelManager.cs / 1305600 / ChannelManager.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The ChannelManager is a helper structure internal to MediaContext // that abstracts out channel creation, storage and destruction. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using MS.Internal; using MS.Utility; using MS.Win32; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; namespace System.Windows.Media { partial class MediaContext { ////// A helper structure that abstracts channel management. /// ////// The common agreement (avcomptm, avpttrev) is that channel creation /// should be considered unsafe and therefore subject to control via /// the SecurityCritical/SecurityTreatAsSafe mechanism. /// /// On the other hand, using a channel that has been created by the /// MediaContext is considered safe. /// /// This class expresses the above policy by hiding channel creation /// and storage within the MediaContext while allowing to access /// the channels at will. /// private struct ChannelManager { ////// Opens an asynchronous channel. /// ////// Critical - Creates a channel, calls a Channel method, calls methods performing elevations. /// TreatAsSafe - It is safe for the media context to create and use a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateChannels() { Invariant.Assert(_asyncChannel == null); Invariant.Assert(_asyncOutOfBandChannel == null); // Create a channel into the async composition device. // Pass in a reference to the global mediasystem channel so that it uses // the same partition. _asyncChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, false, // not out of band System.Windows.Media.MediaSystem.Connection, false // [....] transport ); _asyncOutOfBandChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, true, // out of band System.Windows.Media.MediaSystem.Connection, false // [....] transport ); } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to [....] channels channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveSyncChannels() { // // Close the synchronous channels. // if (_freeSyncChannels != null) { while (_freeSyncChannels.Count > 0) { _freeSyncChannels.Dequeue().Close(); } _freeSyncChannels = null; } if (_syncServiceChannel != null) { _syncServiceChannel.Close(); _syncServiceChannel = null; } } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to close a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveChannels() { if (_asyncChannel != null) { _asyncChannel.Close(); _asyncChannel = null; } if (_asyncOutOfBandChannel != null) { _asyncOutOfBandChannel.Close(); _asyncOutOfBandChannel = null; } RemoveSyncChannels(); if (_pSyncConnection != IntPtr.Zero) { HRESULT.Check(UnsafeNativeMethods.WgxConnection_Disconnect(_pSyncConnection)); _pSyncConnection = IntPtr.Zero; } } ////// Create a fresh or fetch one from the pool synchronous channel. /// ////// Critical - Calls critical methods to create a synchronous channel and return it. /// TreatAsSafe - This function allocates the channel in a Channel class that guards channel /// access from insecure access. It is also ok for this method to allocate the native objects /// since there is nothing security critical about having the channel infrastructure initialized. /// [SecurityCritical, SecurityTreatAsSafe] internal DUCE.Channel AllocateSyncChannel() { DUCE.Channel syncChannel; if (_pSyncConnection == IntPtr.Zero) { HRESULT.Check(UnsafeNativeMethods.WgxConnection_Create( true, // true means synchronous transport out _pSyncConnection)); } if (_freeSyncChannels == null) { // // Ensure the free [....] channels queue... // _freeSyncChannels = new Queue(3); } if (_freeSyncChannels.Count > 0) { // // If there is a free [....] channel in the queue, we're done: // return _freeSyncChannels.Dequeue(); } else { // // Otherwise, create a new channel. We will try to cache it // when the ReleaseSyncChannel call is made. Also ensure the // synchronous service channel and glyph cache. // if (_syncServiceChannel == null) { _syncServiceChannel = new DUCE.Channel( null, false, // not out of band _pSyncConnection, true // synchronous ); } syncChannel = new DUCE.Channel( _syncServiceChannel, false, // not out of band _pSyncConnection, true // synchronous ); return syncChannel; } } /// /// Returns a [....] channel back to the pool. /// ////// Critical - Creates and returns a synchronous channel. /// TreatAsSafe - Closing a channel is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void ReleaseSyncChannel(DUCE.Channel channel) { Invariant.Assert(_freeSyncChannels != null); // // A decision needs to be made whether or not we're interested // in re-using this channel later. For now, store up to three // synchronous channel in the queue. // if (_freeSyncChannels.Count <= 3) { _freeSyncChannels.Enqueue(channel); } else { channel.Close(); } } ////// Returns the asynchronous channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel Channel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncChannel; } } ////// Returns the asynchronous out-of-band channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel OutOfBandChannel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncOutOfBandChannel; } } ////// The asynchronous channel. /// ////// This field will be replaced with an asynchronous channel table as per task #26681. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncChannel; ////// The asynchronous out-of-band channel. /// ////// This is needed by CompositionTarget for handling WM_PAINT, etc. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncOutOfBandChannel; ////// We store the free synchronous channels for later re-use in this queue. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private Queue_freeSyncChannels; /// /// The service channel to the synchronous partition. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _syncServiceChannel; ////// Pointer to the unmanaged connection object. /// ////// Critical - Controlled unmanaged resource. /// [SecurityCritical] private IntPtr _pSyncConnection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
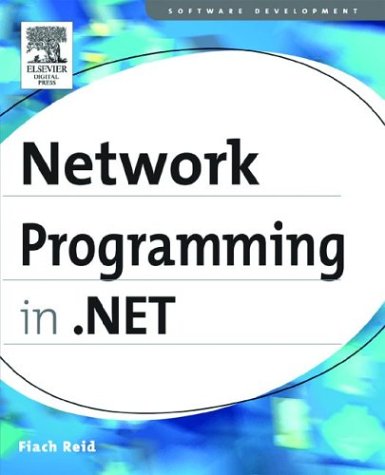
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegexInterpreter.cs
- DocumentSchemaValidator.cs
- HTTP_SERVICE_CONFIG_URLACL_PARAM.cs
- DictionaryBase.cs
- CodeExporter.cs
- InvalidDataException.cs
- ProfileProvider.cs
- SecurityPermission.cs
- WindowsListViewItem.cs
- ListView.cs
- BlobPersonalizationState.cs
- SqlGenerator.cs
- ToolTip.cs
- BuildProviderCollection.cs
- PolyBezierSegmentFigureLogic.cs
- Parameter.cs
- DataGridViewImageCell.cs
- SyndicationSerializer.cs
- EncryptedReference.cs
- ResourceSetExpression.cs
- Size3DConverter.cs
- Win32PrintDialog.cs
- ObjectDataSourceMethodEditor.cs
- TransactedReceiveScope.cs
- FixedSOMPageConstructor.cs
- CharEnumerator.cs
- WindowsRegion.cs
- WhitespaceRuleReader.cs
- Nullable.cs
- WebSysDisplayNameAttribute.cs
- LineInfo.cs
- Panel.cs
- Selection.cs
- CompositeFontFamily.cs
- TerminatorSinks.cs
- TimeEnumHelper.cs
- DataControlFieldHeaderCell.cs
- FileAuthorizationModule.cs
- DataGridViewControlCollection.cs
- ButtonColumn.cs
- UserControl.cs
- PenContexts.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- SqlErrorCollection.cs
- SignatureHelper.cs
- ServicePoint.cs
- PropertyPathWorker.cs
- DrawingAttributesDefaultValueFactory.cs
- ReachPrintTicketSerializerAsync.cs
- MediaPlayerState.cs
- ConfigurationManagerHelper.cs
- HistoryEventArgs.cs
- SmiEventSink_DeferedProcessing.cs
- ColorTransformHelper.cs
- ListViewGroupItemCollection.cs
- ServiceRouteHandler.cs
- HtmlTextArea.cs
- DetailsViewRow.cs
- XmlCDATASection.cs
- EventRouteFactory.cs
- LinkArea.cs
- OracleBinary.cs
- UpdateCommand.cs
- ElementHostAutomationPeer.cs
- FamilyTypefaceCollection.cs
- WorkflowFileItem.cs
- InvokeWebServiceDesigner.cs
- SQLBytes.cs
- WebServiceResponseDesigner.cs
- CryptoStream.cs
- SimpleFileLog.cs
- MulticastDelegate.cs
- mansign.cs
- WeakReferenceList.cs
- SHA1CryptoServiceProvider.cs
- xmlformatgeneratorstatics.cs
- PagePropertiesChangingEventArgs.cs
- Reference.cs
- GridViewUpdatedEventArgs.cs
- DefaultBindingPropertyAttribute.cs
- Relationship.cs
- TemplateBuilder.cs
- IdnMapping.cs
- Rectangle.cs
- WebHostScriptMappingsInstallComponent.cs
- SiteMapProvider.cs
- PerformanceCounterCategory.cs
- IList.cs
- TextTreeTextBlock.cs
- DBSqlParserColumnCollection.cs
- Stylus.cs
- Symbol.cs
- COM2TypeInfoProcessor.cs
- TabletDevice.cs
- Console.cs
- IndexedGlyphRun.cs
- ByteStack.cs
- ListCommandEventArgs.cs
- XmlArrayItemAttributes.cs
- DataGridTableCollection.cs