Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / Int32KeyFrameCollection.cs / 1 / Int32KeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameInt32Animation /// to animate a Int32 property value along a set of key frames. /// public class Int32KeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static Int32KeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new Int32KeyFrameCollection. /// public Int32KeyFrameCollection() : base() { _keyFrames = new List< Int32KeyFrame>(2); } #endregion #region Static Methods ////// An empty Int32KeyFrameCollection. /// public static Int32KeyFrameCollection Empty { get { if (s_emptyCollection == null) { Int32KeyFrameCollection emptyCollection = new Int32KeyFrameCollection(); emptyCollection._keyFrames = new List< Int32KeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this Int32KeyFrameCollection. /// ///The copy public new Int32KeyFrameCollection Clone() { return (Int32KeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Int32KeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the Int32KeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of Int32KeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the Int32KeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the Int32KeyFrames in the collection to an /// array of Int32KeyFrames. /// public void CopyTo(Int32KeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a Int32KeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((Int32KeyFrame)keyFrame); } ////// Adds a Int32KeyFrame to the collection. /// public int Add(Int32KeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all Int32KeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given Int32KeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((Int32KeyFrame)keyFrame); } ////// Returns true of the collection contains the given Int32KeyFrame. /// public bool Contains(Int32KeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given Int32KeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((Int32KeyFrame)keyFrame); } ////// Returns the index of a given Int32KeyFrame in the collection. /// public int IndexOf(Int32KeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a Int32KeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (Int32KeyFrame)keyFrame); } ////// Inserts a Int32KeyFrame into a specific location in the collection. /// public void Insert(int index, Int32KeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a Int32KeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((Int32KeyFrame)keyFrame); } ////// Removes a Int32KeyFrame from the collection. /// public void Remove(Int32KeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the Int32KeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the Int32KeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (Int32KeyFrame)value; } } ////// Gets or sets the Int32KeyFrame at a given index. /// public Int32KeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "Int32KeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameInt32Animation /// to animate a Int32 property value along a set of key frames. /// public class Int32KeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static Int32KeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new Int32KeyFrameCollection. /// public Int32KeyFrameCollection() : base() { _keyFrames = new List< Int32KeyFrame>(2); } #endregion #region Static Methods ////// An empty Int32KeyFrameCollection. /// public static Int32KeyFrameCollection Empty { get { if (s_emptyCollection == null) { Int32KeyFrameCollection emptyCollection = new Int32KeyFrameCollection(); emptyCollection._keyFrames = new List< Int32KeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this Int32KeyFrameCollection. /// ///The copy public new Int32KeyFrameCollection Clone() { return (Int32KeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Int32KeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { Int32KeyFrameCollection sourceCollection = (Int32KeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Int32KeyFrame>(count); for (int i = 0; i < count; i++) { Int32KeyFrame keyFrame = (Int32KeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the Int32KeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of Int32KeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the Int32KeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the Int32KeyFrames in the collection to an /// array of Int32KeyFrames. /// public void CopyTo(Int32KeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a Int32KeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((Int32KeyFrame)keyFrame); } ////// Adds a Int32KeyFrame to the collection. /// public int Add(Int32KeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all Int32KeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given Int32KeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((Int32KeyFrame)keyFrame); } ////// Returns true of the collection contains the given Int32KeyFrame. /// public bool Contains(Int32KeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given Int32KeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((Int32KeyFrame)keyFrame); } ////// Returns the index of a given Int32KeyFrame in the collection. /// public int IndexOf(Int32KeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a Int32KeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (Int32KeyFrame)keyFrame); } ////// Inserts a Int32KeyFrame into a specific location in the collection. /// public void Insert(int index, Int32KeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a Int32KeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((Int32KeyFrame)keyFrame); } ////// Removes a Int32KeyFrame from the collection. /// public void Remove(Int32KeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the Int32KeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the Int32KeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (Int32KeyFrame)value; } } ////// Gets or sets the Int32KeyFrame at a given index. /// public Int32KeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "Int32KeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
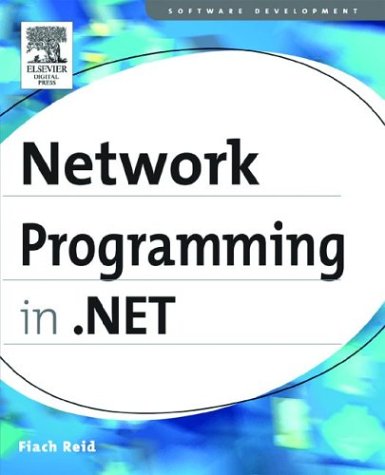
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CorrelationTokenTypeConvertor.cs
- AddInController.cs
- DiscoveryClientDocuments.cs
- OwnerDrawPropertyBag.cs
- StorageEntityContainerMapping.cs
- DelegateHelpers.Generated.cs
- QueuePathDialog.cs
- LayoutExceptionEventArgs.cs
- RequestCachePolicy.cs
- DynamicMethod.cs
- InputLanguageCollection.cs
- SQLChars.cs
- ContentPlaceHolder.cs
- BufferedStream.cs
- Document.cs
- DesignerActionItem.cs
- Decoder.cs
- RegexCapture.cs
- ObjectConverter.cs
- StylusLogic.cs
- ContainerSelectorBehavior.cs
- DependencyObjectProvider.cs
- ArraySortHelper.cs
- MouseButtonEventArgs.cs
- HttpContextWrapper.cs
- AuthorizationSection.cs
- DataListItem.cs
- Schema.cs
- XmlValidatingReader.cs
- ExpressionPrefixAttribute.cs
- AnnotationService.cs
- SqlComparer.cs
- ADConnectionHelper.cs
- CompilerLocalReference.cs
- XsltException.cs
- ManipulationStartingEventArgs.cs
- figurelength.cs
- ContextBase.cs
- DatagridviewDisplayedBandsData.cs
- XPathException.cs
- ImportOptions.cs
- SiteMapDataSourceView.cs
- WindowsAltTab.cs
- TrustManager.cs
- AppSettingsExpressionBuilder.cs
- FamilyTypefaceCollection.cs
- XamlStyleSerializer.cs
- ToolBarTray.cs
- StreamGeometry.cs
- PackageProperties.cs
- RelationshipFixer.cs
- DataGridGeneralPage.cs
- TextEncodedRawTextWriter.cs
- CharKeyFrameCollection.cs
- FlowNode.cs
- FontNamesConverter.cs
- figurelength.cs
- UnsignedPublishLicense.cs
- Authorization.cs
- LocalTransaction.cs
- ActivityWithResultConverter.cs
- QueryCoreOp.cs
- ListControlConvertEventArgs.cs
- XmlEntity.cs
- ZipFileInfoCollection.cs
- OutputCacheProfileCollection.cs
- Delegate.cs
- CopyOnWriteList.cs
- SwitchElementsCollection.cs
- ControlValuePropertyAttribute.cs
- LicenseContext.cs
- DataGridViewRowEventArgs.cs
- MultiView.cs
- OdbcCommandBuilder.cs
- CancellationTokenSource.cs
- ControlCollection.cs
- BookmarkScopeManager.cs
- EncoderParameters.cs
- DelayLoadType.cs
- LoadRetryConstantStrategy.cs
- HtmlInputReset.cs
- CommandValueSerializer.cs
- ContainerParagraph.cs
- XsltQilFactory.cs
- DbDataSourceEnumerator.cs
- SegmentInfo.cs
- SystemTcpStatistics.cs
- BrushValueSerializer.cs
- Point.cs
- NumericPagerField.cs
- ToolStripMenuItem.cs
- VisualStyleTypesAndProperties.cs
- Tool.cs
- TextLineResult.cs
- SplashScreen.cs
- Condition.cs
- DataObjectPastingEventArgs.cs
- AnyAllSearchOperator.cs
- SpecularMaterial.cs
- ConnectionConsumerAttribute.cs