Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / ContentPlaceHolder.cs / 1 / ContentPlaceHolder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using System.Web.UI; using System.Web.Util; internal class ContentPlaceHolderBuilder : ControlBuilder { private string _contentPlaceHolderID; private string _templateName; internal string Name { get { return _templateName; } } public override void Init(TemplateParser parser, ControlBuilder parentBuilder, Type type, string tagName, string ID, IDictionary attribs) { // Copy the ID so that it will be available when BuildObject is called _contentPlaceHolderID = ID; if (parser.FInDesigner) { // shortcut for designer base.Init(parser, parentBuilder, type, tagName, ID, attribs); return; } if (String.IsNullOrEmpty(ID)) { throw new HttpException(SR.GetString(SR.Control_Missing_Attribute, "ID", type.Name)); } _templateName = ID; MasterPageParser masterPageParser = parser as MasterPageParser; if (masterPageParser == null) { throw new HttpException(SR.GetString(SR.ContentPlaceHolder_only_in_master)); } base.Init(parser, parentBuilder, type, tagName, ID, attribs); if (masterPageParser.PlaceHolderList.Contains(Name)) throw new HttpException(SR.GetString(SR.ContentPlaceHolder_duplicate_contentPlaceHolderID, Name)); masterPageParser.PlaceHolderList.Add(Name); } public override object BuildObject() { MasterPage masterPage = TemplateControl as MasterPage; Debug.Assert(masterPage != null || InDesigner); // Instantiate the ContentPlaceHolder ContentPlaceHolder cph = (ContentPlaceHolder) base.BuildObject(); // If the page is providing content, instantiate it in the holder if (PageProvidesMatchingContent(masterPage)) { ITemplate tpl = ((System.Web.UI.ITemplate)(masterPage.ContentTemplates[_contentPlaceHolderID])); HttpContext context = HttpContext.Current; // Remember the old TemplateControl TemplateControl oldControl = context.TemplateControl; // Storing the template control into the context // since each thread needs to set it differently. context.TemplateControl = masterPage._ownerControl; try { // Instantiate the template using the correct TemplateControl tpl.InstantiateIn(cph); } finally { // Revert back to the old templateControl context.TemplateControl = oldControl; } } return cph; } internal override void BuildChildren(object parentObj) { MasterPage masterPage = TemplateControl as MasterPage; // If the page is providing content, don't call the base, which would // instantiate the default content (which we don't want) if (PageProvidesMatchingContent(masterPage)) return; base.BuildChildren(parentObj); } private bool PageProvidesMatchingContent(MasterPage masterPage) { if (masterPage != null && masterPage.ContentTemplates != null && masterPage.ContentTemplates.Contains(_contentPlaceHolderID)) { return true; } return false; } } // Factory used to efficiently create builder instances internal class ContentPlaceHolderBuilderFactory: IWebObjectFactory { object IWebObjectFactory.CreateInstance() { return new ContentPlaceHolderBuilder(); } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [ControlBuilderAttribute(typeof(ContentPlaceHolderBuilder))] [Designer("System.Web.UI.Design.WebControls.ContentPlaceHolderDesigner, " + AssemblyRef.SystemDesign)] [ToolboxItemFilter("System.Web.UI")] [ToolboxItemFilter("Microsoft.VisualStudio.Web.WebForms.MasterPageWebFormDesigner", ToolboxItemFilterType.Require)] [ToolboxData("<{0}:ContentPlaceHolder runat=\"server\">{0}:ContentPlaceHolder>")] public class ContentPlaceHolder : Control, INonBindingContainer { } }
Link Menu
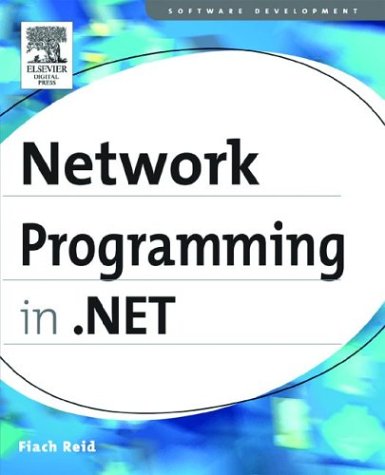
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SourceElementsCollection.cs
- XhtmlBasicListAdapter.cs
- DirectoryNotFoundException.cs
- SelfIssuedTokenFactoryCredential.cs
- ISAPIApplicationHost.cs
- BidPrivateBase.cs
- GorillaCodec.cs
- Context.cs
- MachineKeySection.cs
- MimeTypeAttribute.cs
- ConnectionsZoneDesigner.cs
- VisualBrush.cs
- GridViewItemAutomationPeer.cs
- Assembly.cs
- Win32PrintDialog.cs
- Pair.cs
- _AutoWebProxyScriptHelper.cs
- HttpCapabilitiesBase.cs
- OleDbParameterCollection.cs
- CodeArgumentReferenceExpression.cs
- TreeNodeEventArgs.cs
- QilCloneVisitor.cs
- ToolStripItemClickedEventArgs.cs
- MouseButtonEventArgs.cs
- StyleSheet.cs
- FieldAccessException.cs
- SocketInformation.cs
- QueryOutputWriter.cs
- FixedSchema.cs
- SeekableReadStream.cs
- Typeface.cs
- EdmProviderManifest.cs
- _AuthenticationState.cs
- OdbcCommand.cs
- DataSpaceManager.cs
- SqlRowUpdatingEvent.cs
- EventSetter.cs
- WebErrorHandler.cs
- ControlCachePolicy.cs
- SecurityPermission.cs
- DataRelationCollection.cs
- MachineSettingsSection.cs
- InputGestureCollection.cs
- LocalizableResourceBuilder.cs
- SmtpFailedRecipientException.cs
- BindingExpressionUncommonField.cs
- ChameleonKey.cs
- StructuredTypeEmitter.cs
- PerfCounters.cs
- DataBoundControl.cs
- PanelStyle.cs
- FixUpCollection.cs
- RichTextBoxConstants.cs
- DrawingContextWalker.cs
- ThreadAttributes.cs
- filewebresponse.cs
- AssertFilter.cs
- DotNetATv1WindowsLogEntryDeserializer.cs
- StrokeDescriptor.cs
- VoiceObjectToken.cs
- PauseStoryboard.cs
- XmlRawWriter.cs
- FileDataSourceCache.cs
- SqlCacheDependencyDatabaseCollection.cs
- Base64Encoder.cs
- TextFormatterContext.cs
- MessageAction.cs
- QueueProcessor.cs
- XmlWrappingReader.cs
- XsltInput.cs
- unitconverter.cs
- ResourceContainer.cs
- Vector3D.cs
- PowerModeChangedEventArgs.cs
- AuthenticationSection.cs
- WindowsFormsHelpers.cs
- MaterializeFromAtom.cs
- TypeForwardedToAttribute.cs
- TreeIterators.cs
- CommandEventArgs.cs
- DataGridParentRows.cs
- WhiteSpaceTrimStringConverter.cs
- NamespaceInfo.cs
- RotationValidation.cs
- shaperfactoryquerycachekey.cs
- PassportPrincipal.cs
- GeometryConverter.cs
- EventLogPermissionEntryCollection.cs
- IsolatedStorageException.cs
- ControlTemplate.cs
- ModelUtilities.cs
- PostBackTrigger.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- ProviderException.cs
- PeerInvitationResponse.cs
- VersionPair.cs
- SourceLineInfo.cs
- ExtensionFile.cs
- MemberHolder.cs
- Stack.cs