Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Reflection / StrongNameKeyPair.cs / 1305376 / StrongNameKeyPair.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** File: StrongNameKeyPair.cs ** **[....] ** ** ** Purpose: Encapsulate access to a public/private key pair ** used to sign strong name assemblies. ** ** ===========================================================*/ namespace System.Reflection { using System; using System.IO; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Runtime.Versioning; using Microsoft.Win32; using System.Diagnostics.Contracts; #if !FEATURE_CORECLR using Microsoft.Runtime.Hosting; #endif [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class StrongNameKeyPair : IDeserializationCallback, ISerializable { private bool _keyPairExported; private byte[] _keyPairArray; private String _keyPairContainer; private byte[] _publicKey; // Build key pair from file. [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(FileStream keyPairFile) { if (keyPairFile == null) throw new ArgumentNullException("keyPairFile"); Contract.EndContractBlock(); int length = (int)keyPairFile.Length; _keyPairArray = new byte[length]; keyPairFile.Read(_keyPairArray, 0, length); _keyPairExported = true; } // Build key pair from byte array in memory. [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(byte[] keyPairArray) { if (keyPairArray == null) throw new ArgumentNullException("keyPairArray"); Contract.EndContractBlock(); _keyPairArray = new byte[keyPairArray.Length]; Array.Copy(keyPairArray, _keyPairArray, keyPairArray.Length); _keyPairExported = true; } // Reference key pair in named key container. [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(String keyPairContainer) { if (keyPairContainer == null) throw new ArgumentNullException("keyPairContainer"); Contract.EndContractBlock(); _keyPairContainer = keyPairContainer; _keyPairExported = false; } [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected StrongNameKeyPair (SerializationInfo info, StreamingContext context) { _keyPairExported = (bool) info.GetValue("_keyPairExported", typeof(bool)); _keyPairArray = (byte[]) info.GetValue("_keyPairArray", typeof(byte[])); _keyPairContainer = (string) info.GetValue("_keyPairContainer", typeof(string)); _publicKey = (byte[]) info.GetValue("_publicKey", typeof(byte[])); } // Get the public portion of the key pair. public byte[] PublicKey { [System.Security.SecuritySafeCritical] // auto-generated get { if (_publicKey == null) { _publicKey = ComputePublicKey(); } byte[] publicKey = new byte[_publicKey.Length]; Array.Copy(_publicKey, publicKey, _publicKey.Length); return publicKey; } } #if FEATURE_CORECLR [System.Security.SecurityCritical] // auto-generated private unsafe byte[] ComputePublicKey() { byte[] publicKey = null; // Make sure pbPublicKey is not leaked with async exceptions RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { byte* pbPublicKey = null; int cbPublicKey = 0; try { bool result; if (_keyPairExported) { result = Win32Native.StrongNameGetPublicKey(null, _keyPairArray, _keyPairArray.Length, out pbPublicKey, out cbPublicKey); } else { result = Win32Native.StrongNameGetPublicKey(_keyPairContainer, null, 0, out pbPublicKey, out cbPublicKey); } if (!result) throw new ArgumentException(Environment.GetResourceString("Argument_StrongNameGetPublicKey")); publicKey = new byte[cbPublicKey]; Buffer.memcpy(pbPublicKey, 0, publicKey, 0, cbPublicKey); } finally { if (pbPublicKey != null) Win32Native.StrongNameFreeBuffer(pbPublicKey); } } return publicKey; } #else // FEATURE_CORECLR [System.Security.SecurityCritical] // auto-generated private unsafe byte[] ComputePublicKey() { byte[] publicKey = null; // Make sure pbPublicKey is not leaked with async exceptions RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { IntPtr pbPublicKey = IntPtr.Zero; int cbPublicKey = 0; try { bool result; if (_keyPairExported) { result = StrongNameHelpers.StrongNameGetPublicKey(null, _keyPairArray, _keyPairArray.Length, out pbPublicKey, out cbPublicKey); } else { result = StrongNameHelpers.StrongNameGetPublicKey(_keyPairContainer, null, 0, out pbPublicKey, out cbPublicKey); } if (!result) throw new ArgumentException(Environment.GetResourceString("Argument_StrongNameGetPublicKey")); publicKey = new byte[cbPublicKey]; Buffer.memcpy((byte*) (pbPublicKey.ToPointer()), 0, publicKey, 0, cbPublicKey); } finally { if (pbPublicKey != IntPtr.Zero) StrongNameHelpers.StrongNameFreeBuffer(pbPublicKey); } } return publicKey; } #endif // FEATURE_CORECLR #if FEATURE_SERIALIZATION ///[System.Security.SecurityCritical] void ISerializable.GetObjectData (SerializationInfo info, StreamingContext context) { info.AddValue("_keyPairExported", _keyPairExported); info.AddValue("_keyPairArray", _keyPairArray); info.AddValue("_keyPairContainer", _keyPairContainer); info.AddValue("_publicKey", _publicKey); } /// void IDeserializationCallback.OnDeserialization (Object sender) {} #endif // Internal routine used to retrieve key pair info from unmanaged code. private bool GetKeyPair(out Object arrayOrContainer) { arrayOrContainer = _keyPairExported ? (Object)_keyPairArray : (Object)_keyPairContainer; return _keyPairExported; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** File: StrongNameKeyPair.cs ** ** [....] ** ** ** Purpose: Encapsulate access to a public/private key pair ** used to sign strong name assemblies. ** ** ===========================================================*/ namespace System.Reflection { using System; using System.IO; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Runtime.Versioning; using Microsoft.Win32; using System.Diagnostics.Contracts; #if !FEATURE_CORECLR using Microsoft.Runtime.Hosting; #endif [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class StrongNameKeyPair : IDeserializationCallback, ISerializable { private bool _keyPairExported; private byte[] _keyPairArray; private String _keyPairContainer; private byte[] _publicKey; // Build key pair from file. [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(FileStream keyPairFile) { if (keyPairFile == null) throw new ArgumentNullException("keyPairFile"); Contract.EndContractBlock(); int length = (int)keyPairFile.Length; _keyPairArray = new byte[length]; keyPairFile.Read(_keyPairArray, 0, length); _keyPairExported = true; } // Build key pair from byte array in memory. [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(byte[] keyPairArray) { if (keyPairArray == null) throw new ArgumentNullException("keyPairArray"); Contract.EndContractBlock(); _keyPairArray = new byte[keyPairArray.Length]; Array.Copy(keyPairArray, _keyPairArray, keyPairArray.Length); _keyPairExported = true; } // Reference key pair in named key container. [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(String keyPairContainer) { if (keyPairContainer == null) throw new ArgumentNullException("keyPairContainer"); Contract.EndContractBlock(); _keyPairContainer = keyPairContainer; _keyPairExported = false; } [System.Security.SecuritySafeCritical] // auto-generated [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected StrongNameKeyPair (SerializationInfo info, StreamingContext context) { _keyPairExported = (bool) info.GetValue("_keyPairExported", typeof(bool)); _keyPairArray = (byte[]) info.GetValue("_keyPairArray", typeof(byte[])); _keyPairContainer = (string) info.GetValue("_keyPairContainer", typeof(string)); _publicKey = (byte[]) info.GetValue("_publicKey", typeof(byte[])); } // Get the public portion of the key pair. public byte[] PublicKey { [System.Security.SecuritySafeCritical] // auto-generated get { if (_publicKey == null) { _publicKey = ComputePublicKey(); } byte[] publicKey = new byte[_publicKey.Length]; Array.Copy(_publicKey, publicKey, _publicKey.Length); return publicKey; } } #if FEATURE_CORECLR [System.Security.SecurityCritical] // auto-generated private unsafe byte[] ComputePublicKey() { byte[] publicKey = null; // Make sure pbPublicKey is not leaked with async exceptions RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { byte* pbPublicKey = null; int cbPublicKey = 0; try { bool result; if (_keyPairExported) { result = Win32Native.StrongNameGetPublicKey(null, _keyPairArray, _keyPairArray.Length, out pbPublicKey, out cbPublicKey); } else { result = Win32Native.StrongNameGetPublicKey(_keyPairContainer, null, 0, out pbPublicKey, out cbPublicKey); } if (!result) throw new ArgumentException(Environment.GetResourceString("Argument_StrongNameGetPublicKey")); publicKey = new byte[cbPublicKey]; Buffer.memcpy(pbPublicKey, 0, publicKey, 0, cbPublicKey); } finally { if (pbPublicKey != null) Win32Native.StrongNameFreeBuffer(pbPublicKey); } } return publicKey; } #else // FEATURE_CORECLR [System.Security.SecurityCritical] // auto-generated private unsafe byte[] ComputePublicKey() { byte[] publicKey = null; // Make sure pbPublicKey is not leaked with async exceptions RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { IntPtr pbPublicKey = IntPtr.Zero; int cbPublicKey = 0; try { bool result; if (_keyPairExported) { result = StrongNameHelpers.StrongNameGetPublicKey(null, _keyPairArray, _keyPairArray.Length, out pbPublicKey, out cbPublicKey); } else { result = StrongNameHelpers.StrongNameGetPublicKey(_keyPairContainer, null, 0, out pbPublicKey, out cbPublicKey); } if (!result) throw new ArgumentException(Environment.GetResourceString("Argument_StrongNameGetPublicKey")); publicKey = new byte[cbPublicKey]; Buffer.memcpy((byte*) (pbPublicKey.ToPointer()), 0, publicKey, 0, cbPublicKey); } finally { if (pbPublicKey != IntPtr.Zero) StrongNameHelpers.StrongNameFreeBuffer(pbPublicKey); } } return publicKey; } #endif // FEATURE_CORECLR #if FEATURE_SERIALIZATION ///[System.Security.SecurityCritical] void ISerializable.GetObjectData (SerializationInfo info, StreamingContext context) { info.AddValue("_keyPairExported", _keyPairExported); info.AddValue("_keyPairArray", _keyPairArray); info.AddValue("_keyPairContainer", _keyPairContainer); info.AddValue("_publicKey", _publicKey); } /// void IDeserializationCallback.OnDeserialization (Object sender) {} #endif // Internal routine used to retrieve key pair info from unmanaged code. private bool GetKeyPair(out Object arrayOrContainer) { arrayOrContainer = _keyPairExported ? (Object)_keyPairArray : (Object)_keyPairContainer; return _keyPairExported; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
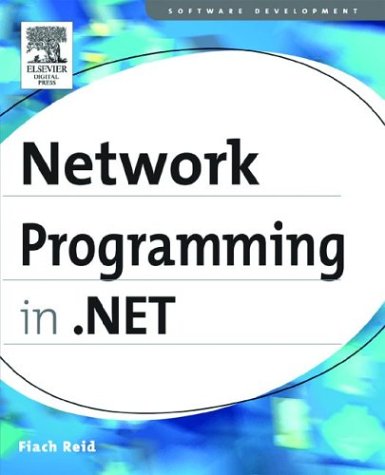
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Shared.cs
- ToolStripDropDownButton.cs
- TransformationRules.cs
- SemaphoreFullException.cs
- RootBrowserWindowAutomationPeer.cs
- ProtectedProviderSettings.cs
- SecurityKeyIdentifierClause.cs
- IDReferencePropertyAttribute.cs
- DataSourceSelectArguments.cs
- BitmapFrame.cs
- SerialPort.cs
- MarkupExtensionParser.cs
- Control.cs
- BaseConfigurationRecord.cs
- OdbcTransaction.cs
- JsonCollectionDataContract.cs
- DataSourceHelper.cs
- SerializerProvider.cs
- RelativeSource.cs
- FileIOPermission.cs
- SystemFonts.cs
- PassportAuthenticationModule.cs
- ResolvedKeyFrameEntry.cs
- SID.cs
- KeyInfo.cs
- XmlCustomFormatter.cs
- JobDuplex.cs
- TypeValidationEventArgs.cs
- ItemList.cs
- ToolStripManager.cs
- TagMapInfo.cs
- shaper.cs
- SqlAliaser.cs
- RecognizeCompletedEventArgs.cs
- InlineObject.cs
- CompatibleComparer.cs
- DataGridViewLinkCell.cs
- DataColumnMappingCollection.cs
- OperationCanceledException.cs
- SvcMapFileSerializer.cs
- SQLInt16Storage.cs
- SettingsProviderCollection.cs
- LinqDataSourceContextEventArgs.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- ShapingEngine.cs
- XamlFigureLengthSerializer.cs
- PolyQuadraticBezierSegment.cs
- xsdvalidator.cs
- Formatter.cs
- ConsoleCancelEventArgs.cs
- BookmarkEventArgs.cs
- ParentQuery.cs
- Transform3D.cs
- ResponseStream.cs
- ProfileParameter.cs
- TypeNameHelper.cs
- ExecutionContext.cs
- DbConnectionPoolGroupProviderInfo.cs
- DiffuseMaterial.cs
- RemotingSurrogateSelector.cs
- FaultPropagationRecord.cs
- PointLight.cs
- Image.cs
- TraceListener.cs
- DataGridViewHitTestInfo.cs
- DataGridViewCheckBoxCell.cs
- ShimAsPublicXamlType.cs
- securitycriticaldataformultiplegetandset.cs
- ScriptModule.cs
- UnknownWrapper.cs
- _ConnectStream.cs
- WindowsSpinner.cs
- SessionPageStateSection.cs
- FirewallWrapper.cs
- VisualStyleElement.cs
- WindowsGraphics.cs
- PrintPreviewControl.cs
- Win32Exception.cs
- Globals.cs
- PropertyBuilder.cs
- CompModSwitches.cs
- ThreadAbortException.cs
- Separator.cs
- WebPartTransformer.cs
- TextFragmentEngine.cs
- Lock.cs
- OleDbRowUpdatedEvent.cs
- SystemGatewayIPAddressInformation.cs
- EntityViewGenerationAttribute.cs
- AppDomainResourcePerfCounters.cs
- FileAuthorizationModule.cs
- SoapProcessingBehavior.cs
- BindingMemberInfo.cs
- xml.cs
- SizeChangedEventArgs.cs
- ResolvedKeyFrameEntry.cs
- RequestUriProcessor.cs
- TextBlockAutomationPeer.cs
- DetailsViewDeletedEventArgs.cs
- InvariantComparer.cs