Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Runtime / Remoting / RemotingSurrogateSelector.cs / 1 / RemotingSurrogateSelector.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Runtime.Remoting.Messaging { using System; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Remoting.Metadata; using System.Runtime.Remoting.Activation; using System.Runtime.Remoting.Proxies; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters; using System.Text; using System.Reflection; using System.Threading; using System.Globalization; using System.Collections; using System.Security.Permissions; [System.Runtime.InteropServices.ComVisible(true)] public delegate bool MessageSurrogateFilter(String key, Object value); [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] [SecurityPermissionAttribute(SecurityAction.InheritanceDemand, Flags=SecurityPermissionFlag.Infrastructure)] [System.Runtime.InteropServices.ComVisible(true)] public class RemotingSurrogateSelector : ISurrogateSelector { // Private static data private static Type s_IMethodCallMessageType = typeof(IMethodCallMessage); private static Type s_IMethodReturnMessageType = typeof(IMethodReturnMessage); private static Type s_ObjRefType = typeof(ObjRef); // Private member data private Object _rootObj = null; private ISurrogateSelector _next = null; private RemotingSurrogate _remotingSurrogate = new RemotingSurrogate(); private ObjRefSurrogate _objRefSurrogate = new ObjRefSurrogate(); private ISerializationSurrogate _messageSurrogate = null; private MessageSurrogateFilter _filter = null; public RemotingSurrogateSelector() { _messageSurrogate = new MessageSurrogate(this); } public MessageSurrogateFilter Filter { set { _filter = value; } get { return _filter; } } public void SetRootObject(Object obj) { if (obj == null) { throw new ArgumentNullException("obj"); } _rootObj = obj; SoapMessageSurrogate soapMsg = _messageSurrogate as SoapMessageSurrogate; if (null != soapMsg) { soapMsg.SetRootObject(_rootObj); } } public Object GetRootObject() { return _rootObj; } // Specifies the next ISurrogateSelector to be examined for surrogates if the current // instance doesn't have a surrogate for the given type and assembly in the given context. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual void ChainSelector(ISurrogateSelector selector) {_next = selector;} // Returns the appropriate surrogate for the given type in the given context. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual ISerializationSurrogate GetSurrogate(Type type, StreamingContext context, out ISurrogateSelector ssout) { if (type == null) { throw new ArgumentNullException("type"); } Message.DebugOut("Entered GetSurrogate for " + type.FullName + "\n"); if (type.IsMarshalByRef) { Message.DebugOut("Selected surrogate for " + type.FullName); ssout = this; return _remotingSurrogate; } else if (s_IMethodCallMessageType.IsAssignableFrom(type) || s_IMethodReturnMessageType.IsAssignableFrom(type)) { ssout = this; return _messageSurrogate; } else if (s_ObjRefType.IsAssignableFrom(type)) { ssout = this; return _objRefSurrogate; } else if (_next != null) { return _next.GetSurrogate(type, context, out ssout); } else { ssout = null; return null; } } // GetSurrogate [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual ISurrogateSelector GetNextSelector() { return _next;} public virtual void UseSoapFormat() { _messageSurrogate = new SoapMessageSurrogate(this); ((SoapMessageSurrogate)_messageSurrogate).SetRootObject(_rootObj); } } internal class RemotingSurrogate : ISerializationSurrogate { [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual void GetObjectData(Object obj, SerializationInfo info, StreamingContext context) { if (obj == null) { throw new ArgumentNullException("obj"); } if (info==null) { throw new ArgumentNullException("info"); } // // This code is to special case marshalling types inheriting from RemotingClientProxy // Check whether type inherits from RemotingClientProxy and serialize the correct ObjRef // after getting the correct proxy to the actual server object // Message.DebugOut("RemotingSurrogate::GetObjectData obj.Type: " + obj.GetType().FullName + " \n"); if(RemotingServices.IsTransparentProxy(obj)) { RealProxy rp = RemotingServices.GetRealProxy(obj); rp.GetObjectData(info, context); } else { RemotingServices.GetObjectData(obj, info, context); } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual Object SetObjectData(Object obj, SerializationInfo info, StreamingContext context, ISurrogateSelector selector) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_PopulateData")); } } // class RemotingSurrogate internal class ObjRefSurrogate : ISerializationSurrogate { [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual void GetObjectData(Object obj, SerializationInfo info, StreamingContext context) { if (obj == null) { throw new ArgumentNullException("obj"); } if (info==null) { throw new ArgumentNullException("info"); } // // This code is to provide special handling for ObjRef's that are supposed // to be passed as parameters. // ((ObjRef)obj).GetObjectData(info, context); // add flag indicating the ObjRef was passed as a parameter info.AddValue("fIsMarshalled", 0); } // GetObjectData [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual Object SetObjectData(Object obj, SerializationInfo info, StreamingContext context, ISurrogateSelector selector) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_PopulateData")); } } // class ObjRefSurrogate internal class SoapMessageSurrogate : ISerializationSurrogate { // Private static data private static Type _voidType = typeof(void); private static Type _soapFaultType = typeof(SoapFault); // Member data String DefaultFakeRecordAssemblyName = "http://schemas.microsoft.com/urt/SystemRemotingSoapTopRecord"; Object _rootObj = null; RemotingSurrogateSelector _ss; internal SoapMessageSurrogate(RemotingSurrogateSelector ss) { _ss = ss; } internal void SetRootObject(Object obj) { _rootObj = obj; } internal virtual String[] GetInArgNames(IMethodCallMessage m, int c) { String[] names = new String[c]; for (int i = 0; i < c; i++) { String name = m.GetInArgName(i); if (name == null) { name = "__param" + i; } names[i] = name; } return names; } internal virtual String[] GetNames(IMethodCallMessage m, int c) { String[] names = new String[c]; for (int i = 0; i < c; i++) { String name = m.GetArgName(i); if (name == null) { name = "__param" + i; } names[i] = name; } return names; } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual void GetObjectData(Object obj, SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } if ( (obj!=null) && (obj !=_rootObj)) { (new MessageSurrogate(_ss)).GetObjectData(obj, info, context); } else { IMethodReturnMessage msg = obj as IMethodReturnMessage; if(null != msg) { if (msg.Exception == null) { String responseElementName; String responseElementNS; String returnElementName; // obtain response element name namespace MethodBase mb = msg.MethodBase; SoapMethodAttribute attr = (SoapMethodAttribute)InternalRemotingServices.GetCachedSoapAttribute(mb); responseElementName = attr.ResponseXmlElementName; responseElementNS = attr.ResponseXmlNamespace; returnElementName = attr.ReturnXmlElementName; ArgMapper mapper = new ArgMapper(msg, true /*fOut*/); Object[] args = mapper.Args; info.FullTypeName = responseElementName; info.AssemblyName = responseElementNS; Type retType = ((MethodInfo)mb).ReturnType; if (!((retType == null) || (retType == _voidType))) { info.AddValue(returnElementName, msg.ReturnValue, retType); } if (args != null) { Type[] types = mapper.ArgTypes; for (int i=0; i
Link Menu
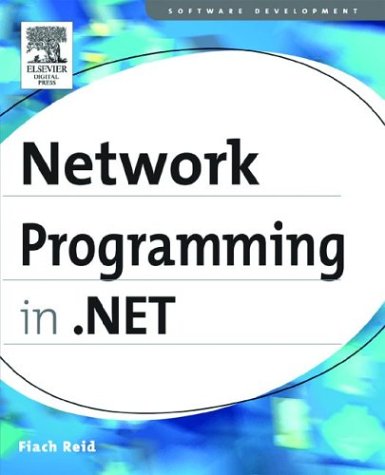
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SourceFileBuildProvider.cs
- FacetDescriptionElement.cs
- WpfKnownType.cs
- HttpApplication.cs
- SecurityManager.cs
- RegexWorker.cs
- MailMessage.cs
- ImageBrush.cs
- SchemaNames.cs
- ControlBuilderAttribute.cs
- XpsFixedPageReaderWriter.cs
- ScriptBehaviorDescriptor.cs
- DataGridTableCollection.cs
- SerializationObjectManager.cs
- IsolatedStoragePermission.cs
- SwitchLevelAttribute.cs
- XmlAttributeCache.cs
- ImageDrawing.cs
- _TLSstream.cs
- mil_sdk_version.cs
- AccessibilityHelperForXpWin2k3.cs
- PriorityQueue.cs
- SurrogateEncoder.cs
- templategroup.cs
- CreateUserWizard.cs
- WSSecureConversationFeb2005.cs
- TemplateControl.cs
- CompressEmulationStream.cs
- WebRequestModulesSection.cs
- versioninfo.cs
- XslAstAnalyzer.cs
- baseaxisquery.cs
- SessionEndingEventArgs.cs
- Set.cs
- QueryCacheManager.cs
- DataContractAttribute.cs
- DefaultTypeArgumentAttribute.cs
- StructuredType.cs
- ValidationRule.cs
- LineUtil.cs
- MLangCodePageEncoding.cs
- CultureTableRecord.cs
- DispatcherExceptionFilterEventArgs.cs
- WorkflowFileItem.cs
- EventDescriptor.cs
- ComAdminInterfaces.cs
- InProcStateClientManager.cs
- XmlSchemaImport.cs
- ToolStripArrowRenderEventArgs.cs
- ListItemConverter.cs
- XmlIncludeAttribute.cs
- XmlEncodedRawTextWriter.cs
- ImageCreator.cs
- SiteOfOriginContainer.cs
- ClientFormsIdentity.cs
- HttpRuntime.cs
- DataBinder.cs
- HttpListenerException.cs
- DrawingDrawingContext.cs
- AdornerPresentationContext.cs
- ObservableDictionary.cs
- _HeaderInfo.cs
- UnauthorizedAccessException.cs
- MsmqInputChannelListener.cs
- SourceElementsCollection.cs
- CollaborationHelperFunctions.cs
- SignedInfo.cs
- DefaultMemberAttribute.cs
- JavaScriptObjectDeserializer.cs
- WinEventTracker.cs
- NativeMethods.cs
- PeerEndPoint.cs
- Cursor.cs
- DatatypeImplementation.cs
- AtlasWeb.Designer.cs
- Pkcs7Recipient.cs
- ModelItemCollection.cs
- VScrollBar.cs
- AuthenticationSchemesHelper.cs
- _SingleItemRequestCache.cs
- MergeLocalizationDirectives.cs
- ScriptingJsonSerializationSection.cs
- RuntimeEnvironment.cs
- XpsS0ValidatingLoader.cs
- ContractsBCL.cs
- RemoteCryptoDecryptRequest.cs
- DiscardableAttribute.cs
- ResizingMessageFilter.cs
- TimerEventSubscriptionCollection.cs
- CheckBoxBaseAdapter.cs
- HttpSessionStateBase.cs
- ContextStack.cs
- HwndHostAutomationPeer.cs
- OrderByLifter.cs
- DesignerValidatorAdapter.cs
- TouchDevice.cs
- EntitySqlQueryBuilder.cs
- StateRuntime.cs
- DesignColumnCollection.cs
- EntityDataSourceStatementEditorForm.cs