Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / TimerEventSubscriptionCollection.cs / 1305376 / TimerEventSubscriptionCollection.cs
using System; using System.Collections.Generic; using System.Text; using System.Workflow.Runtime.Hosting; using System.Workflow.ComponentModel; using System.Diagnostics; using System.Collections; namespace System.Workflow.Runtime { [Serializable] public class TimerEventSubscriptionCollection : ICollection { public readonly static DependencyProperty TimerCollectionProperty = DependencyProperty.RegisterAttached("TimerCollection", typeof(TimerEventSubscriptionCollection), typeof(TimerEventSubscriptionCollection)); private object locker = new Object(); private KeyedPriorityQueuequeue = new KeyedPriorityQueue (); #pragma warning disable 0414 private bool suspended = false; // no longer used but required for binary compatibility of serialization format #pragma warning restore 0414 [NonSerialized] private IWorkflowCoreRuntime executor; private Guid instanceId; internal TimerEventSubscriptionCollection(IWorkflowCoreRuntime executor, Guid instanceId) { this.executor = executor; this.instanceId = instanceId; WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Created", instanceId); this.queue.FirstElementChanged += OnFirstElementChanged; } internal void Enqueue(TimerEventSubscription timerEventSubscription) { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Enqueue Timer {1} for {2} ", instanceId, timerEventSubscription.SubscriptionId, timerEventSubscription.ExpiresAt); queue.Enqueue(timerEventSubscription.SubscriptionId, timerEventSubscription, timerEventSubscription.ExpiresAt); } } internal IWorkflowCoreRuntime Executor { get { return executor; } set { executor = value; } } public TimerEventSubscription Peek() { lock (locker) { return queue.Peek(); } } internal TimerEventSubscription Dequeue() { lock (locker) { TimerEventSubscription retval = queue.Dequeue(); if(retval != null) WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Dequeue Timer {1} for {2} ", instanceId, retval.SubscriptionId, retval.ExpiresAt); return retval; } } public void Remove(Guid timerSubscriptionId) { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Remove Timer {1}", instanceId, timerSubscriptionId); queue.Remove(timerSubscriptionId); } } private void OnFirstElementChanged(object source, KeyedPriorityQueueHeadChangedEventArgs e) { lock (locker) { ITimerService timerService = this.executor.GetService(typeof(ITimerService)) as ITimerService; if (e.NewFirstElement != null && executor != null) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Schedule Timer {1} for {2} ", instanceId, e.NewFirstElement.SubscriptionId, e.NewFirstElement.ExpiresAt); timerService.ScheduleTimer(executor.ProcessTimersCallback, e.NewFirstElement.WorkflowInstanceId, e.NewFirstElement.ExpiresAt, e.NewFirstElement.SubscriptionId); } if (e.OldFirstElement != null) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Unschedule Timer {1} for {2} ", instanceId, e.OldFirstElement.SubscriptionId, e.OldFirstElement.ExpiresAt); timerService.CancelTimer(e.OldFirstElement.SubscriptionId); } } } internal void SuspendDelivery() { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Suspend", instanceId); WorkflowSchedulerService schedulerService = this.executor.GetService(typeof(WorkflowSchedulerService)) as WorkflowSchedulerService; TimerEventSubscription sub = queue.Peek(); if (sub != null) { schedulerService.Cancel(sub.SubscriptionId); } } } internal void ResumeDelivery() { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Resume", instanceId); WorkflowSchedulerService schedulerService = this.executor.GetService(typeof(WorkflowSchedulerService)) as WorkflowSchedulerService; TimerEventSubscription sub = queue.Peek(); if (sub != null) { schedulerService.Schedule(executor.ProcessTimersCallback, sub.WorkflowInstanceId, sub.ExpiresAt, sub.SubscriptionId); } } } public void Add(TimerEventSubscription item) { if (item == null) throw new ArgumentNullException("item"); this.Enqueue(item); } public void Remove(TimerEventSubscription item) { if (item == null) throw new ArgumentNullException("item"); this.Remove(item.SubscriptionId); } #region ICollection Members public void CopyTo(Array array, int index) { TimerEventSubscription[] tes = null; lock (locker) { tes = new TimerEventSubscription[queue.Count]; queue.Values.CopyTo(tes, 0); } if(tes != null) tes.CopyTo(array, index); } public int Count { get { return queue.Count; } } public bool IsSynchronized { get { return true; } } public object SyncRoot { get { return locker; } } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { return queue.Values.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
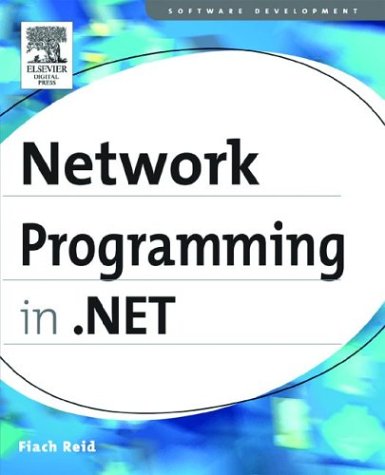
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableLayoutStyleCollection.cs
- SchemaElementDecl.cs
- JournalNavigationScope.cs
- PlacementWorkspace.cs
- BlurBitmapEffect.cs
- VolatileResourceManager.cs
- SurrogateEncoder.cs
- GlyphRunDrawing.cs
- DataGridViewIntLinkedList.cs
- SafeArrayTypeMismatchException.cs
- SubclassTypeValidator.cs
- BinaryFormatterWriter.cs
- FormsAuthenticationTicket.cs
- OracleConnectionFactory.cs
- ColorMap.cs
- XmlIlTypeHelper.cs
- Validator.cs
- ObjectStorage.cs
- SecurityCriticalDataForSet.cs
- OdbcRowUpdatingEvent.cs
- PeerTransportListenAddressValidatorAttribute.cs
- WmlValidatorAdapter.cs
- SmtpMail.cs
- HtmlLink.cs
- ValidationUtility.cs
- HostedHttpRequestAsyncResult.cs
- ErrorWrapper.cs
- DataDocumentXPathNavigator.cs
- DurableEnlistmentState.cs
- RayHitTestParameters.cs
- Part.cs
- SQLCharsStorage.cs
- OSEnvironmentHelper.cs
- ToolStripCustomTypeDescriptor.cs
- xmlglyphRunInfo.cs
- Int32CollectionConverter.cs
- XmlSchemaAppInfo.cs
- SimpleType.cs
- VisualStyleTypesAndProperties.cs
- SystemWebSectionGroup.cs
- AutoGeneratedFieldProperties.cs
- HostProtectionPermission.cs
- CaseStatement.cs
- MetadataArtifactLoader.cs
- NavigationEventArgs.cs
- BaseCollection.cs
- DbProviderFactories.cs
- XmlUtf8RawTextWriter.cs
- FrameworkContextData.cs
- _BufferOffsetSize.cs
- WhitespaceReader.cs
- ProfilePropertyNameValidator.cs
- SymDocumentType.cs
- UriParserTemplates.cs
- Scene3D.cs
- PolicyReader.cs
- VectorAnimationUsingKeyFrames.cs
- ResourceSet.cs
- Knowncolors.cs
- ComNativeDescriptor.cs
- X500Name.cs
- TextLineBreak.cs
- KeyTimeConverter.cs
- SafeThemeHandle.cs
- ContractCodeDomInfo.cs
- XmlDownloadManager.cs
- FormsAuthenticationEventArgs.cs
- IdentityVerifier.cs
- RichTextBoxAutomationPeer.cs
- WinInetCache.cs
- DataSetUtil.cs
- SourceSwitch.cs
- PackageProperties.cs
- TTSVoice.cs
- _AutoWebProxyScriptEngine.cs
- MouseBinding.cs
- XmlLanguageConverter.cs
- XmlSchemaChoice.cs
- CreateParams.cs
- Properties.cs
- FunctionOverloadResolver.cs
- SchemaEntity.cs
- ViewgenContext.cs
- SqlBuffer.cs
- UserControlParser.cs
- _WebProxyDataBuilder.cs
- EdmTypeAttribute.cs
- ObjectDisposedException.cs
- ToolStripGripRenderEventArgs.cs
- DependencyPropertyDescriptor.cs
- EditCommandColumn.cs
- RequestCacheEntry.cs
- WebPartsSection.cs
- RequestFactory.cs
- MimeMapping.cs
- OleDbErrorCollection.cs
- XamlRtfConverter.cs
- CodeTypeDeclarationCollection.cs
- ValidatorCompatibilityHelper.cs
- EqualityComparer.cs