Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Generated / PolyQuadraticBezierSegment.cs / 1 / PolyQuadraticBezierSegment.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class PolyQuadraticBezierSegment : PathSegment { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new PolyQuadraticBezierSegment Clone() { return (PolyQuadraticBezierSegment)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new PolyQuadraticBezierSegment CloneCurrentValue() { return (PolyQuadraticBezierSegment)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Points - PointCollection. Default value is new FreezableDefaultValueFactory(PointCollection.Empty). /// public PointCollection Points { get { return (PointCollection) GetValue(PointsProperty); } set { SetValueInternal(PointsProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PolyQuadraticBezierSegment(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Points // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the PolyQuadraticBezierSegment.Points property. /// public static readonly DependencyProperty PointsProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static PointCollection s_Points = PointCollection.Empty; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static PolyQuadraticBezierSegment() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Points == null || s_Points.IsFrozen, "Detected context bound default value PolyQuadraticBezierSegment.s_Points (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(PolyQuadraticBezierSegment); PointsProperty = RegisterProperty("Points", typeof(PointCollection), typeofThis, new FreezableDefaultValueFactory(PointCollection.Empty), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class PolyQuadraticBezierSegment : PathSegment { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new PolyQuadraticBezierSegment Clone() { return (PolyQuadraticBezierSegment)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new PolyQuadraticBezierSegment CloneCurrentValue() { return (PolyQuadraticBezierSegment)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Points - PointCollection. Default value is new FreezableDefaultValueFactory(PointCollection.Empty). /// public PointCollection Points { get { return (PointCollection) GetValue(PointsProperty); } set { SetValueInternal(PointsProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PolyQuadraticBezierSegment(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Points // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the PolyQuadraticBezierSegment.Points property. /// public static readonly DependencyProperty PointsProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static PointCollection s_Points = PointCollection.Empty; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static PolyQuadraticBezierSegment() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Points == null || s_Points.IsFrozen, "Detected context bound default value PolyQuadraticBezierSegment.s_Points (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(PolyQuadraticBezierSegment); PointsProperty = RegisterProperty("Points", typeof(PointCollection), typeofThis, new FreezableDefaultValueFactory(PointCollection.Empty), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
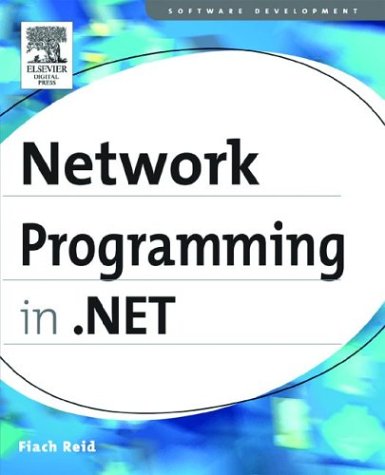
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpProfileGroupBase.cs
- PointCollectionConverter.cs
- WS2007FederationHttpBinding.cs
- ArrayWithOffset.cs
- TypeExtensionSerializer.cs
- SerializerDescriptor.cs
- Pool.cs
- TypeValidationEventArgs.cs
- CustomSignedXml.cs
- DecimalAnimation.cs
- PropertyTab.cs
- MouseActionValueSerializer.cs
- SourceFileBuildProvider.cs
- CaseInsensitiveOrdinalStringComparer.cs
- graph.cs
- CommandValueSerializer.cs
- LiteralControl.cs
- SmtpCommands.cs
- AdvancedBindingEditor.cs
- XmlWriter.cs
- CommandPlan.cs
- MD5.cs
- DataBindingHandlerAttribute.cs
- SignalGate.cs
- Model3D.cs
- DictionarySectionHandler.cs
- DataServiceStreamResponse.cs
- FormCollection.cs
- FlowDocumentReader.cs
- Stacktrace.cs
- XsdBuildProvider.cs
- LinqDataSourceDisposeEventArgs.cs
- SelectedGridItemChangedEvent.cs
- ArrayListCollectionBase.cs
- CheckBoxList.cs
- XmlChildNodes.cs
- SqlLiftWhereClauses.cs
- documentsequencetextcontainer.cs
- DbFunctionCommandTree.cs
- RegexRunnerFactory.cs
- SimpleType.cs
- PropertyInfoSet.cs
- FunctionDescription.cs
- SmtpFailedRecipientsException.cs
- baseaxisquery.cs
- ConsumerConnectionPoint.cs
- SqlTypeConverter.cs
- LocalizeDesigner.cs
- propertyentry.cs
- ValueQuery.cs
- PrintPreviewGraphics.cs
- HitTestParameters3D.cs
- SeekStoryboard.cs
- CompilationUnit.cs
- DataGridViewRowPrePaintEventArgs.cs
- EventLogPermissionEntry.cs
- DateTime.cs
- HtmlInputControl.cs
- ReferentialConstraint.cs
- UInt64Storage.cs
- ScaleTransform3D.cs
- querybuilder.cs
- CheckBoxRenderer.cs
- CanonicalFontFamilyReference.cs
- BroadcastEventHelper.cs
- QilNode.cs
- DuplicateDetector.cs
- SoapFormatter.cs
- HandlerFactoryCache.cs
- RoleManagerModule.cs
- GeneralTransform3D.cs
- AttachInfo.cs
- CFGGrammar.cs
- HtmlTableRow.cs
- ChannelSinkStacks.cs
- StyleBamlTreeBuilder.cs
- ImageMapEventArgs.cs
- ExclusiveCanonicalizationTransform.cs
- DataIdProcessor.cs
- ExpressionPrefixAttribute.cs
- SystemFonts.cs
- FontTypeConverter.cs
- QueryCacheEntry.cs
- OutputCacheProfileCollection.cs
- XsdBuildProvider.cs
- WorkerRequest.cs
- SessionStateSection.cs
- FamilyMap.cs
- ToolBar.cs
- CalendarSelectionChangedEventArgs.cs
- DecoratedNameAttribute.cs
- EventPropertyMap.cs
- ChangesetResponse.cs
- TreeNodeStyle.cs
- StorageModelBuildProvider.cs
- SchemaExporter.cs
- KeyGestureValueSerializer.cs
- SqlGenerator.cs
- SpellerInterop.cs
- EntitySqlQueryCacheEntry.cs