Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DLinq / Dlinq / SqlClient / Query / SqlLiftWhereClauses.cs / 1 / SqlLiftWhereClauses.cs
using System; using System.Collections.Generic; using System.Text; using System.Data.Linq.Mapping; using System.Data.Linq.Provider; namespace System.Data.Linq.SqlClient { ////// Hoist WHERE clauses as close to the root as possible. /// class SqlLiftWhereClauses { internal static SqlNode Lift(SqlNode node, TypeSystemProvider typeProvider, MetaModel model) { return new Lifter(typeProvider, model).Visit(node); } class Lifter : SqlVisitor { private class Scope { internal Scope Parent; internal SqlExpression Where; internal Scope(SqlExpression where, Scope parent) { this.Where = where; this.Parent = parent; } }; Scope current; SqlFactory sql; SqlAggregateChecker aggregateChecker; SqlRowNumberChecker rowNumberChecker; internal Lifter(TypeSystemProvider typeProvider, MetaModel model) { this.sql = new SqlFactory(typeProvider, model); this.aggregateChecker = new SqlAggregateChecker(); this.rowNumberChecker = new SqlRowNumberChecker(); } internal override SqlSelect VisitSelect(SqlSelect select) { Scope save = this.current; this.current = new Scope(select.Where, this.current); SqlSelect result = base.VisitSelect(select); bool stopHoisting = select.IsDistinct || select.GroupBy.Count > 0 || this.aggregateChecker.HasAggregates(select) || select.Top != null || this.rowNumberChecker.HasRowNumber(select); // Shift as much of the current WHERE to the parent as possible. if (this.current != null) { if (this.current.Parent != null && !stopHoisting) { this.current.Parent.Where = sql.AndAccumulate(this.current.Parent.Where, this.current.Where); this.current.Where = null; } select.Where = this.current.Where; } this.current = save; return result; } internal override SqlNode VisitUnion(SqlUnion su) { Scope save = this.current; this.current = null; SqlNode result = base.VisitUnion(su); this.current = save; return result; } internal override SqlSource VisitJoin(SqlJoin join) { // block where clauses from being lifted out of the cardinality-dependent // side of an outer join. Scope save = this.current; try { switch (join.JoinType) { case SqlJoinType.Cross: case SqlJoinType.CrossApply: case SqlJoinType.Inner: return base.VisitJoin(join); case SqlJoinType.LeftOuter: case SqlJoinType.OuterApply: { join.Left = this.VisitSource(join.Left); this.current = null; join.Right = this.VisitSource(join.Right); join.Condition = this.VisitExpression(join.Condition); return join; } default: this.current = null; return base.VisitJoin(join); } } finally { this.current = save; } } internal override SqlExpression VisitSubSelect(SqlSubSelect ss) { // block where clauses from being lifted out of a sub-query Scope save = this.current; this.current = null; SqlExpression result = base.VisitSubSelect(ss); this.current = save; return result; } internal override SqlExpression VisitClientQuery(SqlClientQuery cq) { // block where clauses from being lifted out of a client-materialized sub-query Scope save = this.current; this.current = null; SqlExpression result = base.VisitClientQuery(cq); this.current = save; return result; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; using System.Data.Linq.Mapping; using System.Data.Linq.Provider; namespace System.Data.Linq.SqlClient { ////// Hoist WHERE clauses as close to the root as possible. /// class SqlLiftWhereClauses { internal static SqlNode Lift(SqlNode node, TypeSystemProvider typeProvider, MetaModel model) { return new Lifter(typeProvider, model).Visit(node); } class Lifter : SqlVisitor { private class Scope { internal Scope Parent; internal SqlExpression Where; internal Scope(SqlExpression where, Scope parent) { this.Where = where; this.Parent = parent; } }; Scope current; SqlFactory sql; SqlAggregateChecker aggregateChecker; SqlRowNumberChecker rowNumberChecker; internal Lifter(TypeSystemProvider typeProvider, MetaModel model) { this.sql = new SqlFactory(typeProvider, model); this.aggregateChecker = new SqlAggregateChecker(); this.rowNumberChecker = new SqlRowNumberChecker(); } internal override SqlSelect VisitSelect(SqlSelect select) { Scope save = this.current; this.current = new Scope(select.Where, this.current); SqlSelect result = base.VisitSelect(select); bool stopHoisting = select.IsDistinct || select.GroupBy.Count > 0 || this.aggregateChecker.HasAggregates(select) || select.Top != null || this.rowNumberChecker.HasRowNumber(select); // Shift as much of the current WHERE to the parent as possible. if (this.current != null) { if (this.current.Parent != null && !stopHoisting) { this.current.Parent.Where = sql.AndAccumulate(this.current.Parent.Where, this.current.Where); this.current.Where = null; } select.Where = this.current.Where; } this.current = save; return result; } internal override SqlNode VisitUnion(SqlUnion su) { Scope save = this.current; this.current = null; SqlNode result = base.VisitUnion(su); this.current = save; return result; } internal override SqlSource VisitJoin(SqlJoin join) { // block where clauses from being lifted out of the cardinality-dependent // side of an outer join. Scope save = this.current; try { switch (join.JoinType) { case SqlJoinType.Cross: case SqlJoinType.CrossApply: case SqlJoinType.Inner: return base.VisitJoin(join); case SqlJoinType.LeftOuter: case SqlJoinType.OuterApply: { join.Left = this.VisitSource(join.Left); this.current = null; join.Right = this.VisitSource(join.Right); join.Condition = this.VisitExpression(join.Condition); return join; } default: this.current = null; return base.VisitJoin(join); } } finally { this.current = save; } } internal override SqlExpression VisitSubSelect(SqlSubSelect ss) { // block where clauses from being lifted out of a sub-query Scope save = this.current; this.current = null; SqlExpression result = base.VisitSubSelect(ss); this.current = save; return result; } internal override SqlExpression VisitClientQuery(SqlClientQuery cq) { // block where clauses from being lifted out of a client-materialized sub-query Scope save = this.current; this.current = null; SqlExpression result = base.VisitClientQuery(cq); this.current = save; return result; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
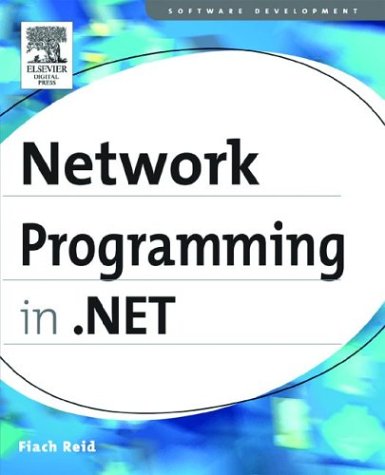
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseAsyncResult.cs
- OutputCacheSection.cs
- ToolStripManager.cs
- DataServiceResponse.cs
- Script.cs
- SpStreamWrapper.cs
- AQNBuilder.cs
- HScrollBar.cs
- Vector3DAnimationBase.cs
- DescendentsWalkerBase.cs
- QilScopedVisitor.cs
- SQLRoleProvider.cs
- RightsController.cs
- ToolStripSystemRenderer.cs
- VisualStyleElement.cs
- WebPartPersonalization.cs
- ProviderConnectionPoint.cs
- HostedHttpTransportManager.cs
- AutoResizedEvent.cs
- SQLByteStorage.cs
- IndexerNameAttribute.cs
- ReferentialConstraintRoleElement.cs
- Stylesheet.cs
- TextElementEnumerator.cs
- ToolStripGrip.cs
- StreamUpdate.cs
- BindingContext.cs
- ConfigXmlSignificantWhitespace.cs
- SafeViewOfFileHandle.cs
- ValidationError.cs
- MailDefinition.cs
- TextElementCollectionHelper.cs
- Logging.cs
- BinHexDecoder.cs
- BypassElementCollection.cs
- SafeNativeMethods.cs
- FixedHyperLink.cs
- FontSourceCollection.cs
- ReflectTypeDescriptionProvider.cs
- ServiceContractListItemList.cs
- MethodBody.cs
- Debugger.cs
- RuleAction.cs
- FileSystemInfo.cs
- GridItemPatternIdentifiers.cs
- FixedSOMLineRanges.cs
- Interfaces.cs
- HttpCookieCollection.cs
- FormsAuthenticationEventArgs.cs
- HttpAsyncResult.cs
- BinaryFormatterSinks.cs
- PrivilegeNotHeldException.cs
- QuotedPairReader.cs
- SqlCacheDependencyDatabaseCollection.cs
- DataViewSettingCollection.cs
- LassoHelper.cs
- base64Transforms.cs
- DataGridItemEventArgs.cs
- PointLight.cs
- FixedTextPointer.cs
- BuilderPropertyEntry.cs
- ClientUtils.cs
- DiagnosticEventProvider.cs
- BuilderInfo.cs
- SystemFonts.cs
- EncoderBestFitFallback.cs
- BinaryMethodMessage.cs
- StickyNote.cs
- HtmlMeta.cs
- safesecurityhelperavalon.cs
- ClrPerspective.cs
- TableLayoutSettings.cs
- PlaceHolder.cs
- BaseResourcesBuildProvider.cs
- EventMap.cs
- HttpException.cs
- Base64Encoding.cs
- Panel.cs
- CustomValidator.cs
- MsmqIntegrationProcessProtocolHandler.cs
- TextLine.cs
- DependencyPropertyKey.cs
- ProfessionalColors.cs
- _TransmitFileOverlappedAsyncResult.cs
- XmlNodeList.cs
- SingleConverter.cs
- SequenceQuery.cs
- EdmProperty.cs
- WasEndpointConfigContainer.cs
- TagNameToTypeMapper.cs
- KeysConverter.cs
- OpCopier.cs
- TemplateControlBuildProvider.cs
- KeyValueConfigurationElement.cs
- ResolveCriteria11.cs
- KeyValueInternalCollection.cs
- DateTimeValueSerializerContext.cs
- ISO2022Encoding.cs
- PriorityChain.cs
- DefaultHttpHandler.cs