Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Configuration / OutputCacheSection.cs / 5 / OutputCacheSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class OutputCacheSection : ConfigurationSection { internal const bool DefaultOmitVaryStar = false; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnableOutputCache = new ConfigurationProperty("enableOutputCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableFragmentCache = new ConfigurationProperty("enableFragmentCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSendCacheControlHeader = new ConfigurationProperty("sendCacheControlHeader", typeof(bool), HttpRuntimeSection.DefaultSendCacheControlHeader, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propOmitVaryStar = new ConfigurationProperty("omitVaryStar", typeof(bool), DefaultOmitVaryStar, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableKernelCacheForVaryByStar = new ConfigurationProperty("enableKernelCacheForVaryByStar", typeof(bool), false, ConfigurationPropertyOptions.None); private bool sendCacheControlHeaderCached = false; private bool sendCacheControlHeaderCache; private bool omitVaryStarCached = false; private bool omitVaryStar; private bool enableKernelCacheForVaryByStarCached = false; private bool enableKernelCacheForVaryByStar; private bool enableOutputCacheCached = false; private bool enableOutputCache; static OutputCacheSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnableOutputCache); _properties.Add(_propEnableFragmentCache); _properties.Add(_propSendCacheControlHeader); _properties.Add(_propOmitVaryStar); _properties.Add(_propEnableKernelCacheForVaryByStar); } public OutputCacheSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enableOutputCache", DefaultValue = true)] public bool EnableOutputCache { get { if (enableOutputCacheCached == false) { enableOutputCache = (bool)base[_propEnableOutputCache]; enableOutputCacheCached = true; } return enableOutputCache; } set { base[_propEnableOutputCache] = value; enableOutputCache = value; } } [ConfigurationProperty("enableFragmentCache", DefaultValue = true)] public bool EnableFragmentCache { get { return (bool)base[_propEnableFragmentCache]; } set { base[_propEnableFragmentCache] = value; } } [ConfigurationProperty("sendCacheControlHeader", DefaultValue = HttpRuntimeSection.DefaultSendCacheControlHeader)] public bool SendCacheControlHeader { get { if (sendCacheControlHeaderCached == false) { sendCacheControlHeaderCache = (bool)base[_propSendCacheControlHeader]; sendCacheControlHeaderCached = true; } return sendCacheControlHeaderCache; } set { base[_propSendCacheControlHeader] = value; sendCacheControlHeaderCache = value; } } [ConfigurationProperty("omitVaryStar", DefaultValue = DefaultOmitVaryStar)] public bool OmitVaryStar { get { if (omitVaryStarCached == false) { omitVaryStar = (bool)base[_propOmitVaryStar]; omitVaryStarCached = true; } return omitVaryStar; } set { base[_propOmitVaryStar] = value; omitVaryStar = value; } } [ConfigurationProperty("enableKernelCacheForVaryByStar", DefaultValue = false)] public bool EnableKernelCacheForVaryByStar { get { if (enableKernelCacheForVaryByStarCached == false) { enableKernelCacheForVaryByStar = (bool)base[_propEnableKernelCacheForVaryByStar]; enableKernelCacheForVaryByStarCached = true; } return enableKernelCacheForVaryByStar; } set { base[_propEnableKernelCacheForVaryByStar] = value; enableKernelCacheForVaryByStar = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class OutputCacheSection : ConfigurationSection { internal const bool DefaultOmitVaryStar = false; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnableOutputCache = new ConfigurationProperty("enableOutputCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableFragmentCache = new ConfigurationProperty("enableFragmentCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSendCacheControlHeader = new ConfigurationProperty("sendCacheControlHeader", typeof(bool), HttpRuntimeSection.DefaultSendCacheControlHeader, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propOmitVaryStar = new ConfigurationProperty("omitVaryStar", typeof(bool), DefaultOmitVaryStar, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableKernelCacheForVaryByStar = new ConfigurationProperty("enableKernelCacheForVaryByStar", typeof(bool), false, ConfigurationPropertyOptions.None); private bool sendCacheControlHeaderCached = false; private bool sendCacheControlHeaderCache; private bool omitVaryStarCached = false; private bool omitVaryStar; private bool enableKernelCacheForVaryByStarCached = false; private bool enableKernelCacheForVaryByStar; private bool enableOutputCacheCached = false; private bool enableOutputCache; static OutputCacheSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnableOutputCache); _properties.Add(_propEnableFragmentCache); _properties.Add(_propSendCacheControlHeader); _properties.Add(_propOmitVaryStar); _properties.Add(_propEnableKernelCacheForVaryByStar); } public OutputCacheSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enableOutputCache", DefaultValue = true)] public bool EnableOutputCache { get { if (enableOutputCacheCached == false) { enableOutputCache = (bool)base[_propEnableOutputCache]; enableOutputCacheCached = true; } return enableOutputCache; } set { base[_propEnableOutputCache] = value; enableOutputCache = value; } } [ConfigurationProperty("enableFragmentCache", DefaultValue = true)] public bool EnableFragmentCache { get { return (bool)base[_propEnableFragmentCache]; } set { base[_propEnableFragmentCache] = value; } } [ConfigurationProperty("sendCacheControlHeader", DefaultValue = HttpRuntimeSection.DefaultSendCacheControlHeader)] public bool SendCacheControlHeader { get { if (sendCacheControlHeaderCached == false) { sendCacheControlHeaderCache = (bool)base[_propSendCacheControlHeader]; sendCacheControlHeaderCached = true; } return sendCacheControlHeaderCache; } set { base[_propSendCacheControlHeader] = value; sendCacheControlHeaderCache = value; } } [ConfigurationProperty("omitVaryStar", DefaultValue = DefaultOmitVaryStar)] public bool OmitVaryStar { get { if (omitVaryStarCached == false) { omitVaryStar = (bool)base[_propOmitVaryStar]; omitVaryStarCached = true; } return omitVaryStar; } set { base[_propOmitVaryStar] = value; omitVaryStar = value; } } [ConfigurationProperty("enableKernelCacheForVaryByStar", DefaultValue = false)] public bool EnableKernelCacheForVaryByStar { get { if (enableKernelCacheForVaryByStarCached == false) { enableKernelCacheForVaryByStar = (bool)base[_propEnableKernelCacheForVaryByStar]; enableKernelCacheForVaryByStarCached = true; } return enableKernelCacheForVaryByStar; } set { base[_propEnableKernelCacheForVaryByStar] = value; enableKernelCacheForVaryByStar = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
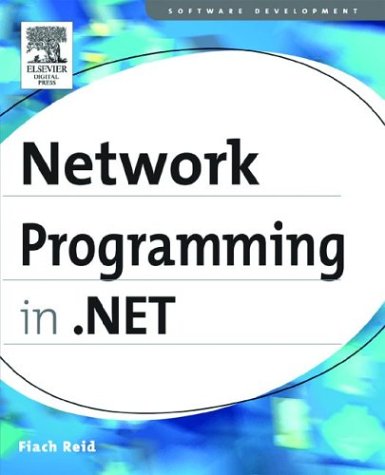
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeometryDrawing.cs
- RequestSecurityTokenForGetBrowserToken.cs
- GlyphInfoList.cs
- Collection.cs
- HandleCollector.cs
- DictionaryKeyPropertyAttribute.cs
- Deserializer.cs
- ImageField.cs
- ValueExpressions.cs
- complextypematerializer.cs
- HttpCapabilitiesBase.cs
- ErrorEventArgs.cs
- ListViewDeleteEventArgs.cs
- DataGridViewButtonColumn.cs
- XmlMapping.cs
- UIElementCollection.cs
- InputGestureCollection.cs
- CqlQuery.cs
- HtmlInputReset.cs
- SmiGettersStream.cs
- CriticalHandle.cs
- ClientConfigurationHost.cs
- EntityContainer.cs
- InputScopeConverter.cs
- CodeGenerator.cs
- CompensationHandlingFilter.cs
- PropertyManager.cs
- WebPartsPersonalizationAuthorization.cs
- AssemblyAttributesGoHere.cs
- LineBreakRecord.cs
- DrawingGroup.cs
- TimeSpanValidatorAttribute.cs
- FileDialog_Vista.cs
- ParameterCollectionEditor.cs
- CapabilitiesAssignment.cs
- DictionaryEntry.cs
- ListViewItem.cs
- Operand.cs
- ScriptModule.cs
- TextRangeEdit.cs
- DataControlFieldHeaderCell.cs
- UserNameSecurityToken.cs
- Margins.cs
- LinqDataSourceContextEventArgs.cs
- LazyInitializer.cs
- Converter.cs
- BaseValidator.cs
- UInt32Storage.cs
- COM2ColorConverter.cs
- CodeExporter.cs
- ExceptionRoutedEventArgs.cs
- SqlFlattener.cs
- CustomLineCap.cs
- HorizontalAlignConverter.cs
- __FastResourceComparer.cs
- ItemCollection.cs
- FixedFindEngine.cs
- IsolatedStorageSecurityState.cs
- InlineObject.cs
- SymLanguageVendor.cs
- FixedSOMElement.cs
- ProxyFragment.cs
- DataGridViewRowCancelEventArgs.cs
- UniqueConstraint.cs
- XmlDomTextWriter.cs
- glyphs.cs
- XmlTypeAttribute.cs
- SplitterCancelEvent.cs
- Grant.cs
- BrowserInteropHelper.cs
- SmiRequestExecutor.cs
- ScrollContentPresenter.cs
- configsystem.cs
- InlineUIContainer.cs
- FunctionMappingTranslator.cs
- LayoutTableCell.cs
- GridPatternIdentifiers.cs
- HttpRawResponse.cs
- XmlWriterTraceListener.cs
- NameValuePair.cs
- XmlDataCollection.cs
- ReflectionTypeLoadException.cs
- CodeExpressionStatement.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- XmlDataSource.cs
- ThrowHelper.cs
- IgnorePropertiesAttribute.cs
- WindowsListViewItem.cs
- RectAnimationBase.cs
- BitmapPalettes.cs
- ThemeInfoAttribute.cs
- PrimaryKeyTypeConverter.cs
- Tokenizer.cs
- AssemblyResourceLoader.cs
- CodeCatchClauseCollection.cs
- PreviewPrintController.cs
- RowSpanVector.cs
- SiteMapSection.cs
- HostedElements.cs
- CodeDirectiveCollection.cs