Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / ReverseInheritProperty.cs / 2 / ReverseInheritProperty.cs
using System; using System.Collections; using System.Windows.Media; using System.Windows.Input; using MS.Internal; namespace System.Windows { internal abstract class ReverseInheritProperty { internal ReverseInheritProperty( DependencyPropertyKey flagKey, CoreFlags flagCache, CoreFlags flagChanged) { FlagKey = flagKey; FlagCache = flagCache; FlagChanged = flagChanged; } internal abstract void FireNotifications(UIElement uie, ContentElement ce, UIElement3D uie3D, bool oldValue); internal void OnOriginValueChanged(DependencyObject oldOrigin, DependencyObject newOrigin, ref DeferredElementTreeState oldTreeState) { DeferredElementTreeState treeStateLocalCopy = oldTreeState; oldTreeState = null; // Step #1 // Update the cache flags for all elements in the ancestry // of the element that got turned off and record the changed nodes if (oldOrigin != null) { SetCacheFlagInAncestry(oldOrigin, false, treeStateLocalCopy); } // Step #2 // Update the cache flags for all elements in the ancestry // of the element that got turned on and record the changed nodes if (newOrigin != null) { SetCacheFlagInAncestry(newOrigin, true, null); } // Step #3 // Fire value changed on elements in the ancestry of the element that got turned off. if (oldOrigin != null) { FirePropertyChangeInAncestry(oldOrigin, true /* oldValue */, treeStateLocalCopy); } // Step #4 // Fire value changed on elements in the ancestry of the element that got turned on. if (newOrigin != null) { FirePropertyChangeInAncestry(newOrigin, false /* oldValue */, null); } if (oldTreeState == null && treeStateLocalCopy != null) { // Now that we have applied the old tree state, throw it away. treeStateLocalCopy.Clear(); oldTreeState = treeStateLocalCopy; } } void SetCacheFlagInAncestry(DependencyObject element, bool newValue, DeferredElementTreeState treeState) { // If the cache flag value is undergoing change, record it and // propagate the change to the ancestors. if ((!newValue && IsFlagSet(element, FlagCache)) || (newValue && !IsFlagSet(element, FlagCache))) { SetFlag(element, FlagCache, newValue); // NOTE: we toggle the changed flag instead of setting it so that that way common // ancestors show resultant unchanged and do not receive any change notification. SetFlag(element, FlagChanged, !IsFlagSet(element, FlagChanged)); // Check for block reverse inheritance flag, elements like popup want to set this. { UIElement uie = element as UIElement; if (uie != null) { if (uie.BlockReverseInheritance()) { return; } } ContentElement ce = element as ContentElement; if (ce != null) { if (ce.BlockReverseInheritance()) { return; } } UIElement3D ui3D = element as UIElement3D; if (ui3D != null) { if (ui3D.BlockReverseInheritance()) { return; } } } // Propagate the flag up the visual and logical trees. Note our // minimal optimization check to avoid walking both the core // and logical parents if they are the same. { DependencyObject coreParent = DeferredElementTreeState.GetInputElementParent(element, treeState); DependencyObject logicalParent = DeferredElementTreeState.GetLogicalParent(element, treeState); if (coreParent != null) { SetCacheFlagInAncestry(coreParent, newValue, treeState); } if (logicalParent != null && logicalParent != coreParent) { SetCacheFlagInAncestry(logicalParent, newValue, treeState); } } } } void FirePropertyChangeInAncestry(DependencyObject element, bool oldValue, DeferredElementTreeState treeState) { // If the cache value on the owner has been changed fire changed event if (IsFlagSet(element, FlagChanged)) { SetFlag(element, FlagChanged, false); if (oldValue) { element.ClearValue(FlagKey); } else { element.SetValue(FlagKey, true); } UIElement uie = element as UIElement; ContentElement ce = (uie != null) ? null : element as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : element as UIElement3D; FireNotifications(uie, ce, uie3D, oldValue); // Check for block reverse inheritance flag, elements like popup want to set this. if (uie != null) { if (uie.BlockReverseInheritance()) { return; } } else if (ce != null) { if (ce.BlockReverseInheritance()) { return; } } else if (uie3D != null) { if (uie3D.BlockReverseInheritance()) { return; } } // Call FirePropertyChange up the visual and logical trees. // Note our minimal optimization check to avoid walking both // the core and logical parents if they are the same. { DependencyObject coreParent = DeferredElementTreeState.GetInputElementParent(element, treeState); DependencyObject logicalParent = DeferredElementTreeState.GetLogicalParent(element, treeState); if (coreParent != null) { FirePropertyChangeInAncestry(coreParent, oldValue, treeState); } if (logicalParent != null && logicalParent != coreParent) { FirePropertyChangeInAncestry(logicalParent, oldValue, treeState); } } } } ///////////////////////////////////////////////////////////////////// void SetFlag(DependencyObject o, CoreFlags flag, bool value) { UIElement uie = o as UIElement; ContentElement ce = (uie != null) ? null : o as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : o as UIElement3D; if (uie != null) { uie.WriteFlag(flag, value); } else if (ce != null) { ce.WriteFlag(flag, value); } else if (uie3D != null) { uie3D.WriteFlag(flag, value); } } ///////////////////////////////////////////////////////////////////// bool IsFlagSet(DependencyObject o, CoreFlags flag) { UIElement uie = o as UIElement; ContentElement ce = (uie != null) ? null : o as ContentElement; UIElement3D uie3D = (uie != null || ce != null) ? null : o as UIElement3D; if (uie != null) { return uie.ReadFlag(flag); } else if (ce != null) { return ce.ReadFlag(flag); } else if (uie3D != null) { return uie3D.ReadFlag(flag); } return false; } ///////////////////////////////////////////////////////////////////// internal DependencyPropertyKey FlagKey; internal CoreFlags FlagCache, FlagChanged; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
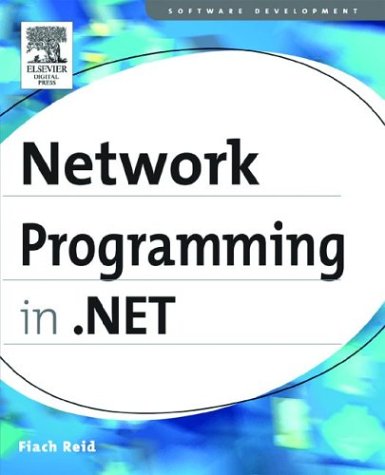
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializationInfo.cs
- ResourceContainer.cs
- StorageModelBuildProvider.cs
- GeometryValueSerializer.cs
- ManualResetEventSlim.cs
- SHA384.cs
- ImpersonationContext.cs
- SessionSymmetricTransportSecurityProtocolFactory.cs
- MatrixTransform.cs
- TransactedReceiveData.cs
- CqlIdentifiers.cs
- Baml2006Reader.cs
- WebEventTraceProvider.cs
- FileUpload.cs
- XPathPatternParser.cs
- BindingListCollectionView.cs
- SafeHandles.cs
- ResourceIDHelper.cs
- streamingZipPartStream.cs
- MissingMethodException.cs
- DockAndAnchorLayout.cs
- SendKeys.cs
- CustomCategoryAttribute.cs
- HuffModule.cs
- ProviderSettings.cs
- TrustLevel.cs
- QueryCacheEntry.cs
- Content.cs
- PropertyChangingEventArgs.cs
- StaticContext.cs
- ReadOnlyHierarchicalDataSource.cs
- JumpTask.cs
- CheckBoxStandardAdapter.cs
- Object.cs
- CommandHelper.cs
- EntityCollection.cs
- WebContext.cs
- Geometry.cs
- ProcessModuleCollection.cs
- LoopExpression.cs
- ProcessModule.cs
- HtmlShimManager.cs
- XmlQueryStaticData.cs
- StandardBindingImporter.cs
- PolyQuadraticBezierSegment.cs
- FeatureSupport.cs
- ServiceReference.cs
- SmiRequestExecutor.cs
- VirtualizingStackPanel.cs
- XslCompiledTransform.cs
- XPathConvert.cs
- ColorConvertedBitmap.cs
- Sequence.cs
- UriExt.cs
- DesignerWebPartChrome.cs
- CounterCreationDataCollection.cs
- XamlFxTrace.cs
- SplashScreen.cs
- DataColumn.cs
- Keywords.cs
- MetaDataInfo.cs
- FontUnit.cs
- CorrelationManager.cs
- HelpEvent.cs
- RectConverter.cs
- InternalConfigSettingsFactory.cs
- ColorMap.cs
- CompilationUnit.cs
- ObjectConverter.cs
- AttributeEmitter.cs
- SrgsSemanticInterpretationTag.cs
- CurrentTimeZone.cs
- DictionaryContent.cs
- CounterSetInstance.cs
- AssociationSetMetadata.cs
- EllipticalNodeOperations.cs
- TextServicesCompartmentEventSink.cs
- ParserStreamGeometryContext.cs
- CqlParserHelpers.cs
- ISAPIRuntime.cs
- ListBoxAutomationPeer.cs
- SerializationSectionGroup.cs
- SystemResources.cs
- CompressionTracing.cs
- StructuralCache.cs
- ScopeElement.cs
- HttpCacheParams.cs
- NameTable.cs
- ConfigXmlWhitespace.cs
- BasicExpandProvider.cs
- ScalarType.cs
- ClientRolePrincipal.cs
- ConstraintCollection.cs
- NameSpaceExtractor.cs
- HybridDictionary.cs
- HttpClientChannel.cs
- GeneralTransformGroup.cs
- ErasingStroke.cs
- ExitEventArgs.cs
- SQLChars.cs