Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / HtmlShimManager.cs / 1 / HtmlShimManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections.Generic; using System.Collections; using System.Diagnostics; ////// HtmlShimManager - this class manages the shims for HtmlWindows, HtmlDocuments, and HtmlElements. /// essentially we need a long-lasting object to call back on events from the web browser, and the /// manager is the one in charge of making sure this list stays around as long as needed. /// /// When a HtmlWindow unloads we prune our list of corresponding document, window, and element shims. /// /// internal sealed class HtmlShimManager :IDisposable { private DictionaryhtmlWindowShims; private Dictionary htmlElementShims; private Dictionary htmlDocumentShims; internal HtmlShimManager() { } /// AddDocumentShim - adds a HtmlDocumentShim to list of shims to manage /// Can create a WindowShim as a side effect so it knows when to self prune from the list. /// public void AddDocumentShim(HtmlDocument doc) { HtmlDocument.HtmlDocumentShim shim = null; if (htmlDocumentShims == null) { htmlDocumentShims = new Dictionary(); shim = new HtmlDocument.HtmlDocumentShim(doc); htmlDocumentShims[doc] = shim; } else if (!htmlDocumentShims.ContainsKey(doc)) { shim = new HtmlDocument.HtmlDocumentShim(doc); htmlDocumentShims[doc] = shim; } if (shim != null) { OnShimAdded(shim); } } /// AddWindowShim - adds a HtmlWindowShim to list of shims to manage /// public void AddWindowShim(HtmlWindow window) { HtmlWindow.HtmlWindowShim shim = null; if (htmlWindowShims == null) { htmlWindowShims = new Dictionary(); shim = new HtmlWindow.HtmlWindowShim(window); htmlWindowShims[window] = shim; } else if (!htmlWindowShims.ContainsKey(window)) { shim = new HtmlWindow.HtmlWindowShim(window); htmlWindowShims[window] = shim; } if (shim != null) { // strictly not necessary, but here for future use. OnShimAdded(shim); } } /// AddElementShim - adds a HtmlDocumentShim to list of shims to manage /// Can create a WindowShim as a side effect so it knows when to self prune from the list. /// public void AddElementShim(HtmlElement element) { HtmlElement.HtmlElementShim shim = null; if (htmlElementShims == null) { htmlElementShims = new Dictionary(); shim = new HtmlElement.HtmlElementShim(element); htmlElementShims[element] = shim; } else if (!htmlElementShims.ContainsKey(element)) { shim = new HtmlElement.HtmlElementShim(element); htmlElementShims[element] = shim; } if (shim != null) { OnShimAdded(shim); } } internal HtmlDocument.HtmlDocumentShim GetDocumentShim(HtmlDocument document) { if (htmlDocumentShims == null) { return null; } if (htmlDocumentShims.ContainsKey(document)) { return htmlDocumentShims[document]; } return null; } internal HtmlElement.HtmlElementShim GetElementShim(HtmlElement element) { if (htmlElementShims == null) { return null; } if (htmlElementShims.ContainsKey(element)) { return htmlElementShims[element]; } return null; } internal HtmlWindow.HtmlWindowShim GetWindowShim(HtmlWindow window) { if (htmlWindowShims == null) { return null; } if (htmlWindowShims.ContainsKey(window)) { return htmlWindowShims[window]; } return null; } private void OnShimAdded(HtmlShim addedShim) { Debug.Assert(addedShim != null, "Why are we calling this with a null shim?"); if (addedShim != null) { if (!(addedShim is HtmlWindow.HtmlWindowShim)) { // we need to add a window shim here for documents and elements // so we can sync Window.Unload. The window shim itself will trap // the unload event and call back on us on OnWindowUnloaded. When // that happens we know we can free all our ptrs to COM. AddWindowShim(new HtmlWindow(this, addedShim.AssociatedWindow)); } } } /// /// HtmlWindowShim calls back on us when it has unloaded the page. At this point we need to /// walk through our lists and make sure we've cleaned up /// internal void OnWindowUnloaded(HtmlWindow unloadedWindow) { Debug.Assert(unloadedWindow != null, "Why are we calling this with a null window?"); if (unloadedWindow != null) { // // prune documents // if (htmlDocumentShims != null) { HtmlDocument.HtmlDocumentShim[] shims = new HtmlDocument.HtmlDocumentShim[htmlDocumentShims.Count]; htmlDocumentShims.Values.CopyTo(shims,0); foreach (HtmlDocument.HtmlDocumentShim shim in shims) { if (shim.AssociatedWindow == unloadedWindow.NativeHtmlWindow) { htmlDocumentShims.Remove(shim.Document); shim.Dispose(); } } } // // prune elements // if (htmlElementShims != null) { HtmlElement.HtmlElementShim[] shims = new HtmlElement.HtmlElementShim[htmlElementShims.Count]; htmlElementShims.Values.CopyTo(shims,0); foreach (HtmlElement.HtmlElementShim shim in shims) { if (shim.AssociatedWindow == unloadedWindow.NativeHtmlWindow) { htmlElementShims.Remove(shim.Element); shim.Dispose(); } } } // // prune the particular window from the list. // if (htmlWindowShims != null) { if (htmlWindowShims.ContainsKey(unloadedWindow)) { HtmlWindow.HtmlWindowShim shim = htmlWindowShims[unloadedWindow]; htmlWindowShims.Remove(unloadedWindow); shim.Dispose(); } } } } public void Dispose() { Dispose(true); } private void Dispose(bool disposing) { if (disposing) { if (htmlElementShims != null){ foreach (HtmlElement.HtmlElementShim shim in htmlElementShims.Values) { shim.Dispose(); } } if (htmlDocumentShims != null) { foreach (HtmlDocument.HtmlDocumentShim shim in htmlDocumentShims.Values) { shim.Dispose(); } } if (htmlWindowShims != null) { foreach (HtmlWindow.HtmlWindowShim shim in htmlWindowShims.Values) { shim.Dispose(); } } htmlWindowShims = null; htmlDocumentShims = null; htmlWindowShims = null; } } ~HtmlShimManager() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
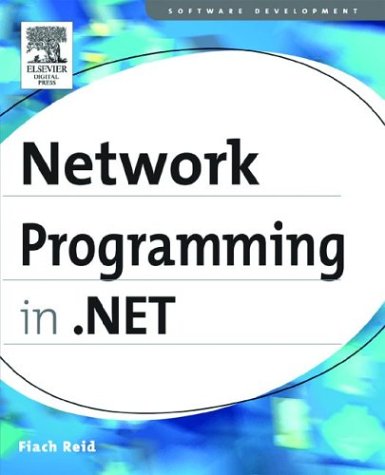
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Camera.cs
- MethodToken.cs
- ConstraintManager.cs
- DomainUpDown.cs
- XmlRawWriter.cs
- TextContainerChangeEventArgs.cs
- SystemIPAddressInformation.cs
- WebBrowserBase.cs
- XmlSchemaComplexContent.cs
- XmlUtil.cs
- TabControlEvent.cs
- IImplicitResourceProvider.cs
- MarshalByValueComponent.cs
- XhtmlBasicPhoneCallAdapter.cs
- UIPermission.cs
- TabRenderer.cs
- Freezable.cs
- PersianCalendar.cs
- ClrProviderManifest.cs
- SequentialUshortCollection.cs
- ColumnWidthChangedEvent.cs
- DetailsViewRow.cs
- HttpDictionary.cs
- Duration.cs
- BitmapData.cs
- ComboBoxItem.cs
- ColorPalette.cs
- SerialErrors.cs
- ToolStripItem.cs
- AttributeParameterInfo.cs
- DisplayInformation.cs
- SizeChangedInfo.cs
- TransformerInfo.cs
- IdentityNotMappedException.cs
- IOException.cs
- ElementUtil.cs
- WebPartEditVerb.cs
- MarkupExtensionParser.cs
- LinqDataSourceContextEventArgs.cs
- ServiceMoniker.cs
- NumberSubstitution.cs
- ScriptReferenceEventArgs.cs
- SqlParameterizer.cs
- TdsParserStateObject.cs
- SQLBoolean.cs
- QilReference.cs
- BreakSafeBase.cs
- DynamicResourceExtension.cs
- Faults.cs
- OutputCacheSettings.cs
- IBuiltInEvidence.cs
- SetState.cs
- RelatedCurrencyManager.cs
- ApplicationSecurityInfo.cs
- Point.cs
- XmlEnumAttribute.cs
- MetabaseSettingsIis7.cs
- Thread.cs
- BitmapFrameEncode.cs
- HtmlFormWrapper.cs
- MonitoringDescriptionAttribute.cs
- DataGridState.cs
- FilteredDataSetHelper.cs
- TargetInvocationException.cs
- ChannelServices.cs
- WebException.cs
- XpsImageSerializationService.cs
- EtwTrace.cs
- SelectedGridItemChangedEvent.cs
- ConnectionManagementElementCollection.cs
- Type.cs
- ParameterCollectionEditor.cs
- Enlistment.cs
- ListArgumentProvider.cs
- PieceDirectory.cs
- HWStack.cs
- ClientUtils.cs
- UrlEncodedParameterWriter.cs
- PermissionSetTriple.cs
- ContainerUIElement3D.cs
- ThemeableAttribute.cs
- GPRECT.cs
- RelationshipConverter.cs
- PathFigure.cs
- ElementProxy.cs
- Msec.cs
- InvokeCompletedEventArgs.cs
- TextCharacters.cs
- LockedHandleGlyph.cs
- Odbc32.cs
- EndPoint.cs
- TextSerializer.cs
- ComponentResourceManager.cs
- HideDisabledControlAdapter.cs
- NetworkCredential.cs
- XmlMembersMapping.cs
- DynamicValueConverter.cs
- Dump.cs
- HwndSourceKeyboardInputSite.cs
- MultiByteCodec.cs