Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / Emitters / AttributeEmitter.cs / 1 / AttributeEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); object[] keys = emitter.Item.KeyMembers.Select(km => (object) km.Name).ToArray(); typeDecl.CustomAttributes.Add(EmitSimpleAttribute(Utils.WebFrameworkCommonNamespace + "." + "DataServiceKeyAttribute", keys)); } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // not emitting System.Runtime.Serializaton.DataContractAttribute // not emitting System.Serializable } #region Static Methods ////// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { // not emitting System.Xml.Serialization.XmlIgnoreAttribute // not emitting System.Xml.Serialization.SoapIgnoreAttribute } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { // not emitting System.ComponentModel.BrowsableAttribute } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); object[] keys = emitter.Item.KeyMembers.Select(km => (object) km.Name).ToArray(); typeDecl.CustomAttributes.Add(EmitSimpleAttribute(Utils.WebFrameworkCommonNamespace + "." + "DataServiceKeyAttribute", keys)); } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // not emitting System.Runtime.Serializaton.DataContractAttribute // not emitting System.Serializable } #region Static Methods ////// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { // not emitting System.Xml.Serialization.XmlIgnoreAttribute // not emitting System.Xml.Serialization.SoapIgnoreAttribute } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { // not emitting System.ComponentModel.BrowsableAttribute } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
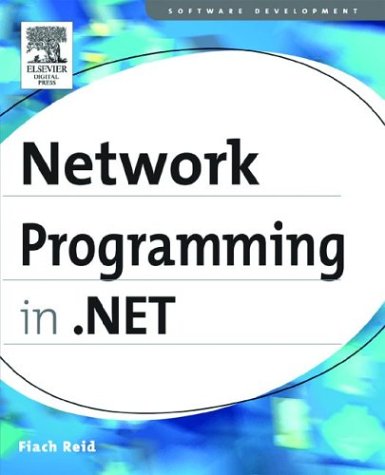
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamespaceInfo.cs
- DataServiceProviderWrapper.cs
- XmlSequenceWriter.cs
- SmiSettersStream.cs
- Button.cs
- WinFormsComponentEditor.cs
- RelationshipConstraintValidator.cs
- PathSegmentCollection.cs
- ProcessHost.cs
- ToolStripMenuItemDesigner.cs
- MatrixCamera.cs
- ModelItemImpl.cs
- XmlTextAttribute.cs
- OdbcConnectionStringbuilder.cs
- ScrollProviderWrapper.cs
- HttpProtocolImporter.cs
- OutputCacheProfile.cs
- PermissionSetTriple.cs
- EpmCustomContentSerializer.cs
- smtpconnection.cs
- ValueType.cs
- UriTemplateDispatchFormatter.cs
- WindowsListView.cs
- InputMethodStateTypeInfo.cs
- ConfigUtil.cs
- CmsInterop.cs
- CodePropertyReferenceExpression.cs
- DrawingBrush.cs
- DataStreamFromComStream.cs
- IMembershipProvider.cs
- SafeReadContext.cs
- HttpVersion.cs
- WebUtil.cs
- WindowsRebar.cs
- InstanceCreationEditor.cs
- PkcsUtils.cs
- XamlWriter.cs
- DataTableMappingCollection.cs
- CodeDomLoader.cs
- TerminatorSinks.cs
- Duration.cs
- VirtualPathProvider.cs
- XComponentModel.cs
- SqlConnectionHelper.cs
- AuthenticationServiceManager.cs
- TypeReference.cs
- VersionedStream.cs
- GeometryConverter.cs
- RNGCryptoServiceProvider.cs
- FormViewUpdateEventArgs.cs
- StorageInfo.cs
- DeviceOverridableAttribute.cs
- TextChange.cs
- HostedTransportConfigurationBase.cs
- ExceptionHandlers.cs
- InvalidWMPVersionException.cs
- PartDesigner.cs
- ProfilePropertySettings.cs
- LookupBindingPropertiesAttribute.cs
- SerializeAbsoluteContext.cs
- ImageInfo.cs
- MetadataPropertyvalue.cs
- SqlBuilder.cs
- WebPartDisplayModeCollection.cs
- DataSourceXmlSerializer.cs
- MissingMemberException.cs
- ISSmlParser.cs
- Monitor.cs
- PostBackOptions.cs
- DispatcherTimer.cs
- ToolStripItemImageRenderEventArgs.cs
- EnvelopedSignatureTransform.cs
- SqlClientWrapperSmiStream.cs
- InternalUserCancelledException.cs
- SignerInfo.cs
- EntityCodeGenerator.cs
- ReadOnlyCollectionBase.cs
- TypeInitializationException.cs
- ClientApiGenerator.cs
- TableLayoutStyleCollection.cs
- ImageButton.cs
- XamlGridLengthSerializer.cs
- SmtpFailedRecipientsException.cs
- ProxySimple.cs
- FigureParagraph.cs
- FileSystemEventArgs.cs
- Environment.cs
- EntityClientCacheEntry.cs
- Configuration.cs
- EventLog.cs
- NominalTypeEliminator.cs
- StylusShape.cs
- HandlerWithFactory.cs
- PropertyEntry.cs
- BmpBitmapEncoder.cs
- LinqDataSourceValidationException.cs
- ObjectToIdCache.cs
- RoleService.cs
- ValueTypePropertyReference.cs
- ConstraintCollection.cs