Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / MaskedTextBoxDesigner.cs / 1 / MaskedTextBoxDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System; using System.Design; using System.Collections; using System.Drawing.Design; using System.Windows.Forms; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; ////// Designer class for the MaskedTextBox control. /// internal class MaskedTextBoxDesigner : TextBoxBaseDesigner { private DesignerVerbCollection verbs; private DesignerActionListCollection actions; ////// MaskedTextBox designer action list property. Gets the design-time supported actions on the control. /// public override DesignerActionListCollection ActionLists { get { if (this.actions == null) { this.actions = new DesignerActionListCollection(); this.actions.Add(new MaskedTextBoxDesignerActionList(this) ); } return actions; } } ////// A utility method to get a design time masked text box based on the masked text box being designed. /// internal static MaskedTextBox GetDesignMaskedTextBox( MaskedTextBox mtb ) { MaskedTextBox designMtb = null; if( mtb == null ) { // return a default control. designMtb = new System.Windows.Forms.MaskedTextBox(); } else { MaskedTextProvider mtp = mtb.MaskedTextProvider; if( mtp == null ) { designMtb = new System.Windows.Forms.MaskedTextBox(); designMtb.Text = mtb.Text; } else { designMtb = new System.Windows.Forms.MaskedTextBox(mtb.MaskedTextProvider); } // Clone MTB properties. designMtb.ValidatingType = mtb.ValidatingType; designMtb.BeepOnError = mtb.BeepOnError; designMtb.InsertKeyMode = mtb.InsertKeyMode; designMtb.RejectInputOnFirstFailure = mtb.RejectInputOnFirstFailure; designMtb.CutCopyMaskFormat = mtb.CutCopyMaskFormat; designMtb.Culture = mtb.Culture; // designMtb.TextMaskFormat = mtb.TextMaskFormat; - Not relevant since it is to be used programatically only. } // Some constant properties at design time. designMtb.UseSystemPasswordChar = false; designMtb.PasswordChar = '\0'; designMtb.ReadOnly = false; designMtb.HidePromptOnLeave = false; return designMtb; } internal static string GetMaskInputRejectedErrorMessage( MaskInputRejectedEventArgs e ) { string rejectionHint; switch( e.RejectionHint ) { case MaskedTextResultHint.AsciiCharacterExpected: rejectionHint = SR.GetString( SR.MaskedTextBoxHintAsciiCharacterExpected ); break; case MaskedTextResultHint.AlphanumericCharacterExpected: rejectionHint = SR.GetString( SR.MaskedTextBoxHintAlphanumericCharacterExpected ); break; case MaskedTextResultHint.DigitExpected: rejectionHint = SR.GetString( SR.MaskedTextBoxHintDigitExpected ); break; case MaskedTextResultHint.LetterExpected: rejectionHint = SR.GetString( SR.MaskedTextBoxHintLetterExpected ); break; case MaskedTextResultHint.SignedDigitExpected: rejectionHint = SR.GetString( SR.MaskedTextBoxHintSignedDigitExpected ); break; case MaskedTextResultHint.PromptCharNotAllowed: rejectionHint = SR.GetString( SR.MaskedTextBoxHintPromptCharNotAllowed ); break; case MaskedTextResultHint.UnavailableEditPosition: rejectionHint = SR.GetString( SR.MaskedTextBoxHintUnavailableEditPosition ); break; case MaskedTextResultHint.NonEditPosition: rejectionHint = SR.GetString( SR.MaskedTextBoxHintNonEditPosition ); break; case MaskedTextResultHint.PositionOutOfRange: rejectionHint = SR.GetString( SR.MaskedTextBoxHintPositionOutOfRange ); break; case MaskedTextResultHint.InvalidInput: rejectionHint = SR.GetString( SR.MaskedTextBoxHintInvalidInput ); break; case MaskedTextResultHint.Unknown: default: Debug.Fail( "Unknown RejectionHint, defaulting to InvalidInput..." ); goto case MaskedTextResultHint.InvalidInput; } return string.Format(CultureInfo.CurrentCulture, SR.GetString(SR.MaskedTextBoxTextEditorErrorFormatString), e.Position, rejectionHint); } ////// Obsolete ComponentDesigner method which sets component default properties. Overriden to avoid setting /// the Mask improperly. /// [Obsolete("This method has been deprecated. Use InitializeNewComponent instead. http://go.microsoft.com/fwlink/?linkid=14202")] public override void OnSetComponentDefaults() { // do nothing. } ////// Event handler for the set mask verb. /// private void OnVerbSetMask(object sender, EventArgs e) { MaskedTextBoxDesignerActionList actionList = new MaskedTextBoxDesignerActionList(this); actionList.SetMask(); } ////// Allows a designer to filter the set of properties /// the component it is designing will expose through the /// TypeDescriptor object. This method is called /// immediately before its corresponding "Post" method. /// If you are overriding this method you should call /// the base implementation before you perform your own /// filtering. /// protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop; string[] shadowProps = new string[] { "Text", "PasswordChar" }; Attribute[] empty = new Attribute[0]; for (int i = 0; i < shadowProps.Length; i++) { prop = (PropertyDescriptor)properties[shadowProps[i]]; if (prop != null) { properties[shadowProps[i]] = TypeDescriptor.CreateProperty(typeof(MaskedTextBoxDesigner), prop, empty); } } } ////// Retrieves a set of rules concerning the movement capabilities of a component. /// This should be one or more flags from the SelectionRules class. If no designer /// provides rules for a component, the component will not get any UI services. /// public override SelectionRules SelectionRules { get { SelectionRules rules = base.SelectionRules; rules &= ~(SelectionRules.TopSizeable | SelectionRules.BottomSizeable); // Height is fixed. return rules; } } ////// Designe time support PasswordChar code serialization. /// //private bool ShouldSerializePasswordChar() //{ // return PasswordChar != '\0'; //} ////// Shadows the PasswordChar. UseSystemPasswordChar overrides PasswordChar so independent on the value /// of PasswordChar it will return the systemp password char. However, the value of PasswordChar is /// cached so if UseSystemPasswordChar is reset at design time the PasswordChar value can be restored. /// So in the case both properties are set, we need to serialize the real PasswordChar value as well. /// /// Note: This code was copied to TextBoxDesigner, if fixing a bug here it is probable that the same /// bug needs to be fixed there. /// private char PasswordChar { get { MaskedTextBox mtb = this.Control as MaskedTextBox; Debug.Assert(mtb != null, "Designed control is not a MaskedTextBox."); if (mtb.UseSystemPasswordChar) { mtb.UseSystemPasswordChar = false; char pwdChar = mtb.PasswordChar; mtb.UseSystemPasswordChar = true; return pwdChar; } else { return mtb.PasswordChar; } } set { MaskedTextBox mtb = this.Control as MaskedTextBox; Debug.Assert(mtb != null, "Designed control is not a MaskedTextBox."); mtb.PasswordChar = value; } } ////// Since we're shadowing Text property, we get called here to determine whether or not to serialize it. /// //private bool ShouldSerializeText() //{ // return !string.Empty.Equals(this.Control.Text); //} /// /// Shadow the Text property to do two things: /// 1. Always show the text without prompt or literals. /// 2. The text from the UITypeEditor is assigned escaping literals, prompt and spaces, this is to allow for partial inputs. /// Observe that if the MTB is hooked to a PropertyBrowser at design time, shadowing of the property won't work unless the /// application is a well written control designer (implements corresponding interfaces). /// private string Text { get { // Return text w/o literals or prompt. MaskedTextBox mtb = this.Control as MaskedTextBox; Debug.Assert( mtb != null, "Designed control is not a MaskedTextBox." ); // Text w/o prompt or literals. if( string.IsNullOrEmpty(mtb.Mask) ) { return mtb.Text; } return mtb.MaskedTextProvider.ToString( false, false ); } set { MaskedTextBox mtb = this.Control as MaskedTextBox; Debug.Assert( mtb != null, "Designed control is not a MaskedTextBox." ); if( string.IsNullOrEmpty(mtb.Mask) ) { mtb.Text = value; } else { bool ResetOnSpace = mtb.ResetOnSpace; bool ResetOnPrompt = mtb.ResetOnPrompt; bool SkipLiterals = mtb.SkipLiterals; mtb.ResetOnSpace = true; mtb.ResetOnPrompt = true; mtb.SkipLiterals = true; // Value is expected to contain literals and prompt. mtb.Text = value; mtb.ResetOnSpace = ResetOnSpace; mtb.ResetOnPrompt = ResetOnPrompt; mtb.SkipLiterals = SkipLiterals; } } } ////// MaskedTextBox designer verb collection property. Gets the design-time supported verbs of the control. /// public override DesignerVerbCollection Verbs { get { if( this.verbs == null ) { this.verbs = new DesignerVerbCollection(); this.verbs.Add(new DesignerVerb( SR.GetString(SR.MaskedTextBoxDesignerVerbsSetMaskDesc), new EventHandler(OnVerbSetMask) )); } return this.verbs; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
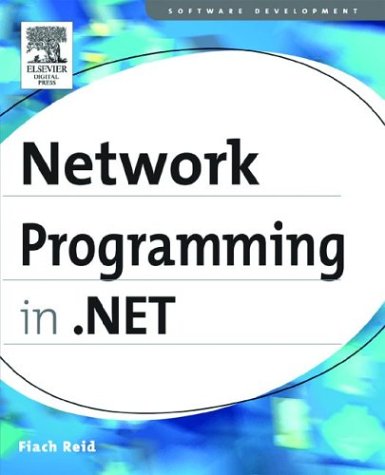
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PathHelper.cs
- MessageQueueEnumerator.cs
- DataError.cs
- EventWaitHandle.cs
- FormsAuthenticationModule.cs
- UInt16Converter.cs
- PointKeyFrameCollection.cs
- ClassData.cs
- ScriptControlManager.cs
- AutomationElementCollection.cs
- ServiceBusyException.cs
- RepeatBehaviorConverter.cs
- KeysConverter.cs
- SizeF.cs
- DataControlFieldCollection.cs
- DataSourceGeneratorException.cs
- ProtocolInformationWriter.cs
- CompilerScopeManager.cs
- NativeMsmqMessage.cs
- MouseGesture.cs
- SharedDp.cs
- TransactionalPackage.cs
- ToolStripPanel.cs
- LinqDataSource.cs
- ProcessInfo.cs
- WbmpConverter.cs
- __FastResourceComparer.cs
- HtmlPhoneCallAdapter.cs
- EnumValidator.cs
- HashHelper.cs
- EntityEntry.cs
- Permission.cs
- ClientSponsor.cs
- PasswordDeriveBytes.cs
- DataTableClearEvent.cs
- smtppermission.cs
- SettingsPropertyNotFoundException.cs
- webbrowsersite.cs
- CfgArc.cs
- MonthChangedEventArgs.cs
- DataGridViewComboBoxCell.cs
- List.cs
- BinaryObjectReader.cs
- DecoratedNameAttribute.cs
- MethodBuilder.cs
- CheckBoxRenderer.cs
- COM2TypeInfoProcessor.cs
- TypeBrowser.xaml.cs
- NextPreviousPagerField.cs
- DataGridParentRows.cs
- ClockGroup.cs
- GridViewColumnHeaderAutomationPeer.cs
- LabelEditEvent.cs
- IIS7ConfigurationLoader.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- SessionEndingCancelEventArgs.cs
- NullableBoolConverter.cs
- RemotingConfiguration.cs
- StrokeCollectionDefaultValueFactory.cs
- GlyphInfoList.cs
- WinInetCache.cs
- EnvironmentPermission.cs
- xmlglyphRunInfo.cs
- ListBoxItemWrapperAutomationPeer.cs
- MappingSource.cs
- CommunicationException.cs
- WinHttpWebProxyFinder.cs
- WeakRefEnumerator.cs
- SafeCancelMibChangeNotify.cs
- BufferModesCollection.cs
- QuaternionRotation3D.cs
- XamlToRtfWriter.cs
- PropertyMapper.cs
- KeyValuePair.cs
- WebPartDisplayModeCollection.cs
- TemplatePagerField.cs
- MobileControl.cs
- HttpListenerPrefixCollection.cs
- SystemInfo.cs
- NumberAction.cs
- SelectingProviderEventArgs.cs
- QuaternionAnimationUsingKeyFrames.cs
- CodeDOMUtility.cs
- XmlSchemaExternal.cs
- StaticDataManager.cs
- VideoDrawing.cs
- CommonObjectSecurity.cs
- SecurityProtocol.cs
- RuleInfoComparer.cs
- RijndaelCryptoServiceProvider.cs
- RootProfilePropertySettingsCollection.cs
- XmlQueryRuntime.cs
- NativeObjectSecurity.cs
- StringSorter.cs
- RegexWorker.cs
- BackEase.cs
- WorkflowMarkupSerializerMapping.cs
- DataGridViewCellMouseEventArgs.cs
- DateTimeFormat.cs
- TargetControlTypeCache.cs