Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / InputScopeNameConverter.cs / 1 / InputScopeNameConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: class for input scope type-converter // // Please refer to the design specfication http://avalon/Cicero/Specifications/Stylable%20InputScope.mht // // History: // 1/20/2005 : yutakan - created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows.Input; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; namespace System.Windows.Input { ////// type-converter which performs type conversions for inputscope /// ///http://avalon/Cicero/Specifications/Stylable%20InputScope.mht public class InputScopeNameConverter : TypeConverter { ////// Returns whether this converter can convert an object of one type to InputScope type /// InputScopeConverter only supports string type to convert from /// /// /// The conversion context. /// /// /// The type to convert from. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } return false; } ////// Returns whether this converter can convert the object to the specified type. /// InputScopeConverter only supports string type to convert to /// /// /// The conversion context. /// /// /// The type to convert to. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (typeof (string) == destinationType && null != context && null != context.Instance && context.Instance is InputScopeName) { return true; } return false; } ////// Converts the given value to InputScope type /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The source object to convert from. /// ////// InputScope object with a specified scope name, otherwise InputScope with Default scope. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { string stringSource = source as string; InputScopeNameValue nameValue = InputScopeNameValue.Default; InputScopeName inputScopeName; if (null != stringSource) { stringSource = stringSource.Trim(); if (-1 != stringSource.LastIndexOf('.')) stringSource = stringSource.Substring(stringSource.LastIndexOf('.')+1); if (!stringSource.Equals(String.Empty)) { nameValue = (InputScopeNameValue)Enum.Parse(typeof(InputScopeNameValue), stringSource); } } inputScopeName = new InputScopeName(); inputScopeName.NameValue = nameValue; return inputScopeName; } ////// Converts the given value as InputScope object to the specified type. /// This converter only supports string type as a type to convert to. /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The value to convert. /// /// /// The type to convert to. /// ////// A new object of the specified type (string) converted from the given InputScope object. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { InputScopeName inputScopeName = value as InputScopeName; if (null != destinationType && null != inputScopeName) { if (destinationType == typeof(string)) { return Enum.GetName(typeof(InputScopeNameValue), inputScopeName.NameValue); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: class for input scope type-converter // // Please refer to the design specfication http://avalon/Cicero/Specifications/Stylable%20InputScope.mht // // History: // 1/20/2005 : yutakan - created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows.Input; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; namespace System.Windows.Input { ////// type-converter which performs type conversions for inputscope /// ///http://avalon/Cicero/Specifications/Stylable%20InputScope.mht public class InputScopeNameConverter : TypeConverter { ////// Returns whether this converter can convert an object of one type to InputScope type /// InputScopeConverter only supports string type to convert from /// /// /// The conversion context. /// /// /// The type to convert from. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } return false; } ////// Returns whether this converter can convert the object to the specified type. /// InputScopeConverter only supports string type to convert to /// /// /// The conversion context. /// /// /// The type to convert to. /// ////// True if conversion is possible, false otherwise. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (typeof (string) == destinationType && null != context && null != context.Instance && context.Instance is InputScopeName) { return true; } return false; } ////// Converts the given value to InputScope type /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The source object to convert from. /// ////// InputScope object with a specified scope name, otherwise InputScope with Default scope. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { string stringSource = source as string; InputScopeNameValue nameValue = InputScopeNameValue.Default; InputScopeName inputScopeName; if (null != stringSource) { stringSource = stringSource.Trim(); if (-1 != stringSource.LastIndexOf('.')) stringSource = stringSource.Substring(stringSource.LastIndexOf('.')+1); if (!stringSource.Equals(String.Empty)) { nameValue = (InputScopeNameValue)Enum.Parse(typeof(InputScopeNameValue), stringSource); } } inputScopeName = new InputScopeName(); inputScopeName.NameValue = nameValue; return inputScopeName; } ////// Converts the given value as InputScope object to the specified type. /// This converter only supports string type as a type to convert to. /// /// /// The conversion context. /// /// /// The current culture that applies to the conversion. /// /// /// The value to convert. /// /// /// The type to convert to. /// ////// A new object of the specified type (string) converted from the given InputScope object. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { InputScopeName inputScopeName = value as InputScopeName; if (null != destinationType && null != inputScopeName) { if (destinationType == typeof(string)) { return Enum.GetName(typeof(InputScopeNameValue), inputScopeName.NameValue); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
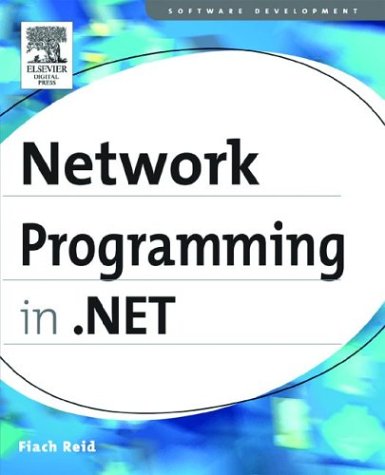
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CharacterBuffer.cs
- DrawingVisual.cs
- UriTemplateTableMatchCandidate.cs
- UTF32Encoding.cs
- DelegateInArgument.cs
- FontFamilyConverter.cs
- CorruptStoreException.cs
- StylusCaptureWithinProperty.cs
- SqlConnectionFactory.cs
- OracleColumn.cs
- ImageFormatConverter.cs
- TransformedBitmap.cs
- CompiledQuery.cs
- CompiledIdentityConstraint.cs
- ExpressionVisitor.cs
- PageClientProxyGenerator.cs
- MenuItem.cs
- XmlUtilWriter.cs
- ClientFormsAuthenticationCredentials.cs
- Vector3D.cs
- FormClosedEvent.cs
- FormViewPageEventArgs.cs
- DPTypeDescriptorContext.cs
- XmlEntity.cs
- BaseServiceProvider.cs
- SystemThemeKey.cs
- DesignerVerb.cs
- RemoteCryptoSignHashRequest.cs
- StrongNamePublicKeyBlob.cs
- SessionEndedEventArgs.cs
- CheckedPointers.cs
- X509CertificateCollection.cs
- DirectionalLight.cs
- HttpRuntime.cs
- XmlRawWriter.cs
- BindingMAnagerBase.cs
- ToolboxComponentsCreatingEventArgs.cs
- ItemMap.cs
- KnowledgeBase.cs
- Instrumentation.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- DispatcherFrame.cs
- RangeBase.cs
- TemplateParser.cs
- DataKeyArray.cs
- ExpandoClass.cs
- TaskCanceledException.cs
- Triangle.cs
- _ContextAwareResult.cs
- TimelineGroup.cs
- CancellationHandler.cs
- IPAddressCollection.cs
- ProxyManager.cs
- ProtocolReflector.cs
- ToolStripItem.cs
- MsdtcWrapper.cs
- TextOnlyOutput.cs
- PointConverter.cs
- StorageTypeMapping.cs
- DbMetaDataColumnNames.cs
- DataColumnCollection.cs
- WebPartCatalogCloseVerb.cs
- RijndaelManaged.cs
- sitestring.cs
- ToolStripDropDownItem.cs
- EntityObject.cs
- HttpContextServiceHost.cs
- DataRowView.cs
- UnwrappedTypesXmlSerializerManager.cs
- PointConverter.cs
- MembershipPasswordException.cs
- AttributeCollection.cs
- WindowsEditBox.cs
- ConfigurationStrings.cs
- BitmapCache.cs
- GeometryGroup.cs
- DrawingGroupDrawingContext.cs
- ComponentManagerBroker.cs
- SoapSchemaImporter.cs
- NameTable.cs
- MiniParameterInfo.cs
- PropertyEmitter.cs
- DbProviderSpecificTypePropertyAttribute.cs
- ListItemCollection.cs
- MultiByteCodec.cs
- ServiceRouteHandler.cs
- AspProxy.cs
- TabControlDesigner.cs
- GeneralTransform3DGroup.cs
- ScrollPattern.cs
- Stylesheet.cs
- SystemInfo.cs
- PropertyGridCommands.cs
- FontFaceLayoutInfo.cs
- KeySplineConverter.cs
- HtmlHistory.cs
- UnsafeNativeMethods.cs
- XmlSchemaAnnotation.cs
- ScrollProperties.cs
- KeyGestureConverter.cs