Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / nulltextnavigator.cs / 1 / nulltextnavigator.cs
//---------------------------------------------------------------------------- // // File: NullTextPointer.cs // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // Description: // TextNavigator implementation for NullTextContainer // This is primarily used by internal code. // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // To enable presharp warning disables (#pragma suppress) below. namespace System.Windows.Documents { using System; using System.Diagnostics; using System.Windows; using MS.Internal; ////// NullTextPointer is an implementation of ITextPointer for NullTextContainer /// internal sealed class NullTextPointer : ITextPointer { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Ctor always set mutable flag to false internal NullTextPointer(NullTextContainer container, LogicalDirection gravity) { _container = container; _gravity = gravity; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region ITextPointer Methods ////// int ITextPointer.CompareTo(ITextPointer position) { Debug.Assert(position is NullTextPointer || position is NullTextPointer); // There is single position in the container. return 0; } int ITextPointer.CompareTo(StaticTextPointer position) { // There is single position in the container. return 0; } ////// /// int ITextPointer.GetOffsetToPosition(ITextPointer position) { Debug.Assert(position is NullTextPointer || position is NullTextPointer); // There is single position in the container. return 0; } ////// /// TextPointerContext ITextPointer.GetPointerContext(LogicalDirection direction) { // There is no content for this container return TextPointerContext.None; } ////// /// ////// Return 0 if non-text run int ITextPointer.GetTextRunLength(LogicalDirection direction) { // There is no content in this container return 0; } //string ITextPointer.GetTextInRun(LogicalDirection direction) { return TextPointerBase.GetTextInRun(this, direction); } /// /// ////// Only reutrn uninterrupted runs of text int ITextPointer.GetTextInRun(LogicalDirection direction, char[] textBuffer, int startIndex, int count) { // There is no content in this container. return 0; } ////// ////// Return null if the embedded object does not exist object ITextPointer.GetAdjacentElement(LogicalDirection direction) { // There is no content in this container. return null; } ////// ////// Return null if no TextElement in the direction Type ITextPointer.GetElementType(LogicalDirection direction) { // There is no content in this container. return null; } ////// bool ITextPointer.HasEqualScope(ITextPointer position) { return true; } ////// /// ////// return property values even if there is no scoping element object ITextPointer.GetValue(DependencyProperty property) { return property.DefaultMetadata.DefaultValue; } ////// object ITextPointer.ReadLocalValue(DependencyProperty property) { return DependencyProperty.UnsetValue; } ////// /// ////// Returns an empty enumerator if there is no scoping element LocalValueEnumerator ITextPointer.GetLocalValueEnumerator() { return (new DependencyObject()).GetLocalValueEnumerator(); } ITextPointer ITextPointer.CreatePointer() { return ((ITextPointer)this).CreatePointer(0, _gravity); } // Unoptimized CreateStaticPointer implementation. // Creates a simple wrapper for an ITextPointer instance. StaticTextPointer ITextPointer.CreateStaticPointer() { return new StaticTextPointer(((ITextPointer)this).TextContainer, ((ITextPointer)this).CreatePointer()); } ITextPointer ITextPointer.CreatePointer(int distance) { return ((ITextPointer)this).CreatePointer(distance, _gravity); } ITextPointer ITextPointer.CreatePointer(LogicalDirection gravity) { return ((ITextPointer)this).CreatePointer(0, gravity); } ////// ITextPointer ITextPointer.CreatePointer(int distance, LogicalDirection gravity) { // There is no content in this container Debug.Assert(distance == 0); return new NullTextPointer(_container, gravity); } ///// void ITextPointer.Freeze() { _isFrozen = true; } /// /// ITextPointer ITextPointer.GetFrozenPointer(LogicalDirection logicalDirection) { return TextPointerBase.GetFrozenPointer(this, logicalDirection); } #endregion ITextPointer Methods #region ITextPointer Methods ////// /// /// void ITextPointer.SetLogicalDirection(LogicalDirection direction) { ValidationHelper.VerifyDirection(direction, "gravity"); Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); _gravity = direction; } ////// /// bool ITextPointer.MoveToNextContextPosition(LogicalDirection direction) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); // Nowhere to move in an empty container return false; } ////// /// int ITextPointer.MoveByOffset(int distance) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); Debug.Assert(distance == 0, "Single possible position in this empty container"); return 0; } ////// /// void ITextPointer.MoveToPosition(ITextPointer position) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); // There is single possible position in this empty container. } ////// /// void ITextPointer.MoveToElementEdge(ElementEdge edge) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); Debug.Assert(false, "No scoping element!"); } ///// int ITextPointer.MoveToLineBoundary(int count) { Debug.Assert(false, "NullTextPointer does not expect layout dependent method calls!"); return 0; } // Rect ITextPointer.GetCharacterRect(LogicalDirection direction) { Debug.Assert(false, "NullTextPointer does not expect layout dependent method calls!"); return new Rect(); } bool ITextPointer.MoveToInsertionPosition(LogicalDirection direction) { return TextPointerBase.MoveToInsertionPosition(this, direction); } bool ITextPointer.MoveToNextInsertionPosition(LogicalDirection direction) { return TextPointerBase.MoveToNextInsertionPosition(this, direction); } void ITextPointer.InsertTextInRun(string textData) { Debug.Assert(false); // must never call this } void ITextPointer.DeleteContentToPosition(ITextPointer limit) { Debug.Assert(false); // must never call this } // Candidate for replacing MoveToNextContextPosition for immutable TextPointer model ITextPointer ITextPointer.GetNextContextPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); if (pointer.MoveToNextContextPosition(direction)) { pointer.Freeze(); } else { pointer = null; } return pointer; } // Candidate for replacing MoveToInsertionPosition for immutable TextPointer model ITextPointer ITextPointer.GetInsertionPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); pointer.MoveToInsertionPosition(direction); pointer.Freeze(); return pointer; } // Returns the closest insertion position, treating all unicode code points // as valid insertion positions. A useful performance win over // GetNextInsertionPosition when only formatting scopes are important. ITextPointer ITextPointer.GetFormatNormalizedPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); TextPointerBase.MoveToFormatNormalizedPosition(pointer, direction); pointer.Freeze(); return pointer; } // Candidate for replacing MoveToNextInsertionPosition for immutable TextPointer model ITextPointer ITextPointer.GetNextInsertionPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); if (pointer.MoveToNextInsertionPosition(direction)) { pointer.Freeze(); } else { pointer = null; } return pointer; } /// bool ITextPointer.ValidateLayout() { return false; } #endregion ITextPointer Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region ITextPointer Properties // Type ITextPointer.ParentType { get { // There is no content in this container. // We want to behave consistently with FixedTextPointer so we know // we're at the root when the parent is a FixedDocument return typeof(FixedDocument); } } /// /// ITextContainer ITextPointer.TextContainer { get { return _container; } } ///// bool ITextPointer.HasValidLayout { get { // NullTextContainer's never have a layout. return false; } } // bool ITextPointer.IsAtCaretUnitBoundary { get { Invariant.Assert(false, "NullTextPointer never has valid layout!"); return false; } } /// /// LogicalDirection ITextPointer.LogicalDirection { get { return _gravity; } } bool ITextPointer.IsAtInsertionPosition { get { return TextPointerBase.IsAtInsertionPosition(this); } } ///// bool ITextPointer.IsFrozen { get { return _isFrozen; } } // int ITextPointer.Offset { get { return 0; } } // Not implemented. int ITextPointer.CharOffset { get { #pragma warning suppress 56503 throw new NotImplementedException(); } } #endregion ITextPointer Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private LogicalDirection _gravity; private NullTextContainer _container; // True if Freeze has been called, in which case // this TextPointer is immutable and may not be repositioned. private bool _isFrozen; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: NullTextPointer.cs // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // Description: // TextNavigator implementation for NullTextContainer // This is primarily used by internal code. // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // To enable presharp warning disables (#pragma suppress) below. namespace System.Windows.Documents { using System; using System.Diagnostics; using System.Windows; using MS.Internal; /// /// NullTextPointer is an implementation of ITextPointer for NullTextContainer /// internal sealed class NullTextPointer : ITextPointer { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Ctor always set mutable flag to false internal NullTextPointer(NullTextContainer container, LogicalDirection gravity) { _container = container; _gravity = gravity; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region ITextPointer Methods ////// int ITextPointer.CompareTo(ITextPointer position) { Debug.Assert(position is NullTextPointer || position is NullTextPointer); // There is single position in the container. return 0; } int ITextPointer.CompareTo(StaticTextPointer position) { // There is single position in the container. return 0; } ////// /// int ITextPointer.GetOffsetToPosition(ITextPointer position) { Debug.Assert(position is NullTextPointer || position is NullTextPointer); // There is single position in the container. return 0; } ////// /// TextPointerContext ITextPointer.GetPointerContext(LogicalDirection direction) { // There is no content for this container return TextPointerContext.None; } ////// /// ////// Return 0 if non-text run int ITextPointer.GetTextRunLength(LogicalDirection direction) { // There is no content in this container return 0; } //string ITextPointer.GetTextInRun(LogicalDirection direction) { return TextPointerBase.GetTextInRun(this, direction); } /// /// ////// Only reutrn uninterrupted runs of text int ITextPointer.GetTextInRun(LogicalDirection direction, char[] textBuffer, int startIndex, int count) { // There is no content in this container. return 0; } ////// ////// Return null if the embedded object does not exist object ITextPointer.GetAdjacentElement(LogicalDirection direction) { // There is no content in this container. return null; } ////// ////// Return null if no TextElement in the direction Type ITextPointer.GetElementType(LogicalDirection direction) { // There is no content in this container. return null; } ////// bool ITextPointer.HasEqualScope(ITextPointer position) { return true; } ////// /// ////// return property values even if there is no scoping element object ITextPointer.GetValue(DependencyProperty property) { return property.DefaultMetadata.DefaultValue; } ////// object ITextPointer.ReadLocalValue(DependencyProperty property) { return DependencyProperty.UnsetValue; } ////// /// ////// Returns an empty enumerator if there is no scoping element LocalValueEnumerator ITextPointer.GetLocalValueEnumerator() { return (new DependencyObject()).GetLocalValueEnumerator(); } ITextPointer ITextPointer.CreatePointer() { return ((ITextPointer)this).CreatePointer(0, _gravity); } // Unoptimized CreateStaticPointer implementation. // Creates a simple wrapper for an ITextPointer instance. StaticTextPointer ITextPointer.CreateStaticPointer() { return new StaticTextPointer(((ITextPointer)this).TextContainer, ((ITextPointer)this).CreatePointer()); } ITextPointer ITextPointer.CreatePointer(int distance) { return ((ITextPointer)this).CreatePointer(distance, _gravity); } ITextPointer ITextPointer.CreatePointer(LogicalDirection gravity) { return ((ITextPointer)this).CreatePointer(0, gravity); } ////// ITextPointer ITextPointer.CreatePointer(int distance, LogicalDirection gravity) { // There is no content in this container Debug.Assert(distance == 0); return new NullTextPointer(_container, gravity); } ///// void ITextPointer.Freeze() { _isFrozen = true; } /// /// ITextPointer ITextPointer.GetFrozenPointer(LogicalDirection logicalDirection) { return TextPointerBase.GetFrozenPointer(this, logicalDirection); } #endregion ITextPointer Methods #region ITextPointer Methods ////// /// /// void ITextPointer.SetLogicalDirection(LogicalDirection direction) { ValidationHelper.VerifyDirection(direction, "gravity"); Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); _gravity = direction; } ////// /// bool ITextPointer.MoveToNextContextPosition(LogicalDirection direction) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); // Nowhere to move in an empty container return false; } ////// /// int ITextPointer.MoveByOffset(int distance) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); Debug.Assert(distance == 0, "Single possible position in this empty container"); return 0; } ////// /// void ITextPointer.MoveToPosition(ITextPointer position) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); // There is single possible position in this empty container. } ////// /// void ITextPointer.MoveToElementEdge(ElementEdge edge) { Debug.Assert(!_isFrozen, "Can't reposition a frozen pointer!"); Debug.Assert(false, "No scoping element!"); } ///// int ITextPointer.MoveToLineBoundary(int count) { Debug.Assert(false, "NullTextPointer does not expect layout dependent method calls!"); return 0; } // Rect ITextPointer.GetCharacterRect(LogicalDirection direction) { Debug.Assert(false, "NullTextPointer does not expect layout dependent method calls!"); return new Rect(); } bool ITextPointer.MoveToInsertionPosition(LogicalDirection direction) { return TextPointerBase.MoveToInsertionPosition(this, direction); } bool ITextPointer.MoveToNextInsertionPosition(LogicalDirection direction) { return TextPointerBase.MoveToNextInsertionPosition(this, direction); } void ITextPointer.InsertTextInRun(string textData) { Debug.Assert(false); // must never call this } void ITextPointer.DeleteContentToPosition(ITextPointer limit) { Debug.Assert(false); // must never call this } // Candidate for replacing MoveToNextContextPosition for immutable TextPointer model ITextPointer ITextPointer.GetNextContextPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); if (pointer.MoveToNextContextPosition(direction)) { pointer.Freeze(); } else { pointer = null; } return pointer; } // Candidate for replacing MoveToInsertionPosition for immutable TextPointer model ITextPointer ITextPointer.GetInsertionPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); pointer.MoveToInsertionPosition(direction); pointer.Freeze(); return pointer; } // Returns the closest insertion position, treating all unicode code points // as valid insertion positions. A useful performance win over // GetNextInsertionPosition when only formatting scopes are important. ITextPointer ITextPointer.GetFormatNormalizedPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); TextPointerBase.MoveToFormatNormalizedPosition(pointer, direction); pointer.Freeze(); return pointer; } // Candidate for replacing MoveToNextInsertionPosition for immutable TextPointer model ITextPointer ITextPointer.GetNextInsertionPosition(LogicalDirection direction) { ITextPointer pointer = ((ITextPointer)this).CreatePointer(); if (pointer.MoveToNextInsertionPosition(direction)) { pointer.Freeze(); } else { pointer = null; } return pointer; } /// bool ITextPointer.ValidateLayout() { return false; } #endregion ITextPointer Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region ITextPointer Properties // Type ITextPointer.ParentType { get { // There is no content in this container. // We want to behave consistently with FixedTextPointer so we know // we're at the root when the parent is a FixedDocument return typeof(FixedDocument); } } /// /// ITextContainer ITextPointer.TextContainer { get { return _container; } } ///// bool ITextPointer.HasValidLayout { get { // NullTextContainer's never have a layout. return false; } } // bool ITextPointer.IsAtCaretUnitBoundary { get { Invariant.Assert(false, "NullTextPointer never has valid layout!"); return false; } } /// /// LogicalDirection ITextPointer.LogicalDirection { get { return _gravity; } } bool ITextPointer.IsAtInsertionPosition { get { return TextPointerBase.IsAtInsertionPosition(this); } } ///// bool ITextPointer.IsFrozen { get { return _isFrozen; } } // int ITextPointer.Offset { get { return 0; } } // Not implemented. int ITextPointer.CharOffset { get { #pragma warning suppress 56503 throw new NotImplementedException(); } } #endregion ITextPointer Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private LogicalDirection _gravity; private NullTextContainer _container; // True if Freeze has been called, in which case // this TextPointer is immutable and may not be repositioned. private bool _isFrozen; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
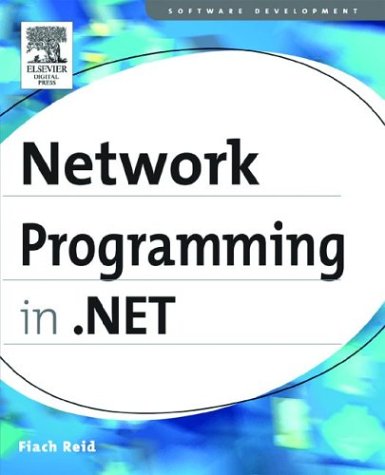
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ItemChangedEventArgs.cs
- SecurityHelper.cs
- FlowDocumentView.cs
- TemplatePartAttribute.cs
- StylusButtonCollection.cs
- Baml2006ReaderSettings.cs
- DataGridItem.cs
- RemoteWebConfigurationHostStream.cs
- SpellerError.cs
- OptimisticConcurrencyException.cs
- DataBoundControlHelper.cs
- AnonymousIdentificationModule.cs
- MSG.cs
- SmtpAuthenticationManager.cs
- AnnotationStore.cs
- BinHexEncoder.cs
- ToolboxItemAttribute.cs
- XmlBinaryWriterSession.cs
- webbrowsersite.cs
- FontSource.cs
- TreeViewItem.cs
- BrowserCapabilitiesFactoryBase.cs
- PasswordPropertyTextAttribute.cs
- IChannel.cs
- SocketManager.cs
- BmpBitmapEncoder.cs
- TypeUsage.cs
- PresentationSource.cs
- SiteMap.cs
- CornerRadiusConverter.cs
- XmlSchemaRedefine.cs
- LinqDataSourceSelectEventArgs.cs
- SystemIcmpV6Statistics.cs
- PaperSize.cs
- TextContainerHelper.cs
- BamlWriter.cs
- VariableElement.cs
- PageClientProxyGenerator.cs
- DataSourceXmlSerializationAttribute.cs
- _NtlmClient.cs
- StdValidatorsAndConverters.cs
- ISAPIWorkerRequest.cs
- TableDetailsRow.cs
- Utils.cs
- MenuItemCollectionEditor.cs
- DrawingBrush.cs
- X509IssuerSerialKeyIdentifierClause.cs
- Italic.cs
- DiffuseMaterial.cs
- TimeSpanSecondsConverter.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- BitmapImage.cs
- ChameleonKey.cs
- GeometryModel3D.cs
- KeyedCollection.cs
- Utils.cs
- SqlAggregateChecker.cs
- XmlCharCheckingReader.cs
- ReferenceConverter.cs
- RowParagraph.cs
- AtomParser.cs
- DbDataSourceEnumerator.cs
- ContentType.cs
- Claim.cs
- Timeline.cs
- TextParagraph.cs
- ScriptingWebServicesSectionGroup.cs
- SimpleTypeResolver.cs
- ImageAttributes.cs
- OutputCacheSettings.cs
- IdentityNotMappedException.cs
- DCSafeHandle.cs
- SpoolingTaskBase.cs
- UserControlParser.cs
- ResourceContainer.cs
- GridLength.cs
- XmlTextWriter.cs
- _ContextAwareResult.cs
- DataSourceXmlClassAttribute.cs
- PrivilegedConfigurationManager.cs
- XmlILConstructAnalyzer.cs
- DesignBindingConverter.cs
- figurelengthconverter.cs
- UTF8Encoding.cs
- SimpleApplicationHost.cs
- PaintEvent.cs
- MsmqHostedTransportConfiguration.cs
- TraceSection.cs
- ListViewCancelEventArgs.cs
- TreeNodeClickEventArgs.cs
- FormViewDeletedEventArgs.cs
- KeyNotFoundException.cs
- TextChangedEventArgs.cs
- TextElementEnumerator.cs
- Baml2006KnownTypes.cs
- ImplicitInputBrush.cs
- XmlC14NWriter.cs
- RelationshipFixer.cs
- WebPartDisplayMode.cs
- X509Certificate2Collection.cs