Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / TextChangedEventArgs.cs / 1305600 / TextChangedEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextChanged event argument. // // History: // 09/27/2004 : psarrett - Created // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; namespace System.Windows.Controls { #region TextChangedEvent ////// How the undo stack caused or is affected by a text change. /// public enum UndoAction { ////// This change will not affect the undo stack at all /// None = 0, ////// This change will merge into the previous undo unit /// Merge = 1, ////// This change is the result of a call to Undo() /// Undo = 2, ////// This change is the result of a call to Redo() /// Redo = 3, ////// This change will clear the undo stack /// Clear = 4, ////// This change will create a new undo unit /// Create = 5 } ////// The delegate to use for handlers that receive TextChangedEventArgs /// public delegate void TextChangedEventHandler(object sender, TextChangedEventArgs e); ////// The TextChangedEventArgs class represents a type of RoutedEventArgs that /// are relevant to events raised by changing editable text. /// public class TextChangedEventArgs : RoutedEventArgs { ////// constructor /// /// event id /// UndoAction /// ReadOnlyCollection public TextChangedEventArgs(RoutedEvent id, UndoAction action, ICollectionchanges) : base() { if (id == null) { throw new ArgumentNullException("id"); } if (action < UndoAction.None || action > UndoAction.Create) { throw new InvalidEnumArgumentException("action", (int)action, typeof(UndoAction)); } RoutedEvent=id; _undoAction = action; _changes = changes; } /// /// constructor /// /// event id /// UndoAction public TextChangedEventArgs(RoutedEvent id, UndoAction action) : this(id, action, new ReadOnlyCollection(new List ())) { } /// /// How the undo stack caused or is affected by this text change /// ///UndoAction enum public UndoAction UndoAction { get { return _undoAction; } } ////// A readonly list of TextChanges, sorted by offset in order from the beginning to the end of /// the document, describing the dirty areas of the document and in what way(s) those /// areas differ from the pre-change document. For example, if content at offset 0 was: /// /// The quick brown fox jumps over the lazy dog. /// /// and changed to: /// /// The brown fox jumps over a dog. /// /// Changes would contain two TextChanges with the following information: /// /// Change 1 /// -------- /// Offset: 5 /// RemovedCount: 6 /// AddedCount: 0 /// PropertyCount: 0 /// /// Change 2 /// -------- /// Offset: 26 /// RemovedCount: 8 /// AddedCount: 1 /// PropertyCount: 0 /// /// If no changes were made, the collection will be empty. /// public ICollectionChanges { get { return _changes; } } /// /// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { TextChangedEventHandler handler; handler = (TextChangedEventHandler)genericHandler; handler(genericTarget, this); } private UndoAction _undoAction; private readonly ICollection_changes; } #endregion TextChangedEvent } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextChanged event argument. // // History: // 09/27/2004 : psarrett - Created // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; namespace System.Windows.Controls { #region TextChangedEvent ////// How the undo stack caused or is affected by a text change. /// public enum UndoAction { ////// This change will not affect the undo stack at all /// None = 0, ////// This change will merge into the previous undo unit /// Merge = 1, ////// This change is the result of a call to Undo() /// Undo = 2, ////// This change is the result of a call to Redo() /// Redo = 3, ////// This change will clear the undo stack /// Clear = 4, ////// This change will create a new undo unit /// Create = 5 } ////// The delegate to use for handlers that receive TextChangedEventArgs /// public delegate void TextChangedEventHandler(object sender, TextChangedEventArgs e); ////// The TextChangedEventArgs class represents a type of RoutedEventArgs that /// are relevant to events raised by changing editable text. /// public class TextChangedEventArgs : RoutedEventArgs { ////// constructor /// /// event id /// UndoAction /// ReadOnlyCollection public TextChangedEventArgs(RoutedEvent id, UndoAction action, ICollectionchanges) : base() { if (id == null) { throw new ArgumentNullException("id"); } if (action < UndoAction.None || action > UndoAction.Create) { throw new InvalidEnumArgumentException("action", (int)action, typeof(UndoAction)); } RoutedEvent=id; _undoAction = action; _changes = changes; } /// /// constructor /// /// event id /// UndoAction public TextChangedEventArgs(RoutedEvent id, UndoAction action) : this(id, action, new ReadOnlyCollection(new List ())) { } /// /// How the undo stack caused or is affected by this text change /// ///UndoAction enum public UndoAction UndoAction { get { return _undoAction; } } ////// A readonly list of TextChanges, sorted by offset in order from the beginning to the end of /// the document, describing the dirty areas of the document and in what way(s) those /// areas differ from the pre-change document. For example, if content at offset 0 was: /// /// The quick brown fox jumps over the lazy dog. /// /// and changed to: /// /// The brown fox jumps over a dog. /// /// Changes would contain two TextChanges with the following information: /// /// Change 1 /// -------- /// Offset: 5 /// RemovedCount: 6 /// AddedCount: 0 /// PropertyCount: 0 /// /// Change 2 /// -------- /// Offset: 26 /// RemovedCount: 8 /// AddedCount: 1 /// PropertyCount: 0 /// /// If no changes were made, the collection will be empty. /// public ICollectionChanges { get { return _changes; } } /// /// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { TextChangedEventHandler handler; handler = (TextChangedEventHandler)genericHandler; handler(genericTarget, this); } private UndoAction _undoAction; private readonly ICollection_changes; } #endregion TextChangedEvent } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
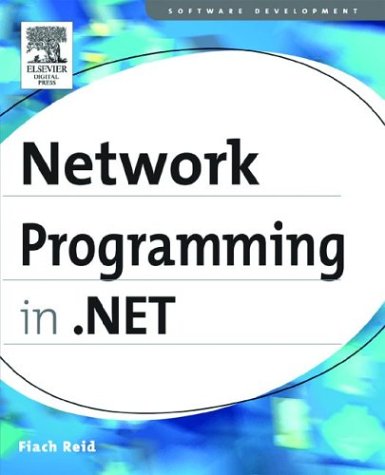
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Converter.cs
- TypeDescriptorContext.cs
- MsmqOutputMessage.cs
- OdbcDataAdapter.cs
- BitmapEffectDrawingContextState.cs
- MonthCalendar.cs
- EntityStoreSchemaGenerator.cs
- OracleColumn.cs
- ConstraintConverter.cs
- VarRefManager.cs
- NullToBooleanConverter.cs
- TemplateColumn.cs
- XamlBrushSerializer.cs
- InstanceDataCollection.cs
- Int64KeyFrameCollection.cs
- CompatibleIComparer.cs
- AccessDataSourceWizardForm.cs
- EncodingInfo.cs
- ControlPaint.cs
- ServiceParser.cs
- UserControl.cs
- TextSelectionHighlightLayer.cs
- AssemblyCache.cs
- ModuleConfigurationInfo.cs
- WebPartDescriptionCollection.cs
- AssociationType.cs
- _Connection.cs
- TempFiles.cs
- PageCodeDomTreeGenerator.cs
- Line.cs
- Adorner.cs
- ToolBarButtonClickEvent.cs
- ConfigXmlText.cs
- ColumnWidthChangingEvent.cs
- StrictModeSecurityHeaderElementInferenceEngine.cs
- WebZone.cs
- BufferBuilder.cs
- StringDictionaryWithComparer.cs
- Baml2006Reader.cs
- Profiler.cs
- PropertyOverridesDialog.cs
- WebHeaderCollection.cs
- MimeParameterWriter.cs
- SettingsBindableAttribute.cs
- VerticalAlignConverter.cs
- TypeDependencyAttribute.cs
- CssStyleCollection.cs
- Track.cs
- MailWriter.cs
- WindowsStatic.cs
- SymLanguageVendor.cs
- GeneratedView.cs
- IdleTimeoutMonitor.cs
- RepeaterDataBoundAdapter.cs
- OleDbErrorCollection.cs
- SystemEvents.cs
- QueryServiceConfigHandle.cs
- PathData.cs
- SqlFacetAttribute.cs
- MessageSecurityOverTcp.cs
- ServicePoint.cs
- ChannelServices.cs
- WebBrowserUriTypeConverter.cs
- DataGridViewToolTip.cs
- CngProperty.cs
- DataBindingCollectionEditor.cs
- RSACryptoServiceProvider.cs
- NameValueConfigurationElement.cs
- ImageInfo.cs
- XmlUtf8RawTextWriter.cs
- LassoHelper.cs
- RightsManagementPermission.cs
- AudioFormatConverter.cs
- WebPartVerbCollection.cs
- HttpMethodAttribute.cs
- sqlmetadatafactory.cs
- ConfigXmlCDataSection.cs
- TreeViewEvent.cs
- EdmSchemaError.cs
- Query.cs
- ScriptResourceAttribute.cs
- MouseButtonEventArgs.cs
- MobileControlsSection.cs
- XAMLParseException.cs
- SubtreeProcessor.cs
- HttpClientCertificate.cs
- RSAPKCS1SignatureFormatter.cs
- activationcontext.cs
- LocalizabilityAttribute.cs
- LastQueryOperator.cs
- ProfessionalColorTable.cs
- InstancePersistenceCommandException.cs
- IResourceProvider.cs
- StylusPoint.cs
- HttpCacheVaryByContentEncodings.cs
- IndexingContentUnit.cs
- FileDataSourceCache.cs
- CurrentChangingEventManager.cs
- ListSourceHelper.cs
- FrameworkTemplate.cs