Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / ItemType.cs / 1 / ItemType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Xml; using System.Data; using System.Diagnostics; using metadata = System.Data.Metadata.Edm; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for Item. /// [System.Diagnostics.DebuggerDisplay("Name={Name}, BaseType={BaseType.FQName}, HasKeys={HasKeys}")] internal sealed class SchemaEntityType : StructuredType { #region Private Fields private const char KEY_DELIMITER = ' '; private ISchemaElementLookUpTable_navigationProperties = null; private EntityKeyElement _keyElement = null; private static List EmptyKeyProperties = new List (0); #endregion #region Public Methods /// /// /// /// public SchemaEntityType(Schema parentElement) : base(parentElement) { } #endregion #region Protected Methods ////// /// internal override void ResolveTopLevelNames() { base.ResolveTopLevelNames(); if (BaseType != null) { if (!(BaseType is SchemaEntityType)) { AddError(ErrorCode.InvalidBaseType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidBaseTypeForItemType(BaseType.FQName, FQName)); } // Since the base type is not null, key must be defined on the base type else if (_keyElement != null && BaseType != null) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyKeyDefinedInBaseClass(FQName, BaseType.FQName)); } } // If the base type is not null, then the key must be defined on the base entity type, since // we don't allow entity type without keys. else if (_keyElement == null) { AddError(ErrorCode.KeyMissingOnEntityType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.KeyMissingOnEntityType(this.FQName)); } else if (null == BaseType && null != UnresolvedBaseType) { // this is already an error situation, we won't do any resolve name further in this type return; } else { _keyElement.ResolveTopLevelNames(); } } #endregion #region Protected Properties protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } return false; } #endregion #region Private Methods #endregion #region Public Properties public EntityKeyElement KeyElement { get { return _keyElement; } } ////// /// public IListDeclaredKeyProperties { get { if (KeyElement == null) { return EmptyKeyProperties; } return KeyElement.KeyProperties; } } /// /// /// ///public IList KeyProperties { get { if (KeyElement == null) { if (BaseType != null) { System.Diagnostics.Debug.Assert(BaseType is SchemaEntityType, "ItemType.BaseType is not ItemType"); return (BaseType as SchemaEntityType).KeyProperties; } return EmptyKeyProperties; } return this.KeyElement.KeyProperties; } } /// /// /// public ISchemaElementLookUpTableNavigationProperties { get { if (_navigationProperties == null) { _navigationProperties = new FilteredSchemaElementLookUpTable (NamedMembers); } return _navigationProperties; } } #endregion #region Protected Methods /// /// /// internal override void Validate() { // structured type base class will validate all members (properties, nav props, etc) base.Validate(); if (this.KeyElement != null) { this.KeyElement.Validate(); } } #endregion #region Protected Properties protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.Key)) { HandleKeyElement(reader); return true; } else if (CanHandleElement(reader, XmlConstants.NavigationProperty)) { HandleNavigationPropertyElement(reader); return true; } return false; } #endregion #region Private Methods ////// /// /// private void HandleNavigationPropertyElement(XmlReader reader) { NavigationProperty navigationProperty = new NavigationProperty(this); navigationProperty.Parse(reader); AddMember(navigationProperty); } ////// /// /// private void HandleKeyElement(XmlReader reader) { _keyElement = new EntityKeyElement(this); _keyElement.Parse(reader); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Xml; using System.Data; using System.Diagnostics; using metadata = System.Data.Metadata.Edm; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for Item. /// [System.Diagnostics.DebuggerDisplay("Name={Name}, BaseType={BaseType.FQName}, HasKeys={HasKeys}")] internal sealed class SchemaEntityType : StructuredType { #region Private Fields private const char KEY_DELIMITER = ' '; private ISchemaElementLookUpTable_navigationProperties = null; private EntityKeyElement _keyElement = null; private static List EmptyKeyProperties = new List (0); #endregion #region Public Methods /// /// /// /// public SchemaEntityType(Schema parentElement) : base(parentElement) { } #endregion #region Protected Methods ////// /// internal override void ResolveTopLevelNames() { base.ResolveTopLevelNames(); if (BaseType != null) { if (!(BaseType is SchemaEntityType)) { AddError(ErrorCode.InvalidBaseType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidBaseTypeForItemType(BaseType.FQName, FQName)); } // Since the base type is not null, key must be defined on the base type else if (_keyElement != null && BaseType != null) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyKeyDefinedInBaseClass(FQName, BaseType.FQName)); } } // If the base type is not null, then the key must be defined on the base entity type, since // we don't allow entity type without keys. else if (_keyElement == null) { AddError(ErrorCode.KeyMissingOnEntityType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.KeyMissingOnEntityType(this.FQName)); } else if (null == BaseType && null != UnresolvedBaseType) { // this is already an error situation, we won't do any resolve name further in this type return; } else { _keyElement.ResolveTopLevelNames(); } } #endregion #region Protected Properties protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } return false; } #endregion #region Private Methods #endregion #region Public Properties public EntityKeyElement KeyElement { get { return _keyElement; } } ////// /// public IListDeclaredKeyProperties { get { if (KeyElement == null) { return EmptyKeyProperties; } return KeyElement.KeyProperties; } } /// /// /// ///public IList KeyProperties { get { if (KeyElement == null) { if (BaseType != null) { System.Diagnostics.Debug.Assert(BaseType is SchemaEntityType, "ItemType.BaseType is not ItemType"); return (BaseType as SchemaEntityType).KeyProperties; } return EmptyKeyProperties; } return this.KeyElement.KeyProperties; } } /// /// /// public ISchemaElementLookUpTableNavigationProperties { get { if (_navigationProperties == null) { _navigationProperties = new FilteredSchemaElementLookUpTable (NamedMembers); } return _navigationProperties; } } #endregion #region Protected Methods /// /// /// internal override void Validate() { // structured type base class will validate all members (properties, nav props, etc) base.Validate(); if (this.KeyElement != null) { this.KeyElement.Validate(); } } #endregion #region Protected Properties protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.Key)) { HandleKeyElement(reader); return true; } else if (CanHandleElement(reader, XmlConstants.NavigationProperty)) { HandleNavigationPropertyElement(reader); return true; } return false; } #endregion #region Private Methods ////// /// /// private void HandleNavigationPropertyElement(XmlReader reader) { NavigationProperty navigationProperty = new NavigationProperty(this); navigationProperty.Parse(reader); AddMember(navigationProperty); } ////// /// /// private void HandleKeyElement(XmlReader reader) { _keyElement = new EntityKeyElement(this); _keyElement.Parse(reader); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
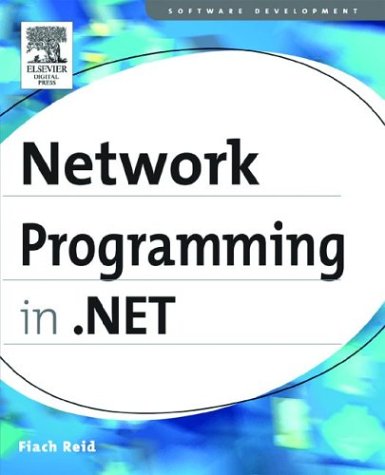
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbConnectionClosed.cs
- MetadataArtifactLoaderFile.cs
- objectquery_tresulttype.cs
- SharedDp.cs
- LinkButton.cs
- SamlDoNotCacheCondition.cs
- MenuItemBinding.cs
- _PooledStream.cs
- KeyValueSerializer.cs
- PhonemeConverter.cs
- Tile.cs
- WindowsPen.cs
- ScriptingScriptResourceHandlerSection.cs
- FormViewAutoFormat.cs
- XD.cs
- XsltInput.cs
- UpdateManifestForBrowserApplication.cs
- OLEDB_Enum.cs
- PipeStream.cs
- BaseParser.cs
- IgnoreDeviceFilterElement.cs
- SessionStateModule.cs
- DataGridViewLinkColumn.cs
- DataServiceQueryException.cs
- PeerMessageDispatcher.cs
- SourceInterpreter.cs
- RegexInterpreter.cs
- StringCollectionMarkupSerializer.cs
- StateMachineHelpers.cs
- FontFamilyIdentifier.cs
- LinkArea.cs
- RecordConverter.cs
- DependencyObjectPropertyDescriptor.cs
- DefaultTraceListener.cs
- SecurityTokenTypes.cs
- NotImplementedException.cs
- AssemblyBuilder.cs
- HitTestParameters.cs
- CrossAppDomainChannel.cs
- PrimarySelectionAdorner.cs
- X509Extension.cs
- ReferencedType.cs
- RadioButtonDesigner.cs
- SafeHandles.cs
- HttpListenerException.cs
- ParserExtension.cs
- Bits.cs
- DesignerCategoryAttribute.cs
- HeaderedItemsControl.cs
- ClientBuildManager.cs
- AutomationElementIdentifiers.cs
- Hex.cs
- FullTextLine.cs
- PermissionSetTriple.cs
- TypefaceMap.cs
- DataIdProcessor.cs
- RoutedEvent.cs
- Permission.cs
- ParagraphResult.cs
- DiscoveryReference.cs
- IISUnsafeMethods.cs
- Guid.cs
- XPathBuilder.cs
- PersonalizationState.cs
- DataViewManager.cs
- WebMessageEncoderFactory.cs
- CfgSemanticTag.cs
- XmlNodeList.cs
- TextSelection.cs
- DataShape.cs
- PrincipalPermission.cs
- NamespaceExpr.cs
- DelegatingHeader.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- JsonCollectionDataContract.cs
- XsltContext.cs
- SmiSettersStream.cs
- MostlySingletonList.cs
- X509Utils.cs
- SelectionEditor.cs
- XmlConvert.cs
- CroppedBitmap.cs
- BitConverter.cs
- RootDesignerSerializerAttribute.cs
- SocketStream.cs
- WSUtilitySpecificationVersion.cs
- FormViewDeletedEventArgs.cs
- ListViewContainer.cs
- TemplateXamlParser.cs
- TextEditor.cs
- PolyLineSegment.cs
- TypeHelper.cs
- ErrorEventArgs.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- PerformanceCountersElement.cs
- WebGetAttribute.cs
- DataBindingCollectionConverter.cs
- WebRequestModuleElement.cs
- TypedColumnHandler.cs
- NetSectionGroup.cs