Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Security / Util / Hex.cs / 1 / Hex.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /* * Hex.cs * * Operations to convert to and from Hex * */ namespace System.Security.Util { using System; using System.Security; internal static class Hex { // converts number to hex digit. Does not do any range checks. static char HexDigit(int num) { return (char)((num < 10) ? (num + '0') : (num + ('A' - 10))); } public static String EncodeHexString(byte[] sArray) { String result = null; if(sArray != null) { char[] hexOrder = new char[sArray.Length * 2]; int digit; for(int i = 0, j = 0; i < sArray.Length; i++) { digit = (int)((sArray[i] & 0xf0) >> 4); hexOrder[j++] = HexDigit(digit); digit = (int)(sArray[i] & 0x0f); hexOrder[j++] = HexDigit(digit); } result = new String(hexOrder); } return result; } internal static string EncodeHexStringFromInt(byte[] sArray) { String result = null; if(sArray != null) { char[] hexOrder = new char[sArray.Length * 2]; int i = sArray.Length; int digit, j=0; while (i-- > 0) { digit = (sArray[i] & 0xf0) >> 4; hexOrder[j++] = HexDigit(digit); digit = sArray[i] & 0x0f; hexOrder[j++] = HexDigit(digit); } result = new String(hexOrder); } return result; } public static int ConvertHexDigit(Char val) { if (val <= '9' && val >= '0') return (val - '0'); else if (val >= 'a' && val <= 'f') return ((val - 'a') + 10); else if (val >= 'A' && val <= 'F') return ((val - 'A') + 10); else throw new ArgumentException( Environment.GetResourceString( "ArgumentOutOfRange_Index" ) ); } public static byte[] DecodeHexString(String hexString) { if (hexString == null) throw new ArgumentNullException( "hexString" ); bool spaceSkippingMode = false; int i = 0; int length = hexString.Length; if ((length >= 2) && (hexString[0] == '0') && ( (hexString[1] == 'x') || (hexString[1] == 'X') )) { length = hexString.Length - 2; i = 2; } // Hex strings must always have 2N or (3N - 1) entries. if (length % 2 != 0 && length % 3 != 2) { throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidHexFormat" ) ); } byte[] sArray; if (length >=3 && hexString[i + 2] == ' ') { spaceSkippingMode = true; // Each hex digit will take three spaces, except the first (hence the plus 1). sArray = new byte[length / 3 + 1]; } else { // Each hex digit will take two spaces sArray = new byte[length / 2]; } int digit; int rawdigit; for (int j = 0; i < hexString.Length; i += 2, j++) { rawdigit = ConvertHexDigit(hexString[i]); digit = ConvertHexDigit(hexString[i+1]); sArray[j] = (byte) (digit | (rawdigit << 4)); if (spaceSkippingMode) i++; } return(sArray); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /* * Hex.cs * * Operations to convert to and from Hex * */ namespace System.Security.Util { using System; using System.Security; internal static class Hex { // converts number to hex digit. Does not do any range checks. static char HexDigit(int num) { return (char)((num < 10) ? (num + '0') : (num + ('A' - 10))); } public static String EncodeHexString(byte[] sArray) { String result = null; if(sArray != null) { char[] hexOrder = new char[sArray.Length * 2]; int digit; for(int i = 0, j = 0; i < sArray.Length; i++) { digit = (int)((sArray[i] & 0xf0) >> 4); hexOrder[j++] = HexDigit(digit); digit = (int)(sArray[i] & 0x0f); hexOrder[j++] = HexDigit(digit); } result = new String(hexOrder); } return result; } internal static string EncodeHexStringFromInt(byte[] sArray) { String result = null; if(sArray != null) { char[] hexOrder = new char[sArray.Length * 2]; int i = sArray.Length; int digit, j=0; while (i-- > 0) { digit = (sArray[i] & 0xf0) >> 4; hexOrder[j++] = HexDigit(digit); digit = sArray[i] & 0x0f; hexOrder[j++] = HexDigit(digit); } result = new String(hexOrder); } return result; } public static int ConvertHexDigit(Char val) { if (val <= '9' && val >= '0') return (val - '0'); else if (val >= 'a' && val <= 'f') return ((val - 'a') + 10); else if (val >= 'A' && val <= 'F') return ((val - 'A') + 10); else throw new ArgumentException( Environment.GetResourceString( "ArgumentOutOfRange_Index" ) ); } public static byte[] DecodeHexString(String hexString) { if (hexString == null) throw new ArgumentNullException( "hexString" ); bool spaceSkippingMode = false; int i = 0; int length = hexString.Length; if ((length >= 2) && (hexString[0] == '0') && ( (hexString[1] == 'x') || (hexString[1] == 'X') )) { length = hexString.Length - 2; i = 2; } // Hex strings must always have 2N or (3N - 1) entries. if (length % 2 != 0 && length % 3 != 2) { throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidHexFormat" ) ); } byte[] sArray; if (length >=3 && hexString[i + 2] == ' ') { spaceSkippingMode = true; // Each hex digit will take three spaces, except the first (hence the plus 1). sArray = new byte[length / 3 + 1]; } else { // Each hex digit will take two spaces sArray = new byte[length / 2]; } int digit; int rawdigit; for (int j = 0; i < hexString.Length; i += 2, j++) { rawdigit = ConvertHexDigit(hexString[i]); digit = ConvertHexDigit(hexString[i+1]); sArray[j] = (byte) (digit | (rawdigit << 4)); if (spaceSkippingMode) i++; } return(sArray); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
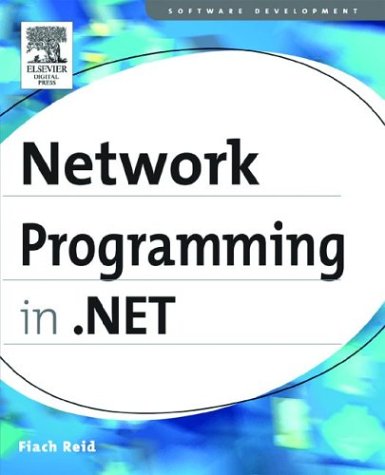
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssemblyAttributes.cs
- DataGridViewImageCell.cs
- SpellerHighlightLayer.cs
- Stack.cs
- PatternMatchRules.cs
- DesignerAttribute.cs
- WebResponse.cs
- ItemCheckEvent.cs
- SqlXmlStorage.cs
- SoapTypeAttribute.cs
- ClickablePoint.cs
- AppearanceEditorPart.cs
- MemberCollection.cs
- AsyncCompletedEventArgs.cs
- DesignerContextDescriptor.cs
- XmlSchemaComplexContentRestriction.cs
- ContentPlaceHolderDesigner.cs
- ClassicBorderDecorator.cs
- UInt64Storage.cs
- SafeNativeMethods.cs
- Stroke.cs
- HiddenFieldPageStatePersister.cs
- DrawingState.cs
- QilGenerator.cs
- BooleanConverter.cs
- OleDbException.cs
- SymbolType.cs
- WindowsScrollBarBits.cs
- _NTAuthentication.cs
- IndexOutOfRangeException.cs
- LambdaCompiler.Generated.cs
- SimpleMailWebEventProvider.cs
- RemoteX509Token.cs
- Vector3DCollection.cs
- DependencyPropertyHelper.cs
- ConstNode.cs
- Nullable.cs
- CircleHotSpot.cs
- CancelEventArgs.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- RenderContext.cs
- SafeRightsManagementEnvironmentHandle.cs
- BehaviorEditorPart.cs
- CqlLexer.cs
- CommonRemoteMemoryBlock.cs
- XmlDataLoader.cs
- CompositeCollection.cs
- CryptoConfig.cs
- BackStopAuthenticationModule.cs
- WeakReferenceList.cs
- FontResourceCache.cs
- MenuItemCollection.cs
- NumberSubstitution.cs
- HebrewNumber.cs
- Scheduler.cs
- UrlEncodedParameterWriter.cs
- RangeValidator.cs
- WorkflowView.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- MenuItemStyleCollection.cs
- QilTernary.cs
- HtmlTable.cs
- ValuePattern.cs
- PipelineModuleStepContainer.cs
- SqlDelegatedTransaction.cs
- Parser.cs
- StateRuntime.cs
- SecurityContextSecurityTokenParameters.cs
- FileCodeGroup.cs
- WeakReferenceList.cs
- StrokeCollectionDefaultValueFactory.cs
- RequestCachePolicy.cs
- SplineKeyFrames.cs
- serverconfig.cs
- TableItemStyle.cs
- HtmlElement.cs
- DataGridPageChangedEventArgs.cs
- SectionUpdates.cs
- ContextStaticAttribute.cs
- ModulesEntry.cs
- ElapsedEventArgs.cs
- DataTemplateSelector.cs
- ChtmlTextWriter.cs
- SimpleNameService.cs
- InputMethod.cs
- ConfigurationSectionGroupCollection.cs
- DocumentViewerHelper.cs
- MetafileHeaderWmf.cs
- LazyInitializer.cs
- DocumentViewerBase.cs
- SqlDataSourceQueryEditor.cs
- AspCompat.cs
- SearchForVirtualItemEventArgs.cs
- EdmFunction.cs
- SiteMapNode.cs
- BrowserCapabilitiesCodeGenerator.cs
- WorkflowLayouts.cs
- ViewBox.cs
- BamlRecordHelper.cs
- EventLogPropertySelector.cs