Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / TextFormatting / DrawingState.cs / 1305600 / DrawingState.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: DrawingState.cs // // Contents: Drawing state of full text // // Created: 1-29-2005 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; namespace MS.Internal.TextFormatting { ////// Formatting state of full text /// internal sealed class DrawingState : IDisposable { private TextMetrics.FullTextLine _currentLine; // full text line currently formatted private DrawingContext _drawingContext; // current drawing context private Point _lineOrigin; // line origin XY relative to drawing context reference location private Point _vectorToLineOrigin; // vector to line origin in UV relative to paragraph start private MatrixTransform _antiInversion; // anti-inversion transform applied on drawing surface private bool _overrideBaseGuidelineY; // a flag indicating whether a new guideline overrides the line's Y guideline private double _baseGuidelineY; // the Y guideline of the text line. ////// Construct drawing state for full text /// internal DrawingState( DrawingContext drawingContext, Point lineOrigin, MatrixTransform antiInversion, TextMetrics.FullTextLine currentLine ) { _drawingContext = drawingContext; _antiInversion = antiInversion; _currentLine = currentLine; if (antiInversion == null) { _lineOrigin = lineOrigin; } else { _vectorToLineOrigin = lineOrigin; } if (_drawingContext != null) { // LineServices draws GlyphRun and TextDecorations in multiple // callbacks and GlyphRuns may have different baselines. Pushing guideline // for each DrawGlyphRun are too costly. We optimize for the common case where // GlyphRuns and TextDecorations in the TextLine share the same baseline. _baseGuidelineY = lineOrigin.Y + currentLine.Baseline; _drawingContext.PushGuidelineY1(_baseGuidelineY); } } ////// Set guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void SetGuidelineY(double runGuidelineY) { if (_drawingContext == null) return; Invariant.Assert(!_overrideBaseGuidelineY); if (runGuidelineY != _baseGuidelineY) { // Push a new guideline to override the line's guideline _drawingContext.PushGuidelineY1(runGuidelineY); _overrideBaseGuidelineY = true; // the new Guideline Y overrides the line's guideline until next unset. } } ////// Unset guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void UnsetGuidelineY() { if (_overrideBaseGuidelineY) { _drawingContext.Pop(); _overrideBaseGuidelineY = false; } } ////// Clean up internal state. /// public void Dispose() { // clear the guideline at line's baseline if (_drawingContext != null) { _drawingContext.Pop(); } } ////// Current drawing context /// internal DrawingContext DrawingContext { get { return _drawingContext; } } ////// Anti-inversion transform applied on drawing surface /// internal MatrixTransform AntiInversion { get { return _antiInversion; } } ////// Origin XY of the current line relative to the drawing context reference location /// internal Point LineOrigin { get { return _lineOrigin; } } ////// Vector to line origin in UV relative to paragraph start /// internal Point VectorToLineOrigin { get { return _vectorToLineOrigin; } } ////// Line currently being drawn /// internal TextMetrics.FullTextLine CurrentLine { get { return _currentLine; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
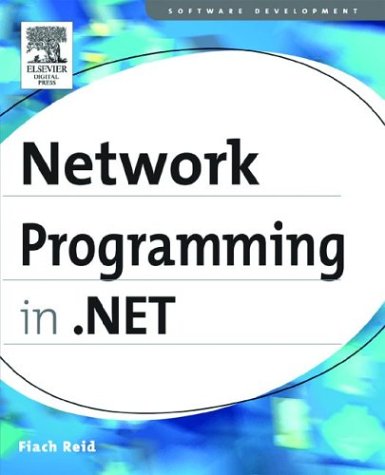
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TripleDESCryptoServiceProvider.cs
- StylusOverProperty.cs
- DataBoundControlAdapter.cs
- ResizeGrip.cs
- SecurityState.cs
- HorizontalAlignConverter.cs
- SafeArrayTypeMismatchException.cs
- WebException.cs
- DesignTable.cs
- Image.cs
- SizeConverter.cs
- PersonalizationDictionary.cs
- SafePointer.cs
- WebBrowserProgressChangedEventHandler.cs
- SystemIPv4InterfaceProperties.cs
- ListViewEditEventArgs.cs
- TextHidden.cs
- XPathAncestorQuery.cs
- Attribute.cs
- TogglePatternIdentifiers.cs
- ExceptionHelpers.cs
- CheckBoxField.cs
- FormViewRow.cs
- EntitySqlException.cs
- FixedSOMTableCell.cs
- StorageMappingItemCollection.cs
- ArgumentNullException.cs
- RadioButtonPopupAdapter.cs
- SiblingIterators.cs
- Literal.cs
- BuildManager.cs
- CodePageEncoding.cs
- SecurityDocument.cs
- ScriptReference.cs
- RoutedEvent.cs
- XmlConvert.cs
- HandleValueEditor.cs
- MaskedTextBox.cs
- HealthMonitoringSectionHelper.cs
- Sql8ConformanceChecker.cs
- SessionStateContainer.cs
- GrammarBuilderWildcard.cs
- FontDifferentiator.cs
- DrawingDrawingContext.cs
- TextRunTypographyProperties.cs
- ModuleBuilderData.cs
- SafeRightsManagementEnvironmentHandle.cs
- ObjectDataSource.cs
- XmlILModule.cs
- MILUtilities.cs
- ToolTipAutomationPeer.cs
- DataGridPagingPage.cs
- SmiConnection.cs
- PathFigureCollectionConverter.cs
- LinqDataSourceSelectEventArgs.cs
- SecurityRequiresReviewAttribute.cs
- SchemaExporter.cs
- PathSegment.cs
- SmtpFailedRecipientException.cs
- BaseParaClient.cs
- ContentHostHelper.cs
- FormsAuthentication.cs
- XmlParserContext.cs
- Msec.cs
- InsufficientMemoryException.cs
- OnOperation.cs
- AssociationType.cs
- GroupBoxAutomationPeer.cs
- ModelPropertyDescriptor.cs
- GeometryGroup.cs
- UIElement.cs
- StructuralCache.cs
- DocumentApplication.cs
- AdRotatorDesigner.cs
- SqlWebEventProvider.cs
- Policy.cs
- FixedSOMGroup.cs
- URLString.cs
- WebConfigurationFileMap.cs
- TextRange.cs
- CapabilitiesState.cs
- FixedElement.cs
- SmtpDateTime.cs
- SqlInternalConnection.cs
- ExtenderProvidedPropertyAttribute.cs
- EventListener.cs
- ManipulationDeltaEventArgs.cs
- RawStylusInput.cs
- AssemblyAttributesGoHere.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- Stream.cs
- ClientBuildManagerCallback.cs
- ErrorProvider.cs
- cookiecontainer.cs
- ArrangedElement.cs
- MultilineStringEditor.cs
- CompilationUnit.cs
- NonBatchDirectoryCompiler.cs
- COSERVERINFO.cs
- EventProviderWriter.cs