Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntitySqlException.cs / 1305376 / EntitySqlException.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- namespace System.Data { using System; using System.IO; using System.Data.Common.EntitySql; using System.Globalization; using System.Runtime.Serialization; using System.Security.Permissions; using System.Diagnostics; using System.Text; ////// Represents an eSQL Query compilation exception; /// The class of exceptional conditions that may cause this exception to be raised are mainly: /// 1) Syntax Errors: raised during query text parsing and when a given query does not conform to eSQL formal grammar; /// 2) Semantic Errors: raised when semantic rules of eSQL language are not met such as metadata or schema information /// not accurate or not present, type validation errors, scoping rule violations, user of undefined variables, etc. /// For more information, see eSQL Language Spec. /// [Serializable] public sealed class EntitySqlException : EntityException { #region Private Fields ////// represents the full error message containing the error description, the error context if available, line /// and column number if available. /// private string _message; ////// error message description. /// private string _errorDescription; ////// information about the context where the error occurred /// private string _errorContext; ////// error line number /// private int _line; ////// error column number /// private int _column; #endregion #region Public Constructors ////// Initializes a new instance of public EntitySqlException() : this(System.Data.Entity.Strings.GeneralQueryError) { HResult = HResults.InvalidQuery; } ///with the generic error message. /// /// Initializes a new instance of public EntitySqlException(string message) { _message = message; HResult = HResults.InvalidQuery; } ///with the given message. /// /// Initializes a new instance of public EntitySqlException(string message, Exception innerException) : base(message, innerException) { _message = message; HResult = HResults.InvalidQuery; } ///with the given message and innerException instance. /// /// Initializes a new instance /// /// private EntitySqlException(SerializationInfo serializationInfo, StreamingContext streamingContext) : base(serializationInfo, streamingContext) { HResult = HResults.InvalidQuery; } #endregion #region Internal Constructors ///with the given serializationInfo and streamingContext. /// /// Initializes a new instance EntityException with an ErrorContext instance and a given error message. /// internal static EntitySqlException Create(ErrorContext errCtx, string errorMessage, Exception innerException) { return EntitySqlException.Create(errCtx.CommandText, errorMessage, errCtx.InputPosition, errCtx.ErrorContextInfo, errCtx.UseContextInfoAsResourceIdentifier, innerException); } ////// Initializes a new instance EntityException with contextual information to allow detailed error feedback. /// internal static EntitySqlException Create(string commandText, string errorDescription, int errorPosition, string errorContextInfo, bool loadErrorContextInfoFromResource, Exception innerException) { int line; int column; string errorContext = FormatErrorContext(commandText, errorPosition, errorContextInfo, loadErrorContextInfoFromResource, out line, out column); string errorMessage = FormatQueryError(errorDescription, errorContext); return new EntitySqlException(errorMessage, errorDescription, errorContext, line, column, innerException); } ////// core constructor /// private EntitySqlException(string message, string errorDescription, string errorContext, int line, int column, Exception innerException) : base(message, innerException) { _message = message; _errorDescription = errorDescription; _errorContext = errorContext; _line = line; _column = column; HResult = HResults.InvalidQuery; } #endregion #region Public Properties ////// Gets the full error message containing the error description, the error context if available, line /// and column numbers if available /// public override string Message { get { return _message ?? String.Empty; } } ////// Gets the error description explaining the reason why the query was not accepted or an empty String.Empty /// public string ErrorDescription { get { return _errorDescription ?? String.Empty; } } ////// Gets the aproximate context where the error occurred if available. /// public string ErrorContext { get { return _errorContext ?? String.Empty; } } ////// Returns the the aproximate line number where the error occurred /// public int Line { get { return _line; } } ////// Returns the the aproximate column number where the error occurred /// public int Column { get { return _column; } } #endregion #region Helpers internal static string GetGenericErrorMessage(string commandText, int position) { int lineNumber = 0; int colNumber = 0; return FormatErrorContext(commandText, position, EntityRes.GenericSyntaxError, true, out lineNumber, out colNumber); } ////// Returns error context in the format [[errorContextInfo, ]line ddd, column ddd]. /// Returns empty string if errorPosition is less than 0 and errorContextInfo is not specified. /// internal static string FormatErrorContext( string commandText, int errorPosition, string errorContextInfo, bool loadErrorContextInfoFromResource, out int lineNumber, out int columnNumber) { Debug.Assert(errorPosition > -1, "position in input stream cannot be < 0"); Debug.Assert(errorPosition <= commandText.Length, "position in input stream cannot be greater than query text size"); if (loadErrorContextInfoFromResource) { errorContextInfo = !String.IsNullOrEmpty(errorContextInfo) ? EntityRes.GetString(errorContextInfo) : String.Empty; } // // Replace control chars and newLines for single representation characters // StringBuilder sb = new StringBuilder(commandText.Length); for (int i = 0; i < commandText.Length; i++) { Char c = commandText[i]; if (CqlLexer.IsNewLine(c)) { c = '\n'; } else if ((Char.IsControl(c) || Char.IsWhiteSpace(c)) && ('\r' != c)) { c = ' '; } sb.Append(c); } commandText = sb.ToString().TrimEnd(new char[] { '\n' }); // // Compute line and column // string[] queryLines = commandText.Split(new char[] { '\n' }, StringSplitOptions.None); for (lineNumber = 0, columnNumber = errorPosition; lineNumber < queryLines.Length && columnNumber > queryLines[lineNumber].Length; columnNumber -= (queryLines[lineNumber].Length + 1), ++lineNumber) ; ++lineNumber; // switch lineNum and colNum to 1-based indexes ++columnNumber; // // Error context format: "[errorContextInfo,] line ddd, column ddd" // sb = new Text.StringBuilder(); if (!String.IsNullOrEmpty(errorContextInfo)) { sb.AppendFormat(CultureInfo.CurrentCulture, "{0}, ", errorContextInfo); } if (errorPosition >= 0) { sb.AppendFormat(CultureInfo.CurrentCulture, "{0} {1}, {2} {3}", System.Data.Entity.Strings.LocalizedLine, lineNumber, System.Data.Entity.Strings.LocalizedColumn, columnNumber); } return sb.ToString(); } ////// Returns error message in the format: "error such and such[, near errorContext]." /// private static string FormatQueryError(string errorMessage, string errorContext) { // // Message format: error such and such[, near errorContextInfo]. // StringBuilder sb = new StringBuilder(); sb.Append(errorMessage); if (!String.IsNullOrEmpty(errorContext)) { sb.AppendFormat(CultureInfo.CurrentCulture, " {0} {1}", System.Data.Entity.Strings.LocalizedNear, errorContext); } return sb.Append(".").ToString(); } #endregion #region ISerializable implementation ////// sets the System.Runtime.Serialization.SerializationInfo /// with information about the exception. /// /// The System.Runtime.Serialization.SerializationInfo that holds the serialized /// object data about the exception being thrown. /// /// [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); info.AddValue("Message", _message); info.AddValue("ErrorDescription", _errorDescription); info.AddValue("ErrorContext", _errorContext); info.AddValue("Line", _line); info.AddValue("Column", _column); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- namespace System.Data { using System; using System.IO; using System.Data.Common.EntitySql; using System.Globalization; using System.Runtime.Serialization; using System.Security.Permissions; using System.Diagnostics; using System.Text; ////// Represents an eSQL Query compilation exception; /// The class of exceptional conditions that may cause this exception to be raised are mainly: /// 1) Syntax Errors: raised during query text parsing and when a given query does not conform to eSQL formal grammar; /// 2) Semantic Errors: raised when semantic rules of eSQL language are not met such as metadata or schema information /// not accurate or not present, type validation errors, scoping rule violations, user of undefined variables, etc. /// For more information, see eSQL Language Spec. /// [Serializable] public sealed class EntitySqlException : EntityException { #region Private Fields ////// represents the full error message containing the error description, the error context if available, line /// and column number if available. /// private string _message; ////// error message description. /// private string _errorDescription; ////// information about the context where the error occurred /// private string _errorContext; ////// error line number /// private int _line; ////// error column number /// private int _column; #endregion #region Public Constructors ////// Initializes a new instance of public EntitySqlException() : this(System.Data.Entity.Strings.GeneralQueryError) { HResult = HResults.InvalidQuery; } ///with the generic error message. /// /// Initializes a new instance of public EntitySqlException(string message) { _message = message; HResult = HResults.InvalidQuery; } ///with the given message. /// /// Initializes a new instance of public EntitySqlException(string message, Exception innerException) : base(message, innerException) { _message = message; HResult = HResults.InvalidQuery; } ///with the given message and innerException instance. /// /// Initializes a new instance /// /// private EntitySqlException(SerializationInfo serializationInfo, StreamingContext streamingContext) : base(serializationInfo, streamingContext) { HResult = HResults.InvalidQuery; } #endregion #region Internal Constructors ///with the given serializationInfo and streamingContext. /// /// Initializes a new instance EntityException with an ErrorContext instance and a given error message. /// internal static EntitySqlException Create(ErrorContext errCtx, string errorMessage, Exception innerException) { return EntitySqlException.Create(errCtx.CommandText, errorMessage, errCtx.InputPosition, errCtx.ErrorContextInfo, errCtx.UseContextInfoAsResourceIdentifier, innerException); } ////// Initializes a new instance EntityException with contextual information to allow detailed error feedback. /// internal static EntitySqlException Create(string commandText, string errorDescription, int errorPosition, string errorContextInfo, bool loadErrorContextInfoFromResource, Exception innerException) { int line; int column; string errorContext = FormatErrorContext(commandText, errorPosition, errorContextInfo, loadErrorContextInfoFromResource, out line, out column); string errorMessage = FormatQueryError(errorDescription, errorContext); return new EntitySqlException(errorMessage, errorDescription, errorContext, line, column, innerException); } ////// core constructor /// private EntitySqlException(string message, string errorDescription, string errorContext, int line, int column, Exception innerException) : base(message, innerException) { _message = message; _errorDescription = errorDescription; _errorContext = errorContext; _line = line; _column = column; HResult = HResults.InvalidQuery; } #endregion #region Public Properties ////// Gets the full error message containing the error description, the error context if available, line /// and column numbers if available /// public override string Message { get { return _message ?? String.Empty; } } ////// Gets the error description explaining the reason why the query was not accepted or an empty String.Empty /// public string ErrorDescription { get { return _errorDescription ?? String.Empty; } } ////// Gets the aproximate context where the error occurred if available. /// public string ErrorContext { get { return _errorContext ?? String.Empty; } } ////// Returns the the aproximate line number where the error occurred /// public int Line { get { return _line; } } ////// Returns the the aproximate column number where the error occurred /// public int Column { get { return _column; } } #endregion #region Helpers internal static string GetGenericErrorMessage(string commandText, int position) { int lineNumber = 0; int colNumber = 0; return FormatErrorContext(commandText, position, EntityRes.GenericSyntaxError, true, out lineNumber, out colNumber); } ////// Returns error context in the format [[errorContextInfo, ]line ddd, column ddd]. /// Returns empty string if errorPosition is less than 0 and errorContextInfo is not specified. /// internal static string FormatErrorContext( string commandText, int errorPosition, string errorContextInfo, bool loadErrorContextInfoFromResource, out int lineNumber, out int columnNumber) { Debug.Assert(errorPosition > -1, "position in input stream cannot be < 0"); Debug.Assert(errorPosition <= commandText.Length, "position in input stream cannot be greater than query text size"); if (loadErrorContextInfoFromResource) { errorContextInfo = !String.IsNullOrEmpty(errorContextInfo) ? EntityRes.GetString(errorContextInfo) : String.Empty; } // // Replace control chars and newLines for single representation characters // StringBuilder sb = new StringBuilder(commandText.Length); for (int i = 0; i < commandText.Length; i++) { Char c = commandText[i]; if (CqlLexer.IsNewLine(c)) { c = '\n'; } else if ((Char.IsControl(c) || Char.IsWhiteSpace(c)) && ('\r' != c)) { c = ' '; } sb.Append(c); } commandText = sb.ToString().TrimEnd(new char[] { '\n' }); // // Compute line and column // string[] queryLines = commandText.Split(new char[] { '\n' }, StringSplitOptions.None); for (lineNumber = 0, columnNumber = errorPosition; lineNumber < queryLines.Length && columnNumber > queryLines[lineNumber].Length; columnNumber -= (queryLines[lineNumber].Length + 1), ++lineNumber) ; ++lineNumber; // switch lineNum and colNum to 1-based indexes ++columnNumber; // // Error context format: "[errorContextInfo,] line ddd, column ddd" // sb = new Text.StringBuilder(); if (!String.IsNullOrEmpty(errorContextInfo)) { sb.AppendFormat(CultureInfo.CurrentCulture, "{0}, ", errorContextInfo); } if (errorPosition >= 0) { sb.AppendFormat(CultureInfo.CurrentCulture, "{0} {1}, {2} {3}", System.Data.Entity.Strings.LocalizedLine, lineNumber, System.Data.Entity.Strings.LocalizedColumn, columnNumber); } return sb.ToString(); } ////// Returns error message in the format: "error such and such[, near errorContext]." /// private static string FormatQueryError(string errorMessage, string errorContext) { // // Message format: error such and such[, near errorContextInfo]. // StringBuilder sb = new StringBuilder(); sb.Append(errorMessage); if (!String.IsNullOrEmpty(errorContext)) { sb.AppendFormat(CultureInfo.CurrentCulture, " {0} {1}", System.Data.Entity.Strings.LocalizedNear, errorContext); } return sb.Append(".").ToString(); } #endregion #region ISerializable implementation ////// sets the System.Runtime.Serialization.SerializationInfo /// with information about the exception. /// /// The System.Runtime.Serialization.SerializationInfo that holds the serialized /// object data about the exception being thrown. /// /// [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); info.AddValue("Message", _message); info.AddValue("ErrorDescription", _errorDescription); info.AddValue("ErrorContext", _errorContext); info.AddValue("Line", _line); info.AddValue("Column", _column); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
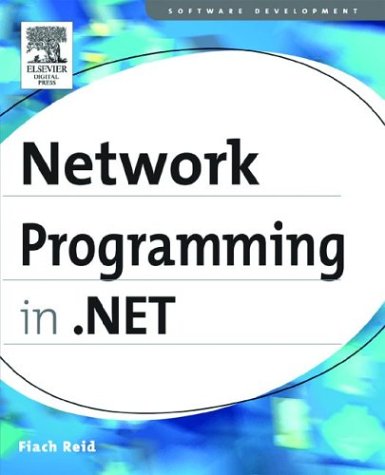
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CacheRequest.cs
- EventTrigger.cs
- Canonicalizers.cs
- XmlNotation.cs
- DataGridViewCheckBoxColumn.cs
- BezierSegment.cs
- WSFederationHttpBindingCollectionElement.cs
- ListItemCollection.cs
- DbConnectionStringBuilder.cs
- SecurityHeaderLayout.cs
- TextDataBindingHandler.cs
- RawAppCommandInputReport.cs
- FrameworkObject.cs
- RegexInterpreter.cs
- Membership.cs
- VectorCollectionConverter.cs
- PeerTransportSecurityElement.cs
- WindowsAuthenticationModule.cs
- DataPager.cs
- ChildrenQuery.cs
- XmlSchemaComplexContentExtension.cs
- HostProtectionPermission.cs
- TableCell.cs
- FormsAuthenticationModule.cs
- UIntPtr.cs
- LinkConverter.cs
- Transform3DGroup.cs
- PropertyDescriptorComparer.cs
- Parser.cs
- XmlDataSource.cs
- StylusShape.cs
- TypeLibConverter.cs
- counter.cs
- TreeNodeCollectionEditor.cs
- ReadOnlyCollectionBase.cs
- TrackingServices.cs
- QuaternionAnimation.cs
- WebOperationContext.cs
- ObjectSet.cs
- figurelengthconverter.cs
- FixedTextView.cs
- ServiceReference.cs
- InstanceData.cs
- ProcessingInstructionAction.cs
- TextTreeInsertElementUndoUnit.cs
- HttpFormatExtensions.cs
- EdmFunction.cs
- WeakReferenceList.cs
- ComponentResourceManager.cs
- DataServiceConfiguration.cs
- XmlSchemaInferenceException.cs
- _AutoWebProxyScriptEngine.cs
- RegexCode.cs
- GreenMethods.cs
- BuiltInExpr.cs
- FacetChecker.cs
- SemanticAnalyzer.cs
- SpellCheck.cs
- ChildChangedEventArgs.cs
- PersonalizationStateQuery.cs
- WebCategoryAttribute.cs
- PreservationFileReader.cs
- Path.cs
- SkinBuilder.cs
- PropertyGroupDescription.cs
- ActivityTypeDesigner.xaml.cs
- UrlAuthFailureHandler.cs
- ThreadStateException.cs
- PageFunction.cs
- TableSectionStyle.cs
- InstalledFontCollection.cs
- BaseAddressPrefixFilterElementCollection.cs
- InputScope.cs
- ToolStripSeparatorRenderEventArgs.cs
- DataSourceControlBuilder.cs
- CoreSwitches.cs
- VBCodeProvider.cs
- RemotingClientProxy.cs
- DataViewListener.cs
- Container.cs
- _ProxyRegBlob.cs
- TextTreeTextElementNode.cs
- CustomAttributeFormatException.cs
- TextModifier.cs
- TreeNodeMouseHoverEvent.cs
- RepeatButton.cs
- SystemInformation.cs
- LineInfo.cs
- OutOfProcStateClientManager.cs
- _LocalDataStoreMgr.cs
- Section.cs
- TemplateManager.cs
- BuildResultCache.cs
- Token.cs
- DynamicRouteExpression.cs
- HtmlContainerControl.cs
- ProfileService.cs
- NegotiateStream.cs
- RegistrySecurity.cs
- CatalogPartCollection.cs