Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / ProcessingInstructionAction.cs / 1 / ProcessingInstructionAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ProcessingInstructionAction : ContainerAction { private const int NameEvaluated = 2; private const int NameReady = 3; private Avt nameAvt; // Compile time precalculated AVT private string name; private const char CharX = 'X'; private const char Charx = 'x'; private const char CharM = 'M'; private const char Charm = 'm'; private const char CharL = 'L'; private const char Charl = 'l'; internal ProcessingInstructionAction() {} internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.nameAvt, Keywords.s_Name); if(this.nameAvt.IsConstant) { this.name = this.nameAvt.Evaluate(null, null); this.nameAvt = null; if (! IsProcessingInstructionName(this.name)) { // For Now: set to null to ignore action late; this.name = null; } } if (compiler.Recurse()) { CompileTemplate(compiler); compiler.ToParent(); } } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { this.nameAvt = Avt.CompileAvt(compiler, value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: if(this.nameAvt == null) { frame.StoredOutput = this.name; if(this.name == null) { // name was static but was bad; frame.Finished(); break; } } else { frame.StoredOutput = this.nameAvt.Evaluate(processor, frame); if (! IsProcessingInstructionName(frame.StoredOutput)) { frame.Finished(); break; } } goto case NameReady; case NameReady: Debug.Assert(frame.StoredOutput != null); if (processor.BeginEvent(XPathNodeType.ProcessingInstruction, string.Empty, frame.StoredOutput, string.Empty, false) == false) { // Come back later frame.State = NameReady; break; } processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; // Allow children to run case ProcessingChildren: if (processor.EndEvent(XPathNodeType.ProcessingInstruction) == false) { frame.State = ProcessingChildren; break; } frame.Finished(); break; default: Debug.Fail("Invalid ElementAction execution state"); frame.Finished(); break; } } internal static bool IsProcessingInstructionName(string name) { if (name == null) { return false; } int nameLength = name.Length; int position = 0; XmlCharType xmlCharType = XmlCharType.Instance; while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position >= nameLength) { return false; } if (position < nameLength && ! xmlCharType.IsStartNCNameChar(name[position])) { return false; } while (position < nameLength && xmlCharType.IsNCNameChar(name[position])) { position ++; } while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position < nameLength) { return false; } if (nameLength == 3 && (name[0] == CharX || name[0] == Charx) && (name[1] == CharM || name[1] == Charm) && (name[2] == CharL || name[2] == Charl) ) { return false; } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ProcessingInstructionAction : ContainerAction { private const int NameEvaluated = 2; private const int NameReady = 3; private Avt nameAvt; // Compile time precalculated AVT private string name; private const char CharX = 'X'; private const char Charx = 'x'; private const char CharM = 'M'; private const char Charm = 'm'; private const char CharL = 'L'; private const char Charl = 'l'; internal ProcessingInstructionAction() {} internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.nameAvt, Keywords.s_Name); if(this.nameAvt.IsConstant) { this.name = this.nameAvt.Evaluate(null, null); this.nameAvt = null; if (! IsProcessingInstructionName(this.name)) { // For Now: set to null to ignore action late; this.name = null; } } if (compiler.Recurse()) { CompileTemplate(compiler); compiler.ToParent(); } } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { this.nameAvt = Avt.CompileAvt(compiler, value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: if(this.nameAvt == null) { frame.StoredOutput = this.name; if(this.name == null) { // name was static but was bad; frame.Finished(); break; } } else { frame.StoredOutput = this.nameAvt.Evaluate(processor, frame); if (! IsProcessingInstructionName(frame.StoredOutput)) { frame.Finished(); break; } } goto case NameReady; case NameReady: Debug.Assert(frame.StoredOutput != null); if (processor.BeginEvent(XPathNodeType.ProcessingInstruction, string.Empty, frame.StoredOutput, string.Empty, false) == false) { // Come back later frame.State = NameReady; break; } processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; // Allow children to run case ProcessingChildren: if (processor.EndEvent(XPathNodeType.ProcessingInstruction) == false) { frame.State = ProcessingChildren; break; } frame.Finished(); break; default: Debug.Fail("Invalid ElementAction execution state"); frame.Finished(); break; } } internal static bool IsProcessingInstructionName(string name) { if (name == null) { return false; } int nameLength = name.Length; int position = 0; XmlCharType xmlCharType = XmlCharType.Instance; while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position >= nameLength) { return false; } if (position < nameLength && ! xmlCharType.IsStartNCNameChar(name[position])) { return false; } while (position < nameLength && xmlCharType.IsNCNameChar(name[position])) { position ++; } while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position < nameLength) { return false; } if (nameLength == 3 && (name[0] == CharX || name[0] == Charx) && (name[1] == CharM || name[1] == Charm) && (name[2] == CharL || name[2] == Charl) ) { return false; } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
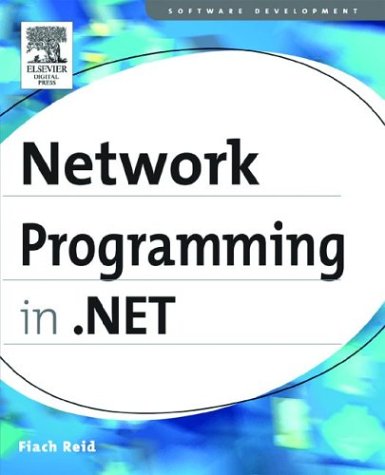
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReadWriteControlDesigner.cs
- Transform3D.cs
- COM2EnumConverter.cs
- ToolStripRenderer.cs
- Vector3D.cs
- EditorPart.cs
- RealizedColumnsBlock.cs
- ObjectNotFoundException.cs
- MetadataUtil.cs
- AbandonedMutexException.cs
- SwitchElementsCollection.cs
- ZoneButton.cs
- Calendar.cs
- Enumerable.cs
- MsmqBindingElementBase.cs
- URI.cs
- TextSelectionHighlightLayer.cs
- ObjectIDGenerator.cs
- Int64Animation.cs
- PlanCompiler.cs
- CompilationUnit.cs
- RegexCompilationInfo.cs
- Win32SafeHandles.cs
- AnchoredBlock.cs
- XPathNodeHelper.cs
- MediaCommands.cs
- MaterializeFromAtom.cs
- CompositeScriptReference.cs
- odbcmetadatafactory.cs
- SystemNetworkInterface.cs
- ContentType.cs
- DbParameterHelper.cs
- SqlNode.cs
- XmlWriterDelegator.cs
- DecoderReplacementFallback.cs
- WindowsFormsLinkLabel.cs
- SqlDataAdapter.cs
- DoubleStorage.cs
- _SSPISessionCache.cs
- MimeTypeAttribute.cs
- BitmapEffect.cs
- XmlParserContext.cs
- FloaterParagraph.cs
- DataGridViewSortCompareEventArgs.cs
- CultureTableRecord.cs
- TagNameToTypeMapper.cs
- ContentPlaceHolder.cs
- BindingExpression.cs
- DataKeyArray.cs
- DomainLiteralReader.cs
- EditingMode.cs
- SpellerHighlightLayer.cs
- SettingsBase.cs
- Process.cs
- FileCodeGroup.cs
- SoapUnknownHeader.cs
- WasEndpointConfigContainer.cs
- Visitor.cs
- ControlValuePropertyAttribute.cs
- MetadataFile.cs
- DnsPermission.cs
- ToolStripSplitStackLayout.cs
- XmlSigningNodeWriter.cs
- FileDialogCustomPlacesCollection.cs
- RecordsAffectedEventArgs.cs
- ManipulationDelta.cs
- WorkflowMarkupSerializer.cs
- SRef.cs
- InvokeGenerator.cs
- Function.cs
- ListCardsInFileRequest.cs
- GroupBoxDesigner.cs
- KeyInstance.cs
- TimestampInformation.cs
- ValidationEventArgs.cs
- JapaneseLunisolarCalendar.cs
- X509Utils.cs
- DbLambda.cs
- OAVariantLib.cs
- CodeAccessSecurityEngine.cs
- DataSourceConverter.cs
- prefixendpointaddressmessagefilter.cs
- EditingCommands.cs
- LoginName.cs
- BStrWrapper.cs
- PasswordBoxAutomationPeer.cs
- StringFunctions.cs
- DataTrigger.cs
- ThreadAttributes.cs
- WCFBuildProvider.cs
- PagedDataSource.cs
- ECDiffieHellmanPublicKey.cs
- HttpWebRequest.cs
- FileRecordSequenceCompletedAsyncResult.cs
- DefaultTextStoreTextComposition.cs
- HtmlUtf8RawTextWriter.cs
- EventLogTraceListener.cs
- Int16.cs
- ChannelParameterCollection.cs
- WebServiceMethodData.cs