Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / TextFormatting / DrawingState.cs / 1 / DrawingState.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: DrawingState.cs // // Contents: Drawing state of full text // // Created: 1-29-2005 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; namespace MS.Internal.TextFormatting { ////// Formatting state of full text /// internal sealed class DrawingState : IDisposable { private TextMetrics.FullTextLine _currentLine; // full text line currently formatted private DrawingContext _drawingContext; // current drawing context private Point _lineOrigin; // line origin XY relative to drawing context reference location private Point _vectorToLineOrigin; // vector to line origin in UV relative to paragraph start private MatrixTransform _antiInversion; // anti-inversion transform applied on drawing surface private bool _overrideBaseGuidelineY; // a flag indicating whether a new guideline overrides the line's Y guideline private double _baseGuidelineY; // the Y guideline of the text line. ////// Construct drawing state for full text /// internal DrawingState( DrawingContext drawingContext, Point lineOrigin, MatrixTransform antiInversion, TextMetrics.FullTextLine currentLine ) { _drawingContext = drawingContext; _antiInversion = antiInversion; _currentLine = currentLine; if (antiInversion == null) { _lineOrigin = lineOrigin; } else { _vectorToLineOrigin = lineOrigin; } if (_drawingContext != null) { // LineServices draws GlyphRun and TextDecorations in multiple // callbacks and GlyphRuns may have different baselines. Pushing guideline // for each DrawGlyphRun are too costly. We optimize for the common case where // GlyphRuns and TextDecorations in the TextLine share the same baseline. _baseGuidelineY = lineOrigin.Y + currentLine.Baseline; _drawingContext.PushGuidelineY1(_baseGuidelineY); } } ////// Set guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void SetGuidelineY(double runGuidelineY) { if (_drawingContext == null) return; Invariant.Assert(!_overrideBaseGuidelineY); if (runGuidelineY != _baseGuidelineY) { // Push a new guideline to override the line's guideline _drawingContext.PushGuidelineY1(runGuidelineY); _overrideBaseGuidelineY = true; // the new Guideline Y overrides the line's guideline until next unset. } } ////// Unset guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void UnsetGuidelineY() { if (_overrideBaseGuidelineY) { _drawingContext.Pop(); _overrideBaseGuidelineY = false; } } ////// Clean up internal state. /// public void Dispose() { // clear the guideline at line's baseline if (_drawingContext != null) { _drawingContext.Pop(); } } ////// Current drawing context /// internal DrawingContext DrawingContext { get { return _drawingContext; } } ////// Anti-inversion transform applied on drawing surface /// internal MatrixTransform AntiInversion { get { return _antiInversion; } } ////// Origin XY of the current line relative to the drawing context reference location /// internal Point LineOrigin { get { return _lineOrigin; } } ////// Vector to line origin in UV relative to paragraph start /// internal Point VectorToLineOrigin { get { return _vectorToLineOrigin; } } ////// Line currently being drawn /// internal TextMetrics.FullTextLine CurrentLine { get { return _currentLine; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: DrawingState.cs // // Contents: Drawing state of full text // // Created: 1-29-2005 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; namespace MS.Internal.TextFormatting { ////// Formatting state of full text /// internal sealed class DrawingState : IDisposable { private TextMetrics.FullTextLine _currentLine; // full text line currently formatted private DrawingContext _drawingContext; // current drawing context private Point _lineOrigin; // line origin XY relative to drawing context reference location private Point _vectorToLineOrigin; // vector to line origin in UV relative to paragraph start private MatrixTransform _antiInversion; // anti-inversion transform applied on drawing surface private bool _overrideBaseGuidelineY; // a flag indicating whether a new guideline overrides the line's Y guideline private double _baseGuidelineY; // the Y guideline of the text line. ////// Construct drawing state for full text /// internal DrawingState( DrawingContext drawingContext, Point lineOrigin, MatrixTransform antiInversion, TextMetrics.FullTextLine currentLine ) { _drawingContext = drawingContext; _antiInversion = antiInversion; _currentLine = currentLine; if (antiInversion == null) { _lineOrigin = lineOrigin; } else { _vectorToLineOrigin = lineOrigin; } if (_drawingContext != null) { // LineServices draws GlyphRun and TextDecorations in multiple // callbacks and GlyphRuns may have different baselines. Pushing guideline // for each DrawGlyphRun are too costly. We optimize for the common case where // GlyphRuns and TextDecorations in the TextLine share the same baseline. _baseGuidelineY = lineOrigin.Y + currentLine.Baseline; _drawingContext.PushGuidelineY1(_baseGuidelineY); } } ////// Set guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void SetGuidelineY(double runGuidelineY) { if (_drawingContext == null) return; Invariant.Assert(!_overrideBaseGuidelineY); if (runGuidelineY != _baseGuidelineY) { // Push a new guideline to override the line's guideline _drawingContext.PushGuidelineY1(runGuidelineY); _overrideBaseGuidelineY = true; // the new Guideline Y overrides the line's guideline until next unset. } } ////// Unset guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void UnsetGuidelineY() { if (_overrideBaseGuidelineY) { _drawingContext.Pop(); _overrideBaseGuidelineY = false; } } ////// Clean up internal state. /// public void Dispose() { // clear the guideline at line's baseline if (_drawingContext != null) { _drawingContext.Pop(); } } ////// Current drawing context /// internal DrawingContext DrawingContext { get { return _drawingContext; } } ////// Anti-inversion transform applied on drawing surface /// internal MatrixTransform AntiInversion { get { return _antiInversion; } } ////// Origin XY of the current line relative to the drawing context reference location /// internal Point LineOrigin { get { return _lineOrigin; } } ////// Vector to line origin in UV relative to paragraph start /// internal Point VectorToLineOrigin { get { return _vectorToLineOrigin; } } ////// Line currently being drawn /// internal TextMetrics.FullTextLine CurrentLine { get { return _currentLine; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
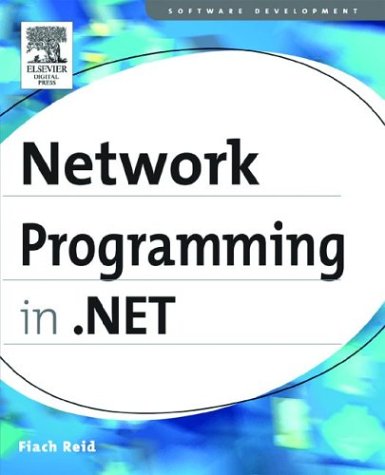
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataObjectFieldAttribute.cs
- SimpleHandlerFactory.cs
- MediaPlayerState.cs
- ListViewGroup.cs
- GetKeyedHashRequest.cs
- WindowsFormsSectionHandler.cs
- Ops.cs
- InputBinder.cs
- HashRepartitionStream.cs
- WebPartDescription.cs
- ComponentCollection.cs
- MaskedTextBox.cs
- FixedTextPointer.cs
- ScrollBarRenderer.cs
- TableLayoutStyle.cs
- DecimalConstantAttribute.cs
- OutgoingWebRequestContext.cs
- SettingsPropertyNotFoundException.cs
- ErrorFormatterPage.cs
- RegexWriter.cs
- TextParagraphView.cs
- RelativeSource.cs
- UIElement3D.cs
- AttributeUsageAttribute.cs
- CodeConditionStatement.cs
- DBConcurrencyException.cs
- BuilderInfo.cs
- SortedDictionary.cs
- FlowThrottle.cs
- RootProjectionNode.cs
- Attributes.cs
- WorkflowTimerService.cs
- FontUnitConverter.cs
- ConnectionManagementElementCollection.cs
- WebPartDisplayModeCancelEventArgs.cs
- SqlVersion.cs
- ListSortDescription.cs
- RefreshEventArgs.cs
- UIInitializationException.cs
- ZipFileInfoCollection.cs
- Trace.cs
- RuntimeHelpers.cs
- ErrorHandler.cs
- TerminatorSinks.cs
- OAVariantLib.cs
- Table.cs
- ImageDrawing.cs
- VarRemapper.cs
- SafeSecurityHandles.cs
- SoapSchemaImporter.cs
- RedirectionProxy.cs
- EntityProviderServices.cs
- BaseCollection.cs
- InheritanceAttribute.cs
- DiscoveryDefaults.cs
- DATA_BLOB.cs
- WebPartConnectionCollection.cs
- TargetFrameworkAttribute.cs
- ProtocolElementCollection.cs
- FieldInfo.cs
- SqlMethodAttribute.cs
- SchemaSetCompiler.cs
- ImpersonateTokenRef.cs
- SerialPort.cs
- BackEase.cs
- Cursor.cs
- ContextInformation.cs
- FormsAuthenticationUserCollection.cs
- DaylightTime.cs
- DataGridBoolColumn.cs
- EmptyQuery.cs
- PhonemeConverter.cs
- NullExtension.cs
- RealizedColumnsBlock.cs
- QilCloneVisitor.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- ViewManager.cs
- DataGridViewCellCancelEventArgs.cs
- TrackingServices.cs
- RSAPKCS1KeyExchangeFormatter.cs
- CrossContextChannel.cs
- UpDownBase.cs
- ListViewGroupItemCollection.cs
- WebPartZoneBaseDesigner.cs
- BitFlagsGenerator.cs
- DrawingVisualDrawingContext.cs
- ReflectionHelper.cs
- TypefaceMap.cs
- CachedFontFace.cs
- Duration.cs
- Stacktrace.cs
- SQLBytesStorage.cs
- GridSplitterAutomationPeer.cs
- PageThemeBuildProvider.cs
- TrackingCondition.cs
- securestring.cs
- SettingsBindableAttribute.cs
- Parser.cs
- SymmetricKey.cs
- TextEffectResolver.cs