Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Runtime / Remoting / RedirectionProxy.cs / 1305376 / RedirectionProxy.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // File: RedirectionProxy.cs using System; using System.Runtime.InteropServices; using System.Runtime.Remoting.Messaging; using System.Runtime.Remoting.Proxies; namespace System.Runtime.Remoting { internal class RedirectionProxy : MarshalByRefObject, IMessageSink { private MarshalByRefObject _proxy; [System.Security.SecurityCritical /*auto-generated*/] private RealProxy _realProxy; private Type _serverType; private WellKnownObjectMode _objectMode; [System.Security.SecurityCritical] // auto-generated internal RedirectionProxy(MarshalByRefObject proxy, Type serverType) { _proxy = proxy; _realProxy = RemotingServices.GetRealProxy(_proxy); _serverType = serverType; _objectMode = WellKnownObjectMode.Singleton; } // RedirectionProxy public WellKnownObjectMode ObjectMode { set { _objectMode = value; } } // ObjectMode [System.Security.SecurityCritical] // auto-generated public virtual IMessage SyncProcessMessage(IMessage msg) { IMessage replyMsg = null; try { msg.Properties["__Uri"] = _realProxy.IdentityObject.URI; if (_objectMode == WellKnownObjectMode.Singleton) { replyMsg = _realProxy.Invoke(msg); } else { // This is a single call object, so we need to create // a new instance. MarshalByRefObject obj = (MarshalByRefObject)Activator.CreateInstance(_serverType, true); BCLDebug.Assert(RemotingServices.IsTransparentProxy(obj), "expecting a proxy"); RealProxy rp = RemotingServices.GetRealProxy(obj); replyMsg = rp.Invoke(msg); } } catch (Exception e) { replyMsg = new ReturnMessage(e, msg as IMethodCallMessage); } return replyMsg; } // SyncProcessMessage [System.Security.SecurityCritical] // auto-generated public virtual IMessageCtrl AsyncProcessMessage(IMessage msg, IMessageSink replySink) { // < IMessage replyMsg = null; replyMsg = SyncProcessMessage(msg); if (replySink != null) replySink.SyncProcessMessage(replyMsg); return null; } // AsyncProcessMessage public IMessageSink NextSink { [System.Security.SecurityCritical] // auto-generated get { return null; } } } // class RedirectionProxy // This is only to be used for wellknown Singleton COM objects. internal class ComRedirectionProxy : MarshalByRefObject, IMessageSink { private MarshalByRefObject _comObject; private Type _serverType; internal ComRedirectionProxy(MarshalByRefObject comObject, Type serverType) { BCLDebug.Assert(serverType.IsCOMObject, "This must be a COM object type."); _comObject = comObject; _serverType = serverType; } // ComRedirectionProxy [System.Security.SecurityCritical] // auto-generated public virtual IMessage SyncProcessMessage(IMessage msg) { IMethodCallMessage mcmReqMsg = (IMethodCallMessage)msg; IMethodReturnMessage replyMsg = null; replyMsg = RemotingServices.ExecuteMessage(_comObject, mcmReqMsg); if (replyMsg != null) { // If an "RPC server not available" (HRESULT=0x800706BA) COM // exception is thrown, we will try to recreate the object once. const int RPC_S_SERVER_UNAVAILABLE = unchecked((int)0x800706BA); const int RPC_S_CALL_FAILED_DNE = unchecked((int)0x800706BF); COMException comException = replyMsg.Exception as COMException; if ((comException != null) && ((comException._HResult == RPC_S_SERVER_UNAVAILABLE) || (comException._HResult == RPC_S_CALL_FAILED_DNE))) { _comObject = (MarshalByRefObject)Activator.CreateInstance(_serverType, true); replyMsg = RemotingServices.ExecuteMessage(_comObject, mcmReqMsg); } } return replyMsg; } // SyncProcessMessage [System.Security.SecurityCritical] // auto-generated public virtual IMessageCtrl AsyncProcessMessage(IMessage msg, IMessageSink replySink) { // < IMessage replyMsg = null; replyMsg = SyncProcessMessage(msg); if (replySink != null) replySink.SyncProcessMessage(replyMsg); return null; } // AsyncProcessMessage public IMessageSink NextSink { [System.Security.SecurityCritical] // auto-generated get { return null; } } } // class ComRedirectionProxy } // namespace System.Runtime.Remoting // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // File: RedirectionProxy.cs using System; using System.Runtime.InteropServices; using System.Runtime.Remoting.Messaging; using System.Runtime.Remoting.Proxies; namespace System.Runtime.Remoting { internal class RedirectionProxy : MarshalByRefObject, IMessageSink { private MarshalByRefObject _proxy; [System.Security.SecurityCritical /*auto-generated*/] private RealProxy _realProxy; private Type _serverType; private WellKnownObjectMode _objectMode; [System.Security.SecurityCritical] // auto-generated internal RedirectionProxy(MarshalByRefObject proxy, Type serverType) { _proxy = proxy; _realProxy = RemotingServices.GetRealProxy(_proxy); _serverType = serverType; _objectMode = WellKnownObjectMode.Singleton; } // RedirectionProxy public WellKnownObjectMode ObjectMode { set { _objectMode = value; } } // ObjectMode [System.Security.SecurityCritical] // auto-generated public virtual IMessage SyncProcessMessage(IMessage msg) { IMessage replyMsg = null; try { msg.Properties["__Uri"] = _realProxy.IdentityObject.URI; if (_objectMode == WellKnownObjectMode.Singleton) { replyMsg = _realProxy.Invoke(msg); } else { // This is a single call object, so we need to create // a new instance. MarshalByRefObject obj = (MarshalByRefObject)Activator.CreateInstance(_serverType, true); BCLDebug.Assert(RemotingServices.IsTransparentProxy(obj), "expecting a proxy"); RealProxy rp = RemotingServices.GetRealProxy(obj); replyMsg = rp.Invoke(msg); } } catch (Exception e) { replyMsg = new ReturnMessage(e, msg as IMethodCallMessage); } return replyMsg; } // SyncProcessMessage [System.Security.SecurityCritical] // auto-generated public virtual IMessageCtrl AsyncProcessMessage(IMessage msg, IMessageSink replySink) { // < IMessage replyMsg = null; replyMsg = SyncProcessMessage(msg); if (replySink != null) replySink.SyncProcessMessage(replyMsg); return null; } // AsyncProcessMessage public IMessageSink NextSink { [System.Security.SecurityCritical] // auto-generated get { return null; } } } // class RedirectionProxy // This is only to be used for wellknown Singleton COM objects. internal class ComRedirectionProxy : MarshalByRefObject, IMessageSink { private MarshalByRefObject _comObject; private Type _serverType; internal ComRedirectionProxy(MarshalByRefObject comObject, Type serverType) { BCLDebug.Assert(serverType.IsCOMObject, "This must be a COM object type."); _comObject = comObject; _serverType = serverType; } // ComRedirectionProxy [System.Security.SecurityCritical] // auto-generated public virtual IMessage SyncProcessMessage(IMessage msg) { IMethodCallMessage mcmReqMsg = (IMethodCallMessage)msg; IMethodReturnMessage replyMsg = null; replyMsg = RemotingServices.ExecuteMessage(_comObject, mcmReqMsg); if (replyMsg != null) { // If an "RPC server not available" (HRESULT=0x800706BA) COM // exception is thrown, we will try to recreate the object once. const int RPC_S_SERVER_UNAVAILABLE = unchecked((int)0x800706BA); const int RPC_S_CALL_FAILED_DNE = unchecked((int)0x800706BF); COMException comException = replyMsg.Exception as COMException; if ((comException != null) && ((comException._HResult == RPC_S_SERVER_UNAVAILABLE) || (comException._HResult == RPC_S_CALL_FAILED_DNE))) { _comObject = (MarshalByRefObject)Activator.CreateInstance(_serverType, true); replyMsg = RemotingServices.ExecuteMessage(_comObject, mcmReqMsg); } } return replyMsg; } // SyncProcessMessage [System.Security.SecurityCritical] // auto-generated public virtual IMessageCtrl AsyncProcessMessage(IMessage msg, IMessageSink replySink) { // < IMessage replyMsg = null; replyMsg = SyncProcessMessage(msg); if (replySink != null) replySink.SyncProcessMessage(replyMsg); return null; } // AsyncProcessMessage public IMessageSink NextSink { [System.Security.SecurityCritical] // auto-generated get { return null; } } } // class ComRedirectionProxy } // namespace System.Runtime.Remoting // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
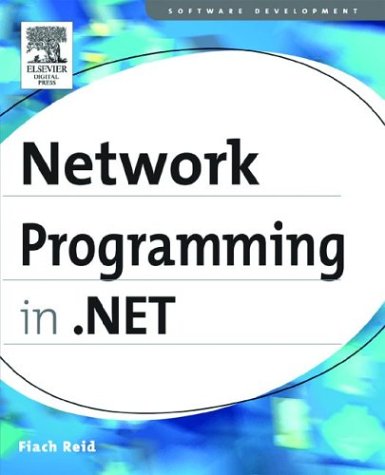
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableLayoutStyleCollection.cs
- SchemaElementDecl.cs
- JournalNavigationScope.cs
- PlacementWorkspace.cs
- BlurBitmapEffect.cs
- VolatileResourceManager.cs
- SurrogateEncoder.cs
- GlyphRunDrawing.cs
- DataGridViewIntLinkedList.cs
- SafeArrayTypeMismatchException.cs
- SubclassTypeValidator.cs
- BinaryFormatterWriter.cs
- FormsAuthenticationTicket.cs
- OracleConnectionFactory.cs
- ColorMap.cs
- XmlIlTypeHelper.cs
- Validator.cs
- ObjectStorage.cs
- SecurityCriticalDataForSet.cs
- OdbcRowUpdatingEvent.cs
- PeerTransportListenAddressValidatorAttribute.cs
- WmlValidatorAdapter.cs
- SmtpMail.cs
- HtmlLink.cs
- ValidationUtility.cs
- HostedHttpRequestAsyncResult.cs
- ErrorWrapper.cs
- DataDocumentXPathNavigator.cs
- DurableEnlistmentState.cs
- RayHitTestParameters.cs
- Part.cs
- SQLCharsStorage.cs
- OSEnvironmentHelper.cs
- ToolStripCustomTypeDescriptor.cs
- xmlglyphRunInfo.cs
- Int32CollectionConverter.cs
- XmlSchemaAppInfo.cs
- SimpleType.cs
- VisualStyleTypesAndProperties.cs
- SystemWebSectionGroup.cs
- AutoGeneratedFieldProperties.cs
- HostProtectionPermission.cs
- CaseStatement.cs
- MetadataArtifactLoader.cs
- NavigationEventArgs.cs
- BaseCollection.cs
- DbProviderFactories.cs
- XmlUtf8RawTextWriter.cs
- FrameworkContextData.cs
- _BufferOffsetSize.cs
- WhitespaceReader.cs
- ProfilePropertyNameValidator.cs
- SymDocumentType.cs
- UriParserTemplates.cs
- Scene3D.cs
- PolicyReader.cs
- VectorAnimationUsingKeyFrames.cs
- ResourceSet.cs
- Knowncolors.cs
- ComNativeDescriptor.cs
- X500Name.cs
- TextLineBreak.cs
- KeyTimeConverter.cs
- SafeThemeHandle.cs
- ContractCodeDomInfo.cs
- XmlDownloadManager.cs
- FormsAuthenticationEventArgs.cs
- IdentityVerifier.cs
- RichTextBoxAutomationPeer.cs
- WinInetCache.cs
- DataSetUtil.cs
- SourceSwitch.cs
- PackageProperties.cs
- TTSVoice.cs
- _AutoWebProxyScriptEngine.cs
- MouseBinding.cs
- XmlLanguageConverter.cs
- XmlSchemaChoice.cs
- CreateParams.cs
- Properties.cs
- FunctionOverloadResolver.cs
- SchemaEntity.cs
- ViewgenContext.cs
- SqlBuffer.cs
- UserControlParser.cs
- _WebProxyDataBuilder.cs
- EdmTypeAttribute.cs
- ObjectDisposedException.cs
- ToolStripGripRenderEventArgs.cs
- DependencyPropertyDescriptor.cs
- EditCommandColumn.cs
- RequestCacheEntry.cs
- WebPartsSection.cs
- RequestFactory.cs
- MimeMapping.cs
- OleDbErrorCollection.cs
- XamlRtfConverter.cs
- CodeTypeDeclarationCollection.cs
- ValidatorCompatibilityHelper.cs
- EqualityComparer.cs