Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Ink / StrokeCollectionConverter.cs / 1 / StrokeCollectionConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Windows.Ink; using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Threading; using System.Security; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// StrokeCollectionConverter is a class that can be used to convert StrokeCollection objects /// to strings representing base64 encoded ink, and strings to StrokeCollection objects. /// public class StrokeCollectionConverter : TypeConverter { ////// Public constructor /// public StrokeCollectionConverter() { } ////// Determines if this converter can convert an object to an StrokeCollection object /// /// An ITypeDescriptorContext that provides a format context. /// A Type that represents the type you want to convert from. ///true if this converter can perform the conversion; otherwise, false. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. /// /// An ITypeDescriptorContext that provides a format context. /// A Type that represents the type you want to convert to. ///true if this converter can perform the conversion; otherwise, false. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // This method overrides CanConvertTo from TypeConverter. This is called when someone // wants to convert an instance of StrokeCollection to another type. Here, // only conversion to an InstanceDescriptor is supported. if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Converts the given object to the converter's native type. In this implementation, a string /// representing base64 encoded ink will be converted to an StrokeCollection object. /// /// An ITypeDescriptorContext that provides a format context. /// The CultureInfo to use as the current culture. /// The Object to convert. ///An Object that represents the converted value. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { //we only support converting to / from a string string text = value as string; // [....] - presharp issue // diabling the warning for not using IsNullOrEmpty on the string since // are using the two operations for differnt results. #pragma warning disable 1634, 1691 #pragma warning disable 6507 if (text != null) { //always return an ink object //even if the string is empty text = text.Trim(); if (text.Length != 0) { using (MemoryStream ms = new MemoryStream(Convert.FromBase64String(text))) { return new StrokeCollection(ms); } } else { return new StrokeCollection(); } } #pragma warning restore 6507 #pragma warning restore 1634, 1691 return base.ConvertFrom(context, culture, value); } ////// Converts the given object to another type. In this implementation, the only supported /// convertion is from an StrokeCollection object to a string. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// /// An ITypeDescriptorContext that provides a format context. /// A CultureInfo object. If a null reference (Nothing in Visual Basic) is passed, the current culture is assumed. /// The Object to convert. /// The Type to convert the value parameter to. ///An Object that represents the converted value ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for StrokeCollection, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } //if someone wants to convert to a string... StrokeCollection strokes = value as StrokeCollection; if (strokes != null) { if (destinationType == typeof(string)) { using (MemoryStream ms = new MemoryStream()) { strokes.Save(ms, true); ms.Position = 0; return Convert.ToBase64String(ms.ToArray()); } } //if someone wants to convert to an InstanceDescriptor else if (destinationType == typeof(InstanceDescriptor)) { //get a ref to the StrokeCollection objects constructor that takes a byte[] ConstructorInfo ci = typeof(StrokeCollection).GetConstructor(new Type[] { typeof(Stream) }); // [....] - Presharp issue // Presharp gives a warning when local IDisposable variables are not closed // in this case, we can't call Dispose since it will also close the underlying stream // which strokecollection needs open to read in the constructor #pragma warning disable 1634, 1691 #pragma warning disable 6518 MemoryStream stream = new MemoryStream(); strokes.Save(stream, true/*compress*/); stream.Position = 0; return new InstanceDescriptor(ci, new object[] { stream }); #pragma warning restore 6518 #pragma warning restore 1634, 1691 } } return base.ConvertTo(context, culture, value, destinationType); } ////// Returns whether this object supports a standard set of values that can be /// picked from a list, using the specified context. /// /// An ITypeDescriptorContext that provides a format context. ////// true if GetStandardValues should be called to find a common set /// of values the object supports; otherwise, false. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
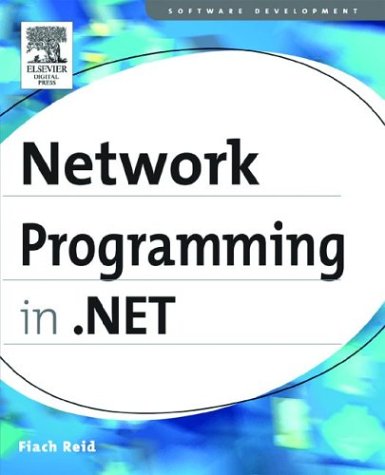
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceQueryException.cs
- WindowInteractionStateTracker.cs
- Site.cs
- EntityDataSourceView.cs
- OleDbFactory.cs
- MsmqBindingBase.cs
- ExpressionBuilderCollection.cs
- CompilationSection.cs
- InputMethod.cs
- HwndTarget.cs
- HyperLinkStyle.cs
- WebServiceResponse.cs
- ReadOnlyTernaryTree.cs
- Maps.cs
- PerformanceCounterPermission.cs
- DataViewSettingCollection.cs
- MailBnfHelper.cs
- StyleCollection.cs
- ChoiceConverter.cs
- MD5CryptoServiceProvider.cs
- Command.cs
- FormsAuthenticationConfiguration.cs
- Opcode.cs
- SchemaImporterExtensionElement.cs
- SQlBooleanStorage.cs
- HealthMonitoringSectionHelper.cs
- LocalFileSettingsProvider.cs
- ChangeProcessor.cs
- JoinQueryOperator.cs
- CryptoProvider.cs
- Scene3D.cs
- Compiler.cs
- DbCommandTree.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- CodeSubDirectoriesCollection.cs
- MouseCaptureWithinProperty.cs
- UrlPath.cs
- NonVisualControlAttribute.cs
- OptimalBreakSession.cs
- Style.cs
- SparseMemoryStream.cs
- DataGridViewLinkColumn.cs
- SelectionService.cs
- HitTestDrawingContextWalker.cs
- OleDbInfoMessageEvent.cs
- CodeCompiler.cs
- securitycriticaldataformultiplegetandset.cs
- RowsCopiedEventArgs.cs
- QueryCursorEventArgs.cs
- XmlAttributeHolder.cs
- ActivationArguments.cs
- mediaeventshelper.cs
- GridViewRowPresenterBase.cs
- QueryStringParameter.cs
- WmlTextViewAdapter.cs
- StylusTip.cs
- TextSegment.cs
- PackageController.cs
- Parameter.cs
- StringToken.cs
- Exceptions.cs
- Queue.cs
- ImageListStreamer.cs
- WebPartHelpVerb.cs
- SessionStateContainer.cs
- MessageCredentialType.cs
- SystemWebExtensionsSectionGroup.cs
- XmlHierarchicalDataSourceView.cs
- QuaternionAnimationUsingKeyFrames.cs
- FileSystemWatcher.cs
- Brush.cs
- CqlQuery.cs
- WindowsGrip.cs
- Duration.cs
- DbRetry.cs
- __TransparentProxy.cs
- Accessible.cs
- SubqueryTrackingVisitor.cs
- XmlNodeChangedEventManager.cs
- RuntimeWrappedException.cs
- CodeConditionStatement.cs
- RangeValidator.cs
- Restrictions.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- LoginDesigner.cs
- TableLayoutStyle.cs
- DocumentOrderQuery.cs
- RewritingSimplifier.cs
- StructuralCache.cs
- X509ChainElement.cs
- AnonymousIdentificationModule.cs
- OperationPickerDialog.designer.cs
- Trigger.cs
- DeviceFilterDictionary.cs
- XmlConverter.cs
- SqlOuterApplyReducer.cs
- PropertyGridEditorPart.cs
- OracleString.cs
- CheckBoxAutomationPeer.cs
- Convert.cs