Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / ClientServices / Providers / ClientWindowsAuthenticationMembershipProvider.cs / 1305376 / ClientWindowsAuthenticationMembershipProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ClientServices.Providers { using System; using System.Data; using System.Data.OleDb; using System.IO; using System.Windows.Forms; using System.Web; using System.Web.Resources; using System.Web.Security; using System.Threading; using System.Security; using System.Security.Principal; using System.Collections.Specialized; using System.Web.ClientServices; using System.Globalization; using System.Diagnostics.CodeAnalysis; public class ClientWindowsAuthenticationMembershipProvider : MembershipProvider { public override bool ValidateUser(string username, string password) { WindowsIdentity id = WindowsIdentity.GetCurrent(); if (!string.IsNullOrEmpty(password)) throw new ArgumentException(AtlasWeb.ArgumentMustBeNull, "password"); if (!string.IsNullOrEmpty(username) && string.Compare(username, id.Name, StringComparison.OrdinalIgnoreCase) != 0) throw new ArgumentException(AtlasWeb.ArgumentMustBeNull, "username"); Thread.CurrentPrincipal = new ClientRolePrincipal(id); return true; } public void Logout() { Thread.CurrentPrincipal = new WindowsPrincipal(WindowsIdentity.GetCurrent()); } public override bool EnablePasswordRetrieval { get { return false; } } public override bool EnablePasswordReset { get { return false; } } public override bool RequiresQuestionAndAnswer { get { return false; } } public override string ApplicationName { get { return ""; } set { } } public override int MaxInvalidPasswordAttempts { get { return int.MaxValue; } } public override int PasswordAttemptWindow { get { return int.MaxValue; } } public override bool RequiresUniqueEmail { get { return false; } } public override MembershipPasswordFormat PasswordFormat { get { return MembershipPasswordFormat.Hashed; } } public override int MinRequiredPasswordLength { get { return 1; } } public override int MinRequiredNonAlphanumericCharacters { get { return 0; } } public override string PasswordStrengthRegularExpression { get { return "*"; } } public override MembershipUser CreateUser(string username, string password, string email, string passwordQuestion, string passwordAnswer, bool isApproved, object providerUserKey, out MembershipCreateStatus status) { throw new NotSupportedException(); } public override bool ChangePasswordQuestionAndAnswer(string username, string password, string newPasswordQuestion, string newPasswordAnswer) { throw new NotSupportedException(); } public override string GetPassword(string username, string answer) { throw new NotSupportedException(); } public override bool ChangePassword(string username, string oldPassword, string newPassword) { throw new NotSupportedException(); } public override string ResetPassword(string username, string answer) { throw new NotSupportedException(); } public override void UpdateUser(MembershipUser user) { throw new NotSupportedException(); } public override bool UnlockUser(string username) { throw new NotSupportedException(); } public override MembershipUser GetUser(object providerUserKey, bool userIsOnline) { throw new NotSupportedException(); } public override MembershipUser GetUser(string username, bool userIsOnline) { throw new NotSupportedException(); } public override string GetUserNameByEmail(string email) { throw new NotSupportedException(); } public override bool DeleteUser(string username, bool deleteAllRelatedData) { throw new NotSupportedException(); } public override MembershipUserCollection GetAllUsers(int pageIndex, int pageSize, out int totalRecords) { throw new NotSupportedException(); } public override int GetNumberOfUsersOnline() { throw new NotSupportedException(); } public override MembershipUserCollection FindUsersByName(string usernameToMatch, int pageIndex, int pageSize, out int totalRecords) { throw new NotSupportedException(); } public override MembershipUserCollection FindUsersByEmail(string emailToMatch, int pageIndex, int pageSize, out int totalRecords) { throw new NotSupportedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
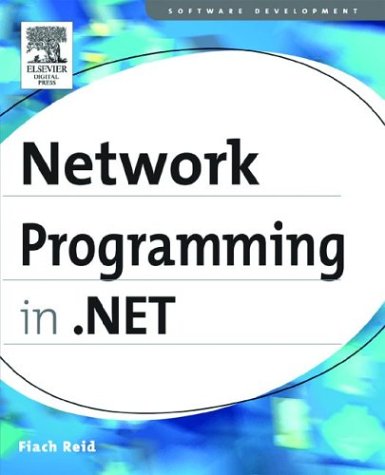
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlTypeMapping.cs
- DataSysAttribute.cs
- SimplePropertyEntry.cs
- RegularExpressionValidator.cs
- GradientStopCollection.cs
- SafeBitVector32.cs
- PrePrepareMethodAttribute.cs
- ConfigXmlComment.cs
- TextSelectionProcessor.cs
- CanExecuteRoutedEventArgs.cs
- WebPartConnection.cs
- SHA512Cng.cs
- AccessedThroughPropertyAttribute.cs
- TagPrefixAttribute.cs
- StaticContext.cs
- ToolBarDesigner.cs
- IItemProperties.cs
- NodeLabelEditEvent.cs
- AliasExpr.cs
- ExpressionBuilderCollection.cs
- StaticResourceExtension.cs
- RTLAwareMessageBox.cs
- HtmlTextBoxAdapter.cs
- ReliableMessagingVersionConverter.cs
- SQLInt16Storage.cs
- FileChangesMonitor.cs
- StorageMappingItemLoader.cs
- CommonObjectSecurity.cs
- MarkupExtensionReturnTypeAttribute.cs
- FileCodeGroup.cs
- SqlParameter.cs
- DateTimeFormatInfoScanner.cs
- ADMembershipProvider.cs
- TypeNameHelper.cs
- RemoteWebConfigurationHost.cs
- TagPrefixCollection.cs
- ResetableIterator.cs
- ControlCollection.cs
- InfoCardTraceRecord.cs
- HttpRuntimeSection.cs
- TraceContextRecord.cs
- Bold.cs
- AttributeUsageAttribute.cs
- DesignerVerbCollection.cs
- HttpRuntime.cs
- XmlSchemaDocumentation.cs
- PseudoWebRequest.cs
- Emitter.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- CacheVirtualItemsEvent.cs
- MimeReturn.cs
- OutOfProcStateClientManager.cs
- PluralizationService.cs
- _Win32.cs
- ModelServiceImpl.cs
- filewebresponse.cs
- ICspAsymmetricAlgorithm.cs
- DataGridViewRowPrePaintEventArgs.cs
- Helpers.cs
- SQLBinary.cs
- FontInfo.cs
- DoubleLinkList.cs
- SubstitutionList.cs
- oledbmetadatacollectionnames.cs
- HashJoinQueryOperatorEnumerator.cs
- DataListItemCollection.cs
- WebPartZone.cs
- FileDialog_Vista_Interop.cs
- Propagator.Evaluator.cs
- SafeBitVector32.cs
- SoapSchemaImporter.cs
- SoapSchemaExporter.cs
- RadioButtonFlatAdapter.cs
- SequenceDesigner.cs
- ValidationHelper.cs
- GetImportedCardRequest.cs
- SafeSystemMetrics.cs
- DataControlField.cs
- DataGridRowEventArgs.cs
- SoundPlayer.cs
- UniformGrid.cs
- SQLBinary.cs
- safelinkcollection.cs
- ThousandthOfEmRealDoubles.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ListenerElementsCollection.cs
- TemplateParser.cs
- CodeGeneratorOptions.cs
- XmlObjectSerializerReadContextComplexJson.cs
- CompilerGeneratedAttribute.cs
- _ChunkParse.cs
- JumpPath.cs
- WebControlParameterProxy.cs
- SubpageParagraph.cs
- PageAsyncTaskManager.cs
- SmiSettersStream.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- Automation.cs
- CompilerGeneratedAttribute.cs
- SpellerError.cs