Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / CheckBoxField.cs / 2 / CheckBoxField.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CheckBoxField : BoundField { private bool _suppressPropertyThrows = false; ///Creates a field bounded to a data field in a ///. /// public CheckBoxField() { } ///Initializes a new instance of a ///class. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override bool ApplyFormatInEditMode { get { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "ApplyFormatInEditMode")); } return false; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "ApplyFormatInEditMode")); } } } ///Indicates whether to apply the DataFormatString in edit mode ////// [ TypeConverter("System.Web.UI.Design.DataSourceBooleanViewSchemaConverter, " + AssemblyRef.SystemDesign), ] public override string DataField { get { return base.DataField; } set { base.DataField = value; } } ///Gets or sets the field name from the data model bound to this field. /// Overridden to change the type converter attribute. ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override string DataFormatString { get { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "DataFormatString")); } return String.Empty; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "DataFormatString")); } } } ///Gets or sets the display format of data in this /// field. ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override bool HtmlEncode { get { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "HtmlEncode")); } return false; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "HtmlEncode")); } } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override bool HtmlEncodeFormatString { get { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "HtmlEncodeFormatString")); } return false; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "HtmlEncodeFormatString")); } } } ///Gets or sets a property indicating whether data should be HtmlEncoded when it is displayed to the user. ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override string NullDisplayText { get { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "NullDisplayText")); } return String.Empty; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "NullDisplayText")); } } } protected override bool SupportsHtmlEncode { get { return false; } } ///Gets or sets the property that determines what text is displayed if the value /// of the field is null. ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.CheckBoxField_Text) ] public virtual string Text { get { object o = ViewState["Text"]; if (o != null) return (string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["Text"])) { ViewState["Text"] = value; OnFieldChanged(); } } } ///Gets or sets the CheckBox's Text property in this /// field. ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override bool ConvertEmptyStringToNull { get { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "ConvertEmptyStringToNull")); } return false; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(SR.GetString(SR.CheckBoxField_NotSupported, "ConvertEmptyStringToNull")); } } } protected override void CopyProperties(DataControlField newField) { ((CheckBoxField)newField).Text = Text; _suppressPropertyThrows = true; ((CheckBoxField)newField)._suppressPropertyThrows = true; base.CopyProperties(newField); _suppressPropertyThrows = false; ((CheckBoxField)newField)._suppressPropertyThrows = false; } protected override DataControlField CreateField() { return new CheckBoxField(); } ///Gets or sets the property that determines whether the BoundField treats empty string as /// null when the field values are extracted. ////// Extracts the value(s) from the given cell and puts the value(s) into a dictionary. Indicate includeReadOnly /// to have readonly fields' values inserted into the dictionary. /// public override void ExtractValuesFromCell(IOrderedDictionary dictionary, DataControlFieldCell cell, DataControlRowState rowState, bool includeReadOnly) { Control childControl = null; string dataField = DataField; object value = null; if (cell.Controls.Count > 0) { childControl = cell.Controls[0]; CheckBox checkBox = childControl as CheckBox; if (checkBox != null) { if (includeReadOnly || checkBox.Enabled) { value = checkBox.Checked; } } } if (value != null) { if (dictionary.Contains(dataField)) { dictionary[dataField] = value; } else { dictionary.Add(dataField, value); } } } ////// Returns a value to be used for design-time rendering /// protected override object GetDesignTimeValue() { return true; } ////// protected override void InitializeDataCell(DataControlFieldCell cell, DataControlRowState rowState) { CheckBox childControl = null; CheckBox boundControl = null; if (((rowState & DataControlRowState.Edit) != 0 && ReadOnly == false) || (rowState & DataControlRowState.Insert) != 0) { // CheckBox editor = new CheckBox(); editor.ToolTip = HeaderText; childControl = editor; if (DataField.Length != 0 && (rowState & DataControlRowState.Edit) != 0) { boundControl = editor; } } else if (DataField.Length != 0) { CheckBox editor = new CheckBox(); editor.Text = Text; editor.Enabled = false; childControl = editor; boundControl = editor; } if (childControl != null) { cell.Controls.Add(childControl); } if (boundControl != null && Visible) { boundControl.DataBinding += new EventHandler(this.OnDataBindField); } } ///Initializes a cell in the DataControlField. ////// Performs databinding the given field with data from the data source. /// protected override void OnDataBindField(object sender, EventArgs e) { Control boundControl = (Control)sender; Control controlContainer = boundControl.NamingContainer; object data = GetValue(controlContainer); if (!(boundControl is CheckBox)) { throw new HttpException(SR.GetString(SR.CheckBoxField_WrongControlType, DataField)); } if (DataBinder.IsNull(data)) { ((CheckBox)boundControl).Checked = false; } else { if (data is Boolean) { ((CheckBox)boundControl).Checked = (Boolean)data; } else { try { ((CheckBox)boundControl).Checked = Boolean.Parse(data.ToString()); } catch (FormatException fe) { throw new HttpException(SR.GetString(SR.CheckBoxField_CouldntParseAsBoolean, DataField), fe); } } } ((CheckBox)boundControl).Text = Text; } ////// public override void ValidateSupportsCallback() { } } }Override with an empty body if the field's controls all support callback. /// Otherwise, override and throw a useful error message about why the field can't support callbacks. ///
Link Menu
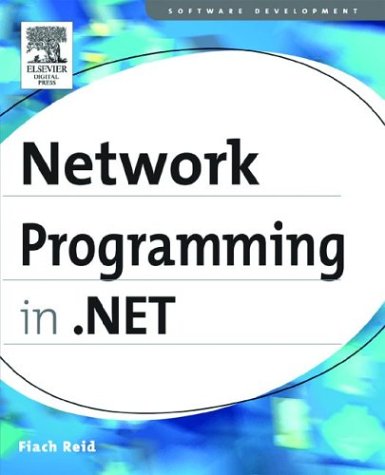
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataSourceTableQuery.cs
- TrackingValidationObjectDictionary.cs
- EntityDataSourceContextCreatedEventArgs.cs
- DynamicMethod.cs
- SignedInfo.cs
- SqlTriggerContext.cs
- RequestQueue.cs
- XmlObjectSerializerWriteContext.cs
- DockPanel.cs
- IconHelper.cs
- AuthenticationConfig.cs
- LinqDataSourceValidationException.cs
- DeleteHelper.cs
- DLinqAssociationProvider.cs
- ListViewTableRow.cs
- HeaderUtility.cs
- PageBreakRecord.cs
- ClientData.cs
- OdbcConnection.cs
- Automation.cs
- HeaderLabel.cs
- BitArray.cs
- TypeConverterHelper.cs
- AxisAngleRotation3D.cs
- ObfuscateAssemblyAttribute.cs
- SingleKeyFrameCollection.cs
- Pair.cs
- ItemContainerGenerator.cs
- DataGridViewAutoSizeModeEventArgs.cs
- TableRow.cs
- ManifestSignedXml.cs
- FatalException.cs
- Bidi.cs
- DbMetaDataColumnNames.cs
- ItemAutomationPeer.cs
- Int32Converter.cs
- FormatterServices.cs
- XmlAnyElementAttribute.cs
- HitTestParameters3D.cs
- TemplateControl.cs
- EmptyStringExpandableObjectConverter.cs
- Configuration.cs
- XmlWriter.cs
- SerTrace.cs
- KeyConverter.cs
- SrgsSubset.cs
- TextFormatterImp.cs
- ControlCachePolicy.cs
- Package.cs
- EmptyQuery.cs
- JsonServiceDocumentSerializer.cs
- BamlRecordWriter.cs
- SocketPermission.cs
- CodeAccessSecurityEngine.cs
- TransactionInformation.cs
- JournalEntryStack.cs
- SizeValueSerializer.cs
- XsdDateTime.cs
- SignerInfo.cs
- RectValueSerializer.cs
- SqlParameter.cs
- AssemblyLoader.cs
- EntityDataSourceSelectingEventArgs.cs
- BitmapImage.cs
- GridViewAutomationPeer.cs
- XmlUtil.cs
- EasingQuaternionKeyFrame.cs
- CqlIdentifiers.cs
- ExpressionNode.cs
- XmlAnyElementAttribute.cs
- WinEventWrap.cs
- LinkButton.cs
- PackageRelationship.cs
- SecurityManager.cs
- PanelStyle.cs
- CellTreeNode.cs
- PictureBox.cs
- FormClosingEvent.cs
- SharedConnectionListener.cs
- CapabilitiesState.cs
- PreservationFileWriter.cs
- GroupAggregateExpr.cs
- Visitor.cs
- CreatingCookieEventArgs.cs
- KeyFrames.cs
- XmlHierarchicalDataSourceView.cs
- XmlIgnoreAttribute.cs
- WindowsGraphicsCacheManager.cs
- MimeWriter.cs
- RegistrySecurity.cs
- AccessedThroughPropertyAttribute.cs
- ScriptingWebServicesSectionGroup.cs
- PropertyIdentifier.cs
- SqlClientPermission.cs
- FixedTextBuilder.cs
- XLinq.cs
- CollectionChangedEventManager.cs
- dbdatarecord.cs
- SelectionItemPatternIdentifiers.cs
- AddInPipelineAttributes.cs