Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / XPathBinder.cs / 1 / XPathBinder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Security.Permissions; using System.Xml; using System.Xml.XPath; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class XPathBinder { ///private XPathBinder() { } /// /// public static object Eval(object container, string xPath) { IXmlNamespaceResolver resolver = null; return Eval(container, xPath, resolver); } public static object Eval(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); object retValue = navigator.Evaluate(xPath, resolver); // If we get back an XPathNodeIterator instead of a simple object, advance // the iterator to the first node and return the value. XPathNodeIterator iterator = retValue as XPathNodeIterator; if (iterator != null) { if (iterator.MoveNext()) { retValue = iterator.Current.Value; } else { retValue = null; } } return retValue; } ////// public static string Eval(object container, string xPath, string format) { return Eval(container, xPath, format, null); } public static string Eval(object container, string xPath, string format, IXmlNamespaceResolver resolver) { object value = XPathBinder.Eval(container, xPath, resolver); if (value == null) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return String.Format(format, value); } } } ////// Evaluates an XPath query with respect to a context IXPathNavigable object that returns a NodeSet. /// public static IEnumerable Select(object container, string xPath) { return Select(container, xPath, null); } public static IEnumerable Select(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } ArrayList results = new ArrayList(); IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); XPathNodeIterator iterator = navigator.Select(xPath, resolver); while (iterator.MoveNext()) { IHasXmlNode hasXmlNode = iterator.Current as IHasXmlNode; if (hasXmlNode == null) { throw new InvalidOperationException(SR.GetString(SR.XPathBinder_MustHaveXmlNodes)); } results.Add(hasXmlNode.GetNode()); } return results; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Security.Permissions; using System.Xml; using System.Xml.XPath; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class XPathBinder { ///private XPathBinder() { } /// /// public static object Eval(object container, string xPath) { IXmlNamespaceResolver resolver = null; return Eval(container, xPath, resolver); } public static object Eval(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); object retValue = navigator.Evaluate(xPath, resolver); // If we get back an XPathNodeIterator instead of a simple object, advance // the iterator to the first node and return the value. XPathNodeIterator iterator = retValue as XPathNodeIterator; if (iterator != null) { if (iterator.MoveNext()) { retValue = iterator.Current.Value; } else { retValue = null; } } return retValue; } ////// public static string Eval(object container, string xPath, string format) { return Eval(container, xPath, format, null); } public static string Eval(object container, string xPath, string format, IXmlNamespaceResolver resolver) { object value = XPathBinder.Eval(container, xPath, resolver); if (value == null) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return String.Format(format, value); } } } ////// Evaluates an XPath query with respect to a context IXPathNavigable object that returns a NodeSet. /// public static IEnumerable Select(object container, string xPath) { return Select(container, xPath, null); } public static IEnumerable Select(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } ArrayList results = new ArrayList(); IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); XPathNodeIterator iterator = navigator.Select(xPath, resolver); while (iterator.MoveNext()) { IHasXmlNode hasXmlNode = iterator.Current as IHasXmlNode; if (hasXmlNode == null) { throw new InvalidOperationException(SR.GetString(SR.XPathBinder_MustHaveXmlNodes)); } results.Add(hasXmlNode.GetNode()); } return results; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
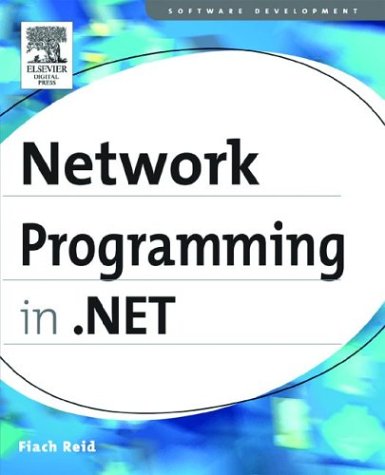
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TCPClient.cs
- WebPartConnectionsCancelVerb.cs
- ImageConverter.cs
- CodeDefaultValueExpression.cs
- SimplePropertyEntry.cs
- TypeDelegator.cs
- DBPropSet.cs
- SchemaDeclBase.cs
- PnrpPermission.cs
- HandleCollector.cs
- MultipartContentParser.cs
- Table.cs
- SimpleHandlerFactory.cs
- WindowsSpinner.cs
- ProcessHostConfigUtils.cs
- File.cs
- ASCIIEncoding.cs
- ProviderConnectionPoint.cs
- ExpressionPrefixAttribute.cs
- RelationshipType.cs
- Encoder.cs
- BaseTemplateParser.cs
- PageParser.cs
- ClientUrlResolverWrapper.cs
- TimeZone.cs
- diagnosticsswitches.cs
- DrawingBrush.cs
- ClientRuntimeConfig.cs
- TextCompositionManager.cs
- Point3DCollection.cs
- FloaterBaseParagraph.cs
- Message.cs
- TargetPerspective.cs
- SystemIcmpV4Statistics.cs
- FilterQuery.cs
- DecoderNLS.cs
- ErrorRuntimeConfig.cs
- CmsInterop.cs
- XmlDownloadManager.cs
- ServiceModelSectionGroup.cs
- PerfService.cs
- PropertyDescriptors.cs
- CompletionBookmark.cs
- ToolStripStatusLabel.cs
- RowUpdatingEventArgs.cs
- StylusEditingBehavior.cs
- ObjectDataSourceFilteringEventArgs.cs
- ToolboxItemSnapLineBehavior.cs
- VisualStyleElement.cs
- AdRotator.cs
- SqlParameterizer.cs
- ComponentCollection.cs
- TypefaceCollection.cs
- QueryContext.cs
- WsatServiceAddress.cs
- XmlComment.cs
- SqlDataSourceRefreshSchemaForm.cs
- PointLight.cs
- StreamInfo.cs
- CompModSwitches.cs
- Options.cs
- EventLogger.cs
- CatalogPart.cs
- SqlConnectionString.cs
- UpDownBase.cs
- TextServicesCompartment.cs
- EventHandlersStore.cs
- elementinformation.cs
- CachedFontFamily.cs
- InstanceDataCollection.cs
- ScriptModule.cs
- InvocationExpression.cs
- DeflateStream.cs
- FontDialog.cs
- ScopedKnownTypes.cs
- PeerChannelListener.cs
- WebPartDisplayModeEventArgs.cs
- AbsoluteQuery.cs
- HtmlInputCheckBox.cs
- ObjectSpanRewriter.cs
- XPathArrayIterator.cs
- CalendarDay.cs
- MD5CryptoServiceProvider.cs
- ApplicationServiceHelper.cs
- PlaceHolder.cs
- thaishape.cs
- SimpleTypeResolver.cs
- FactoryId.cs
- MarshalDirectiveException.cs
- TrustManager.cs
- TableLayoutRowStyleCollection.cs
- OutputCacheSettingsSection.cs
- InvokeProviderWrapper.cs
- Wildcard.cs
- DataConnectionHelper.cs
- RightsManagementEncryptedStream.cs
- COM2ColorConverter.cs
- DataGridTextBoxColumn.cs
- EventPropertyMap.cs
- UnsafeNativeMethods.cs