Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Data / System / Data / SQLTypes / SQLInt32.cs / 1 / SQLInt32.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlInt32.cs // // Create by: JunFang // // Purpose: Implementation of SqlInt32 which is equivalent to // data type "int" in SQL Server // // Notes: // // History: // // 09/17/99 JunFang Created and implemented as first drop. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Runtime.InteropServices; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [StructLayout(LayoutKind.Sequential)] [XmlSchemaProvider("GetXsdType")] public struct SqlInt32 : INullable, IComparable, IXmlSerializable { private bool m_fNotNull; // false if null, the default ctor (plain 0) will make it Null private int m_value; private static readonly long x_iIntMin = Int32.MinValue; // minimum (signed) int value private static readonly long x_lBitNotIntMax = ~(long)(Int32.MaxValue); // constructor // construct a Null private SqlInt32(bool fNull) { m_fNotNull = false; m_value = 0; } ////// Represents a 32-bit signed integer to be stored in /// or retrieved from a database. /// ////// public SqlInt32(int value) { m_value = value; m_fNotNull = true; } // INullable ///[To be supplied.] ////// public bool IsNull { get { return !m_fNotNull;} } // property: Value ///[To be supplied.] ////// public int Value { get { if (IsNull) throw new SqlNullValueException(); else return m_value; } } // Implicit conversion from int to SqlInt32 ///[To be supplied.] ////// public static implicit operator SqlInt32(int x) { return new SqlInt32(x); } // Explicit conversion from SqlInt32 to int. Throw exception if x is Null. ///[To be supplied.] ////// public static explicit operator int(SqlInt32 x) { return x.Value; } ///[To be supplied.] ////// public override String ToString() { return IsNull ? SQLResource.NullString : m_value.ToString((IFormatProvider)null); } ///[To be supplied.] ////// public static SqlInt32 Parse(String s) { if (s == SQLResource.NullString) return SqlInt32.Null; else return new SqlInt32(Int32.Parse(s, (IFormatProvider)null)); } // Unary operators ///[To be supplied.] ////// public static SqlInt32 operator -(SqlInt32 x) { return x.IsNull ? Null : new SqlInt32(-x.m_value); } ///[To be supplied.] ////// public static SqlInt32 operator ~(SqlInt32 x) { return x.IsNull ? Null : new SqlInt32(~x.m_value); } // Binary operators // Arithmetic operators ///[To be supplied.] ////// public static SqlInt32 operator +(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; int iResult = x.m_value + y.m_value; if (SameSignInt(x.m_value, y.m_value) && !SameSignInt(x.m_value, iResult)) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32(iResult); } ///[To be supplied.] ////// public static SqlInt32 operator -(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; int iResult = x.m_value - y.m_value; if (!SameSignInt(x.m_value, y.m_value) && SameSignInt(y.m_value, iResult)) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32(iResult); } ///[To be supplied.] ////// public static SqlInt32 operator *(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; long lResult = (long)x.m_value * (long)y.m_value; long lTemp = lResult & x_lBitNotIntMax; if (lTemp != 0 && lTemp != x_lBitNotIntMax) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)lResult); } ///[To be supplied.] ////// public static SqlInt32 operator /(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == x_iIntMin) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt32(x.m_value / y.m_value); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } ///[To be supplied.] ////// public static SqlInt32 operator %(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == x_iIntMin) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt32(x.m_value % y.m_value); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } // Bitwise operators ///[To be supplied.] ////// public static SqlInt32 operator &(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt32(x.m_value & y.m_value); } ///[To be supplied.] ////// public static SqlInt32 operator |(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt32(x.m_value | y.m_value); } ///[To be supplied.] ////// public static SqlInt32 operator ^(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt32(x.m_value ^ y.m_value); } // Implicit conversions // Implicit conversion from SqlBoolean to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlBoolean x) { return x.IsNull ? Null : new SqlInt32((int)x.ByteValue); } // Implicit conversion from SqlByte to SqlInt32 ///[To be supplied.] ////// public static implicit operator SqlInt32(SqlByte x) { return x.IsNull ? Null : new SqlInt32(x.Value); } // Implicit conversion from SqlInt16 to SqlInt32 ///[To be supplied.] ////// public static implicit operator SqlInt32(SqlInt16 x) { return x.IsNull ? Null : new SqlInt32(x.Value); } // Explicit conversions // Explicit conversion from SqlInt64 to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlInt64 x) { if (x.IsNull) return Null; long value = x.Value; if (value > (long)Int32.MaxValue || value < (long)Int32.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)value); } // Explicit conversion from SqlSingle to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlSingle x) { if (x.IsNull) return Null; float value = x.Value; if (value > (float)Int32.MaxValue || value < (float)Int32.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)value); } // Explicit conversion from SqlDouble to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlDouble x) { if (x.IsNull) return Null; double value = x.Value; if (value > (double)Int32.MaxValue || value < (double)Int32.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)value); } // Explicit conversion from SqlMoney to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlMoney x) { return x.IsNull ? Null : new SqlInt32(x.ToInt32()); } // Explicit conversion from SqlDecimal to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlDecimal x) { if (x.IsNull) return SqlInt32.Null; x.AdjustScale(-x.Scale, true); long ret = (long)x.m_data1; if (!x.IsPositive) ret = - ret; if (x.m_bLen > 1 || ret > (long)Int32.MaxValue || ret < (long)Int32.MinValue) throw new OverflowException(SQLResource.ConversionOverflowMessage); return new SqlInt32((int)ret); } // Explicit conversion from SqlString to SqlInt // Throws FormatException or OverflowException if necessary. ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlString x) { return x.IsNull ? SqlInt32.Null : new SqlInt32(Int32.Parse(x.Value, (IFormatProvider)null)); } // Utility functions private static bool SameSignInt(int x, int y) { return((x ^ y) & 0x80000000) == 0; } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value == y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlInt32 x, SqlInt32 y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value < y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value > y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value <= y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value >= y.m_value); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator ~ public static SqlInt32 OnesComplement(SqlInt32 x) { return ~x; } // Alternative method for operator + public static SqlInt32 Add(SqlInt32 x, SqlInt32 y) { return x + y; } // Alternative method for operator - public static SqlInt32 Subtract(SqlInt32 x, SqlInt32 y) { return x - y; } // Alternative method for operator * public static SqlInt32 Multiply(SqlInt32 x, SqlInt32 y) { return x * y; } // Alternative method for operator / public static SqlInt32 Divide(SqlInt32 x, SqlInt32 y) { return x / y; } // Alternative method for operator % public static SqlInt32 Mod(SqlInt32 x, SqlInt32 y) { return x % y; } public static SqlInt32 Modulus(SqlInt32 x, SqlInt32 y) { return x % y; } // Alternative method for operator & public static SqlInt32 BitwiseAnd(SqlInt32 x, SqlInt32 y) { return x & y; } // Alternative method for operator | public static SqlInt32 BitwiseOr(SqlInt32 x, SqlInt32 y) { return x | y; } // Alternative method for operator ^ public static SqlInt32 Xor(SqlInt32 x, SqlInt32 y) { return x ^ y; } // Alternative method for operator == public static SqlBoolean Equals(SqlInt32 x, SqlInt32 y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlInt32 x, SqlInt32 y) { return (x != y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlInt32 x, SqlInt32 y) { return (x < y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlInt32 x, SqlInt32 y) { return (x > y); } // Alternative method for operator <= public static SqlBoolean LessThanOrEqual(SqlInt32 x, SqlInt32 y) { return (x <= y); } // Alternative method for operator >= public static SqlBoolean GreaterThanOrEqual(SqlInt32 x, SqlInt32 y) { return (x >= y); } // Alternative method for conversions. public SqlBoolean ToSqlBoolean() { return (SqlBoolean)this; } public SqlByte ToSqlByte() { return (SqlByte)this; } public SqlDouble ToSqlDouble() { return (SqlDouble)this; } public SqlInt16 ToSqlInt16() { return (SqlInt16)this; } public SqlInt64 ToSqlInt64() { return (SqlInt64)this; } public SqlMoney ToSqlMoney() { return (SqlMoney)this; } public SqlDecimal ToSqlDecimal() { return (SqlDecimal)this; } public SqlSingle ToSqlSingle() { return (SqlSingle)this; } public SqlString ToSqlString() { return (SqlString)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlInt32) { SqlInt32 i = (SqlInt32)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlInt32)); } public int CompareTo(SqlInt32 value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this < value) return -1; if (this > value) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlInt32)) { return false; } SqlInt32 i = (SqlInt32)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { m_fNotNull = false; } else { m_value = XmlConvert.ToInt32(reader.ReadElementString()); m_fNotNull = true; } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(XmlConvert.ToString(m_value)); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("int", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlInt32 Null = new SqlInt32(true); ///[To be supplied.] ////// public static readonly SqlInt32 Zero = new SqlInt32(0); ///[To be supplied.] ////// public static readonly SqlInt32 MinValue = new SqlInt32(Int32.MinValue); ///[To be supplied.] ////// public static readonly SqlInt32 MaxValue = new SqlInt32(Int32.MaxValue); } // SqlInt32 } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlInt32.cs // // Create by: JunFang // // Purpose: Implementation of SqlInt32 which is equivalent to // data type "int" in SQL Server // // Notes: // // History: // // 09/17/99 JunFang Created and implemented as first drop. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Runtime.InteropServices; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [StructLayout(LayoutKind.Sequential)] [XmlSchemaProvider("GetXsdType")] public struct SqlInt32 : INullable, IComparable, IXmlSerializable { private bool m_fNotNull; // false if null, the default ctor (plain 0) will make it Null private int m_value; private static readonly long x_iIntMin = Int32.MinValue; // minimum (signed) int value private static readonly long x_lBitNotIntMax = ~(long)(Int32.MaxValue); // constructor // construct a Null private SqlInt32(bool fNull) { m_fNotNull = false; m_value = 0; } ////// Represents a 32-bit signed integer to be stored in /// or retrieved from a database. /// ////// public SqlInt32(int value) { m_value = value; m_fNotNull = true; } // INullable ///[To be supplied.] ////// public bool IsNull { get { return !m_fNotNull;} } // property: Value ///[To be supplied.] ////// public int Value { get { if (IsNull) throw new SqlNullValueException(); else return m_value; } } // Implicit conversion from int to SqlInt32 ///[To be supplied.] ////// public static implicit operator SqlInt32(int x) { return new SqlInt32(x); } // Explicit conversion from SqlInt32 to int. Throw exception if x is Null. ///[To be supplied.] ////// public static explicit operator int(SqlInt32 x) { return x.Value; } ///[To be supplied.] ////// public override String ToString() { return IsNull ? SQLResource.NullString : m_value.ToString((IFormatProvider)null); } ///[To be supplied.] ////// public static SqlInt32 Parse(String s) { if (s == SQLResource.NullString) return SqlInt32.Null; else return new SqlInt32(Int32.Parse(s, (IFormatProvider)null)); } // Unary operators ///[To be supplied.] ////// public static SqlInt32 operator -(SqlInt32 x) { return x.IsNull ? Null : new SqlInt32(-x.m_value); } ///[To be supplied.] ////// public static SqlInt32 operator ~(SqlInt32 x) { return x.IsNull ? Null : new SqlInt32(~x.m_value); } // Binary operators // Arithmetic operators ///[To be supplied.] ////// public static SqlInt32 operator +(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; int iResult = x.m_value + y.m_value; if (SameSignInt(x.m_value, y.m_value) && !SameSignInt(x.m_value, iResult)) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32(iResult); } ///[To be supplied.] ////// public static SqlInt32 operator -(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; int iResult = x.m_value - y.m_value; if (!SameSignInt(x.m_value, y.m_value) && SameSignInt(y.m_value, iResult)) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32(iResult); } ///[To be supplied.] ////// public static SqlInt32 operator *(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; long lResult = (long)x.m_value * (long)y.m_value; long lTemp = lResult & x_lBitNotIntMax; if (lTemp != 0 && lTemp != x_lBitNotIntMax) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)lResult); } ///[To be supplied.] ////// public static SqlInt32 operator /(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == x_iIntMin) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt32(x.m_value / y.m_value); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } ///[To be supplied.] ////// public static SqlInt32 operator %(SqlInt32 x, SqlInt32 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == x_iIntMin) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt32(x.m_value % y.m_value); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } // Bitwise operators ///[To be supplied.] ////// public static SqlInt32 operator &(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt32(x.m_value & y.m_value); } ///[To be supplied.] ////// public static SqlInt32 operator |(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt32(x.m_value | y.m_value); } ///[To be supplied.] ////// public static SqlInt32 operator ^(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt32(x.m_value ^ y.m_value); } // Implicit conversions // Implicit conversion from SqlBoolean to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlBoolean x) { return x.IsNull ? Null : new SqlInt32((int)x.ByteValue); } // Implicit conversion from SqlByte to SqlInt32 ///[To be supplied.] ////// public static implicit operator SqlInt32(SqlByte x) { return x.IsNull ? Null : new SqlInt32(x.Value); } // Implicit conversion from SqlInt16 to SqlInt32 ///[To be supplied.] ////// public static implicit operator SqlInt32(SqlInt16 x) { return x.IsNull ? Null : new SqlInt32(x.Value); } // Explicit conversions // Explicit conversion from SqlInt64 to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlInt64 x) { if (x.IsNull) return Null; long value = x.Value; if (value > (long)Int32.MaxValue || value < (long)Int32.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)value); } // Explicit conversion from SqlSingle to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlSingle x) { if (x.IsNull) return Null; float value = x.Value; if (value > (float)Int32.MaxValue || value < (float)Int32.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)value); } // Explicit conversion from SqlDouble to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlDouble x) { if (x.IsNull) return Null; double value = x.Value; if (value > (double)Int32.MaxValue || value < (double)Int32.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt32((int)value); } // Explicit conversion from SqlMoney to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlMoney x) { return x.IsNull ? Null : new SqlInt32(x.ToInt32()); } // Explicit conversion from SqlDecimal to SqlInt32 ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlDecimal x) { if (x.IsNull) return SqlInt32.Null; x.AdjustScale(-x.Scale, true); long ret = (long)x.m_data1; if (!x.IsPositive) ret = - ret; if (x.m_bLen > 1 || ret > (long)Int32.MaxValue || ret < (long)Int32.MinValue) throw new OverflowException(SQLResource.ConversionOverflowMessage); return new SqlInt32((int)ret); } // Explicit conversion from SqlString to SqlInt // Throws FormatException or OverflowException if necessary. ///[To be supplied.] ////// public static explicit operator SqlInt32(SqlString x) { return x.IsNull ? SqlInt32.Null : new SqlInt32(Int32.Parse(x.Value, (IFormatProvider)null)); } // Utility functions private static bool SameSignInt(int x, int y) { return((x ^ y) & 0x80000000) == 0; } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value == y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlInt32 x, SqlInt32 y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value < y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value > y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value <= y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlInt32 x, SqlInt32 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value >= y.m_value); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator ~ public static SqlInt32 OnesComplement(SqlInt32 x) { return ~x; } // Alternative method for operator + public static SqlInt32 Add(SqlInt32 x, SqlInt32 y) { return x + y; } // Alternative method for operator - public static SqlInt32 Subtract(SqlInt32 x, SqlInt32 y) { return x - y; } // Alternative method for operator * public static SqlInt32 Multiply(SqlInt32 x, SqlInt32 y) { return x * y; } // Alternative method for operator / public static SqlInt32 Divide(SqlInt32 x, SqlInt32 y) { return x / y; } // Alternative method for operator % public static SqlInt32 Mod(SqlInt32 x, SqlInt32 y) { return x % y; } public static SqlInt32 Modulus(SqlInt32 x, SqlInt32 y) { return x % y; } // Alternative method for operator & public static SqlInt32 BitwiseAnd(SqlInt32 x, SqlInt32 y) { return x & y; } // Alternative method for operator | public static SqlInt32 BitwiseOr(SqlInt32 x, SqlInt32 y) { return x | y; } // Alternative method for operator ^ public static SqlInt32 Xor(SqlInt32 x, SqlInt32 y) { return x ^ y; } // Alternative method for operator == public static SqlBoolean Equals(SqlInt32 x, SqlInt32 y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlInt32 x, SqlInt32 y) { return (x != y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlInt32 x, SqlInt32 y) { return (x < y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlInt32 x, SqlInt32 y) { return (x > y); } // Alternative method for operator <= public static SqlBoolean LessThanOrEqual(SqlInt32 x, SqlInt32 y) { return (x <= y); } // Alternative method for operator >= public static SqlBoolean GreaterThanOrEqual(SqlInt32 x, SqlInt32 y) { return (x >= y); } // Alternative method for conversions. public SqlBoolean ToSqlBoolean() { return (SqlBoolean)this; } public SqlByte ToSqlByte() { return (SqlByte)this; } public SqlDouble ToSqlDouble() { return (SqlDouble)this; } public SqlInt16 ToSqlInt16() { return (SqlInt16)this; } public SqlInt64 ToSqlInt64() { return (SqlInt64)this; } public SqlMoney ToSqlMoney() { return (SqlMoney)this; } public SqlDecimal ToSqlDecimal() { return (SqlDecimal)this; } public SqlSingle ToSqlSingle() { return (SqlSingle)this; } public SqlString ToSqlString() { return (SqlString)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlInt32) { SqlInt32 i = (SqlInt32)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlInt32)); } public int CompareTo(SqlInt32 value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this < value) return -1; if (this > value) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlInt32)) { return false; } SqlInt32 i = (SqlInt32)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { m_fNotNull = false; } else { m_value = XmlConvert.ToInt32(reader.ReadElementString()); m_fNotNull = true; } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(XmlConvert.ToString(m_value)); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("int", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlInt32 Null = new SqlInt32(true); ///[To be supplied.] ////// public static readonly SqlInt32 Zero = new SqlInt32(0); ///[To be supplied.] ////// public static readonly SqlInt32 MinValue = new SqlInt32(Int32.MinValue); ///[To be supplied.] ////// public static readonly SqlInt32 MaxValue = new SqlInt32(Int32.MaxValue); } // SqlInt32 } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
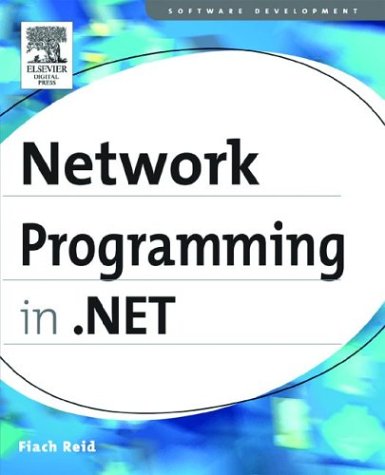
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateBindingExtensionConverter.cs
- ColorConverter.cs
- SqlLiftIndependentRowExpressions.cs
- XmlObjectSerializerWriteContext.cs
- CodeCastExpression.cs
- CodePrimitiveExpression.cs
- recordstate.cs
- DataColumnPropertyDescriptor.cs
- ZoomingMessageFilter.cs
- XmlBinaryReader.cs
- StreamReader.cs
- RootProfilePropertySettingsCollection.cs
- DBConnectionString.cs
- ProxyWebPartConnectionCollection.cs
- SystemGatewayIPAddressInformation.cs
- DateTimeFormatInfoScanner.cs
- ApplicationServiceHelper.cs
- InputLanguageManager.cs
- CheckBoxBaseAdapter.cs
- EntityDataSourceChangingEventArgs.cs
- DataTableTypeConverter.cs
- ComponentTray.cs
- XmlUtilWriter.cs
- SecureStringHasher.cs
- CodeIdentifiers.cs
- WebScriptEndpointElement.cs
- DirectoryGroupQuery.cs
- QuaternionIndependentAnimationStorage.cs
- Transform3D.cs
- CounterSampleCalculator.cs
- PersonalizationProviderHelper.cs
- Point3DCollection.cs
- PersonalizationState.cs
- MenuItemAutomationPeer.cs
- SerializableTypeCodeDomSerializer.cs
- Calendar.cs
- StrongNameKeyPair.cs
- XmlReflectionImporter.cs
- Parallel.cs
- PipeStream.cs
- CheckBoxList.cs
- EntityModelBuildProvider.cs
- RequestValidator.cs
- SchemaNamespaceManager.cs
- OleDbParameterCollection.cs
- SafeNativeMethods.cs
- UpdatePanelTrigger.cs
- ToolStripSeparatorRenderEventArgs.cs
- ListViewItem.cs
- HttpFileCollection.cs
- TimeIntervalCollection.cs
- InternalConfigSettingsFactory.cs
- ISAPIRuntime.cs
- ExpandCollapseProviderWrapper.cs
- ResourceWriter.cs
- ArrayEditor.cs
- IODescriptionAttribute.cs
- GridViewSortEventArgs.cs
- EntityCommand.cs
- TaskbarItemInfo.cs
- QueryResultOp.cs
- ItemDragEvent.cs
- JsonUriDataContract.cs
- EditorPart.cs
- RightsManagementEncryptedStream.cs
- AsyncPostBackTrigger.cs
- TextWriter.cs
- ScrollProviderWrapper.cs
- TraceListeners.cs
- Point3DConverter.cs
- ImpersonationContext.cs
- BaseAsyncResult.cs
- TagPrefixInfo.cs
- GetPageNumberCompletedEventArgs.cs
- TextTreeDeleteContentUndoUnit.cs
- SiteMapNode.cs
- DeleteIndexBinder.cs
- SmuggledIUnknown.cs
- ColorContext.cs
- StylusPointProperty.cs
- ValuePatternIdentifiers.cs
- AsyncOperationManager.cs
- ScriptResourceHandler.cs
- TextStore.cs
- CodeNamespaceCollection.cs
- BasicExpressionVisitor.cs
- HtmlElement.cs
- WebPartCollection.cs
- ToolStripArrowRenderEventArgs.cs
- ModifiableIteratorCollection.cs
- HMACRIPEMD160.cs
- SQLDecimalStorage.cs
- OAVariantLib.cs
- AuditLevel.cs
- DirectoryNotFoundException.cs
- RootBrowserWindow.cs
- SettingsPropertyValueCollection.cs
- CompiledRegexRunnerFactory.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- XmlSchemaException.cs