Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Diagnostics / TraceListeners.cs / 1 / TraceListeners.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Diagnostics { using System; using System.Collections; using Microsoft.Win32; ////// public class TraceListenerCollection : IList { ArrayList list; internal TraceListenerCollection() { list = new ArrayList(1); } ///Provides a thread-safe list of ///. A thread-safe list is synchronized. /// public TraceListener this[int i] { get { return (TraceListener)list[i]; } set { InitializeListener(value); list[i] = value; } } ///Gets or sets the ///at /// the specified index. /// public TraceListener this[string name] { get { foreach (TraceListener listener in this) { if (listener.Name == name) return listener; } return null; } } ///Gets the first ///in the list with the specified name. /// public int Count { get { return list.Count; } } ////// Gets the number of listeners in the list. /// ////// public int Add(TraceListener listener) { InitializeListener(listener); lock (TraceInternal.critSec) { return list.Add(listener); } } ///Adds a ///to the list. /// public void AddRange(TraceListener[] value) { if (value == null) { throw new ArgumentNullException("value"); } for (int i = 0; ((i) < (value.Length)); i = ((i) + (1))) { this.Add(value[i]); } } ///[To be supplied.] ////// public void AddRange(TraceListenerCollection value) { if (value == null) { throw new ArgumentNullException("value"); } int currentCount = value.Count; for (int i = 0; i < currentCount; i = ((i) + (1))) { this.Add(value[i]); } } ///[To be supplied.] ////// public void Clear() { list = new ArrayList(); } ////// Clears all the listeners from the /// list. /// ////// public bool Contains(TraceListener listener) { return ((IList)this).Contains(listener); } ///Checks whether the list contains the specified /// listener. ////// public void CopyTo(TraceListener[] listeners, int index) { ((ICollection)this).CopyTo((Array) listeners, index); } ///Copies a section of the current ///list to the specified array at the specified /// index. /// public IEnumerator GetEnumerator() { return list.GetEnumerator(); } internal void InitializeListener(TraceListener listener) { if (listener == null) throw new ArgumentNullException("listener"); listener.IndentSize = TraceInternal.IndentSize; listener.IndentLevel = TraceInternal.IndentLevel; } ////// Gets an enumerator for this list. /// ////// public int IndexOf(TraceListener listener) { return ((IList)this).IndexOf(listener); } ///Gets the index of the specified listener. ////// public void Insert(int index, TraceListener listener) { InitializeListener(listener); lock (TraceInternal.critSec) { list.Insert(index, listener); } } ///Inserts the listener at the specified index. ////// public void Remove(TraceListener listener) { ((IList)this).Remove(listener); } ////// Removes the specified instance of the ///class from the list. /// /// public void Remove(string name) { TraceListener listener = this[name]; if (listener != null) ((IList)this).Remove(listener); } ///Removes the first listener in the list that has the /// specified name. ////// public void RemoveAt(int index) { lock (TraceInternal.critSec) { list.RemoveAt(index); } } ///Removes the ///at the specified index. object IList.this[int index] { get { return list[index]; } set { TraceListener listener = value as TraceListener; if (listener == null) throw new ArgumentException(SR.GetString(SR.MustAddListener),"value"); InitializeListener(listener); list[index] = listener; } } /// bool IList.IsReadOnly { get { return false; } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object value) { TraceListener listener = value as TraceListener; if (listener == null) throw new ArgumentException(SR.GetString(SR.MustAddListener),"value"); InitializeListener(listener); lock (TraceInternal.critSec) { return list.Add(value); } } /// bool IList.Contains(object value) { return list.Contains(value); } /// int IList.IndexOf(object value) { return list.IndexOf(value); } /// void IList.Insert(int index, object value) { TraceListener listener = value as TraceListener; if (listener == null) throw new ArgumentException(SR.GetString(SR.MustAddListener),"value"); InitializeListener(listener); lock (TraceInternal.critSec) { list.Insert(index, value); } } /// void IList.Remove(object value) { lock (TraceInternal.critSec) { list.Remove(value); } } /// object ICollection.SyncRoot { get { return this; } } /// bool ICollection.IsSynchronized { get { return true; } } /// void ICollection.CopyTo(Array array, int index) { lock (TraceInternal.critSec) { list.CopyTo(array, index); } } } }
Link Menu
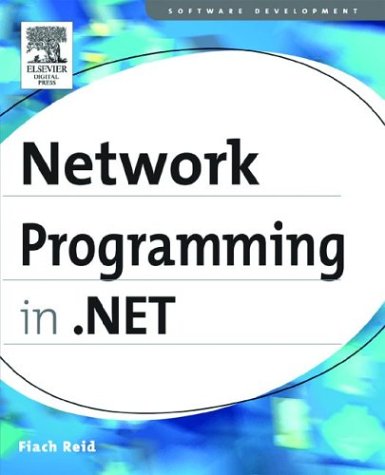
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerAttribute.cs
- KerberosSecurityTokenAuthenticator.cs
- FunctionParameter.cs
- FigureParagraph.cs
- MessageQueueException.cs
- StrokeNode.cs
- AdapterDictionary.cs
- ResumeStoryboard.cs
- IriParsingElement.cs
- TcpChannelListener.cs
- TraceAsyncResult.cs
- Preprocessor.cs
- FixedDocumentPaginator.cs
- MissingSatelliteAssemblyException.cs
- SecurityTokenProviderContainer.cs
- _SingleItemRequestCache.cs
- NetworkStream.cs
- WindowsAuthenticationEventArgs.cs
- HttpCapabilitiesSectionHandler.cs
- XmlSchemaNotation.cs
- SqlClientMetaDataCollectionNames.cs
- PolicyException.cs
- _HelperAsyncResults.cs
- InkCollectionBehavior.cs
- PTManager.cs
- DrawingGroup.cs
- EntityModelSchemaGenerator.cs
- BindingExpressionUncommonField.cs
- ToolStripStatusLabel.cs
- TargetControlTypeAttribute.cs
- TagPrefixInfo.cs
- HitTestWithPointDrawingContextWalker.cs
- NetSectionGroup.cs
- InternalMappingException.cs
- StateManagedCollection.cs
- FileFormatException.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- Knowncolors.cs
- PropertyGeneratedEventArgs.cs
- FileChangeNotifier.cs
- AvTraceDetails.cs
- PseudoWebRequest.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- SystemIPAddressInformation.cs
- HtmlToClrEventProxy.cs
- WindowsListViewItemStartMenu.cs
- TabControlCancelEvent.cs
- FileStream.cs
- XPathNodeList.cs
- Triplet.cs
- SecurityCriticalDataForSet.cs
- Site.cs
- EntityConnectionStringBuilder.cs
- CompositeControl.cs
- WorkflowPersistenceContext.cs
- ClientSideQueueItem.cs
- HtmlInputFile.cs
- AuthenticatedStream.cs
- ProfileSection.cs
- DynamicMethod.cs
- JsonWriter.cs
- DbProviderManifest.cs
- securestring.cs
- TableDetailsRow.cs
- CookieParameter.cs
- GridSplitter.cs
- XmlSerializerOperationBehavior.cs
- GeneralTransform3D.cs
- PropertyItem.cs
- PlatformCulture.cs
- DeclarativeCatalogPart.cs
- BooleanFunctions.cs
- VisualTarget.cs
- CompilerGeneratedAttribute.cs
- XmlSchemaSimpleTypeUnion.cs
- KeyPressEvent.cs
- BitmapDecoder.cs
- NumberSubstitution.cs
- __Filters.cs
- WorkflowItemPresenter.cs
- Registry.cs
- HtmlFormWrapper.cs
- InputMethodStateTypeInfo.cs
- TreeNodeCollection.cs
- ScriptModule.cs
- SQlBooleanStorage.cs
- DynamicILGenerator.cs
- OdbcCommand.cs
- ToolStripDesigner.cs
- WeakHashtable.cs
- TreeChangeInfo.cs
- DataSetUtil.cs
- Bold.cs
- UnitySerializationHolder.cs
- CategoryAttribute.cs
- OleDbSchemaGuid.cs
- OdbcConnectionStringbuilder.cs
- MergeFilterQuery.cs
- ApplicationTrust.cs
- PathSegment.cs