Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / WSIdentityFaultException.cs / 1 / WSIdentityFaultException.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Globalization; using System.Xml; using System.Text; using System.Runtime.Serialization; using Microsoft.InfoCards.Diagnostics; using System.ServiceModel; using System.ServiceModel.Channels; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Wraps a fault message returned from the sts in an appropriate infocard exception. // internal class WSIdentityFaultException :InfoCardBaseException { // // Default exception that we return if we do not understand the fault. // const int HRESULT = (int)EventCode.E_ICARD_TRUSTEXCHANGE; // // Summary: // Throws an infocardBase derived exception if the incoming message is a fault. // Notes: This logically lives in the diagnostics classes, but cannot be moved there // due to the sharing of the code across client and service since the client does // not reference System.ServiceModel. // public static void ThrowIfFaultMessage( Message message, CultureInfo displayCulture ) { // // const int MAX_FAULT_BUFFER = 2 * 1000 * 1000; if( !message.IsFault ) { return; } MessageFault fault = MessageFault.CreateFault( message, MAX_FAULT_BUFFER ); FaultException exception = FaultException.CreateFault( fault ); // // If we have a fault detail in the message then log that also // otherwise just log the actual fault reason text.. // string description = exception.Message; string extendedMessage = string.Empty; if( null != fault.Reason ) { // // GetMatchingTranslation returns the first reason from the collection in case a match fails. // FaultReasonText faultReason = fault.Reason.GetMatchingTranslation( displayCulture ); if( null != faultReason ) { extendedMessage = faultReason.Text; } } if( fault.HasDetail ) { using( XmlReader reader = fault.GetReaderAtDetailContents() ) { if( reader.Read() ) { description = String.Format( System.Globalization.CultureInfo.CurrentUICulture, SR.GetString( SR.FaultMessageFormat ), exception.Message, reader.ReadOuterXml() ); } } } // // We don't save fe, only use it to switch the hresult. // throw IDT.ThrowHelperErrorWithNoLogging( new WSIdentityFaultException( description, extendedMessage, exception ) ); } public WSIdentityFaultException() : base( HRESULT ) { } public WSIdentityFaultException( string message ) : base( HRESULT, message ) { } public WSIdentityFaultException( string message, string extendedMessage, Exception inner ) : base( HRESULT, message, extendedMessage ) { if( inner is FaultException ) { MapFaultException( inner as FaultException ); } } protected WSIdentityFaultException( SerializationInfo si, StreamingContext sc ) : base( HRESULT, si, sc ) { } // // Summary: // Maps an incoming fault exception to one of the recognised infocard exceptions, // or to a generic exception if it is not one of the set we recognise. // private void MapFaultException( FaultException fe ) { if( null != fe && null != fe.Code && null != fe.Code.SubCode && ( fe.Code.SubCode.Namespace == XmlNames.WSIdentity.FaultNamespace ) ) { switch( fe.Code.SubCode.Name ) { case XmlNames.WSIdentity.FaultRefreshRequired: HResult = (int)EventCode.E_ICARD_REFRESH_REQUIRED; break; case XmlNames.WSIdentity.FaultMissingAppliesTo: HResult = (int)EventCode.E_ICARD_MISSING_APPLIESTO; break; case XmlNames.WSIdentity.FaultInvalidProofKey: HResult = (int)EventCode.E_ICARD_INVALID_PROOF_KEY; break; case XmlNames.WSIdentity.FaultUnknownReference: HResult = (int)EventCode.E_ICARD_UNKNOWN_REFERENCE; break; case XmlNames.WSIdentity.FaultRequiredClaims: HResult = (int)EventCode.E_ICARD_FAILED_REQUIRED_CLAIMS; break; default: break; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
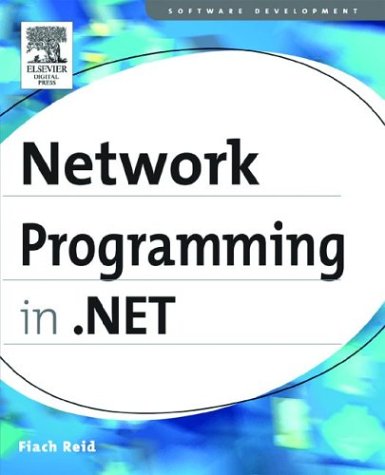
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferedReadStream.cs
- BaseProcessor.cs
- SafeNativeMethods.cs
- HWStack.cs
- MenuAdapter.cs
- MessageQueue.cs
- NotSupportedException.cs
- InvokeSchedule.cs
- ImageResources.Designer.cs
- SQLInt32Storage.cs
- Parameter.cs
- SchemaTypeEmitter.cs
- SqlDeflator.cs
- CompositeDataBoundControl.cs
- TypeSystemHelpers.cs
- _ConnectionGroup.cs
- MultiByteCodec.cs
- PagedDataSource.cs
- RedistVersionInfo.cs
- UniqueEventHelper.cs
- ModelUtilities.cs
- RowToFieldTransformer.cs
- NamespaceCollection.cs
- JsonCollectionDataContract.cs
- IntegerCollectionEditor.cs
- DesignerGeometryHelper.cs
- ValidationErrorInfo.cs
- HexParser.cs
- ButtonBase.cs
- WinEventQueueItem.cs
- TreeBuilderBamlTranslator.cs
- DateTimeValueSerializerContext.cs
- DefaultObjectMappingItemCollection.cs
- HMACSHA256.cs
- DataGridPagerStyle.cs
- Types.cs
- webeventbuffer.cs
- FixedBufferAttribute.cs
- PngBitmapEncoder.cs
- SQLResource.cs
- BinHexDecoder.cs
- UpdatePanelTriggerCollection.cs
- BaseCodeDomTreeGenerator.cs
- DetailsViewPageEventArgs.cs
- DataBoundLiteralControl.cs
- BadImageFormatException.cs
- VersionConverter.cs
- SchemaElementLookUpTableEnumerator.cs
- SerialErrors.cs
- DocumentViewerHelper.cs
- PopupEventArgs.cs
- EntityDataSourceDataSelection.cs
- AudioFormatConverter.cs
- LogicalTreeHelper.cs
- FormatException.cs
- WebResponse.cs
- CommandField.cs
- NavigationHelper.cs
- Verify.cs
- SettingsProviderCollection.cs
- StrokeNodeOperations.cs
- ReachVisualSerializerAsync.cs
- PageAdapter.cs
- MenuItemBinding.cs
- FormViewRow.cs
- X509CertificateChain.cs
- OptimalTextSource.cs
- BasicCellRelation.cs
- DefaultValueAttribute.cs
- CatalogPartCollection.cs
- Operators.cs
- AttributeSetAction.cs
- XmlByteStreamReader.cs
- XslCompiledTransform.cs
- Substitution.cs
- FileAuthorizationModule.cs
- GenericEnumerator.cs
- StateItem.cs
- DbMetaDataCollectionNames.cs
- CodeDomConfigurationHandler.cs
- ContravarianceAdapter.cs
- BitmapData.cs
- FrameworkReadOnlyPropertyMetadata.cs
- RTLAwareMessageBox.cs
- DoubleAnimationBase.cs
- ContentFilePart.cs
- SqlEnums.cs
- EnumerableCollectionView.cs
- PageContentAsyncResult.cs
- ValidatorCompatibilityHelper.cs
- EntryIndex.cs
- DropDownList.cs
- DbProviderServices.cs
- MenuBindingsEditorForm.cs
- AsymmetricKeyExchangeFormatter.cs
- TagPrefixInfo.cs
- PrinterUnitConvert.cs
- FormViewRow.cs
- WindowsRegion.cs
- HeaderCollection.cs