Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / BufferAllocator.cs / 1 / BufferAllocator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Buffer Allocators with recycling * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web { using System.Collections; using System.IO; using System.Globalization; using System.Web.Util; ////////////////////////////////////////////////////////////////////////////// // Generic buffer recycling /* * Base class for allocator doing buffer recycling */ internal abstract class BufferAllocator { private int _maxFree; private int _numFree; private Stack _buffers; private static int s_ProcsFudgeFactor; static BufferAllocator() { s_ProcsFudgeFactor = SystemInfo.GetNumProcessCPUs(); if (s_ProcsFudgeFactor < 1) s_ProcsFudgeFactor = 1; if (s_ProcsFudgeFactor > 4) s_ProcsFudgeFactor = 4; } internal BufferAllocator(int maxFree) { _buffers = new Stack(); _numFree = 0; _maxFree = maxFree * s_ProcsFudgeFactor; } internal /*public*/ Object GetBuffer() { Object buffer = null; if (_numFree > 0) { lock(this) { if (_numFree > 0) { buffer = _buffers.Pop(); _numFree--; } } } if (buffer == null) buffer = AllocBuffer(); return buffer; } internal void ReuseBuffer(Object buffer) { if (_numFree < _maxFree) { lock(this) { if (_numFree < _maxFree) { _buffers.Push(buffer); _numFree++; } } } } /* * To be implemented by a derived class */ abstract protected Object AllocBuffer(); } /* * Concrete allocator class for ubyte[] buffer recycling */ internal class UbyteBufferAllocator : BufferAllocator { private int _bufferSize; internal UbyteBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new byte[_bufferSize]; } } /* * Concrete allocator class for char[] buffer recycling */ internal class CharBufferAllocator : BufferAllocator { private int _bufferSize; internal CharBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new char[_bufferSize]; } } /* * Concrete allocator class for int[] buffer recycling */ internal class IntegerArrayAllocator : BufferAllocator { private int _arraySize; internal IntegerArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new int[_arraySize]; } } /* * Concrete allocator class for IntPtr[] buffer recycling */ internal class IntPtrArrayAllocator : BufferAllocator { private int _arraySize; internal IntPtrArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new IntPtr[_arraySize]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Buffer Allocators with recycling * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web { using System.Collections; using System.IO; using System.Globalization; using System.Web.Util; ////////////////////////////////////////////////////////////////////////////// // Generic buffer recycling /* * Base class for allocator doing buffer recycling */ internal abstract class BufferAllocator { private int _maxFree; private int _numFree; private Stack _buffers; private static int s_ProcsFudgeFactor; static BufferAllocator() { s_ProcsFudgeFactor = SystemInfo.GetNumProcessCPUs(); if (s_ProcsFudgeFactor < 1) s_ProcsFudgeFactor = 1; if (s_ProcsFudgeFactor > 4) s_ProcsFudgeFactor = 4; } internal BufferAllocator(int maxFree) { _buffers = new Stack(); _numFree = 0; _maxFree = maxFree * s_ProcsFudgeFactor; } internal /*public*/ Object GetBuffer() { Object buffer = null; if (_numFree > 0) { lock(this) { if (_numFree > 0) { buffer = _buffers.Pop(); _numFree--; } } } if (buffer == null) buffer = AllocBuffer(); return buffer; } internal void ReuseBuffer(Object buffer) { if (_numFree < _maxFree) { lock(this) { if (_numFree < _maxFree) { _buffers.Push(buffer); _numFree++; } } } } /* * To be implemented by a derived class */ abstract protected Object AllocBuffer(); } /* * Concrete allocator class for ubyte[] buffer recycling */ internal class UbyteBufferAllocator : BufferAllocator { private int _bufferSize; internal UbyteBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new byte[_bufferSize]; } } /* * Concrete allocator class for char[] buffer recycling */ internal class CharBufferAllocator : BufferAllocator { private int _bufferSize; internal CharBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new char[_bufferSize]; } } /* * Concrete allocator class for int[] buffer recycling */ internal class IntegerArrayAllocator : BufferAllocator { private int _arraySize; internal IntegerArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new int[_arraySize]; } } /* * Concrete allocator class for IntPtr[] buffer recycling */ internal class IntPtrArrayAllocator : BufferAllocator { private int _arraySize; internal IntPtrArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new IntPtr[_arraySize]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
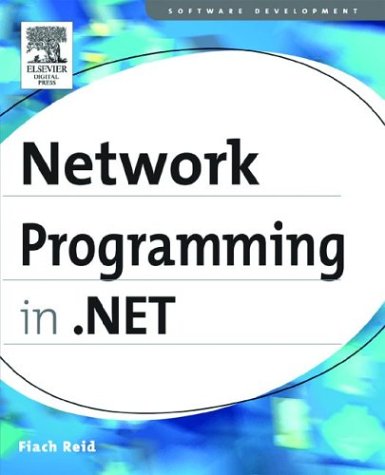
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MouseEvent.cs
- GetLastErrorDetailsRequest.cs
- AdjustableArrowCap.cs
- PageThemeCodeDomTreeGenerator.cs
- AggregateNode.cs
- HostingEnvironmentSection.cs
- PartialCachingAttribute.cs
- WebPartCatalogAddVerb.cs
- ContentDisposition.cs
- HealthMonitoringSectionHelper.cs
- SrgsItemList.cs
- PropertyChangingEventArgs.cs
- SchemaLookupTable.cs
- ComponentManagerBroker.cs
- RegisteredDisposeScript.cs
- TraceLog.cs
- EditCommandColumn.cs
- FreezableOperations.cs
- _NestedMultipleAsyncResult.cs
- TypeSystemProvider.cs
- TextEditorDragDrop.cs
- Logging.cs
- SharedUtils.cs
- XPathArrayIterator.cs
- WebReferenceCollection.cs
- _FtpControlStream.cs
- EventSourceCreationData.cs
- LeaseManager.cs
- Parser.cs
- TaskResultSetter.cs
- IPEndPoint.cs
- ClientSettingsProvider.cs
- TdsValueSetter.cs
- DescendantBaseQuery.cs
- GridViewAutomationPeer.cs
- EdmEntityTypeAttribute.cs
- IsolationInterop.cs
- ThreadNeutralSemaphore.cs
- _AuthenticationState.cs
- XmlSchemaAnyAttribute.cs
- PointLightBase.cs
- COM2IPerPropertyBrowsingHandler.cs
- NetTcpBinding.cs
- XPathBuilder.cs
- XmlSchemaComplexType.cs
- EraserBehavior.cs
- DataGridViewComponentPropertyGridSite.cs
- Journaling.cs
- SmtpAuthenticationManager.cs
- PhysicalOps.cs
- ToolStripLocationCancelEventArgs.cs
- SpellerHighlightLayer.cs
- IProducerConsumerCollection.cs
- TextElementCollection.cs
- ProfileProvider.cs
- PropertyCollection.cs
- SystemDiagnosticsSection.cs
- DataViewListener.cs
- InputBindingCollection.cs
- InkCanvasSelection.cs
- Function.cs
- CompoundFileReference.cs
- HttpListenerRequestUriBuilder.cs
- FormatConvertedBitmap.cs
- HttpConfigurationSystem.cs
- PageThemeBuildProvider.cs
- HtmlTableCellCollection.cs
- DecoderBestFitFallback.cs
- HttpPostProtocolImporter.cs
- shaperfactoryquerycacheentry.cs
- Annotation.cs
- XmlMessageFormatter.cs
- ControlUtil.cs
- TranslateTransform.cs
- BinHexDecoder.cs
- SQLInt32Storage.cs
- SimpleApplicationHost.cs
- List.cs
- RoleManagerEventArgs.cs
- webeventbuffer.cs
- Int64KeyFrameCollection.cs
- CharUnicodeInfo.cs
- DbConnectionPoolOptions.cs
- CursorEditor.cs
- SiteMapDataSource.cs
- MergeLocalizationDirectives.cs
- filewebresponse.cs
- StorageEntitySetMapping.cs
- CreateUserErrorEventArgs.cs
- BitmapFrame.cs
- BindingNavigator.cs
- XmlBaseWriter.cs
- JoinElimination.cs
- PolygonHotSpot.cs
- DirtyTextRange.cs
- WorkflowInstanceProvider.cs
- CacheOutputQuery.cs
- DataStreams.cs
- FontSource.cs
- AssociatedControlConverter.cs