Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / AesManaged.cs / 1305376 / AesManaged.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; #if !SILVERLIGHT using System.Diagnostics.Contracts; #endif // !SILVERLIGHT namespace System.Security.Cryptography { ////// Managed implementation of the AES algorithm. AES is esentially Rijndael with a fixed block size /// and iteration count, so we just wrap the RijndaelManaged class and allow only 128 bit blocks to /// be used. /// public sealed class AesManaged : Aes { private RijndaelManaged m_rijndael; public AesManaged() { #if !SILVERLIGHT Contract.Ensures(m_rijndael != null); if (CryptoConfig.AllowOnlyFipsAlgorithms) { throw new InvalidOperationException(SR.GetString(SR.Cryptography_NonCompliantFIPSAlgorithm)); } #endif // !SILVERLIGHT m_rijndael = new RijndaelManaged(); m_rijndael.BlockSize = BlockSize; m_rijndael.KeySize = KeySize; } #if !SILVERLIGHT public override int FeedbackSize { get { return m_rijndael.FeedbackSize; } set { m_rijndael.FeedbackSize = value; } } #endif // !SILVERLIGHT public override byte[] IV { get { return m_rijndael.IV; } set { m_rijndael.IV = value; } } public override byte[] Key { get { return m_rijndael.Key; } set { m_rijndael.Key = value; } } public override int KeySize { get { return m_rijndael.KeySize; } set { m_rijndael.KeySize = value; } } #if !SILVERLIGHT public override CipherMode Mode { get { return m_rijndael.Mode; } set { Contract.Ensures(m_rijndael.Mode != CipherMode.CFB && m_rijndael.Mode != CipherMode.OFB); // RijndaelManaged will implicitly change the block size of an algorithm to match the number // of feedback bits being used. Since AES requires a block size of 128 bits, we cannot allow // the user to use the feedback modes, as this will end up breaking that invarient. if (value == CipherMode.CFB || value == CipherMode.OFB) { throw new CryptographicException(SR.GetString(SR.Cryptography_InvalidCipherMode)); } m_rijndael.Mode = value; } } public override PaddingMode Padding { get { return m_rijndael.Padding; } set { m_rijndael.Padding = value; } } #endif // !SILVERLIGHT public override ICryptoTransform CreateDecryptor() { return m_rijndael.CreateDecryptor(); } public override ICryptoTransform CreateDecryptor(byte[] key, byte[] iv) { if (key == null) { throw new ArgumentNullException("key"); } #if !SILVERLIGHT if (!ValidKeySize(key.Length * 8)) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidKeySize), "key"); } if (iv != null && iv.Length * 8 != BlockSizeValue) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidIVSize), "iv"); } #endif return m_rijndael.CreateDecryptor(key, iv); } public override ICryptoTransform CreateEncryptor() { return m_rijndael.CreateEncryptor(); } public override ICryptoTransform CreateEncryptor(byte[] key, byte[] iv) { if (key == null) { throw new ArgumentNullException("key"); } #if !SILVERLIGHT if (!ValidKeySize(key.Length * 8)) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidKeySize), "key"); } if (iv != null && iv.Length * 8 != BlockSizeValue) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidIVSize), "iv"); } #endif // SILVERLIGHT return m_rijndael.CreateEncryptor(key, iv); } protected override void Dispose(bool disposing) { try { if (disposing) { (m_rijndael as IDisposable).Dispose(); } } finally { base.Dispose(disposing); } } public override void GenerateIV() { m_rijndael.GenerateIV(); } public override void GenerateKey() { m_rijndael.GenerateKey(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; #if !SILVERLIGHT using System.Diagnostics.Contracts; #endif // !SILVERLIGHT namespace System.Security.Cryptography { ////// Managed implementation of the AES algorithm. AES is esentially Rijndael with a fixed block size /// and iteration count, so we just wrap the RijndaelManaged class and allow only 128 bit blocks to /// be used. /// public sealed class AesManaged : Aes { private RijndaelManaged m_rijndael; public AesManaged() { #if !SILVERLIGHT Contract.Ensures(m_rijndael != null); if (CryptoConfig.AllowOnlyFipsAlgorithms) { throw new InvalidOperationException(SR.GetString(SR.Cryptography_NonCompliantFIPSAlgorithm)); } #endif // !SILVERLIGHT m_rijndael = new RijndaelManaged(); m_rijndael.BlockSize = BlockSize; m_rijndael.KeySize = KeySize; } #if !SILVERLIGHT public override int FeedbackSize { get { return m_rijndael.FeedbackSize; } set { m_rijndael.FeedbackSize = value; } } #endif // !SILVERLIGHT public override byte[] IV { get { return m_rijndael.IV; } set { m_rijndael.IV = value; } } public override byte[] Key { get { return m_rijndael.Key; } set { m_rijndael.Key = value; } } public override int KeySize { get { return m_rijndael.KeySize; } set { m_rijndael.KeySize = value; } } #if !SILVERLIGHT public override CipherMode Mode { get { return m_rijndael.Mode; } set { Contract.Ensures(m_rijndael.Mode != CipherMode.CFB && m_rijndael.Mode != CipherMode.OFB); // RijndaelManaged will implicitly change the block size of an algorithm to match the number // of feedback bits being used. Since AES requires a block size of 128 bits, we cannot allow // the user to use the feedback modes, as this will end up breaking that invarient. if (value == CipherMode.CFB || value == CipherMode.OFB) { throw new CryptographicException(SR.GetString(SR.Cryptography_InvalidCipherMode)); } m_rijndael.Mode = value; } } public override PaddingMode Padding { get { return m_rijndael.Padding; } set { m_rijndael.Padding = value; } } #endif // !SILVERLIGHT public override ICryptoTransform CreateDecryptor() { return m_rijndael.CreateDecryptor(); } public override ICryptoTransform CreateDecryptor(byte[] key, byte[] iv) { if (key == null) { throw new ArgumentNullException("key"); } #if !SILVERLIGHT if (!ValidKeySize(key.Length * 8)) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidKeySize), "key"); } if (iv != null && iv.Length * 8 != BlockSizeValue) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidIVSize), "iv"); } #endif return m_rijndael.CreateDecryptor(key, iv); } public override ICryptoTransform CreateEncryptor() { return m_rijndael.CreateEncryptor(); } public override ICryptoTransform CreateEncryptor(byte[] key, byte[] iv) { if (key == null) { throw new ArgumentNullException("key"); } #if !SILVERLIGHT if (!ValidKeySize(key.Length * 8)) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidKeySize), "key"); } if (iv != null && iv.Length * 8 != BlockSizeValue) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidIVSize), "iv"); } #endif // SILVERLIGHT return m_rijndael.CreateEncryptor(key, iv); } protected override void Dispose(bool disposing) { try { if (disposing) { (m_rijndael as IDisposable).Dispose(); } } finally { base.Dispose(disposing); } } public override void GenerateIV() { m_rijndael.GenerateIV(); } public override void GenerateKey() { m_rijndael.GenerateKey(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
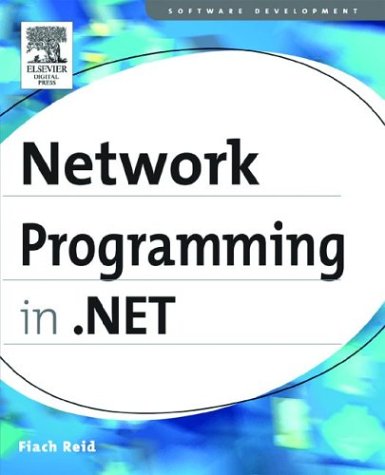
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlMessageFormatter.cs
- IisTraceListener.cs
- AuthorizationRule.cs
- WebPartConnectionsConfigureVerb.cs
- PartialCachingAttribute.cs
- AutoGeneratedField.cs
- TypeDependencyAttribute.cs
- QueryOutputWriter.cs
- TileModeValidation.cs
- ValidationErrorInfo.cs
- OleDbConnection.cs
- ToolStripPanelRenderEventArgs.cs
- TimelineClockCollection.cs
- DataGridViewComboBoxEditingControl.cs
- XmlSchemaImport.cs
- RawTextInputReport.cs
- ErrorWrapper.cs
- DropDownList.cs
- ObjectQueryProvider.cs
- RevocationPoint.cs
- Int32KeyFrameCollection.cs
- RectAnimationBase.cs
- XamlToRtfParser.cs
- XslVisitor.cs
- ReferenceSchema.cs
- Assert.cs
- ToolBar.cs
- ConnectionProviderAttribute.cs
- HttpProfileBase.cs
- ResourcePermissionBaseEntry.cs
- XslException.cs
- PrincipalPermission.cs
- LicenseContext.cs
- LicenseManager.cs
- Rotation3D.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- RequestQueue.cs
- KeyValuePair.cs
- SimpleWorkerRequest.cs
- PerformanceCountersElement.cs
- IndexerReference.cs
- ProcessThread.cs
- CompilationPass2TaskInternal.cs
- CodeFieldReferenceExpression.cs
- IconHelper.cs
- ToolStripDropDownClosingEventArgs.cs
- XmlSecureResolver.cs
- VBIdentifierTrimConverter.cs
- LambdaCompiler.Logical.cs
- DbFunctionCommandTree.cs
- Path.cs
- counter.cs
- OdbcCommand.cs
- CodeMethodReturnStatement.cs
- HybridWebProxyFinder.cs
- UInt32.cs
- DoubleAnimationUsingKeyFrames.cs
- TransformedBitmap.cs
- ObjectSet.cs
- Parameter.cs
- DataViewSetting.cs
- FieldBuilder.cs
- CodeSubDirectory.cs
- SingleResultAttribute.cs
- DefaultMergeHelper.cs
- RoleGroupCollectionEditor.cs
- _Win32.cs
- FloatMinMaxAggregationOperator.cs
- XPathParser.cs
- ParallelEnumerable.cs
- Size3D.cs
- StsCommunicationException.cs
- MethodRental.cs
- PrinterResolution.cs
- ProvidePropertyAttribute.cs
- initElementDictionary.cs
- CacheOutputQuery.cs
- XmlSchemaAll.cs
- ProcessStartInfo.cs
- UrlRoutingHandler.cs
- InstanceNameConverter.cs
- ExplicitDiscriminatorMap.cs
- ISFTagAndGuidCache.cs
- _ConnectionGroup.cs
- XPathNavigator.cs
- LinqDataSourceDisposeEventArgs.cs
- WebServiceParameterData.cs
- AccessDataSourceView.cs
- InkSerializer.cs
- CatalogZoneDesigner.cs
- ActivityMarkupSerializationProvider.cs
- ObjectDataSourceMethodEventArgs.cs
- FormsAuthenticationCredentials.cs
- ObjectConverter.cs
- DaylightTime.cs
- VSWCFServiceContractGenerator.cs
- ApplicationFileCodeDomTreeGenerator.cs
- GlobalizationAssembly.cs
- SkinBuilder.cs
- EncodingNLS.cs