Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / WebUtil.cs / 1305376 / WebUtil.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Reflection; using System.Runtime.CompilerServices; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif ///web utility functions internal static partial class WebUtil { ////// Whether DataServiceCollection<> type is available. /// private static bool? dataServiceCollectionAvailable = null; ////// Returns true if DataServiceCollection<> type is available or false otherwise. /// private static bool DataServiceCollectionAvailable { get { if (dataServiceCollectionAvailable == null) { try { dataServiceCollectionAvailable = GetDataServiceCollectionOfTType() != null; } catch (FileNotFoundException) { // the assembly or one of its dependencies (read: WindowsBase.dll) was not found. DataServiceCollection is not available. dataServiceCollectionAvailable = false; } } Debug.Assert(dataServiceCollectionAvailable != null, "observableCollectionOfTAvailable must not be null here."); return (bool)dataServiceCollectionAvailable; } } ///copy from one stream to another /// input stream /// output stream /// reusable buffer ///count of copied bytes internal static long CopyStream(Stream input, Stream output, ref byte[] refBuffer) { Debug.Assert(null != input, "null input stream"); Debug.Assert(null != output, "null output stream"); long total = 0; byte[] buffer = refBuffer; if (null == buffer) { refBuffer = buffer = new byte[1000]; } int count = 0; while (input.CanRead && (0 < (count = input.Read(buffer, 0, buffer.Length)))) { output.Write(buffer, 0, count); total += count; } return total; } ///get response object from possible WebException /// exception to probe /// http web respose object from exception internal static void GetHttpWebResponse(InvalidOperationException exception, ref HttpWebResponse response) { if (null == response) { WebException webexception = (exception as WebException); if (null != webexception) { response = (HttpWebResponse)webexception.Response; } } } ///is this a success status code /// status code ///true if status is between 200-299 internal static bool SuccessStatusCode(HttpStatusCode status) { return (200 <= (int)status && (int)status < 300); } ////// turn the response object headers into a dictionary /// /// response ///dictionary internal static DictionaryWrapResponseHeaders(HttpWebResponse response) { Dictionary headers = new Dictionary (EqualityComparer .Default); if (null != response) { foreach (string name in response.Headers.AllKeys) { headers.Add(name, response.Headers[name]); } } return headers; } /// /// Applies headers in the dictionary to a web request. /// This has a special case for some of the headers to set the properties on the request instead of using the Headers collection. /// /// The dictionary with the headers to apply. /// The request to apply the headers to. /// If set to true the Accept header will be ignored /// and the request.Accept property will not be touched. internal static void ApplyHeadersToRequest(Dictionaryheaders, HttpWebRequest request, bool ignoreAcceptHeader) { foreach (KeyValuePair header in headers) { if (string.Equals(header.Key, XmlConstants.HttpRequestAccept, StringComparison.Ordinal)) { if (!ignoreAcceptHeader) { request.Accept = header.Value; } } else if (string.Equals(header.Key, XmlConstants.HttpContentType, StringComparison.Ordinal)) { request.ContentType = header.Value; } else { request.Headers[header.Key] = header.Value; } } } /// /// Checks if the given type is DataServiceCollection<> type. /// /// Type to be checked. ///true if the provided type is DataServiceCollection<> or false otherwise. internal static bool IsDataServiceCollectionType(Type t) { if (DataServiceCollectionAvailable) { return t == GetDataServiceCollectionOfTType(); } return false; } ////// Creates an instance of DataServiceCollection<> class using provided types. /// /// Types to be used for creating DataServiceCollection<> object. ////// Instance of DataServiceCollection<> class created using provided types or null if DataServiceCollection<> /// type is not avaiable. /// internal static Type GetDataServiceCollectionOfT(params Type[] typeArguments) { if (DataServiceCollectionAvailable) { Debug.Assert( GetDataServiceCollectionOfTType() != null, "DataServiceCollection is available so GetDataServiceCollectionOfTType() must not return null."); return GetDataServiceCollectionOfTType().MakeGenericType(typeArguments); } return null; } ////// Forces loading WindowsBase assembly. If WindowsBase assembly is not present JITter will throw an exception. /// This method MUST NOT be inlined otherwise we won't be able to catch the exception by JITter in the caller. /// ///typeof(DataServiceCollection<>) [MethodImpl(MethodImplOptions.NoInlining)] private static Type GetDataServiceCollectionOfTType() { return typeof(DataServiceCollection<>); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
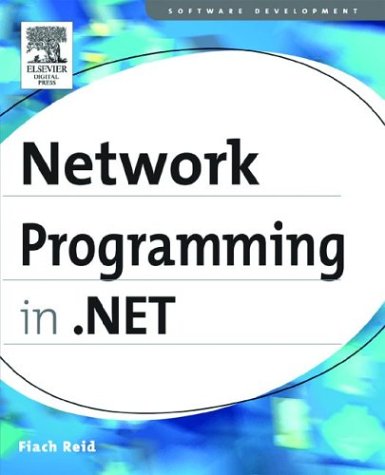
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParenthesizePropertyNameAttribute.cs
- Columns.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- Pair.cs
- TemplatePagerField.cs
- KnowledgeBase.cs
- VerticalAlignConverter.cs
- UshortList2.cs
- RepeaterItemCollection.cs
- BamlVersionHeader.cs
- MenuItemAutomationPeer.cs
- WindowsListViewItemStartMenu.cs
- SimpleMailWebEventProvider.cs
- MediaTimeline.cs
- TextServicesCompartmentContext.cs
- SqlClientMetaDataCollectionNames.cs
- InternalMappingException.cs
- BuildProviderAppliesToAttribute.cs
- QuaternionIndependentAnimationStorage.cs
- BindingsCollection.cs
- HtmlGenericControl.cs
- EventLogTraceListener.cs
- Events.cs
- CodeTypeDelegate.cs
- BamlStream.cs
- CompositeActivityTypeDescriptor.cs
- Scripts.cs
- QueryableDataSource.cs
- ApplicationDirectoryMembershipCondition.cs
- PasswordRecovery.cs
- designeractionlistschangedeventargs.cs
- ZoneIdentityPermission.cs
- QfeChecker.cs
- ServiceSecurityAuditBehavior.cs
- UnsafeNativeMethods.cs
- ListViewItem.cs
- ScriptingProfileServiceSection.cs
- OperatingSystem.cs
- XPathNode.cs
- AdornerPresentationContext.cs
- TraceRecords.cs
- NativeActivityMetadata.cs
- SHA256Cng.cs
- BinaryObjectReader.cs
- AsyncPostBackErrorEventArgs.cs
- ResourceExpressionEditorSheet.cs
- initElementDictionary.cs
- ConfigXmlComment.cs
- DataControlFieldCollection.cs
- Attributes.cs
- TemplateBamlRecordReader.cs
- BasicHttpBinding.cs
- XmlSchemaObjectTable.cs
- Annotation.cs
- SmtpNegotiateAuthenticationModule.cs
- PathFigureCollectionConverter.cs
- MeasurementDCInfo.cs
- ParameterEditorUserControl.cs
- XmlSerializer.cs
- DataControlFieldCollection.cs
- PieceNameHelper.cs
- BamlBinaryReader.cs
- Highlights.cs
- JsonReaderDelegator.cs
- AesCryptoServiceProvider.cs
- XmlDocumentFragment.cs
- ToolStripMenuItem.cs
- _ListenerAsyncResult.cs
- XmlSchemaInfo.cs
- DropSource.cs
- PageEventArgs.cs
- EntityViewGenerationConstants.cs
- TextContainerHelper.cs
- TimeoutValidationAttribute.cs
- NativeMethods.cs
- EncryptedData.cs
- Rotation3DKeyFrameCollection.cs
- WebRequestModuleElementCollection.cs
- PageClientProxyGenerator.cs
- EntityTypeEmitter.cs
- FieldBuilder.cs
- ComPlusServiceHost.cs
- MatrixConverter.cs
- SegmentInfo.cs
- PolyBezierSegment.cs
- SafeLibraryHandle.cs
- ToolStripDropDownClosedEventArgs.cs
- AuthenticationSection.cs
- FileDialogCustomPlacesCollection.cs
- FilterInvalidBodyAccessException.cs
- TemplateGroupCollection.cs
- BezierSegment.cs
- HyperLinkDataBindingHandler.cs
- Roles.cs
- ProxyElement.cs
- XhtmlBasicPhoneCallAdapter.cs
- KeySpline.cs
- CngAlgorithmGroup.cs
- Win32PrintDialog.cs
- OdbcStatementHandle.cs