Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Security / Permissions / ZoneIdentityPermission.cs / 1 / ZoneIdentityPermission.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // ZoneIdentityPermission.cs // namespace System.Security.Permissions { using System; using SecurityElement = System.Security.SecurityElement; using System.Globalization; using System.Runtime.Serialization; [System.Runtime.InteropServices.ComVisible(true)] [Serializable()] sealed public class ZoneIdentityPermission : CodeAccessPermission, IBuiltInPermission { //------------------------------------------------------ // // PRIVATE STATE DATA // //----------------------------------------------------- // Zone Enum Flag // ----- ----- ----- // NoZone -1 0x00 // MyComputer 0 0x01 (1 << 0) // Intranet 1 0x02 (1 << 1) // Trusted 2 0x04 (1 << 2) // Internet 3 0x08 (1 << 3) // Untrusted 4 0x10 (1 << 4) private const uint AllZones = 0x1f; [OptionalField(VersionAdded = 2)] private uint m_zones; // This field will be populated only for non X-AD scenarios where we create a XML-ised string of the Permission [OptionalField(VersionAdded = 2)] private String m_serializedPermission; // This field is legacy info from v1.x and is never used in v2.0 and beyond: purely for serialization purposes private SecurityZone m_zone = SecurityZone.NoZone; [OnDeserialized] private void OnDeserialized(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { // v2.0 and beyond XML case if (m_serializedPermission != null) { FromXml(SecurityElement.FromString(m_serializedPermission)); m_serializedPermission = null; } else //v1.x case where we read the m_zone value { SecurityZone = m_zone; m_zone = SecurityZone.NoZone; } } } [OnSerializing] private void OnSerializing(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { m_serializedPermission = ToXml().ToString(); //for the v2 and beyond case m_zone = SecurityZone; } } [OnSerialized] private void OnSerialized(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { m_serializedPermission = null; m_zone = SecurityZone.NoZone; } } //----------------------------------------------------- // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- public ZoneIdentityPermission(PermissionState state) { if (state == PermissionState.Unrestricted) { if(CodeAccessSecurityEngine.DoesFullTrustMeanFullTrust()) m_zones = AllZones; else throw new ArgumentException(Environment.GetResourceString("Argument_UnrestrictedIdentityPermission")); } else if (state == PermissionState.None) { m_zones = 0; } else { throw new ArgumentException(Environment.GetResourceString("Argument_InvalidPermissionState")); } } public ZoneIdentityPermission( SecurityZone zone ) { this.SecurityZone = zone; } internal ZoneIdentityPermission( uint zones ) { m_zones = (zones & AllZones); } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //----------------------------------------------------- public SecurityZone SecurityZone { set { VerifyZone( value ); if(value == SecurityZone.NoZone) m_zones = 0; else m_zones = (uint)1 << (int)value; } get { SecurityZone z = SecurityZone.NoZone; int nEnum = 0; uint nFlag; for(nFlag = 1; nFlag < AllZones; nFlag <<= 1) { if((m_zones & nFlag) != 0) { if(z == SecurityZone.NoZone) z = (SecurityZone)nEnum; else return SecurityZone.NoZone; } nEnum++; } return z; } } //------------------------------------------------------ // // PRIVATE AND PROTECTED HELPERS FOR ACCESSORS AND CONSTRUCTORS // //------------------------------------------------------ private static void VerifyZone( SecurityZone zone ) { if (zone < SecurityZone.NoZone || zone > SecurityZone.Untrusted) { throw new ArgumentException( Environment.GetResourceString("Argument_IllegalZone") ); } } //----------------------------------------------------- // // CODEACCESSPERMISSION IMPLEMENTATION // //------------------------------------------------------ //----------------------------------------------------- // // IPERMISSION IMPLEMENTATION // //----------------------------------------------------- public override IPermission Copy() { return new ZoneIdentityPermission(this.m_zones); } public override bool IsSubsetOf(IPermission target) { if (target == null) return this.m_zones == 0; ZoneIdentityPermission that = target as ZoneIdentityPermission; if (that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); return (this.m_zones & that.m_zones) == this.m_zones; } public override IPermission Intersect(IPermission target) { if (target == null) return null; ZoneIdentityPermission that = target as ZoneIdentityPermission; if (that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); uint newZones = this.m_zones & that.m_zones; if(newZones == 0) return null; return new ZoneIdentityPermission(newZones); } public override IPermission Union(IPermission target) { if (target == null) return this.m_zones != 0 ? this.Copy() : null; ZoneIdentityPermission that = target as ZoneIdentityPermission; if (that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); return new ZoneIdentityPermission(this.m_zones | that.m_zones); } public override SecurityElement ToXml() { SecurityElement esd = CodeAccessPermission.CreatePermissionElement( this, "System.Security.Permissions.ZoneIdentityPermission" ); if (SecurityZone != SecurityZone.NoZone) { esd.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), this.SecurityZone ) ); } else { int nEnum = 0; uint nFlag; for(nFlag = 1; nFlag < AllZones; nFlag <<= 1) { if((m_zones & nFlag) != 0) { SecurityElement child = new SecurityElement("Zone"); child.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), (SecurityZone)nEnum ) ); esd.AddChild(child); } nEnum++; } } return esd; } public override void FromXml(SecurityElement esd) { m_zones = 0; CodeAccessPermission.ValidateElement( esd, this ); String eZone = esd.Attribute( "Zone" ); if (eZone != null) SecurityZone = (SecurityZone)Enum.Parse( typeof( SecurityZone ), eZone ); if(esd.Children != null) { foreach(SecurityElement child in esd.Children) { eZone = child.Attribute( "Zone" ); int enm = (int)Enum.Parse( typeof( SecurityZone ), eZone ); if(enm == (int)SecurityZone.NoZone) continue; m_zones |= ((uint)1 << enm); } } } ///int IBuiltInPermission.GetTokenIndex() { return ZoneIdentityPermission.GetTokenIndex(); } internal static int GetTokenIndex() { return BuiltInPermissionIndex.ZoneIdentityPermissionIndex; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // ZoneIdentityPermission.cs // namespace System.Security.Permissions { using System; using SecurityElement = System.Security.SecurityElement; using System.Globalization; using System.Runtime.Serialization; [System.Runtime.InteropServices.ComVisible(true)] [Serializable()] sealed public class ZoneIdentityPermission : CodeAccessPermission, IBuiltInPermission { //------------------------------------------------------ // // PRIVATE STATE DATA // //----------------------------------------------------- // Zone Enum Flag // ----- ----- ----- // NoZone -1 0x00 // MyComputer 0 0x01 (1 << 0) // Intranet 1 0x02 (1 << 1) // Trusted 2 0x04 (1 << 2) // Internet 3 0x08 (1 << 3) // Untrusted 4 0x10 (1 << 4) private const uint AllZones = 0x1f; [OptionalField(VersionAdded = 2)] private uint m_zones; // This field will be populated only for non X-AD scenarios where we create a XML-ised string of the Permission [OptionalField(VersionAdded = 2)] private String m_serializedPermission; // This field is legacy info from v1.x and is never used in v2.0 and beyond: purely for serialization purposes private SecurityZone m_zone = SecurityZone.NoZone; [OnDeserialized] private void OnDeserialized(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { // v2.0 and beyond XML case if (m_serializedPermission != null) { FromXml(SecurityElement.FromString(m_serializedPermission)); m_serializedPermission = null; } else //v1.x case where we read the m_zone value { SecurityZone = m_zone; m_zone = SecurityZone.NoZone; } } } [OnSerializing] private void OnSerializing(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { m_serializedPermission = ToXml().ToString(); //for the v2 and beyond case m_zone = SecurityZone; } } [OnSerialized] private void OnSerialized(StreamingContext ctx) { if ((ctx.State & ~(StreamingContextStates.Clone|StreamingContextStates.CrossAppDomain)) != 0) { m_serializedPermission = null; m_zone = SecurityZone.NoZone; } } //----------------------------------------------------- // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- public ZoneIdentityPermission(PermissionState state) { if (state == PermissionState.Unrestricted) { if(CodeAccessSecurityEngine.DoesFullTrustMeanFullTrust()) m_zones = AllZones; else throw new ArgumentException(Environment.GetResourceString("Argument_UnrestrictedIdentityPermission")); } else if (state == PermissionState.None) { m_zones = 0; } else { throw new ArgumentException(Environment.GetResourceString("Argument_InvalidPermissionState")); } } public ZoneIdentityPermission( SecurityZone zone ) { this.SecurityZone = zone; } internal ZoneIdentityPermission( uint zones ) { m_zones = (zones & AllZones); } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //----------------------------------------------------- public SecurityZone SecurityZone { set { VerifyZone( value ); if(value == SecurityZone.NoZone) m_zones = 0; else m_zones = (uint)1 << (int)value; } get { SecurityZone z = SecurityZone.NoZone; int nEnum = 0; uint nFlag; for(nFlag = 1; nFlag < AllZones; nFlag <<= 1) { if((m_zones & nFlag) != 0) { if(z == SecurityZone.NoZone) z = (SecurityZone)nEnum; else return SecurityZone.NoZone; } nEnum++; } return z; } } //------------------------------------------------------ // // PRIVATE AND PROTECTED HELPERS FOR ACCESSORS AND CONSTRUCTORS // //------------------------------------------------------ private static void VerifyZone( SecurityZone zone ) { if (zone < SecurityZone.NoZone || zone > SecurityZone.Untrusted) { throw new ArgumentException( Environment.GetResourceString("Argument_IllegalZone") ); } } //----------------------------------------------------- // // CODEACCESSPERMISSION IMPLEMENTATION // //------------------------------------------------------ //----------------------------------------------------- // // IPERMISSION IMPLEMENTATION // //----------------------------------------------------- public override IPermission Copy() { return new ZoneIdentityPermission(this.m_zones); } public override bool IsSubsetOf(IPermission target) { if (target == null) return this.m_zones == 0; ZoneIdentityPermission that = target as ZoneIdentityPermission; if (that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); return (this.m_zones & that.m_zones) == this.m_zones; } public override IPermission Intersect(IPermission target) { if (target == null) return null; ZoneIdentityPermission that = target as ZoneIdentityPermission; if (that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); uint newZones = this.m_zones & that.m_zones; if(newZones == 0) return null; return new ZoneIdentityPermission(newZones); } public override IPermission Union(IPermission target) { if (target == null) return this.m_zones != 0 ? this.Copy() : null; ZoneIdentityPermission that = target as ZoneIdentityPermission; if (that == null) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Argument_WrongType"), this.GetType().FullName)); return new ZoneIdentityPermission(this.m_zones | that.m_zones); } public override SecurityElement ToXml() { SecurityElement esd = CodeAccessPermission.CreatePermissionElement( this, "System.Security.Permissions.ZoneIdentityPermission" ); if (SecurityZone != SecurityZone.NoZone) { esd.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), this.SecurityZone ) ); } else { int nEnum = 0; uint nFlag; for(nFlag = 1; nFlag < AllZones; nFlag <<= 1) { if((m_zones & nFlag) != 0) { SecurityElement child = new SecurityElement("Zone"); child.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), (SecurityZone)nEnum ) ); esd.AddChild(child); } nEnum++; } } return esd; } public override void FromXml(SecurityElement esd) { m_zones = 0; CodeAccessPermission.ValidateElement( esd, this ); String eZone = esd.Attribute( "Zone" ); if (eZone != null) SecurityZone = (SecurityZone)Enum.Parse( typeof( SecurityZone ), eZone ); if(esd.Children != null) { foreach(SecurityElement child in esd.Children) { eZone = child.Attribute( "Zone" ); int enm = (int)Enum.Parse( typeof( SecurityZone ), eZone ); if(enm == (int)SecurityZone.NoZone) continue; m_zones |= ((uint)1 << enm); } } } /// int IBuiltInPermission.GetTokenIndex() { return ZoneIdentityPermission.GetTokenIndex(); } internal static int GetTokenIndex() { return BuiltInPermissionIndex.ZoneIdentityPermissionIndex; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
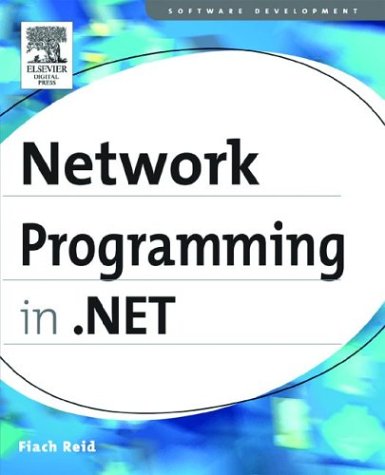
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourcePropertyMemberCodeDomSerializer.cs
- SafeLocalAllocation.cs
- TagPrefixInfo.cs
- StorageMappingItemCollection.cs
- OdbcConnection.cs
- CanExecuteRoutedEventArgs.cs
- AlgoModule.cs
- WmfPlaceableFileHeader.cs
- UriExt.cs
- OleDbStruct.cs
- ReferenceConverter.cs
- MatchAttribute.cs
- UpdatePanelTrigger.cs
- GiveFeedbackEvent.cs
- EntityDataSourceDesignerHelper.cs
- DbProviderServices.cs
- ConfigurationProperty.cs
- FormatterConverter.cs
- DictionaryCustomTypeDescriptor.cs
- PointUtil.cs
- ImageInfo.cs
- CodeParameterDeclarationExpression.cs
- MergeFailedEvent.cs
- SqlDataSourceSelectingEventArgs.cs
- NetworkInterface.cs
- DateTimeOffsetAdapter.cs
- Vector.cs
- Cursor.cs
- MonikerSyntaxException.cs
- COAUTHIDENTITY.cs
- EdmToObjectNamespaceMap.cs
- ObservableDictionary.cs
- PromptBuilder.cs
- EntityWrapper.cs
- UrlPropertyAttribute.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- MailMessageEventArgs.cs
- SqlParameterCollection.cs
- StringValidator.cs
- ErrorHandler.cs
- ServiceProviders.cs
- Range.cs
- COM2Properties.cs
- BulletedListEventArgs.cs
- activationcontext.cs
- SiteMapNodeItemEventArgs.cs
- BasicHttpSecurityElement.cs
- PassportPrincipal.cs
- ProtectedProviderSettings.cs
- BufferedOutputStream.cs
- RIPEMD160.cs
- HotSpotCollection.cs
- EndEvent.cs
- XmlSchemaAttributeGroupRef.cs
- _NestedSingleAsyncResult.cs
- SystemWebExtensionsSectionGroup.cs
- OracleParameterBinding.cs
- SessionState.cs
- assemblycache.cs
- RenderOptions.cs
- DataGridAutomationPeer.cs
- GeometryConverter.cs
- TextDecorationUnitValidation.cs
- CrossContextChannel.cs
- SourceFileBuildProvider.cs
- ClassData.cs
- GridViewCellAutomationPeer.cs
- CalculatedColumn.cs
- XmlSchemaObjectCollection.cs
- DataGridViewCellLinkedList.cs
- ViewValidator.cs
- TrustSection.cs
- Utility.cs
- XmlSchemaObjectCollection.cs
- SafeTokenHandle.cs
- MailAddress.cs
- ZipIOModeEnforcingStream.cs
- WindowsRichEditRange.cs
- RoutedEvent.cs
- ConfigurationException.cs
- InvokeFunc.cs
- StrokeNode.cs
- EntitySqlException.cs
- SerializationSectionGroup.cs
- GenericTextProperties.cs
- UInt32.cs
- UnknownBitmapEncoder.cs
- ReadOnlyDataSourceView.cs
- DispatcherFrame.cs
- ApplicationCommands.cs
- GPStream.cs
- ValidatingReaderNodeData.cs
- ResourcePart.cs
- GuidTagList.cs
- HandlerMappingMemo.cs
- Span.cs
- DataObjectMethodAttribute.cs
- MenuTracker.cs
- WindowsGraphicsWrapper.cs
- FontStyles.cs