Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / Adorner.cs / 1305600 / Adorner.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Info needed to draw decorations on objects // // See spec at http://avalon/uis/Specs/AdornerLayer%20Spec.htm // // History: // 10/01/2003 : psarrett - Created // 10/22/2003 : psarrett - modified as per current spec // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows.Data; using System.Windows.Media; using System.Windows.Input; using System.Windows.Threading; using MS.Internal; // DoubleUtil namespace System.Windows.Documents { ////// An Adorner is a UIElement "attached" to another UIElement. Adorners render /// on an AdornerLayer, which puts them higher in the Z-order than the element /// to which they are attached so they visually appear on top of that element. /// By default, the AdornerLayer positions an Adorner at the upper-left corner /// of the element it adorns. However, the AdornerLayer passes the Adorner its /// proposed transform, and the Adorner can modify that proposed transform as it /// wishes. /// /// Since Adorners are UIElements, they can respond to input events. /// public abstract class Adorner : FrameworkElement { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// protected Adorner(UIElement adornedElement) { if (adornedElement == null) throw new ArgumentNullException("adornedElement"); _adornedElement = adornedElement; _isClipEnabled = false; // Bug 1383424: We need to make sure our FlowDirection is always that of our adorned element. // Need to allow derived class constructor to execute first Dispatcher.CurrentDispatcher.BeginInvoke(DispatcherPriority.Normal, new DispatcherOperationCallback(CreateFlowDirectionBinding), this); } #endregion Constructors //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// Measure adorner. Default behavior is to size to match the adorned element. /// protected override Size MeasureOverride(Size constraint) { Size desiredSize; desiredSize = new Size(AdornedElement.RenderSize.Width, AdornedElement.RenderSize.Height); int count = this.VisualChildrenCount; for (int i = 0; i < count; i++) { UIElement ch = this.GetVisualChild(i) as UIElement; if (ch != null) { ch.Measure(desiredSize); } } return desiredSize; } ////// Override of ///. /// null ////// Clip gets generated before transforms are applied, which means that /// Adorners can get inappropriately clipped if they draw outside of the bounding rect /// of the element they're adorning. This is against the Adorner philosophy of being /// topmost, so we choose to ignore clip instead. protected override Geometry GetLayoutClip(Size layoutSlotSize) { return null; } #endregion Protected Methods //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Adorners don't always want to be transformed in the same way as the elements they /// adorn. Adorners which adorn points, such as resize handles, want to be translated /// and rotated but not scaled. Adorners adorning an object, like a marquee, may want /// all transforms. This method is called by AdornerLayer to allow the adorner to /// filter out the transforms it doesn't want and return a new transform with just the /// transforms it wants applied. An adorner can also add an additional translation /// transform at this time, allowing it to be positioned somewhere other than the upper /// left corner of its adorned element. /// /// The transform applied to the object the adorner adorns ///Transform to apply to the adorner public virtual GeneralTransform GetDesiredTransform(GeneralTransform transform) { return transform; } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Gets or sets the clip of this Visual. /// Needed by AdornerLayer /// internal Geometry AdornerClip { get { return Clip; } set { Clip = value; } } ////// Gets or sets the transform of this Visual. /// Needed by AdornerLayer /// internal Transform AdornerTransform { get { return RenderTransform; } set { RenderTransform = value; } } ////// UIElement this Adorner adorns. /// public UIElement AdornedElement { get { return _adornedElement; } } ////// If set to true, the adorner will be clipped using the same clip geometry as the /// AdornedElement. This is expensive, and therefore should not normally be used. /// Defaults to false. /// public bool IsClipEnabled { get { return _isClipEnabled; } set { _isClipEnabled = value; InvalidateArrange(); AdornerLayer.GetAdornerLayer(_adornedElement).InvalidateArrange(); } } #endregion Public Properties //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- #region Private Methods // Callback for binding the FlowDirection property. private static object CreateFlowDirectionBinding(object o) { Adorner adorner = (Adorner)o; Binding binding = new Binding("FlowDirection"); binding.Mode = BindingMode.OneWay; binding.Source = adorner.AdornedElement; adorner.SetBinding(FlowDirectionProperty, binding); return null; } ////// Says if the Adorner needs update based on the /// previously cached size if the AdornedElement. /// internal virtual bool NeedsUpdate(Size oldSize) { return !DoubleUtil.AreClose(AdornedElement.RenderSize, oldSize); } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private readonly UIElement _adornedElement; private bool _isClipEnabled; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
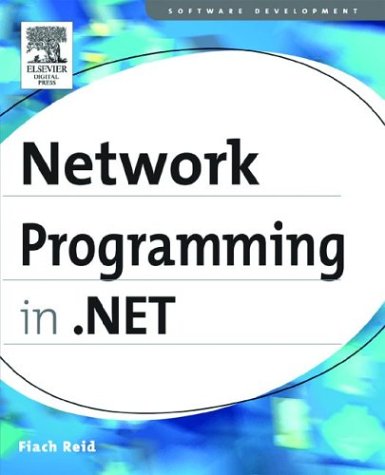
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlNotation.cs
- DoubleIndependentAnimationStorage.cs
- BitmapDownload.cs
- PropertyChangedEventManager.cs
- Style.cs
- UnionExpr.cs
- ItemDragEvent.cs
- BamlResourceSerializer.cs
- IPEndPointCollection.cs
- GrowingArray.cs
- ToolZone.cs
- ToolBarPanel.cs
- ObservableCollection.cs
- FixUp.cs
- Color.cs
- ThreadInterruptedException.cs
- EventLogPermissionEntryCollection.cs
- MultiBindingExpression.cs
- SqlRemoveConstantOrderBy.cs
- FatalException.cs
- DbExpressionRules.cs
- Converter.cs
- TypedTableBase.cs
- ConditionCollection.cs
- WebPartConnectionsEventArgs.cs
- StatusBarItemAutomationPeer.cs
- FtpCachePolicyElement.cs
- DataControlFieldHeaderCell.cs
- ColumnPropertiesGroup.cs
- DeferredElementTreeState.cs
- Polyline.cs
- HtmlShimManager.cs
- WebConfigManager.cs
- ObjectDesignerDataSourceView.cs
- ClientSettingsStore.cs
- SizeConverter.cs
- ValidateNames.cs
- CorrelationTokenTypeConvertor.cs
- TextEndOfLine.cs
- PriorityChain.cs
- WindowsFormsHelpers.cs
- GenerateTemporaryTargetAssembly.cs
- SchemaTableOptionalColumn.cs
- RegistryKey.cs
- SinglePageViewer.cs
- LayoutTable.cs
- SingleObjectCollection.cs
- ViewKeyConstraint.cs
- XmlSchemaComplexContentRestriction.cs
- Compilation.cs
- ListViewItem.cs
- TransactionFlowAttribute.cs
- ApplicationBuildProvider.cs
- XPathNodePointer.cs
- PathFigureCollection.cs
- FileFormatException.cs
- ToolStripDropDownItem.cs
- XmlSchemaValidationException.cs
- CLSCompliantAttribute.cs
- HttpHandler.cs
- TextRangeProviderWrapper.cs
- HtmlInputControl.cs
- EntityDataSourceValidationException.cs
- SkinBuilder.cs
- DataServiceQueryException.cs
- BitVector32.cs
- externdll.cs
- WebConfigurationManager.cs
- GridViewRowPresenter.cs
- ContextQuery.cs
- _ListenerAsyncResult.cs
- FlowDocument.cs
- TokenBasedSet.cs
- Timeline.cs
- ControlsConfig.cs
- HtmlLabelAdapter.cs
- BamlRecords.cs
- DesignerAutoFormat.cs
- xml.cs
- __ConsoleStream.cs
- sqlstateclientmanager.cs
- StdValidatorsAndConverters.cs
- ServiceDesigner.xaml.cs
- ResourceSet.cs
- NameValuePermission.cs
- DataFormats.cs
- PassportPrincipal.cs
- TextMessageEncodingElement.cs
- ToolStripDropDownClosingEventArgs.cs
- TextTreeTextElementNode.cs
- XmlBinaryReader.cs
- DataGridAddNewRow.cs
- FamilyTypeface.cs
- UnsafeNativeMethodsPenimc.cs
- DbProviderFactory.cs
- CollectionCodeDomSerializer.cs
- AutomationPropertyInfo.cs
- DefaultObjectSerializer.cs
- KeyPressEvent.cs
- SoapExtension.cs